Carousel
Overview
Carousel is a good way to show multiple contents in one place by alterning between them via a navigation-like.
Best example is gallery photos from ecommerce product detail.
JSXPine's Carousel provide properties which will enabled you to deal with many customization cases. Here below are some examples of what you can achieved with this component:
Installation
Notes
To use this component, you need to initialize your project first. If not done yet, run one of the following command:
npx jsxpine init or yarn jsxpine init or pnpm jsxpine init or bunx jsxpine init.
Go to the installation and usage page to learn more.
jsxpine add carousel
Copied !
-------------------- Components Dependencies -------------------------
import clsx from "clsx";
/**
* @typedef HTMLButtonTagWithChildren
* @type {import("@kitajs/html").PropsWithChildren<Omit<JSX.HtmlButtonTag, "className"> & import("../common/props").CLSXClassProps>}
*/
/**
* @typedef {Object} ButtonProps
* @type {{ text?: string } & HTMLButtonTagWithChildren & import("../common/props").BorderRadiusProps & import("../common/props").SizeProps & import("../common/props").VariantColorProps}
*/
/**
* Button component props
* @param {ButtonProps} props
*/
export function Button({
children,
class: className,
size = "md",
text,
variant = "solid",
borderRadius = "rounded",
...restProps
}) {
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantColorMap = new Map([
["solid", "btn"],
["outlined", "btn-outlined"],
["inversed", "btn-inversed"]
]);
/**
* @type {Map<import("../common/types").SizeType, string>}
*/
const sizeMap = new Map([
["xs", "text-xs px-2 py-1"],
["sm", "px-4 py-2 text-sm"],
["md", "px-4 py-2"],
["lg", "px-8 py-4 text-lg"],
["xl", "px-12 py-6 text-xl"],
["2xl", "px-16 py-8 text-4xl"]
]);
/**
* @type {Map<import("../common/types").BorderRadiusType, string>}
*/
const borderRadiusMap = new Map([
["square", "rounded-none"],
["rounded", "rounded"],
["arc", "rounded-xl"],
["pill", "rounded-full"],
["curve", "rounded-lg"],
["circle", "aspect-square rounded-full"]
]);
return (
<button
class={clsx(
variantColorMap.get(variant),
sizeMap.get(size),
borderRadiusMap.get(borderRadius),
className
)}
{...restProps}
>
{text ? <span safe>{text}</span> : children}
</button>
);
}
/**
* Primary Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function PrimaryButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-primary"],
["outlined", "btn-primary-outlined"],
["inversed", "btn-primary-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
/**
* Secondary Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function SecondaryButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-secondary"],
["outlined", "btn-secondary-outlined"],
["inversed", "btn-secondary-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
/**
* Success Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function SuccessButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-success"],
["outlined", "btn-success-outlined"],
["inversed", "btn-success-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
/**
* Danger Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function DangerButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-danger"],
["outlined", "btn-danger-outlined"],
["inversed", "btn-danger-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
/**
* Info Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function InfoButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-info"],
["outlined", "btn-info-outlined"],
["inversed", "btn-info-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
/**
* Warning Button component props
* @type {import("../common/props").JSXComponent<ButtonProps>}
*/
export function WarningButton(props) {
const { children, class: className, variant = "solid", ...restProps } = props;
/**
* @type {Map<import("../common/types").VariantColorType, string>}
*/
const variantClassMap = new Map([
["solid", "btn-warning"],
["outlined", "btn-warning-outlined"],
["inversed", "btn-warning-inversed"]
]);
return (
<Button
class={[variantClassMap.get(variant), className].join(" ")}
{...restProps}
>
{children}
</Button>
);
}
import { icons } from "@iconify-json/ri/icons.json";
import clsx from "clsx";
import { SVG } from "./svg.component";
/**
* @typedef {`ri.${keyof typeof import("@iconify-json/ri/icons.json")["icons"]}`} IconName
*/
/**
* @typedef IconProps
* @type {{size?: number, name: IconName, color?: string, applyDefsId?: string} & import("./svg.component").SVGProps}
*/
/**
* Icon component props
* @param {Omit<IconProps, "viewBox">} props
*/
export function Icon({
children,
size = 4,
name,
applyDefsId,
class: className,
...restProps
}) {
const iconType = name.split(".")[0];
const iconName = /** @type {keyof typeof icons} */ (name.split(".")[1]);
if (!icons[iconName]) {
console.error(`"${name}" is not an icon from iconify/${iconType}`);
return "";
}
const { body } = icons[iconName];
// The purpose is to retrieve value from d attribute
let retrieveDValue = "";
const bodyMatch = body.match(/d=".+"/g);
const retrieveDAttribute = bodyMatch ? bodyMatch[0] : "";
if (retrieveDAttribute) {
retrieveDValue = retrieveDAttribute.slice(3, -2);
}
return (
<SVG
viewBox={"0 0 24 24"}
{...restProps}
class={clsx(`size-${size}`, className)}
>
{children}
<path
stroke-linecap="round"
stroke-linejoin="round"
fill={applyDefsId ? `url(#${applyDefsId})` : "currentColor"}
d={retrieveDValue}
/>
</SVG>
);
}
/**
* @typedef SVGProps
* @type {{fill?: string, stroke?: string, strokeWidth?: number, viewBox: string } & Omit<JSX.HtmlTag, "className"> & import("../common/props").CLSXClassProps}
*/
/**
* SVG component props
* @type {import("../common/props").JSXComponent<SVGProps>}
*/
export function SVG(props) {
const {
children,
class: className,
fill = "none",
stroke = "currentColor",
strokeWidth = 0.5,
...restProps
} = props;
return (
<svg
xmlns="http://www.w3.org/2000/svg"
fill={fill}
stroke={stroke}
class={className}
style={{ strokeWidth: String(strokeWidth) }}
{...restProps}
>
{children}
</svg>
)
}
/**
* @typedef {Object} ImageType
* @property {string} src
* @property {string} [alt]
*/
/**
* @typedef ImageProps
* @type {Omit<JSX.HtmlImageTag, "className" | "loading"> & import("../common/props").CLSXClassProps}
*/
/**
* Image Component props
* @type {import("../common/props").JSXComponent<ImageProps>}
*/
export function Image(props) {
const { ...restProps } = props;
return (
<img {...{ ...restProps, loading: "lazy" }} />
)
}
-------------------- Carousel Component -------------------------
import { Button } from "./button.component";
import { Icon } from "./icon.component";
import { Image } from "./image.component";
import clsx from "clsx";
/**
* @typedef CarouselNavigationProps
* @property {import("../common/types").DirectionType} direction
*/
/**
* @typedef CarouselIndicatorsProps
* @type {import("../common/props").HTMLTagWithChildren & { indicator?: boolean | import("../common/types").PositionType }}
*/
/**
* @typedef CarouselProps
* @type {{ slides?: import("./image.component").ImageType[], loop?: boolean, indicator?: boolean | import("../common/types").PositionType, direction?: import("../common/types").DirectionType, hideNavigations?: boolean, slidesToShow?: number, navigations?: JSX.Element, indicators?: JSX.Element } & import("../common/props").HTMLTag}
*/
/**
* Carousel Navigation component props
* @type {import("../common/props").JSXComponent<CarouselNavigationProps>}
*/
export function CarouselNavigations(props) {
const { direction } = props;
/**
* @type {Map<import("../common/types").DirectionType, { previous: string; next: string }>}
*/
const navigationButtonDirectionClassMap = new Map([
[
"horizontal",
{
previous: "top-1/2 -translate-y-1/2 left-4",
next: "top-1/2 -translate-y-1/2 right-4"
}
],
[
"vertical",
{
previous: "left-1/2 -translate-x-1/2 top-4",
next: "left-1/2 -translate-x-1/2 bottom-4"
}
]
]);
/**
* @type {Map<import("../common/types").DirectionType, { previous: import("../common/types").IconName; next: import("../common/types").IconName }>}
*/
const navigationIconDirectionClassMap = new Map([
[
"horizontal",
{ previous: "ri.arrow-left-s-line", next: "ri.arrow-right-s-line" }
],
[
"vertical",
{ previous: "ri.arrow-up-s-line", next: "ri.arrow-down-s-line" }
]
]);
const navIcon = navigationIconDirectionClassMap.get(direction);
return (
<>
<Button
x-bind:disabled="!loop && areFirstSlidesToShow()"
x-on:click="previous()"
borderRadius="circle"
class={`absolute ${
navigationButtonDirectionClassMap.get(direction)?.previous
}`}
>
{navIcon ? (
<Icon
name={navIcon.previous}
size={6}
fill="none"
stroke="currentColor"
/>
) : (
""
)}
</Button>
<Button
x-bind:disabled="!loop && areLastSlidesToShow()"
x-on:click="next()"
borderRadius="circle"
class={`absolute ${
navigationButtonDirectionClassMap.get(direction)?.next
}`}
>
{navIcon ? (
<Icon
name={navIcon.next}
size={6}
fill="none"
stroke="currentColor"
/>
) : (
""
)}
</Button>
</>
);
}
/**
* Carousel Indicators component props
* @type {import("../common/props").JSXComponent<CarouselIndicatorsProps>}
*/
export function CarouselIndicators(props) {
const { children, indicator } = props;
/**
* @type {Map<import("../common/types").PositionType, string>}
*/
const indicatorPositionClassMap = new Map([
["top", "top-0 left-1/2 -translate-x-1/2 gap-x-2"],
["bottom", "bottom-0 left-1/2 -translate-x-1/2 gap-x-2"],
["left", "left-0 top-1/2 -translate-y-1/2 flex-col gap-y-2"],
["right", "right-0 top-1/2 -translate-y-1/2 flex-col gap-y-2"]
]);
return (
<ul
class={`absolute flex ${
indicator && typeof indicator !== "boolean"
? indicatorPositionClassMap.get(indicator)
: indicatorPositionClassMap.get("bottom")
} p-4`}
>
{children ?? (
<template x-for="(indicator, index) in $refs.carousel.children">
<li>
<button
x-on:click="setActiveIndex(Number(index))"
x-bind:disabled="isActive(Number(index))"
class="cursor-pointer p-2 rounded-full btn"
></button>
</li>
</template>
)}
</ul>
);
}
/**
* Carousel component props
* @type {import("../common/props").JSXComponent<CarouselProps>}
*/
export function Carousel(props) {
const {
children,
slides,
class: className,
loop = false,
indicator = false,
direction = "horizontal",
hideNavigations = false,
slidesToShow = 1,
navigations,
indicators,
...restProps
} = props;
/**
* @type {Map<import("../common/types").DirectionType, string>}
*/
const directionClassMap = new Map([
["horizontal", "flex items-center"],
[
"vertical",
slidesToShow > 1 ? "flex flex-col items-center" : "grid grid-flow-dense"
]
]);
/**
* @type {Map<import("../common/types").DirectionType, string>}
*/
const slideDirectionClassMap = new Map([
["horizontal", slidesToShow > 1 ? "" : "min-w-full h-full"],
["vertical", slidesToShow > 1 ? "" : "min-w-full h-[inherit]"]
]);
const slideDirectionDimensionStyle =
direction === "horizontal"
? `width: ${100 / slidesToShow}%; height: 100%;`
: `width: 100%; height: ${100 / slidesToShow}%;`;
return (
<div
x-data={`carousel({ loop: ${loop}, slidesToShow: ${slidesToShow} })`}
class={clsx("relative flex", className)}
{...restProps}
>
<div class="relative flex grow h-full">
<div
x-ref="carousel"
class={`${directionClassMap.get(direction)} overflow-hidden`}
>
{children ??
slides?.map((slide) => (
<Image
class={slideDirectionClassMap.get(direction)}
style={slideDirectionDimensionStyle}
src={slide.src}
alt={slide.alt}
/>
))}
</div>
{!hideNavigations ? (
<>{navigations ?? <CarouselNavigations direction={direction} />}</>
) : null}
</div>
{indicator === true || typeof indicator !== "boolean" ? (
<>{indicators ?? <CarouselIndicators indicator={indicator} />}</>
) : null}
</div>
);
}
-------------------- Alpine Dependencies -------------------------
/**
* @typedef {Object} CarouselDataInput
* @property {boolean} loop
* @property {number} slidesToShow
*/
/**
* @typedef {Object} CarouselOutput
* @property {HTMLElement[]} items
* @property {number} activeIndex
* @property {HTMLElement | null} activeItem
* @property {boolean} loop
* @property {number} slidesToShow
* @property {(index: number) => boolean} isActive
* @property {(index: number) => void} setActiveIndex
* @property {Function} [previous]
* @property {Function} [next]
* @property {() => boolean} areFirstSlidesToShow
* @property {() => boolean} areLastSlidesToShow
*/
/**
* Carousel alpine data
* @param {CarouselDataInput} carouselInputs
* @returns {import("alpinejs").AlpineComponent<CarouselOutput>}
*/
export function carouselData(carouselInputs) {
return {
items: this.$refs.carousel.children,
activeIndex: 0,
activeItem: null,
loop: carouselInputs?.loop ?? false,
slidesToShow: carouselInputs?.slidesToShow ?? 1,
init() {
this.activeItem = this.items[this.activeIndex];
this.$refs.carousel.style.height = this.activeItem.clientHeight + "px";
window.addEventListener("resize", () => {
this.$refs.carousel.style.height = this.activeItem?.clientHeight + "px";
});
this.$watch("activeIndex", (value) => {
this.$dispatch("selected-slide", { selectedIndex: value });
this.activeItem = this.items[value];
const activeItemBoundingClientRect =
this.activeItem.getBoundingClientRect();
const carouselBoundingClientRect =
this.$refs.carousel.getBoundingClientRect();
const xDistance =
activeItemBoundingClientRect.x - carouselBoundingClientRect.x;
const yDistance =
activeItemBoundingClientRect.y - carouselBoundingClientRect.y;
this.$refs.carousel.scrollBy(xDistance, yDistance);
});
},
setActiveIndex(index) {
this.activeIndex = index;
},
previous() {
if (this.loop && this.areFirstSlidesToShow()) {
this.activeIndex = this.items.length - this.slidesToShow;
} else {
this.activeIndex -= this.slidesToShow;
}
},
next() {
if (this.loop && this.areLastSlidesToShow()) {
this.activeIndex = 0;
} else {
this.activeIndex +=
this.activeIndex + this.slidesToShow < this.items.length
? this.slidesToShow
: this.items.length % this.slidesToShow;
}
},
isActive(index) {
return this.activeIndex === index;
},
areFirstSlidesToShow() {
return this.activeIndex < this.slidesToShow;
},
areLastSlidesToShow() {
return this.activeIndex + this.slidesToShow >= this.items.length;
},
};
}
Default Carousel
By default, Carousel is aligned horizontally with navigation button in the middle and each one to the opposite side. Slides are images.
We will see further that you can customize slides and put whatever contents you can.
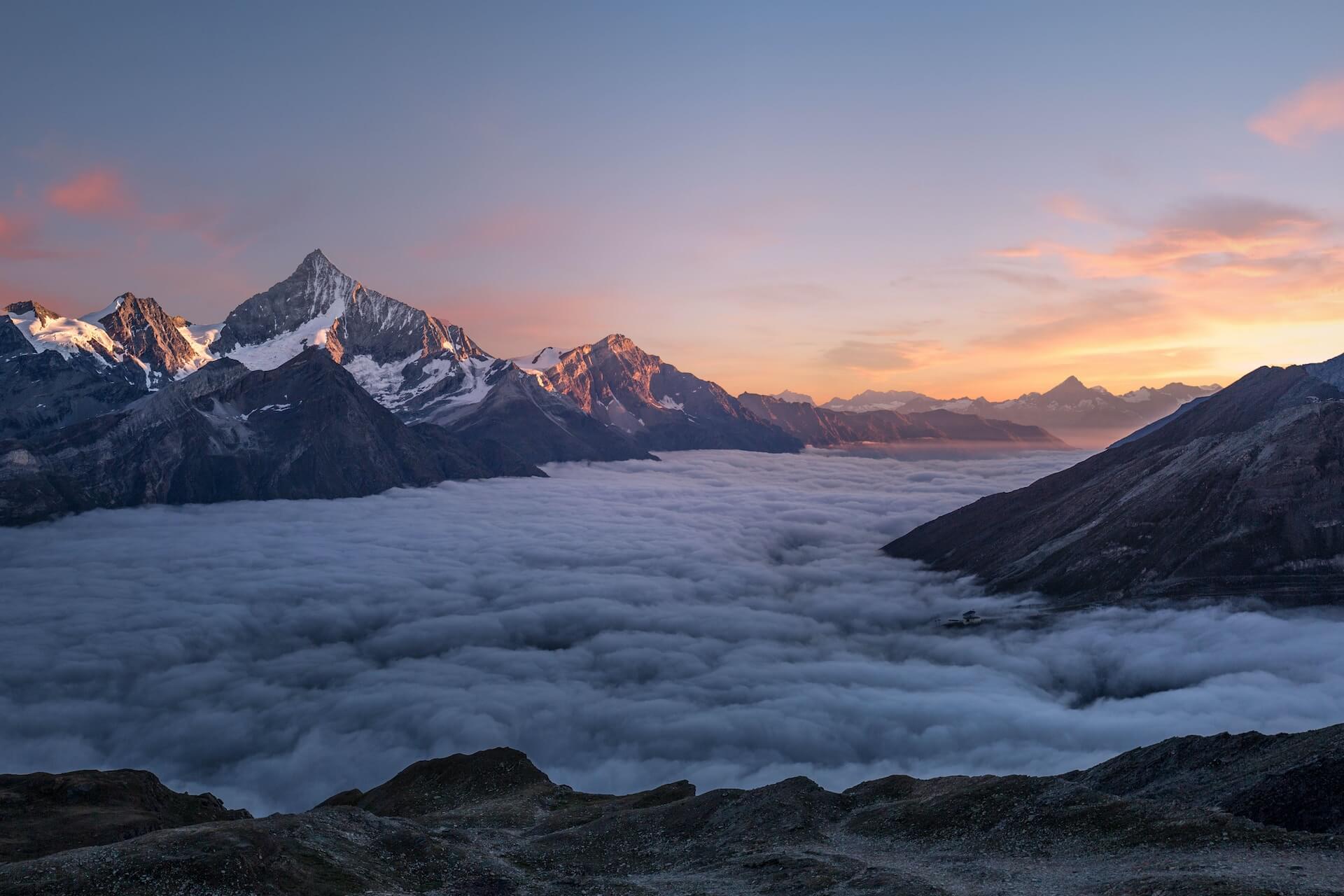
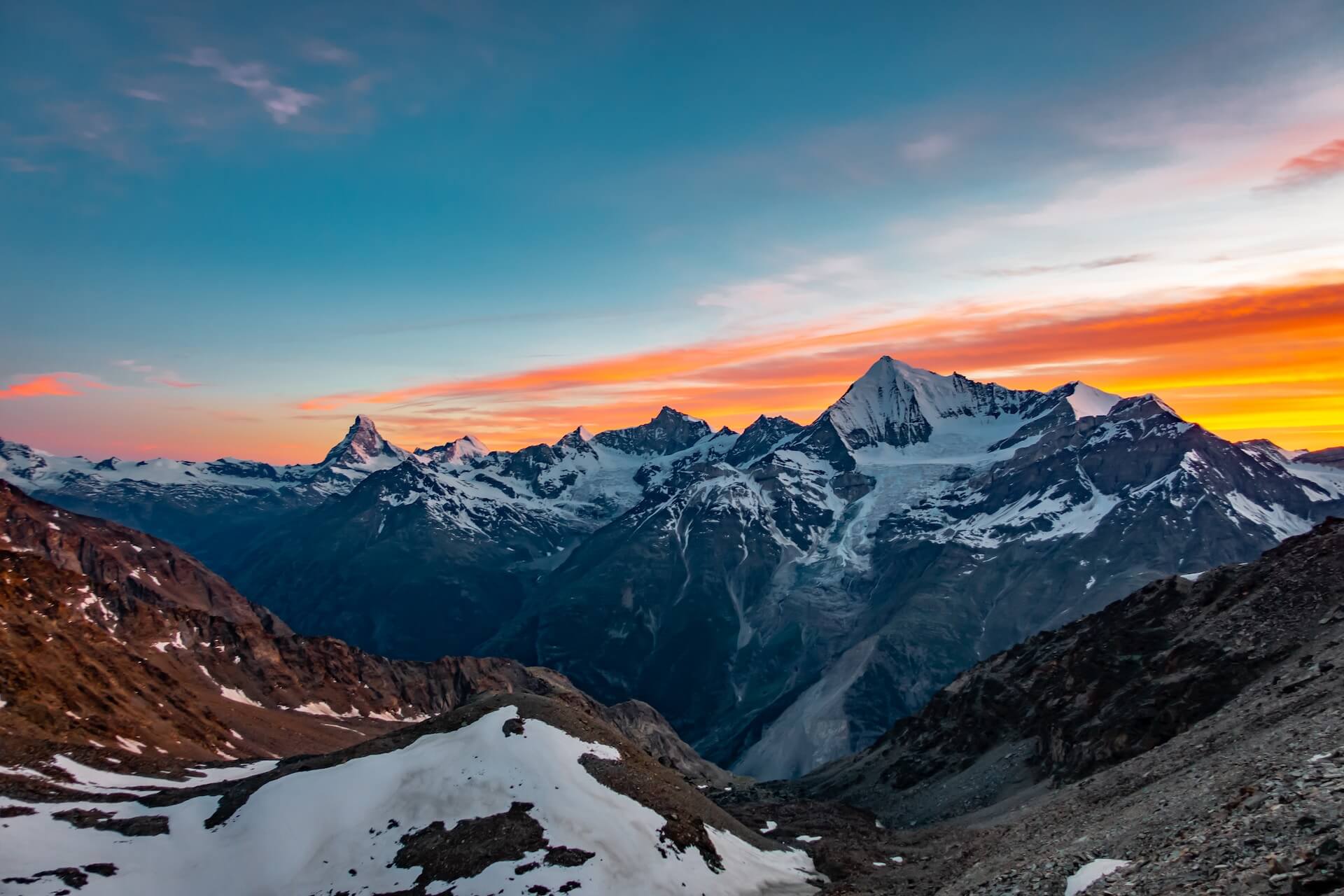
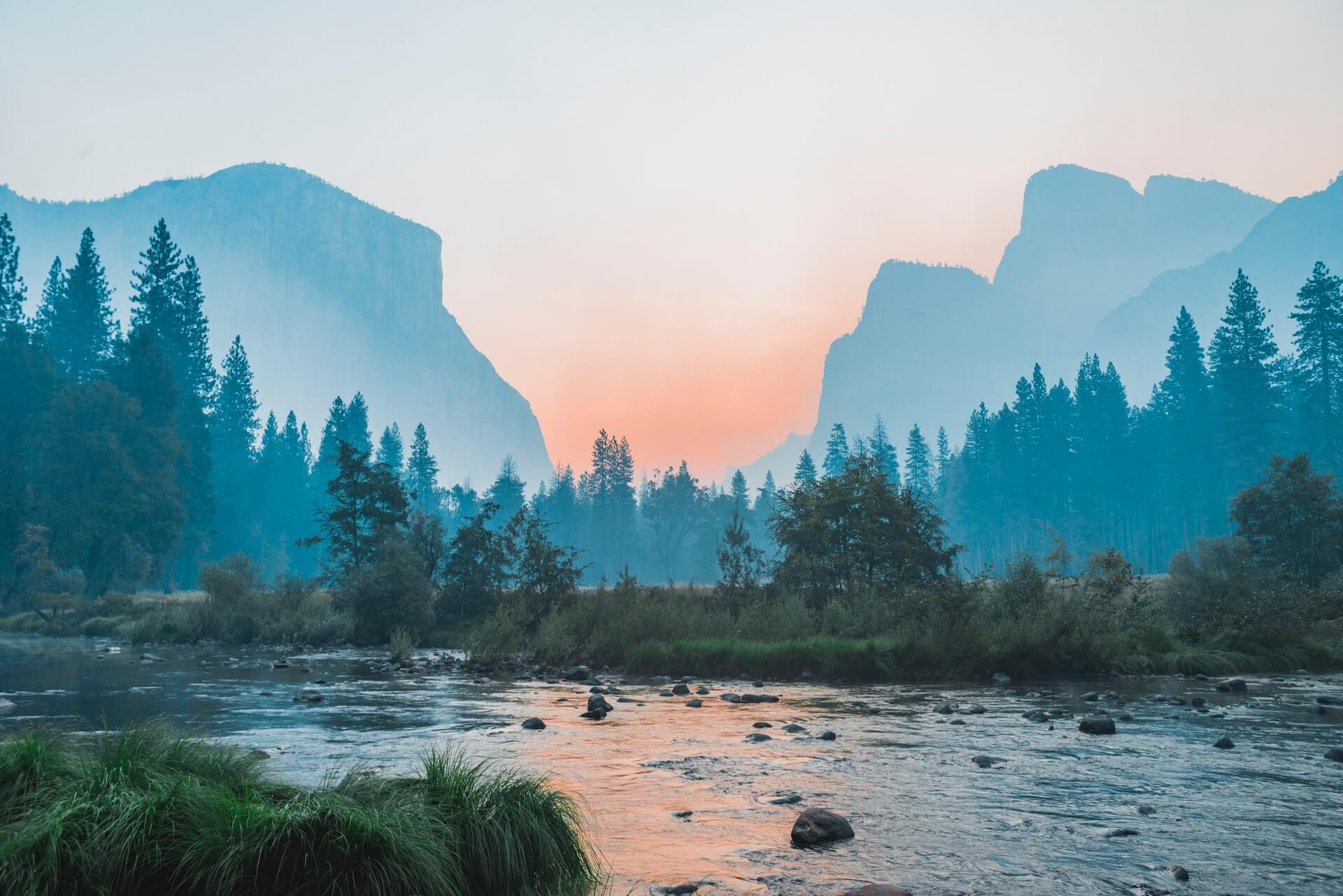
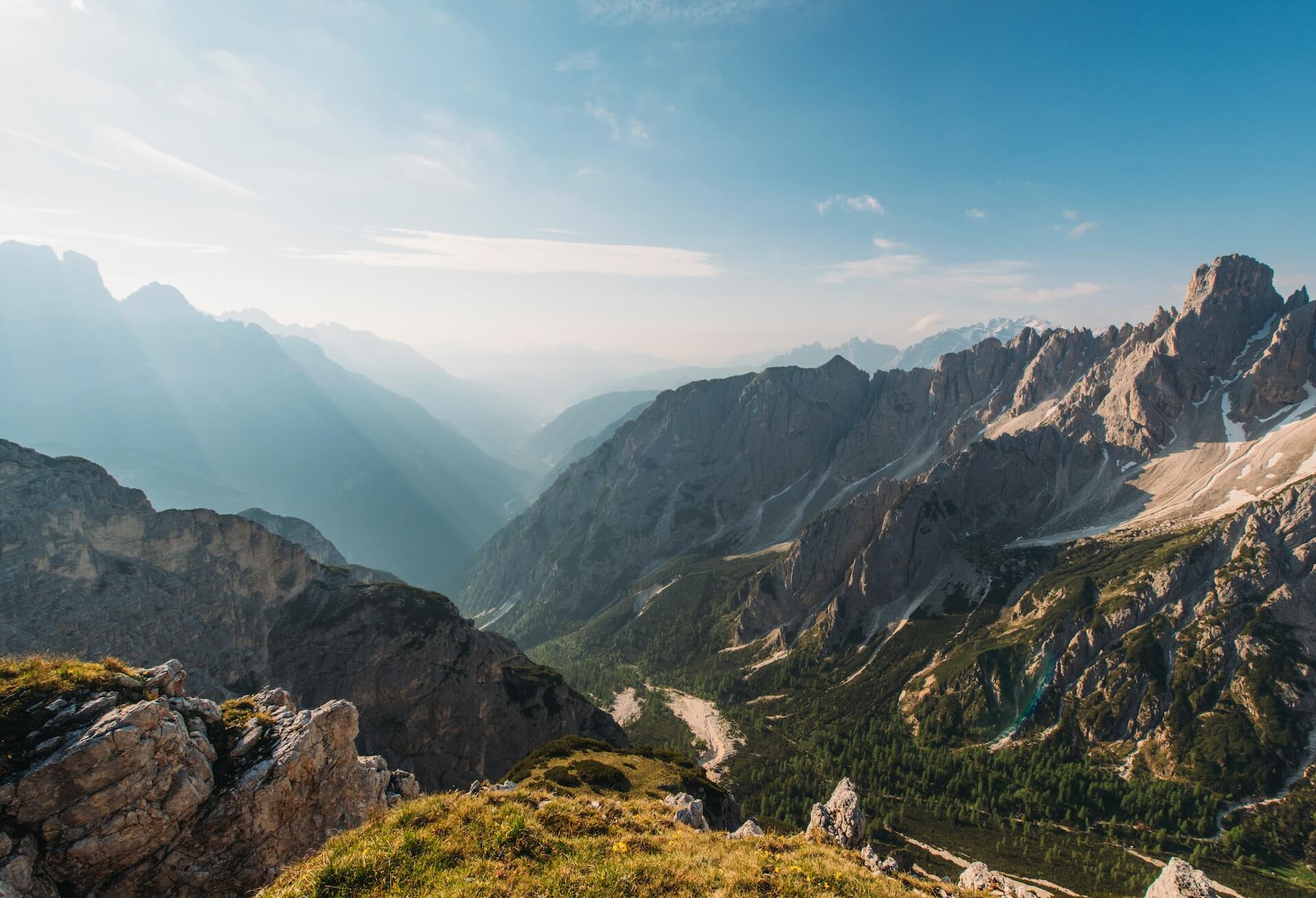
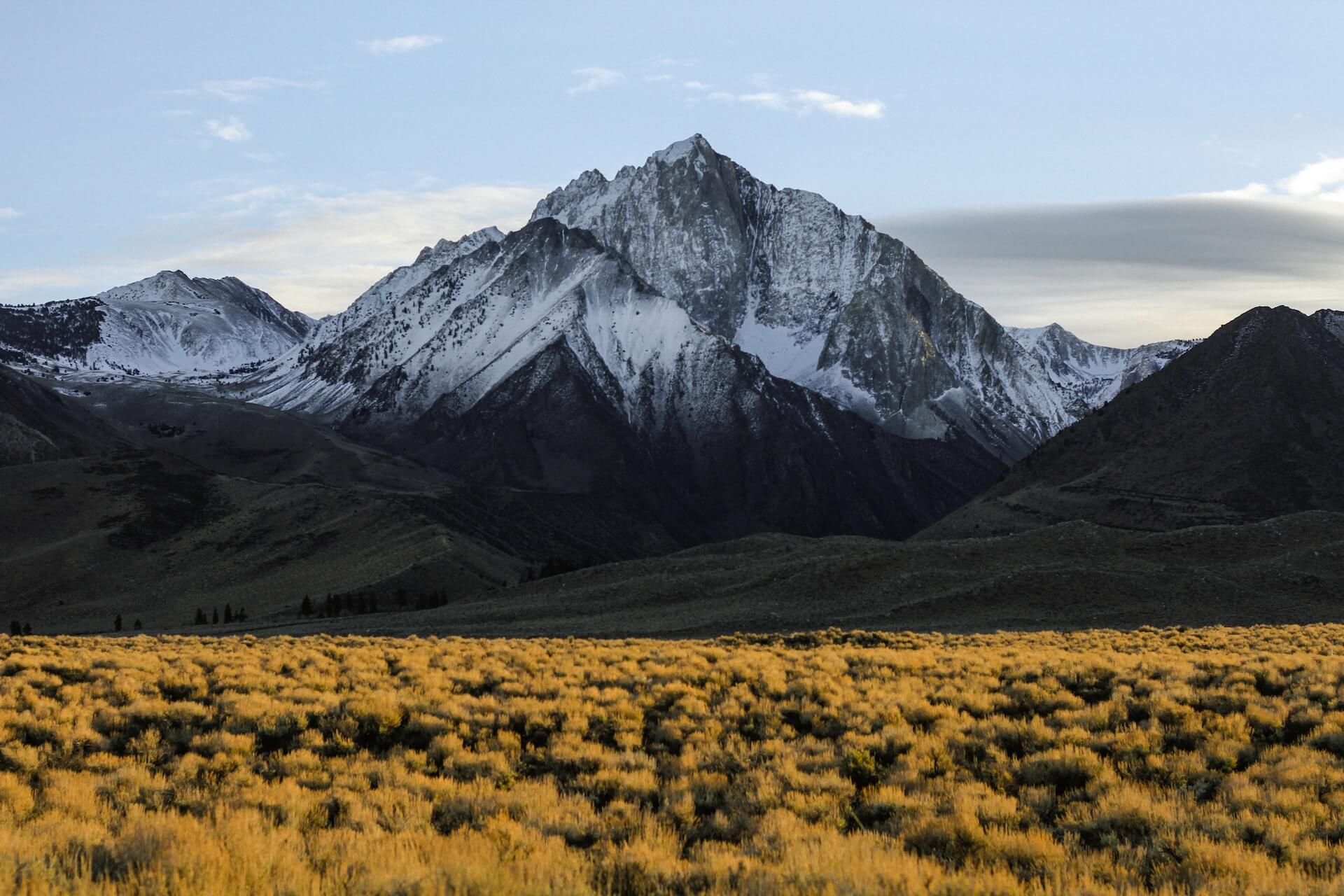
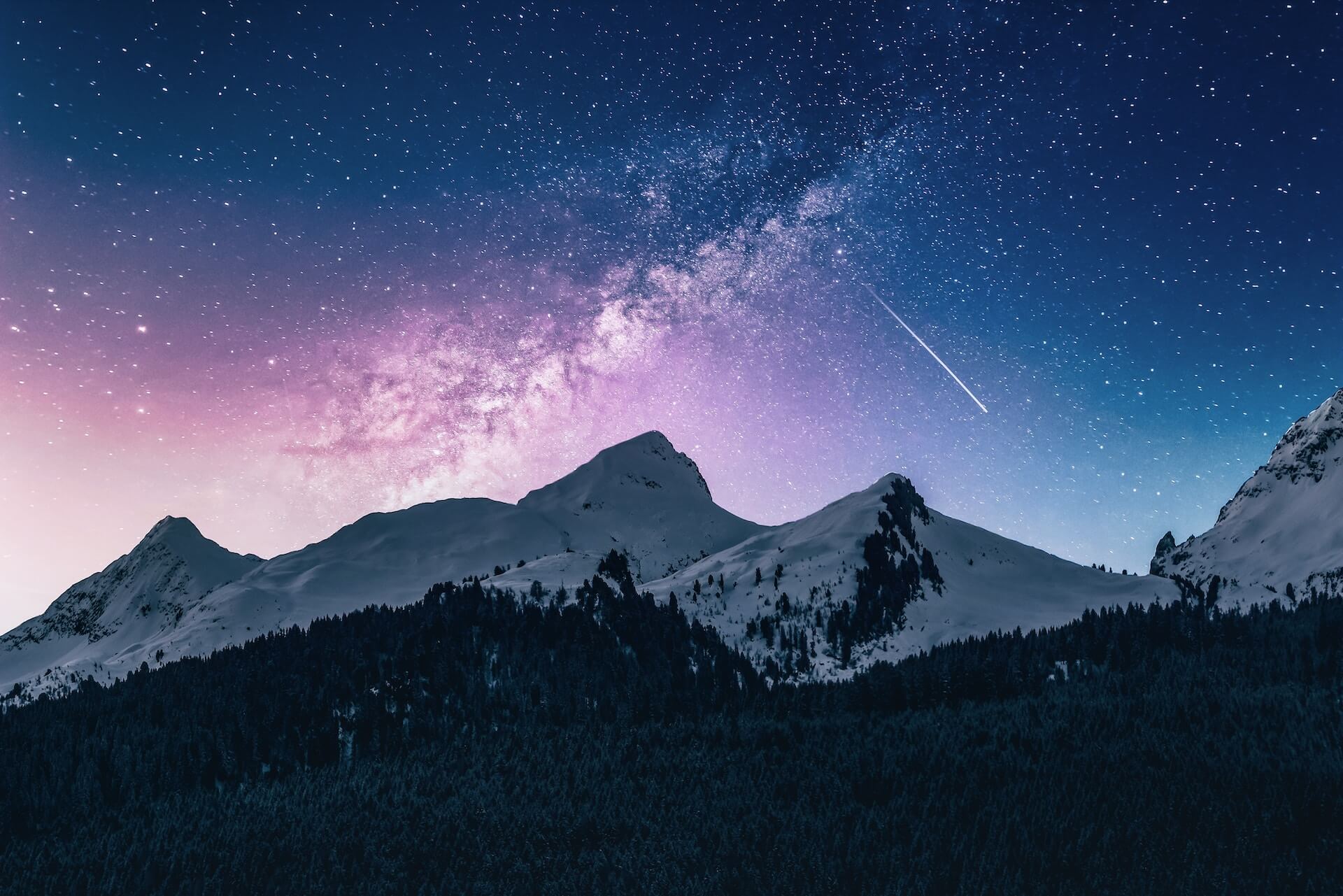
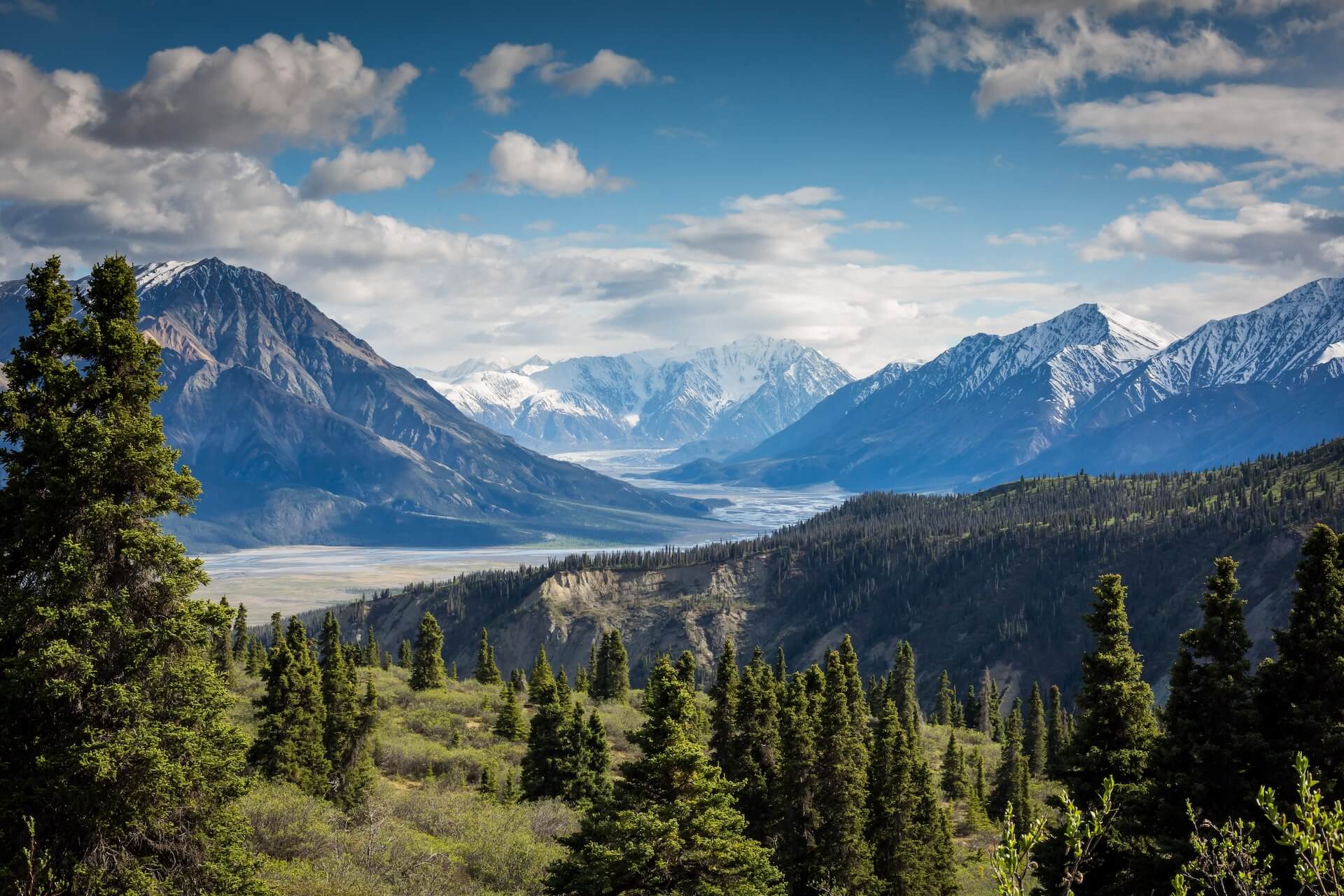
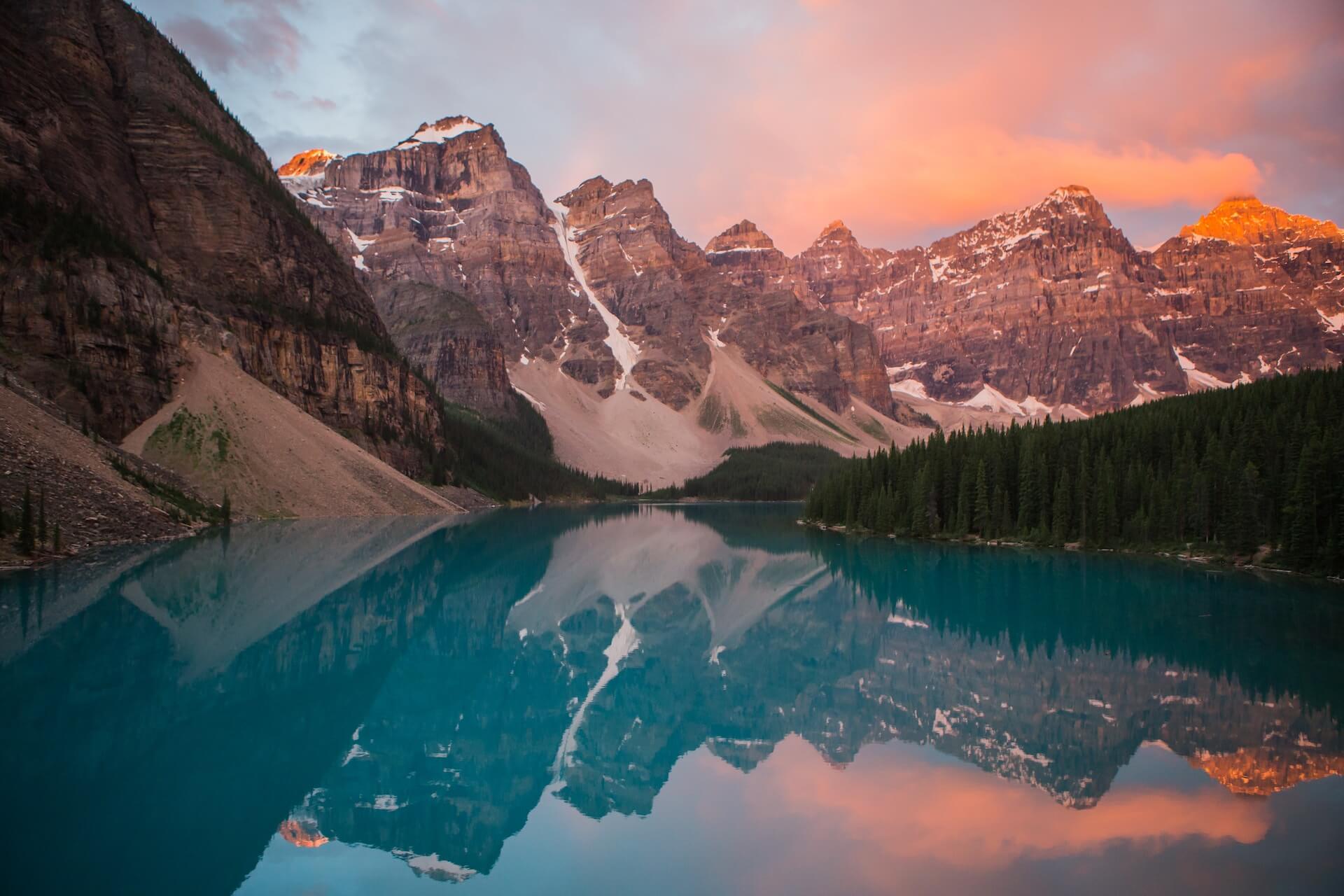
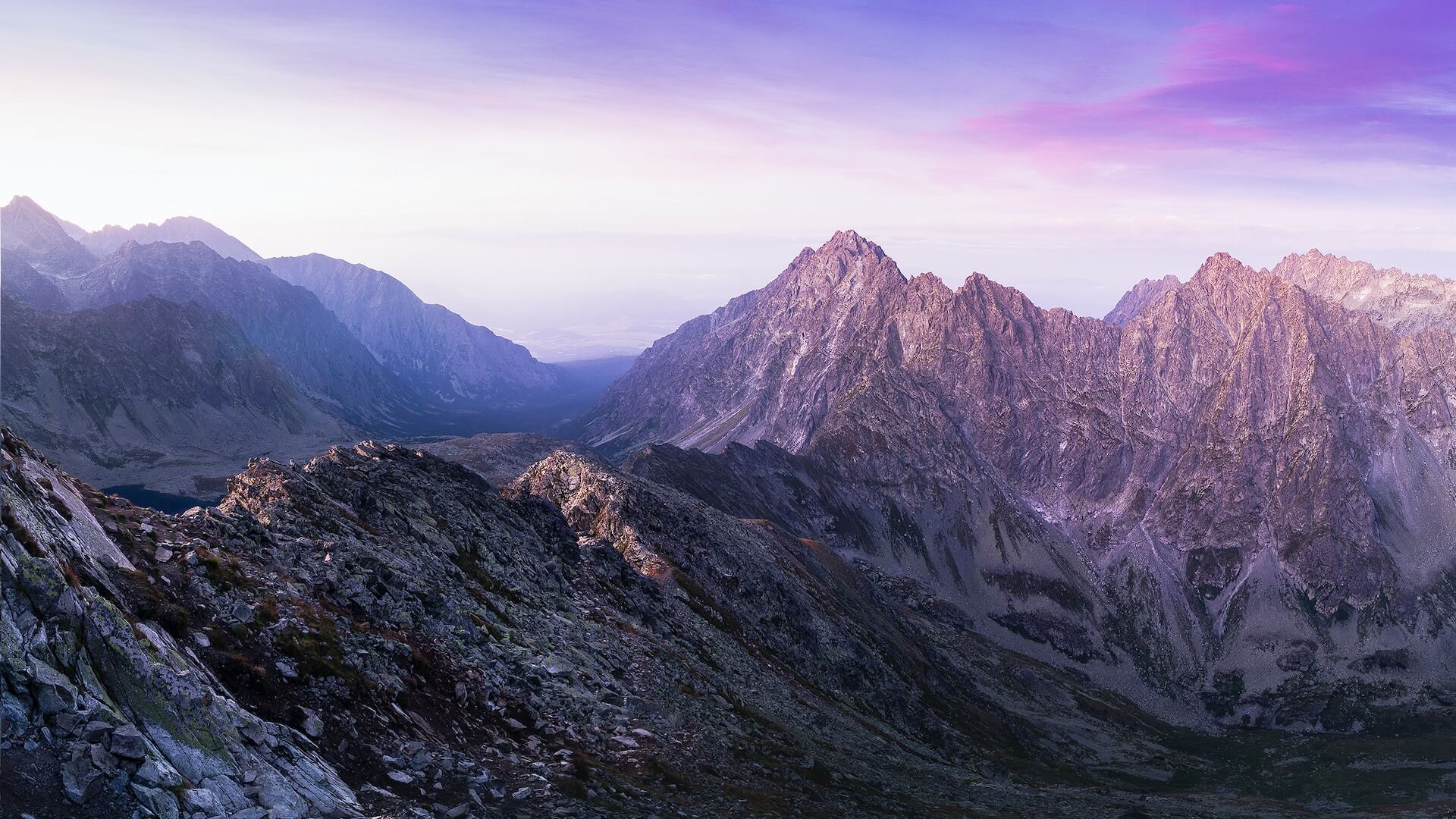
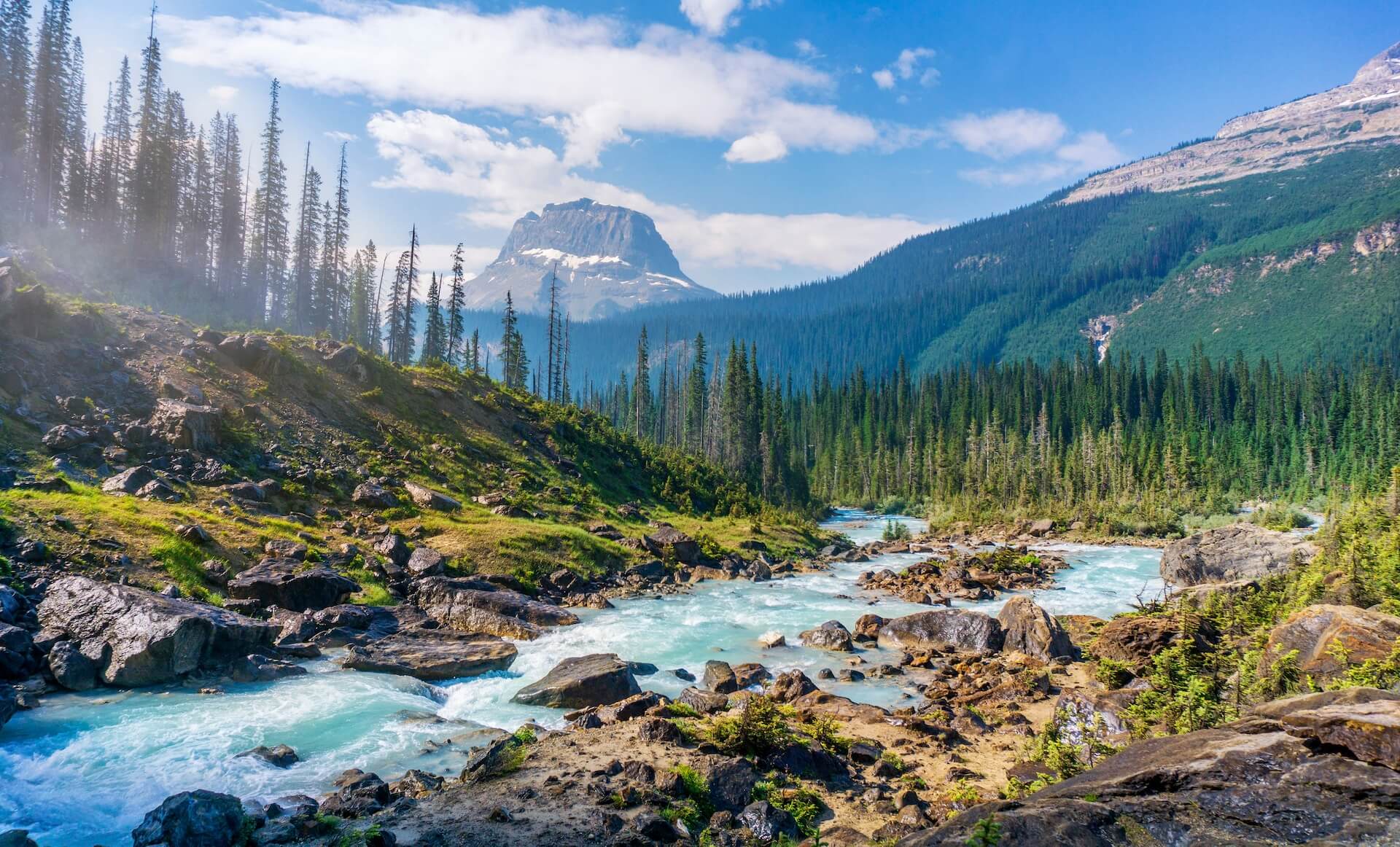
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function DefaultCarouselExample(props) {
const { slides } = props;
return <Carousel slides={slides} />;
}
With Indicators
By setting indicator props to true, dot points will appeared at the bottom of the carousel.
It displays the number of items the carousel contains.
These are indicator props value: true, top, bottom (default value when true), left, right.
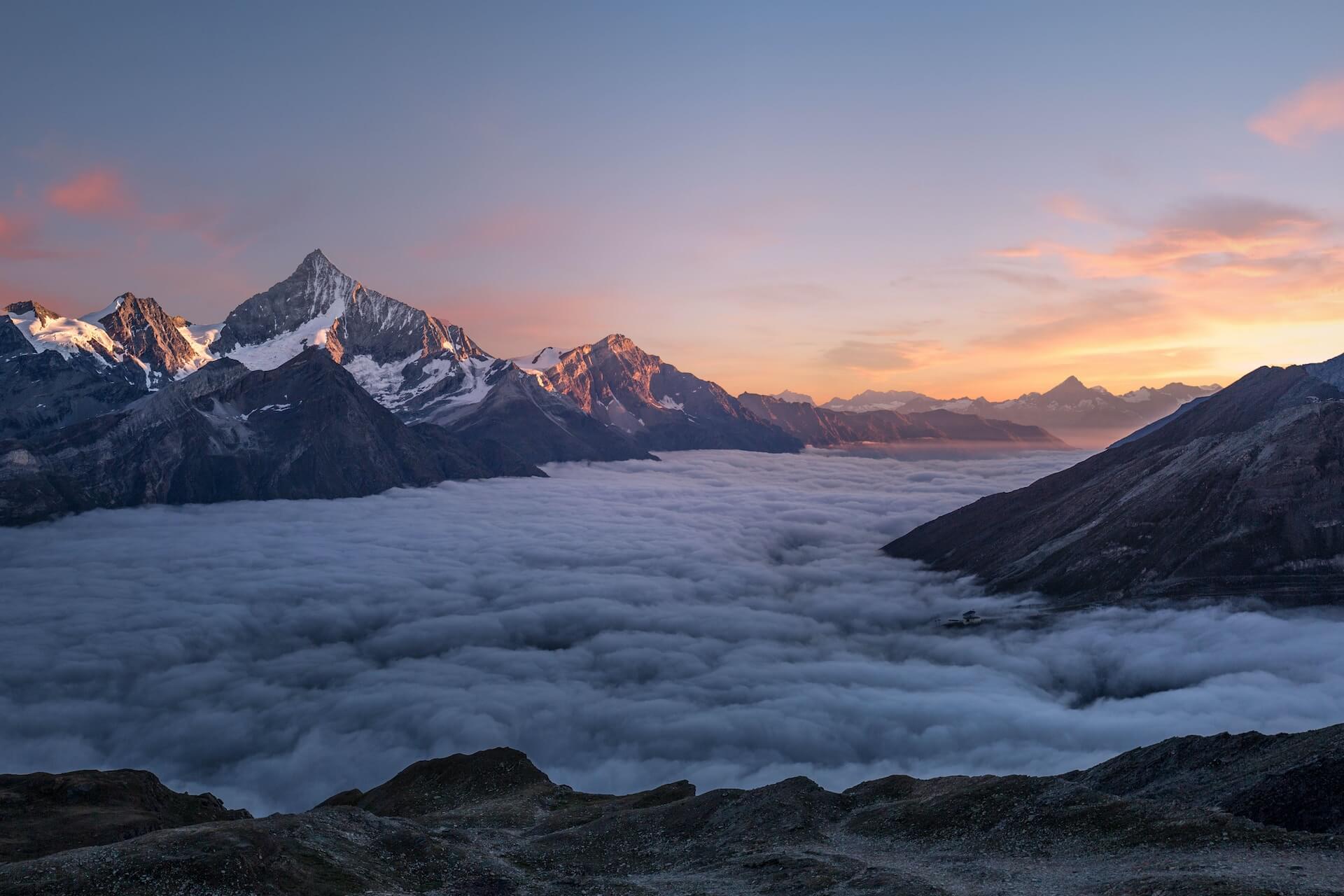
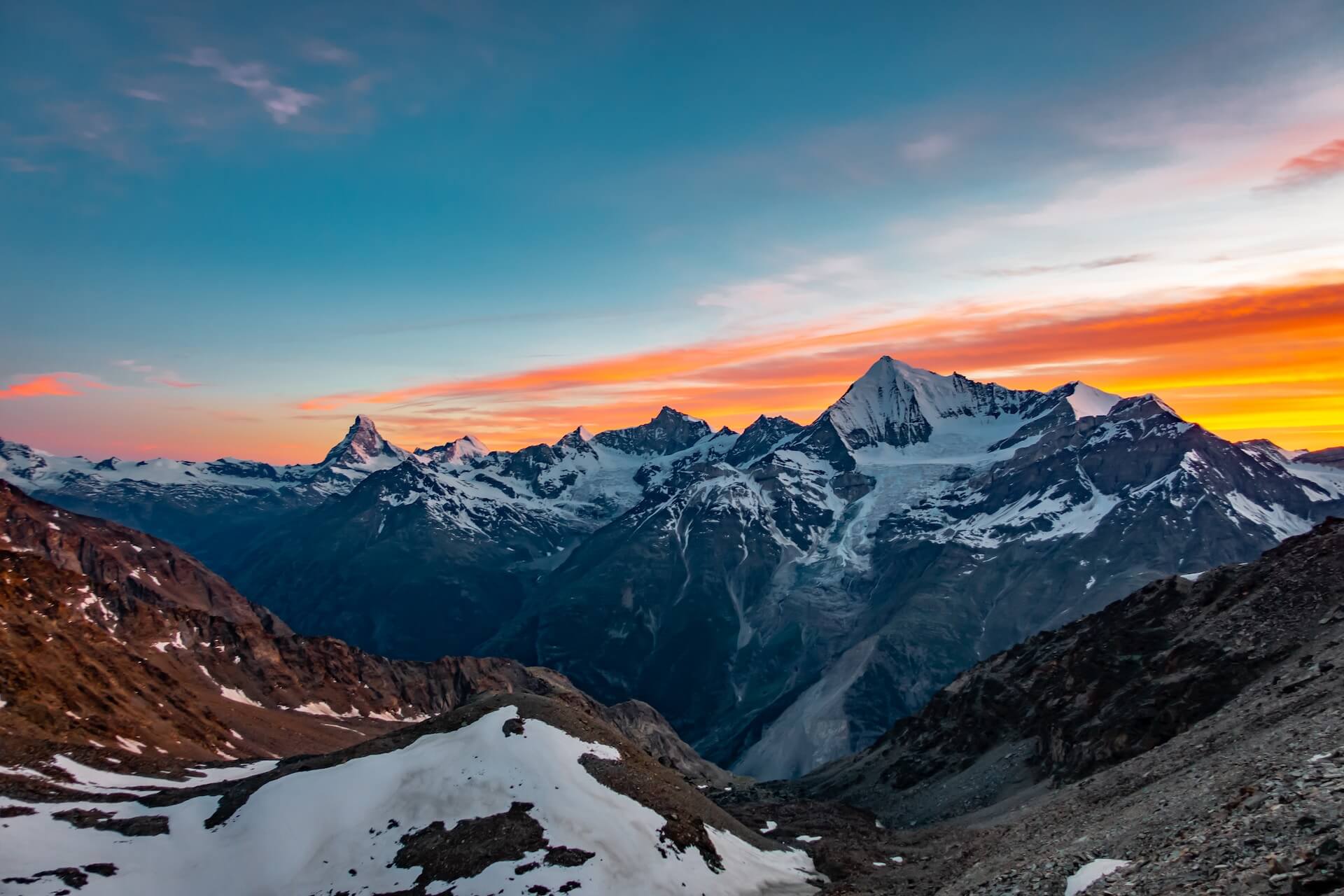
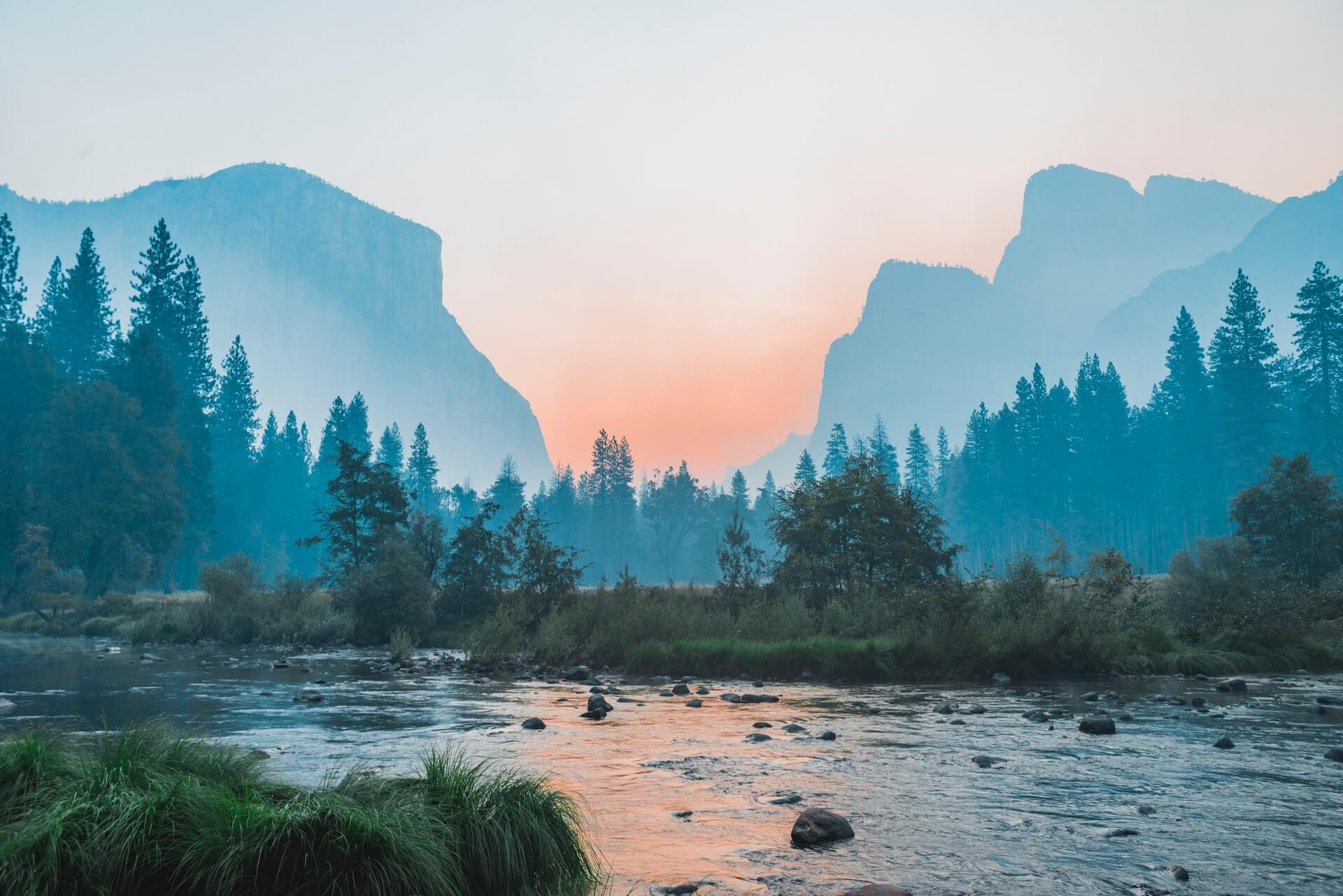
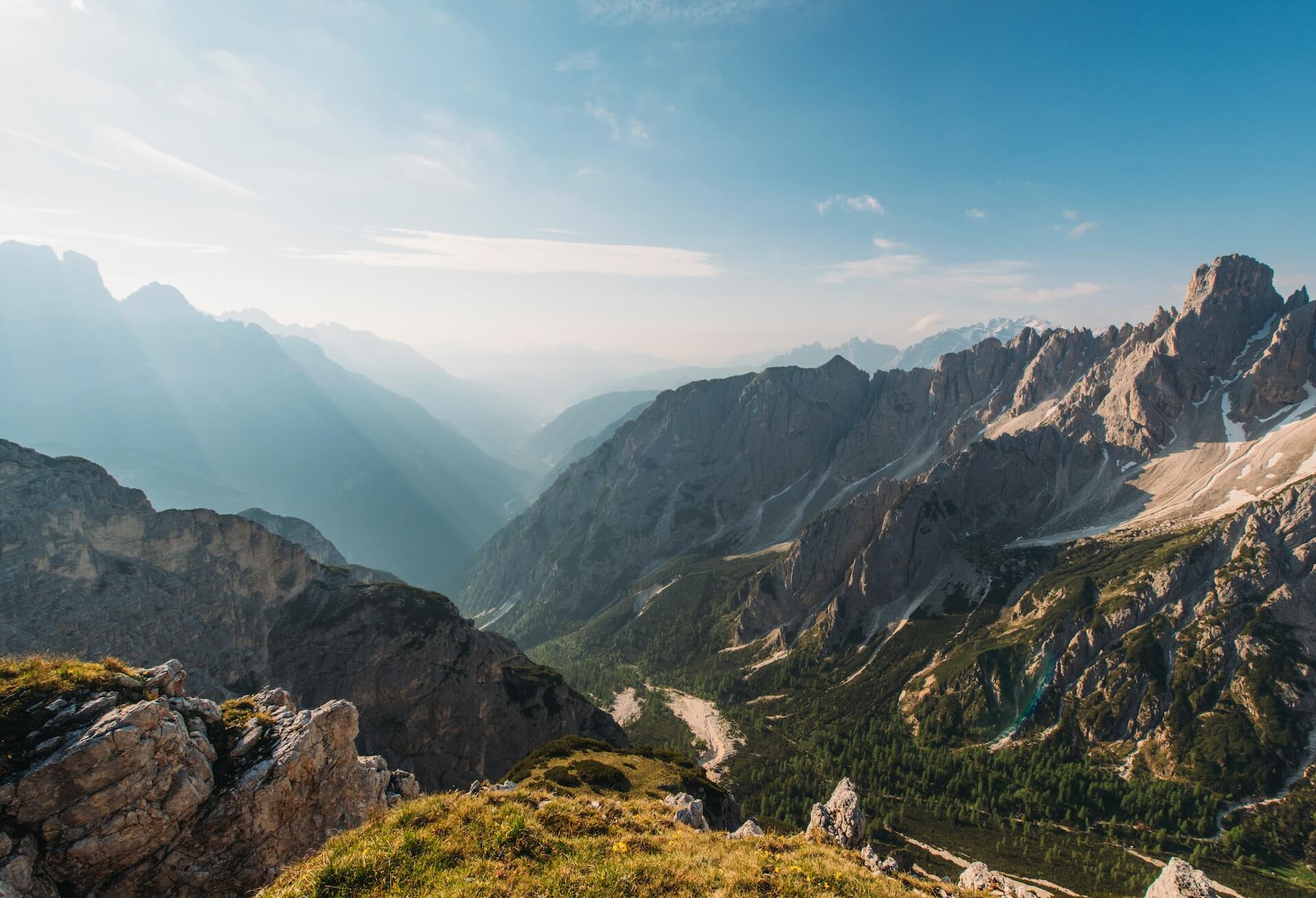
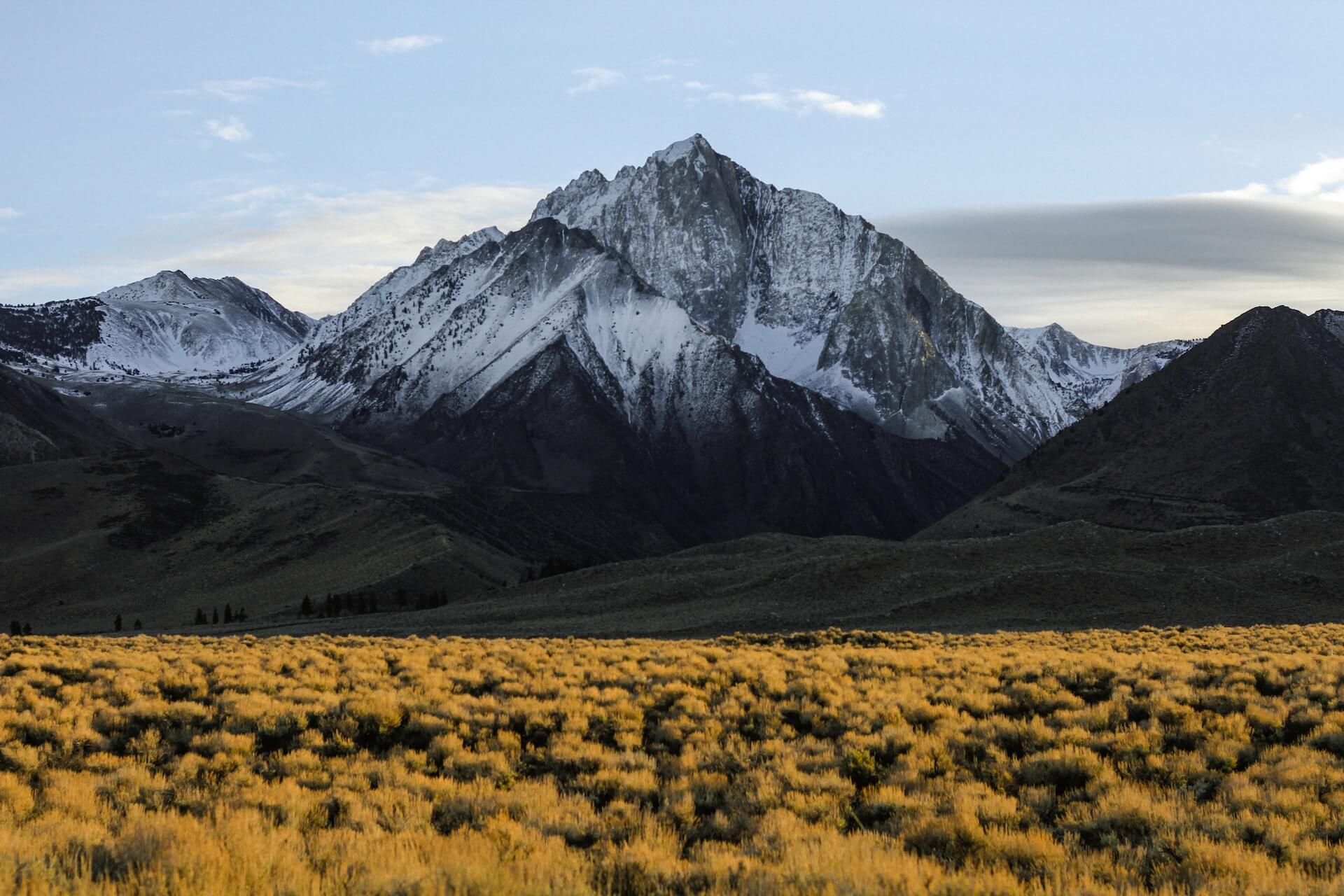
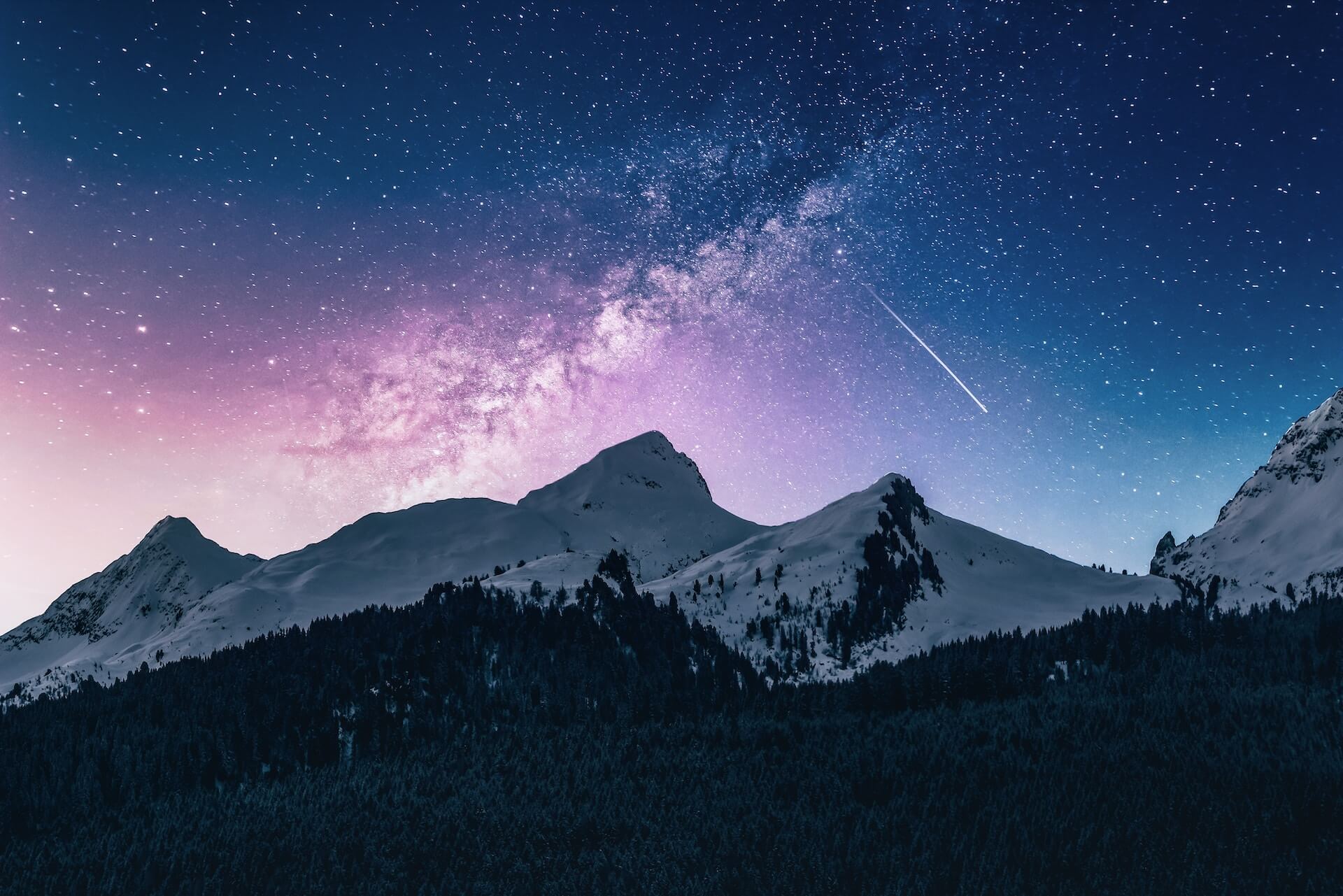
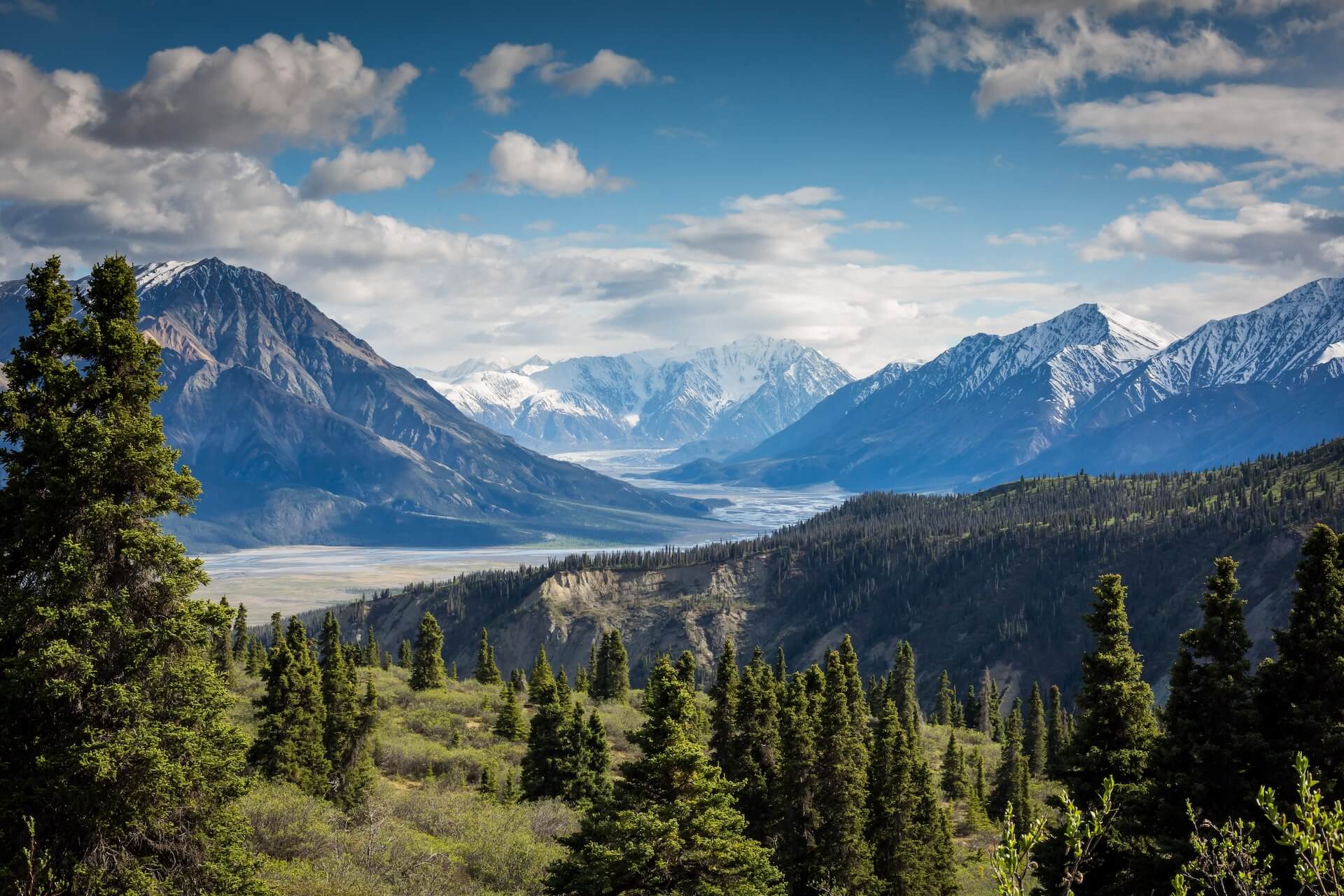
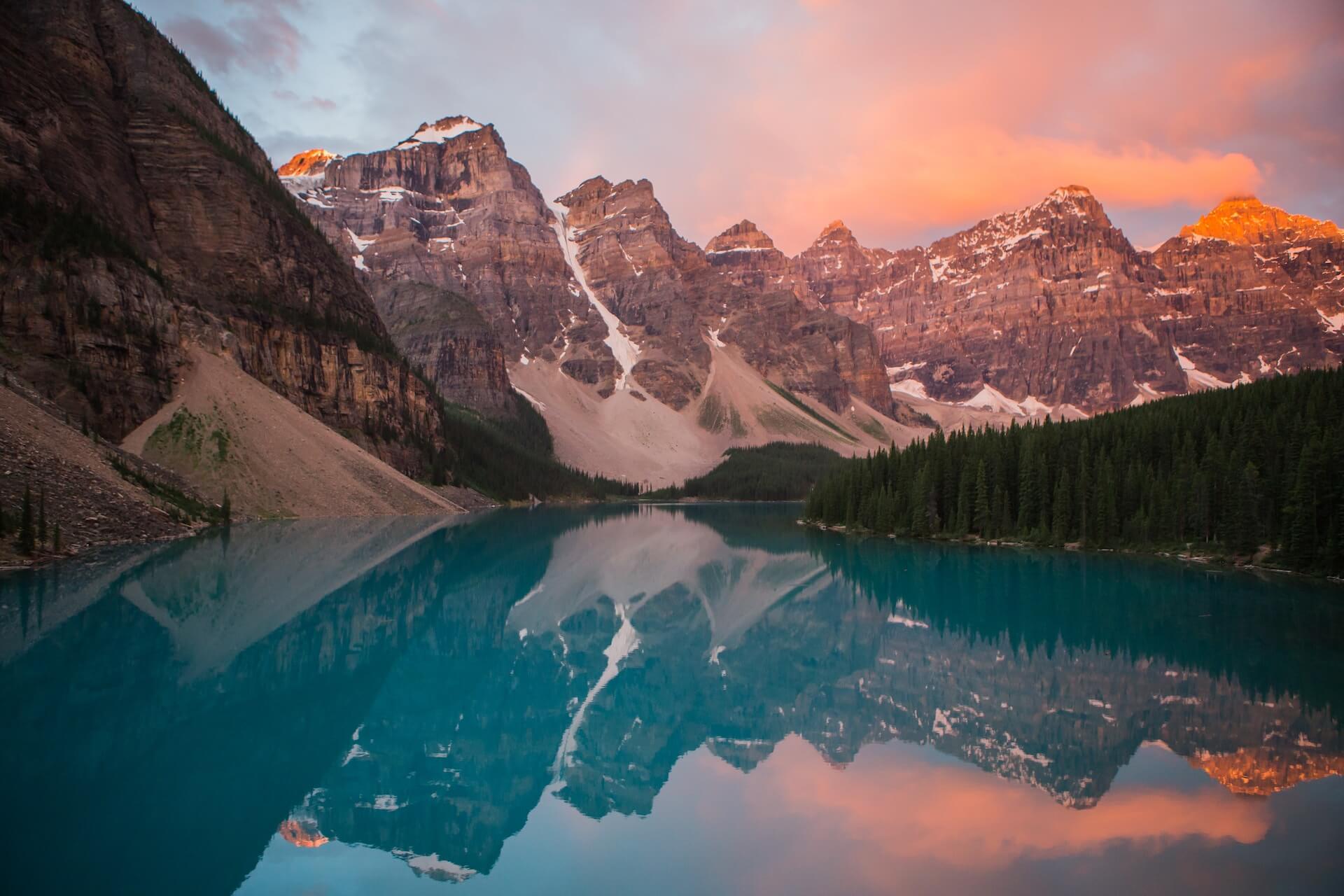
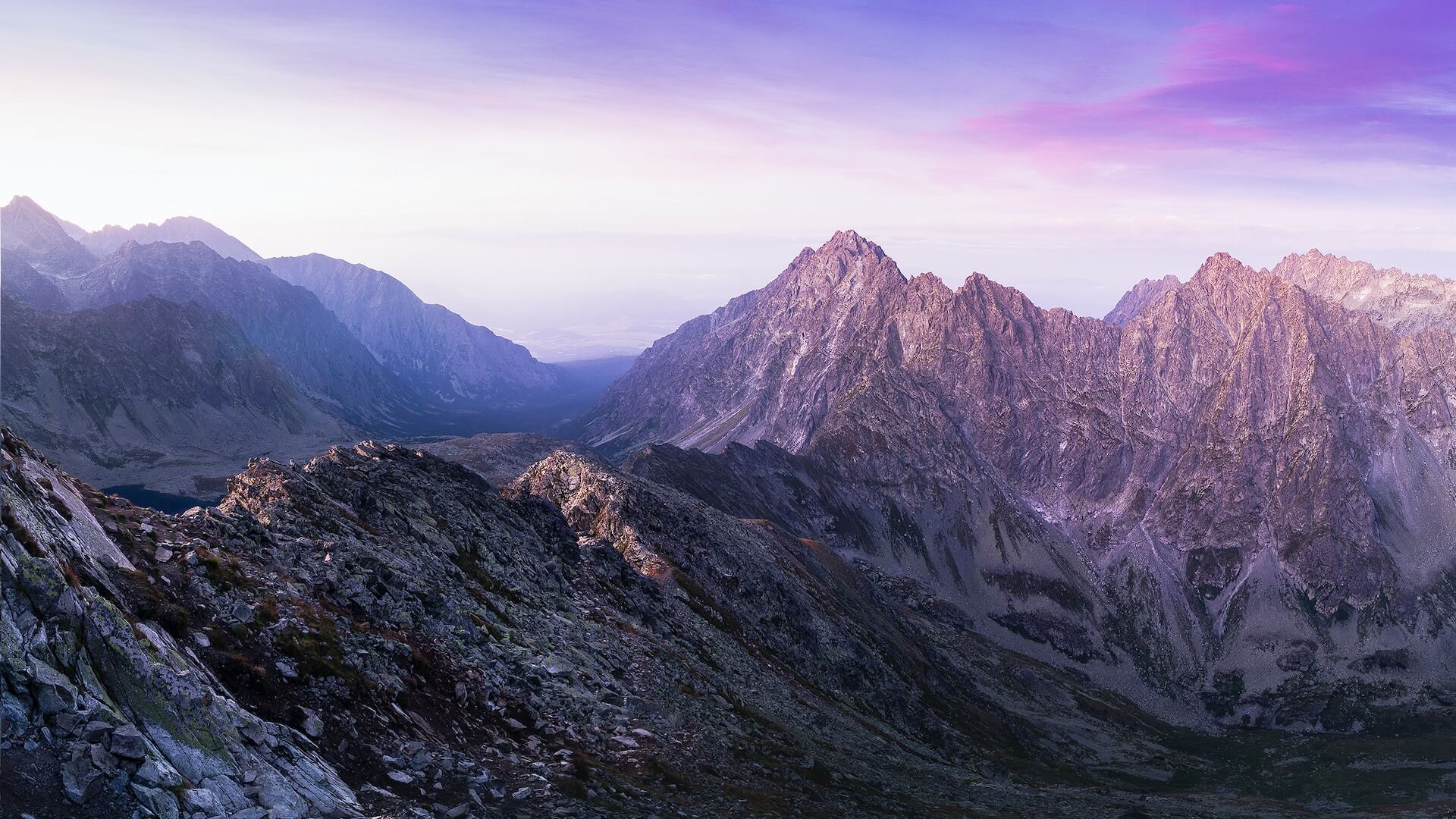
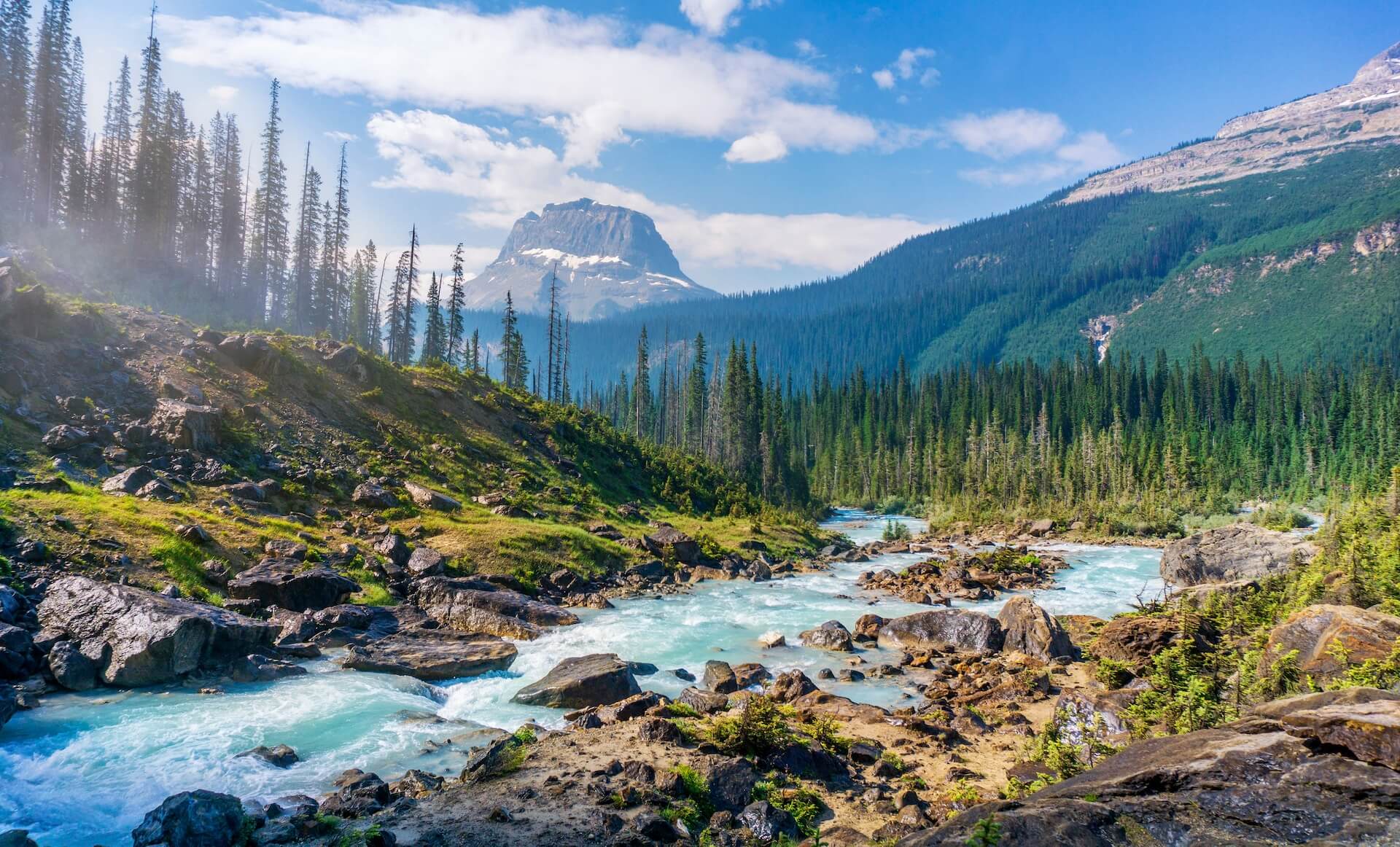
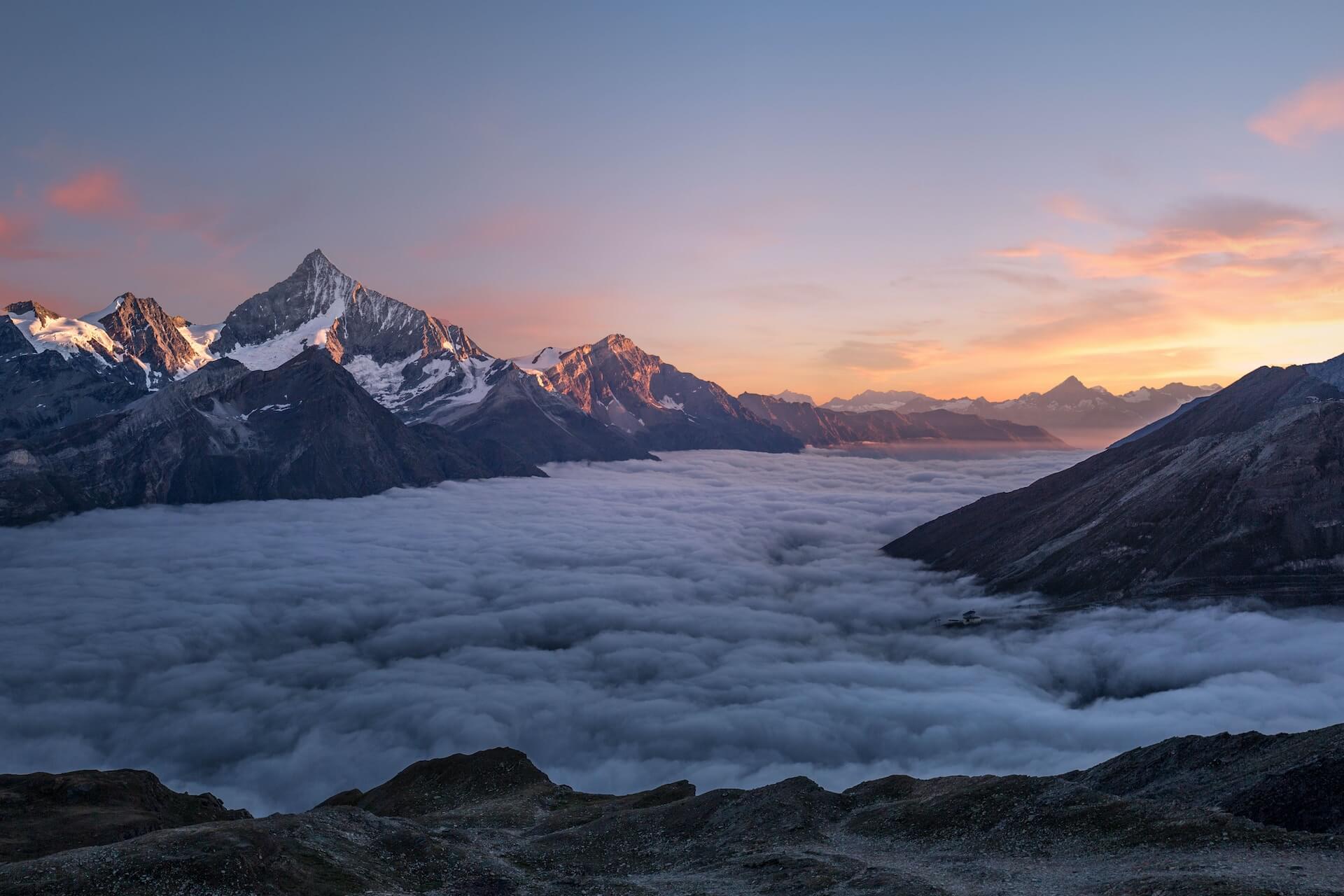
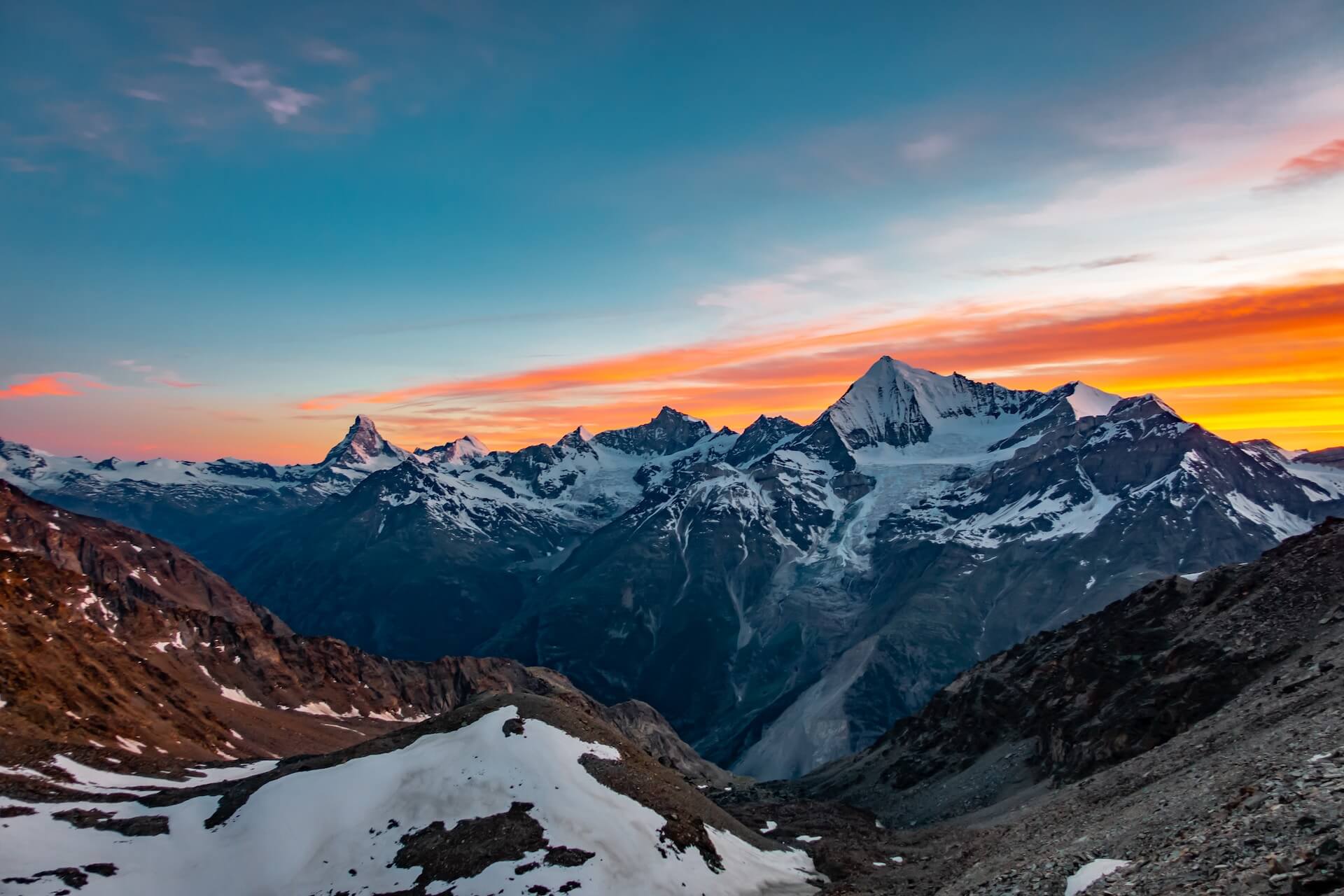
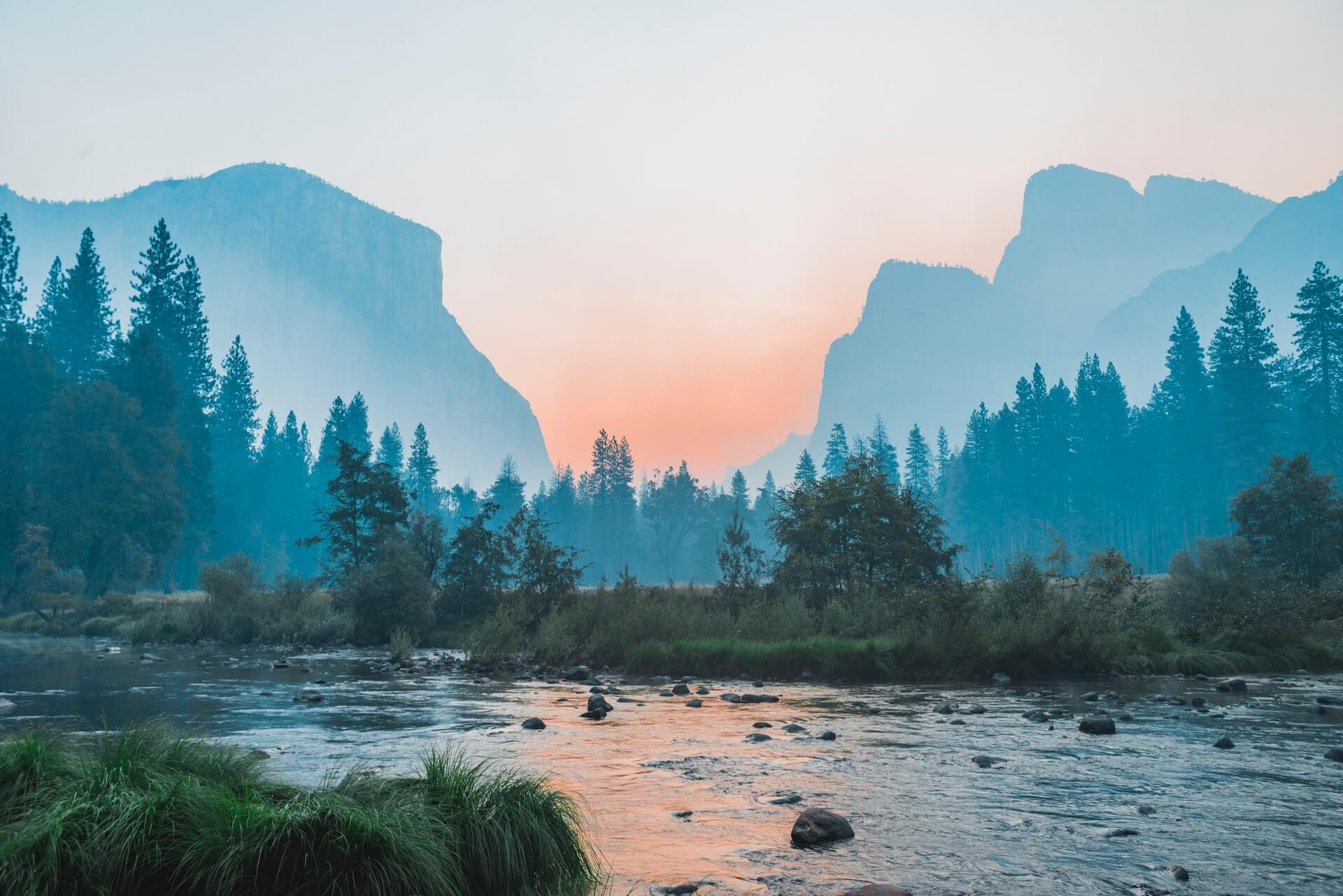
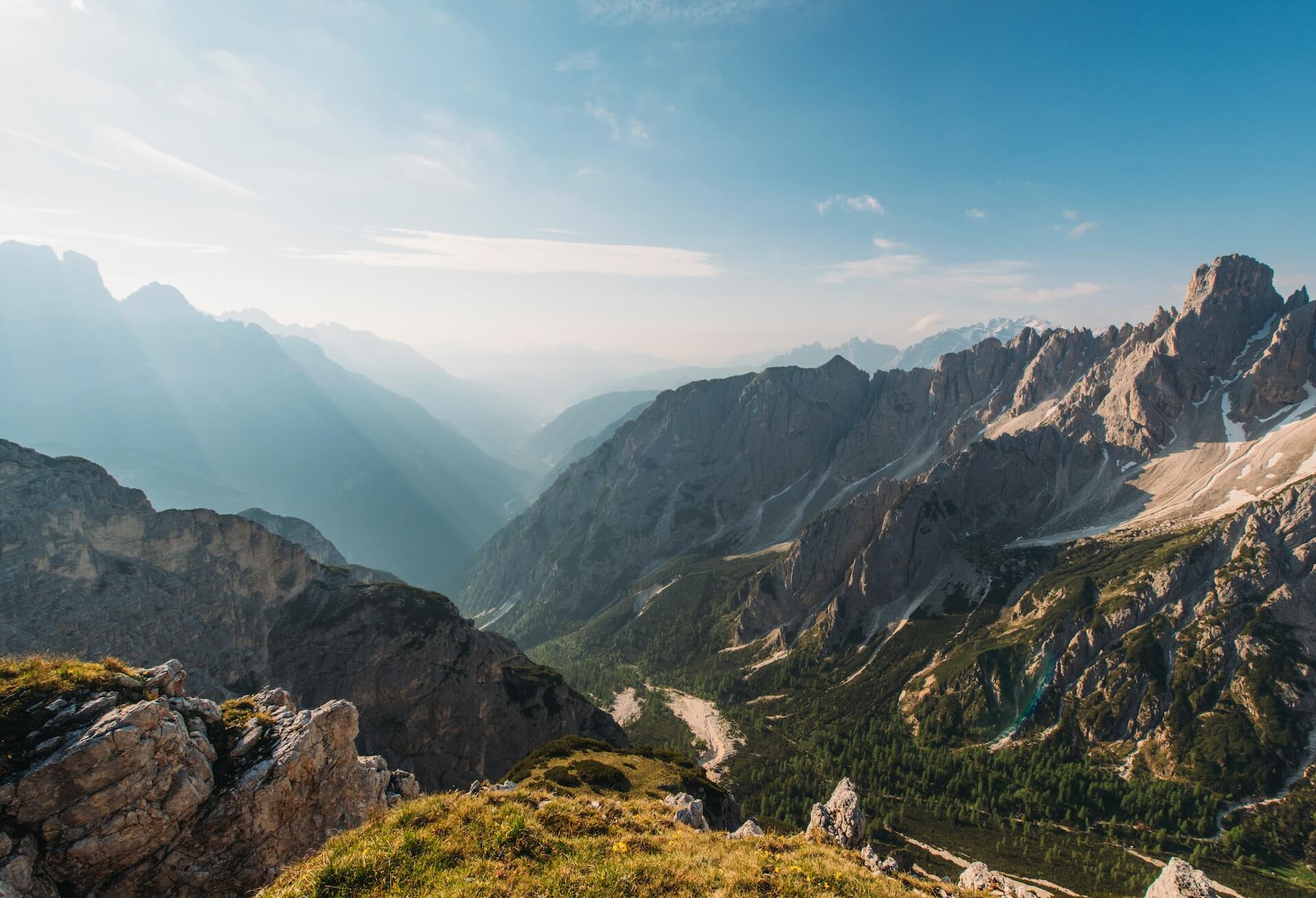
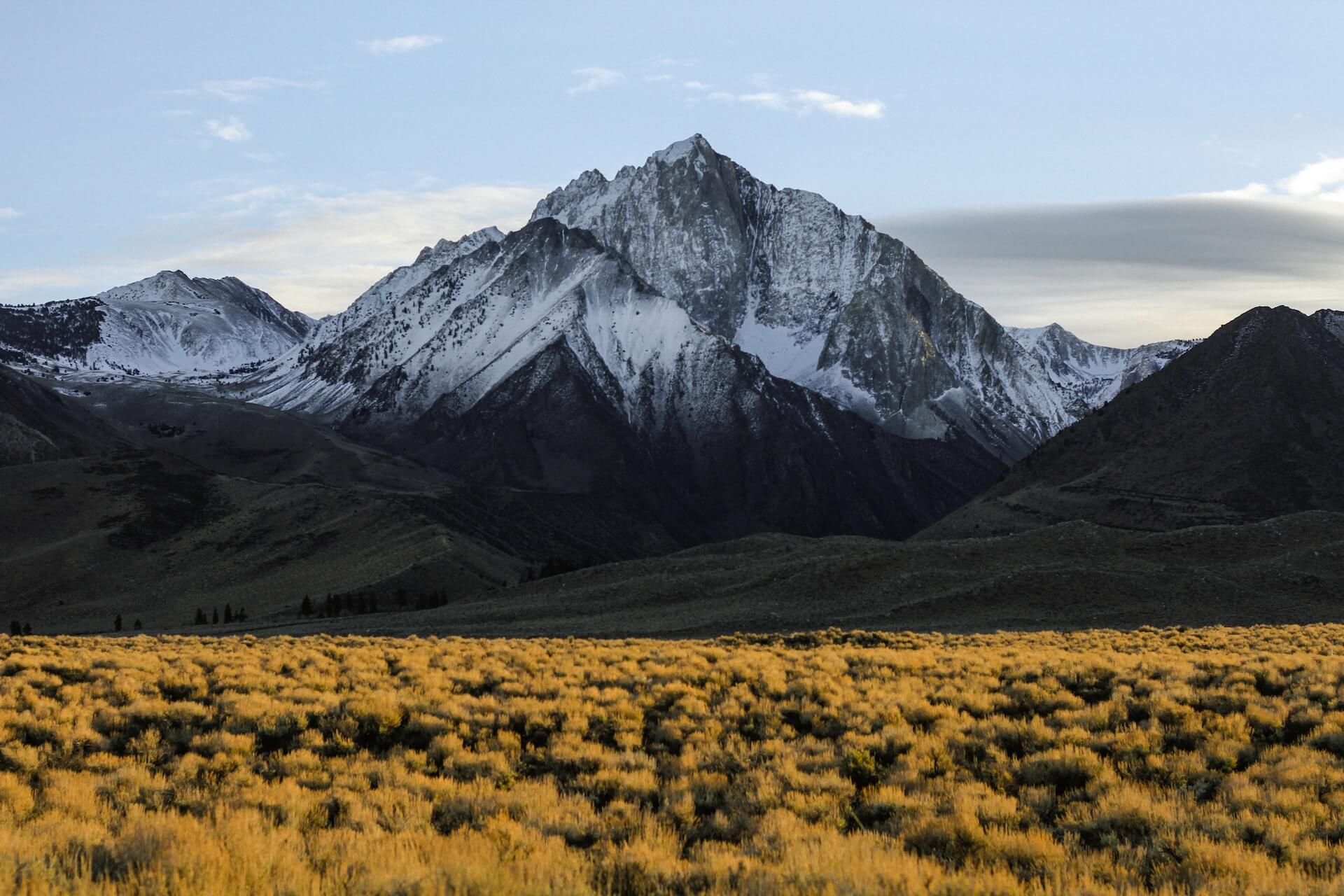
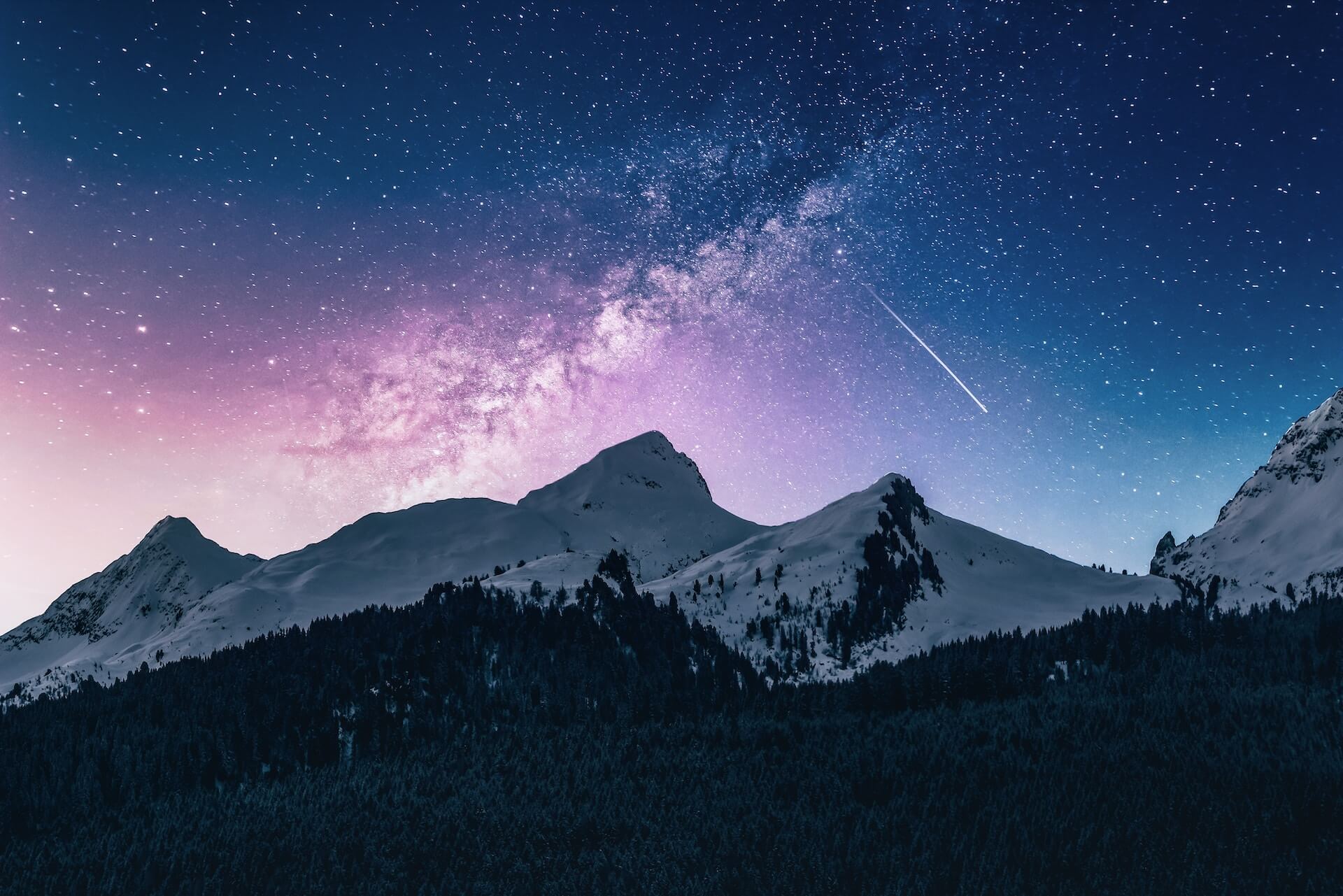
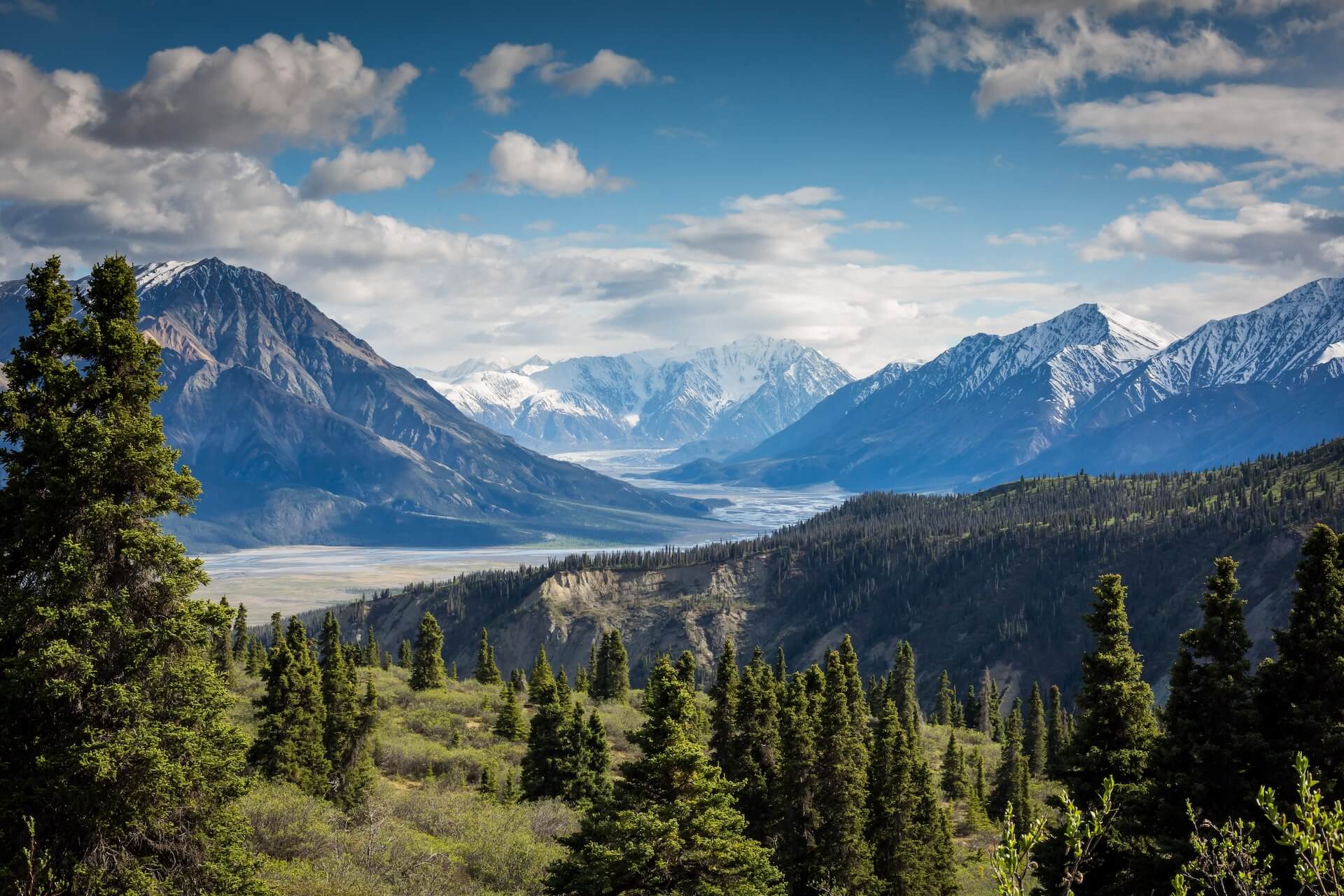
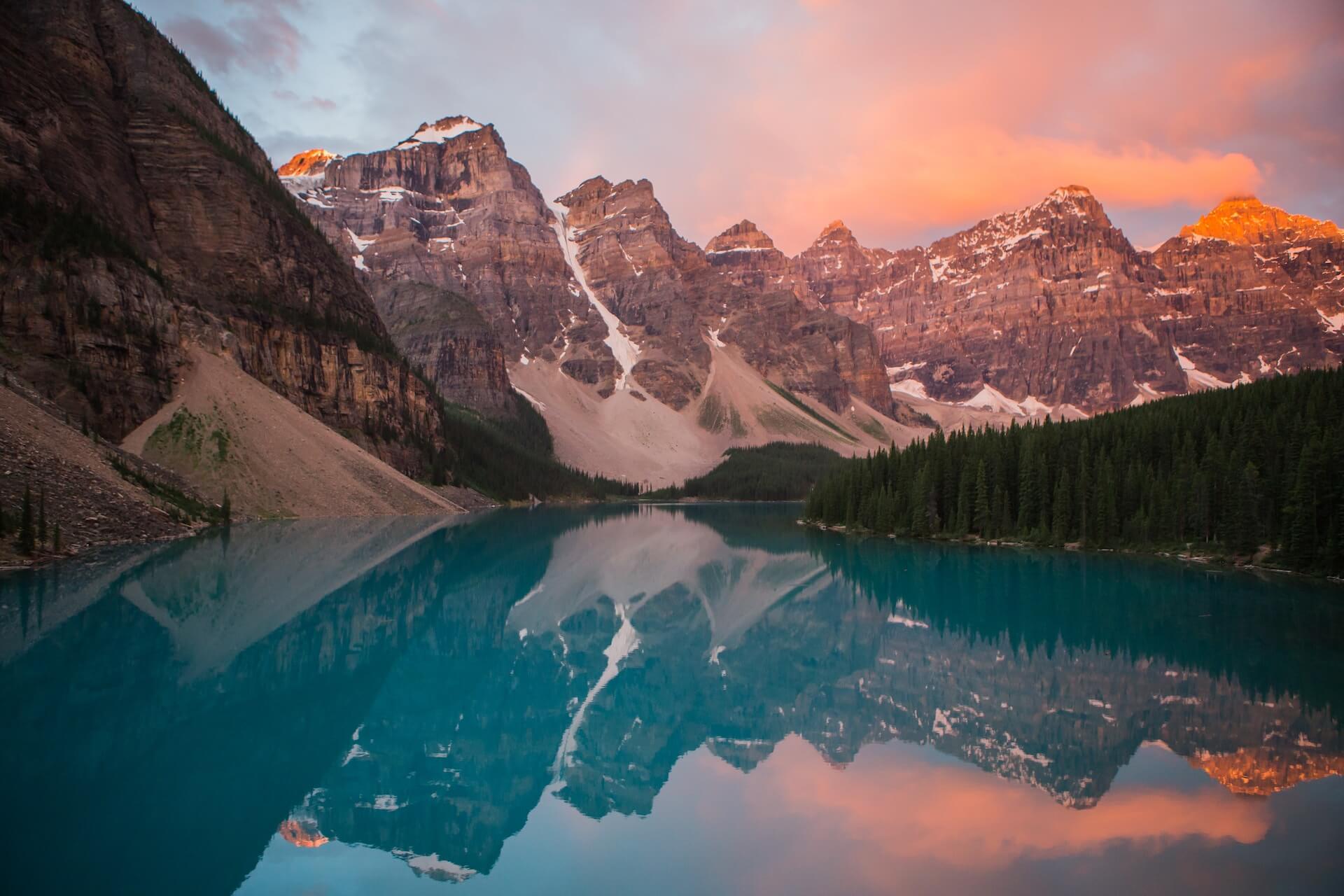
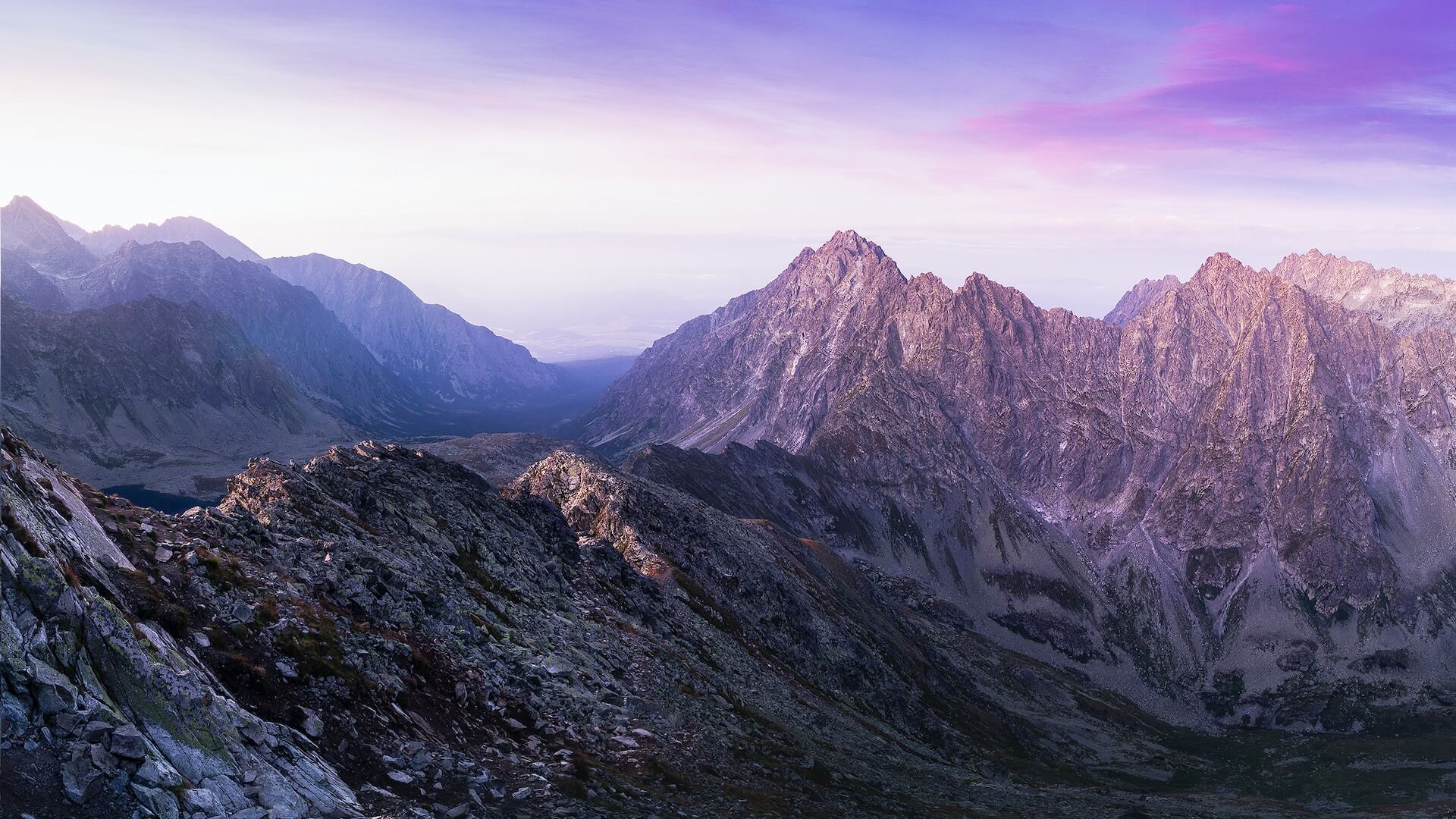
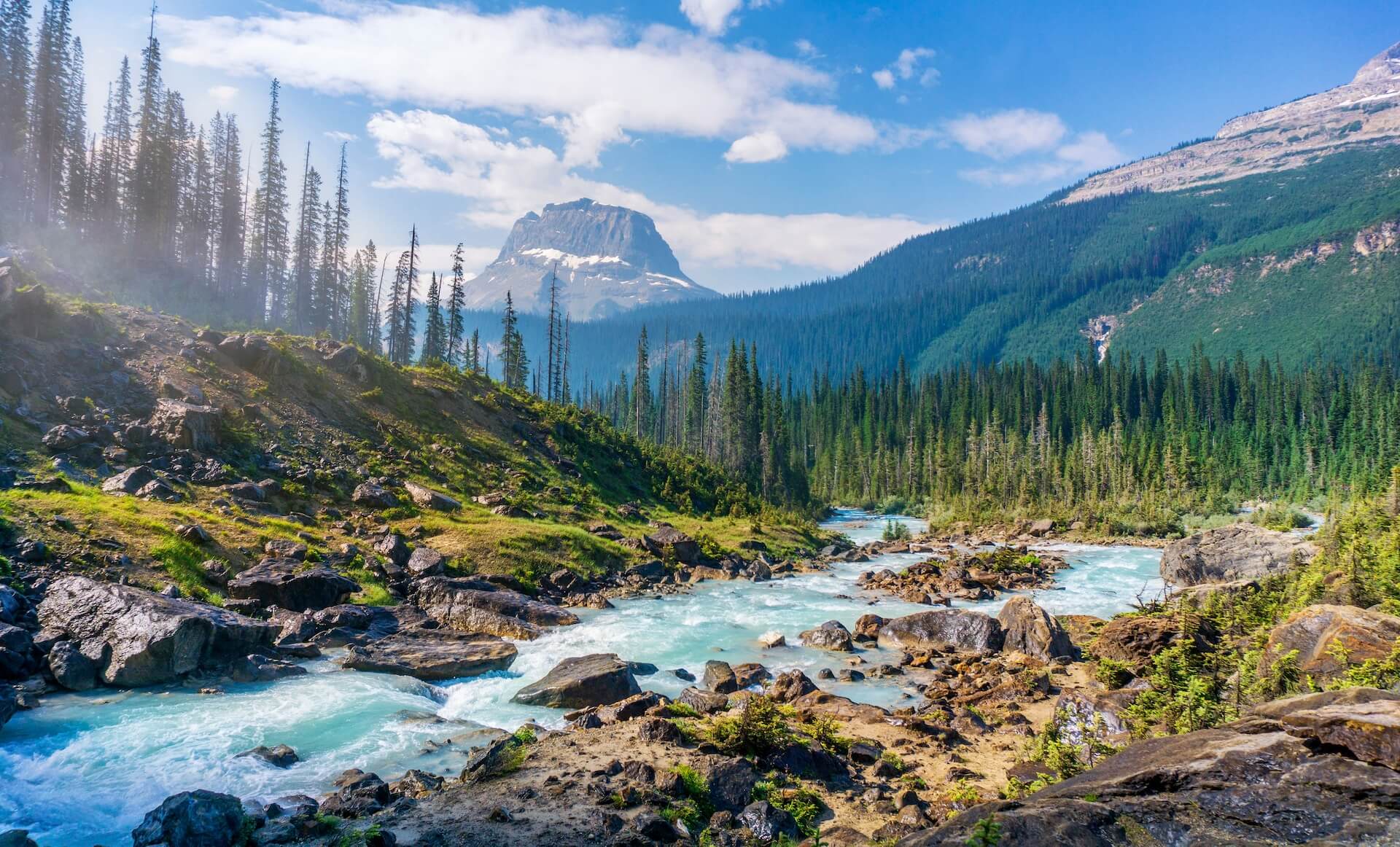
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function IndicatorsCarouselExample(props) {
const { slides } = props;
return (
<div class="flex flex-col gap-y-6">
<Carousel slides={slides} indicator />
<Carousel slides={slides} indicator="top" />
</div>
);
}
Carousel Direction
With direction props, you will be able to slide items from carousel horizontally (by default) or vertically !
Below an example of the design.
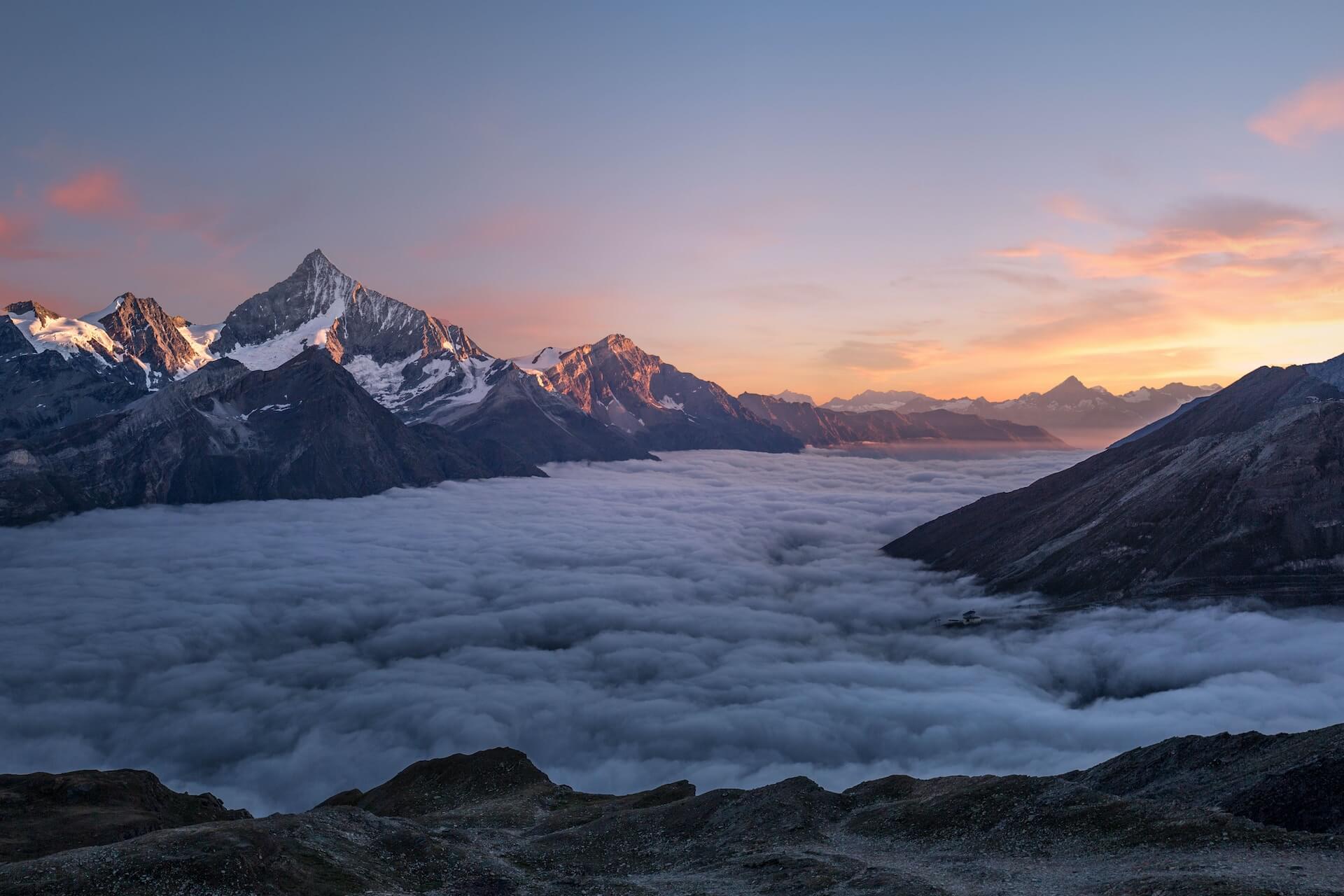
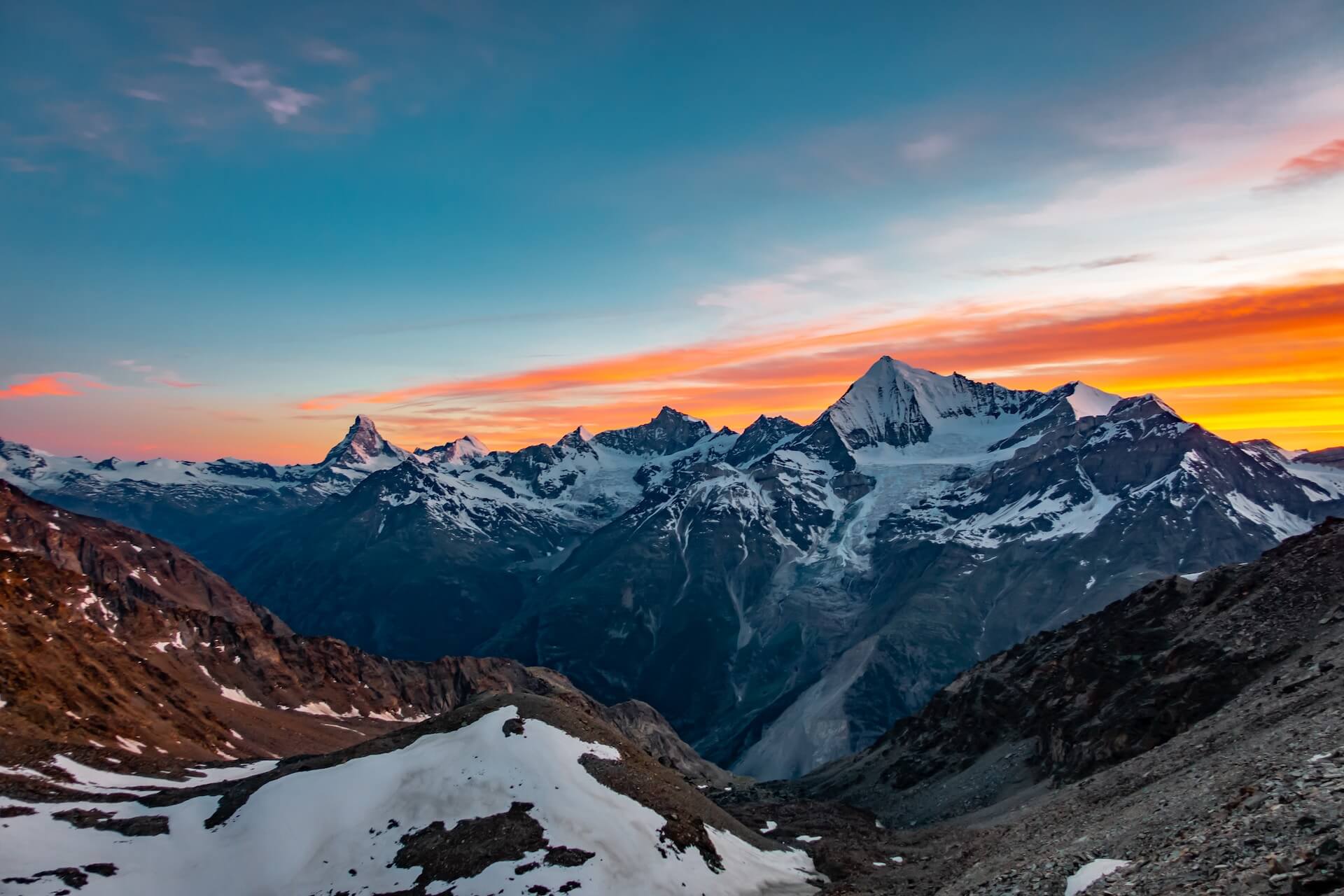
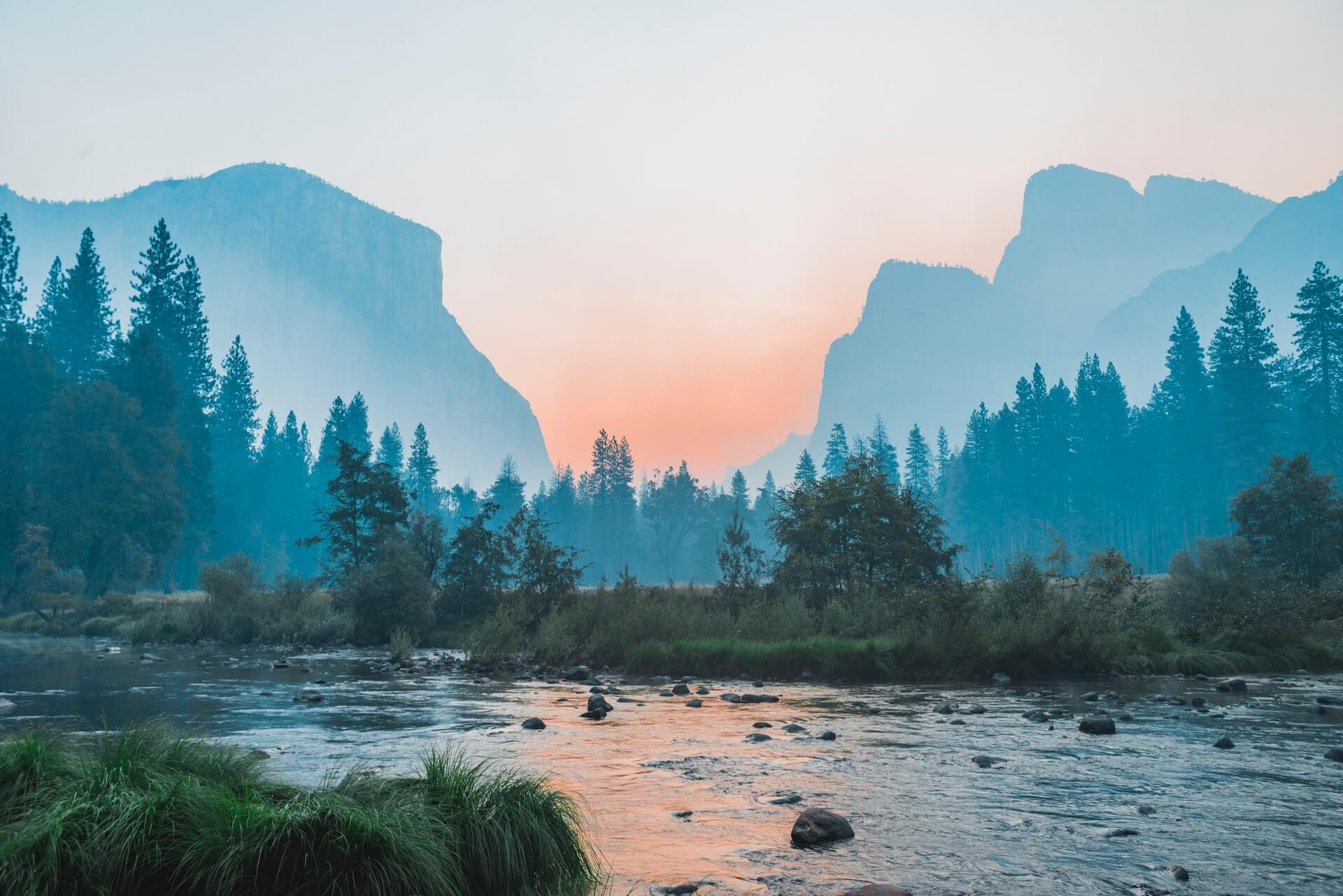
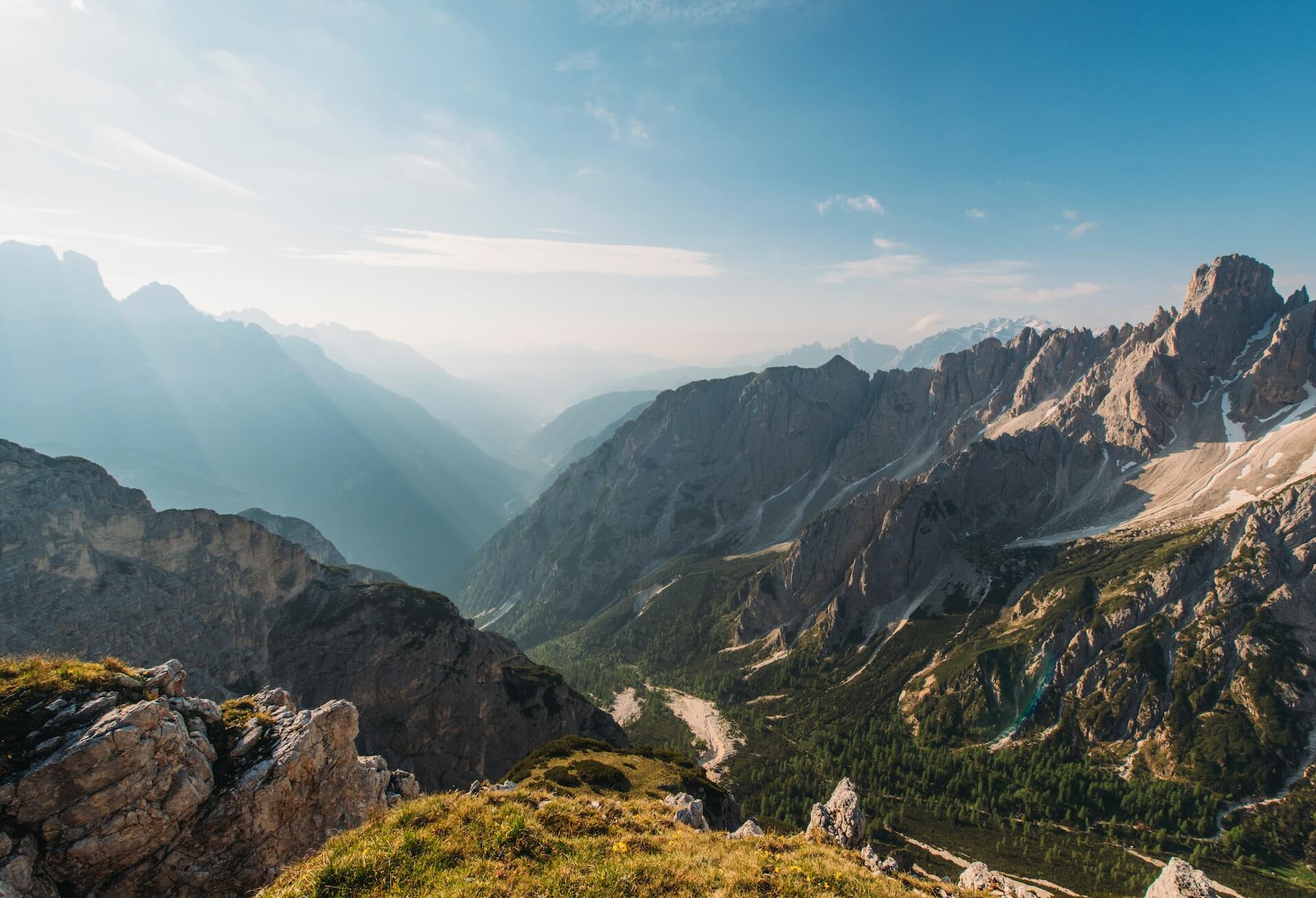
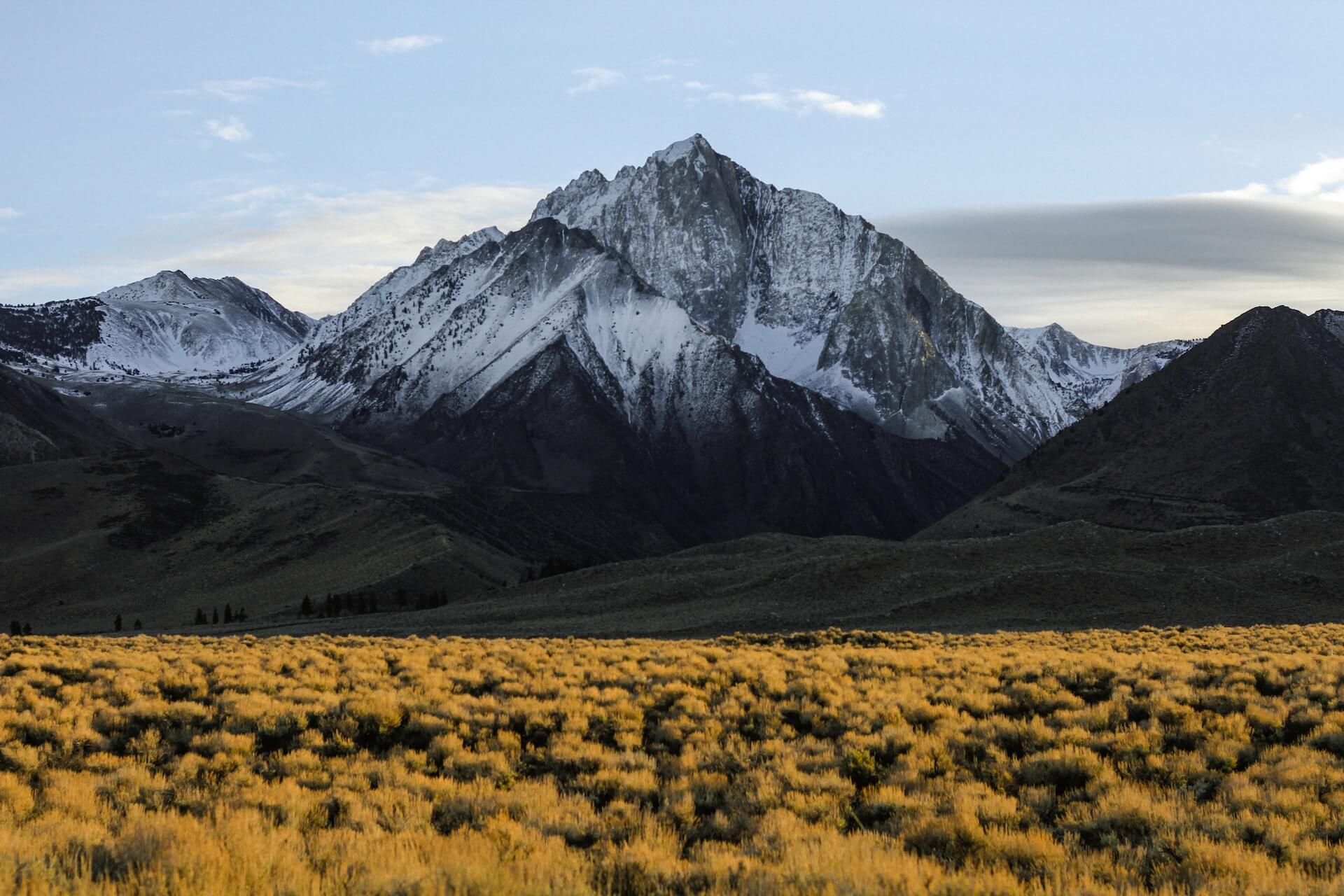
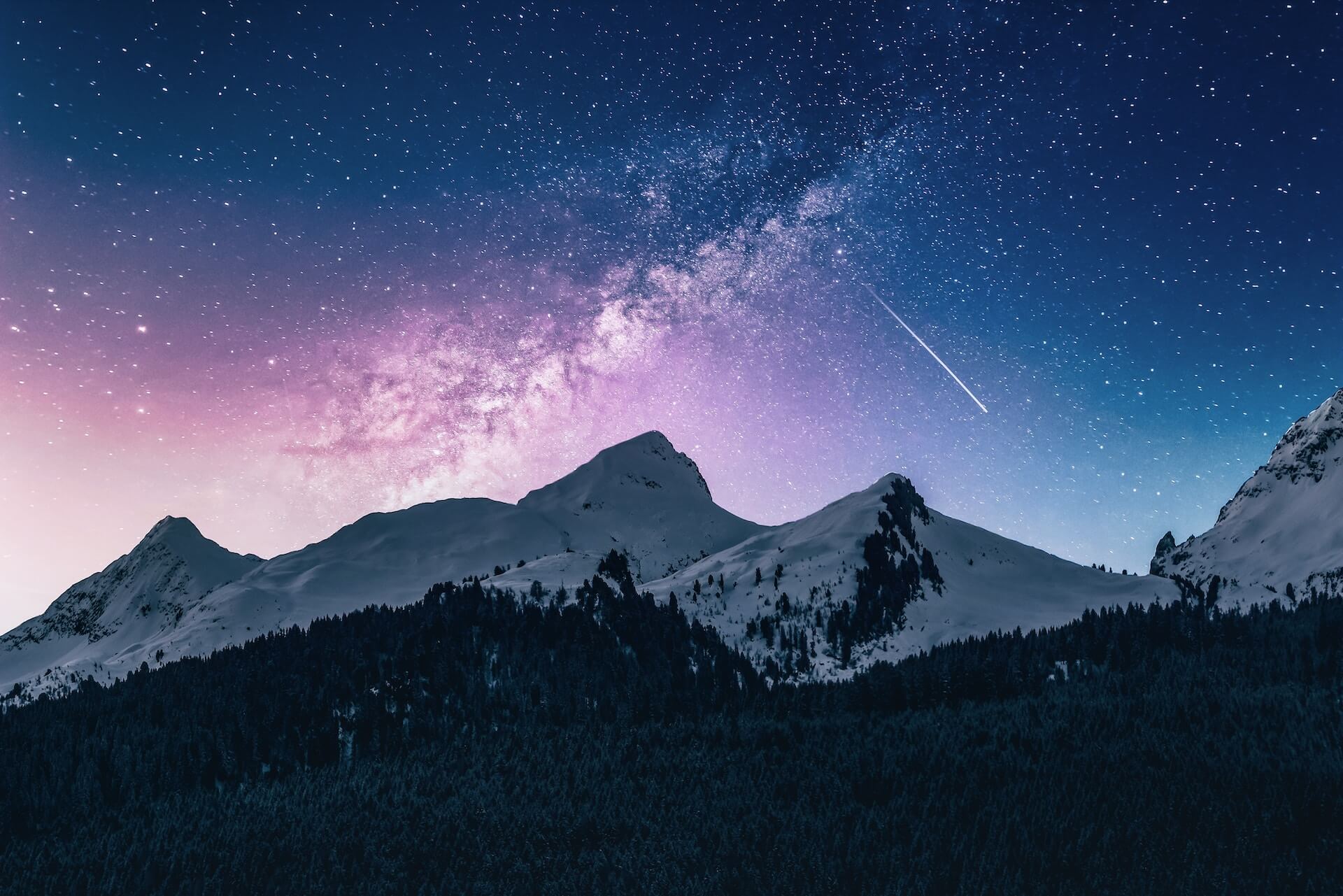
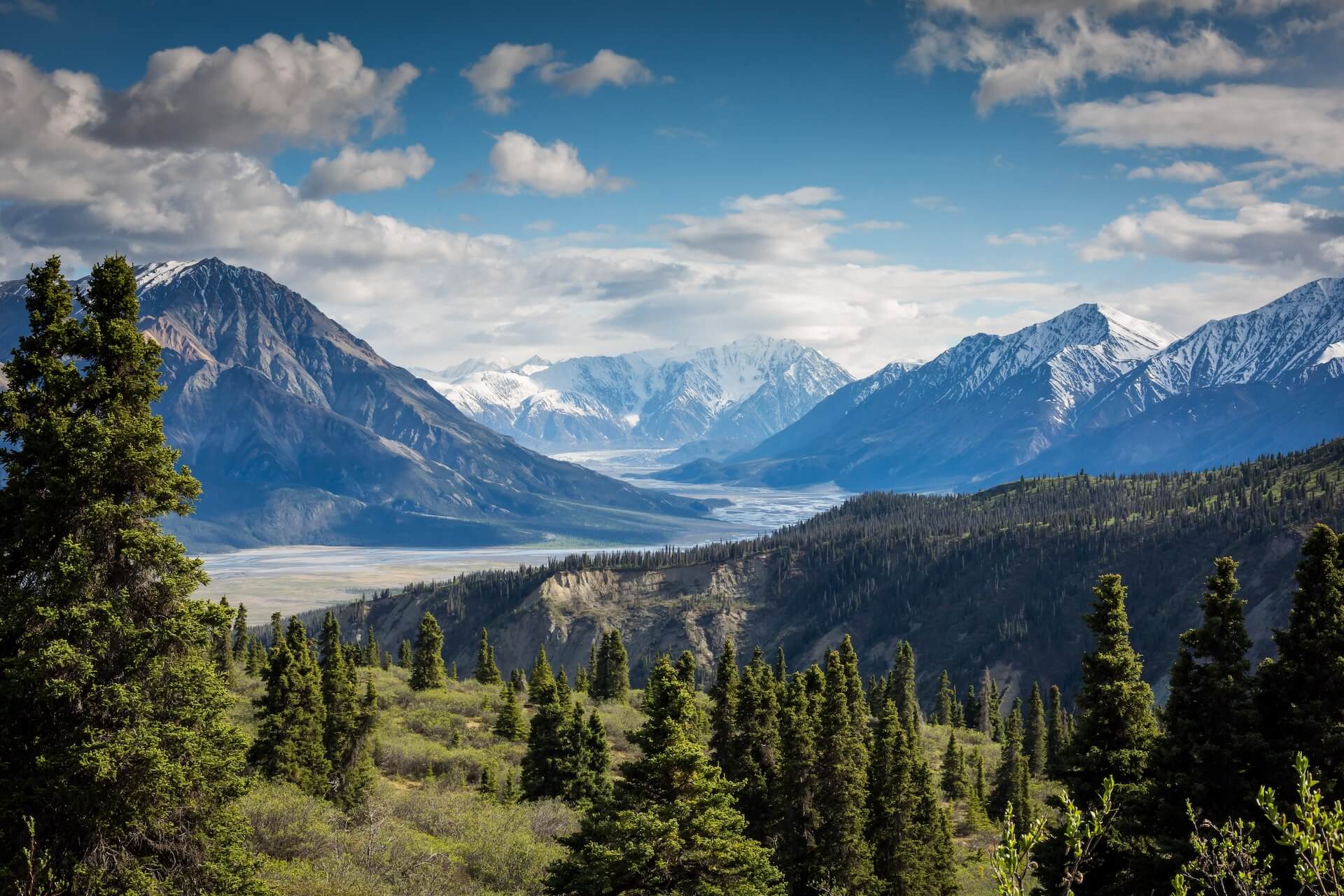
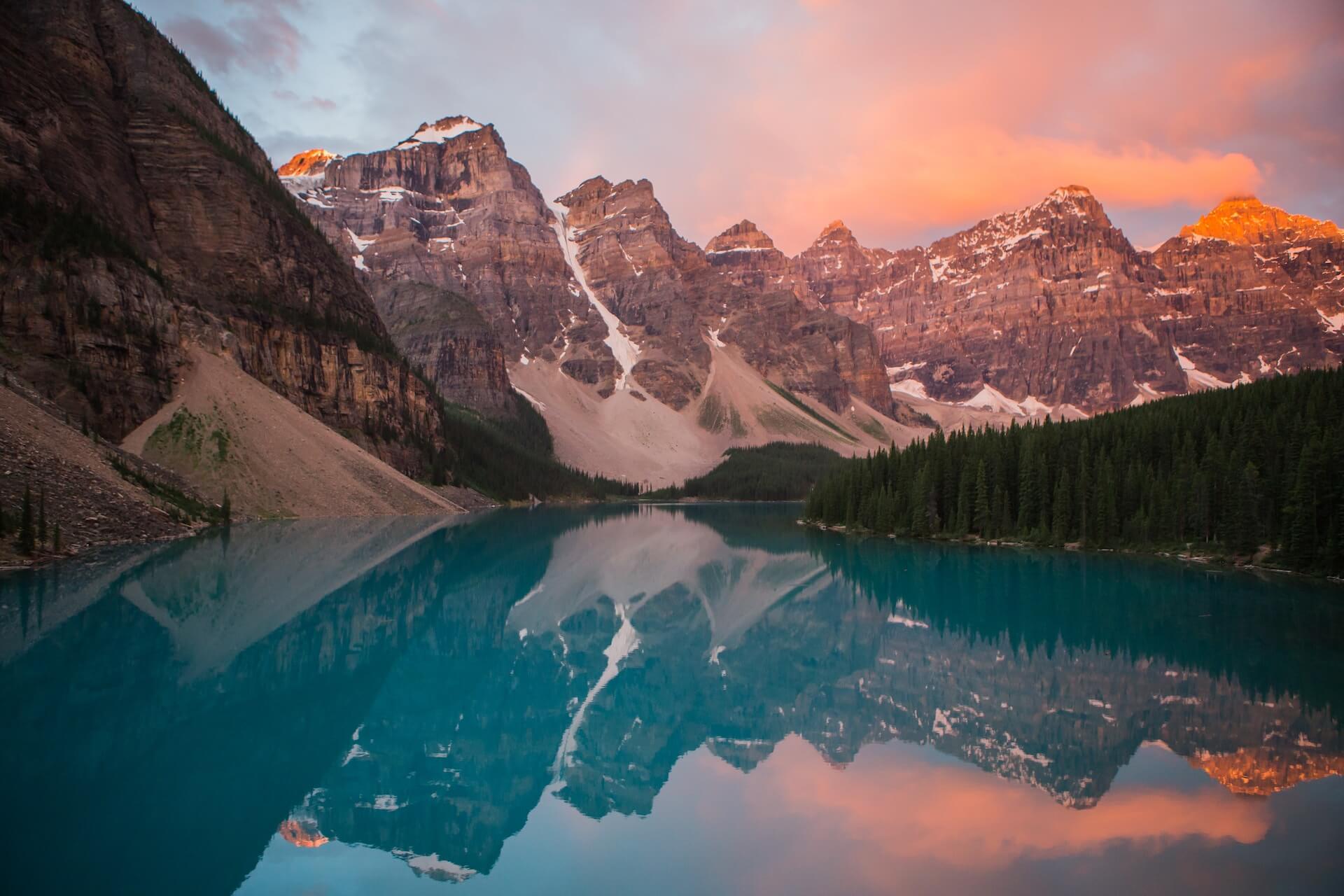
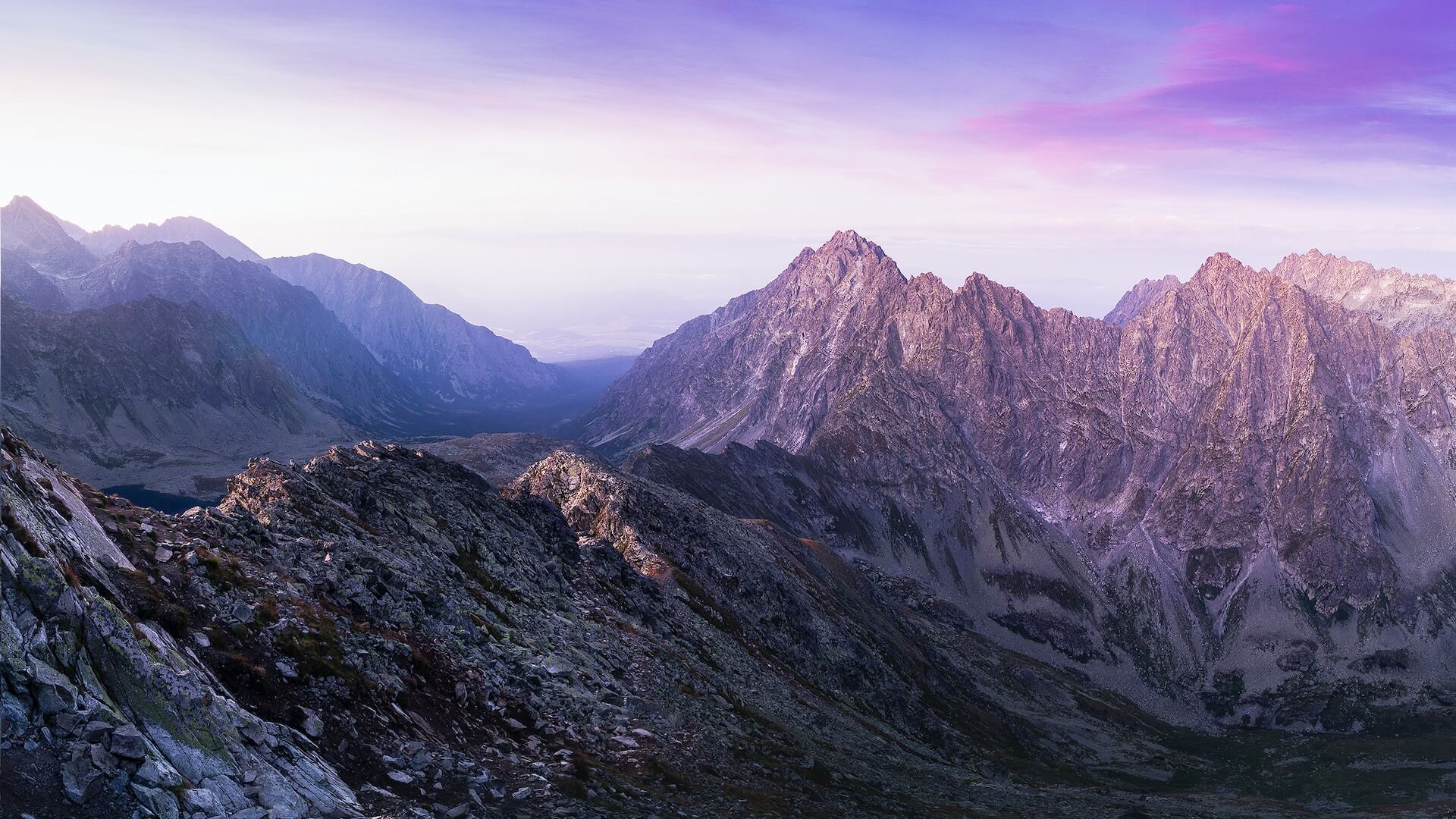
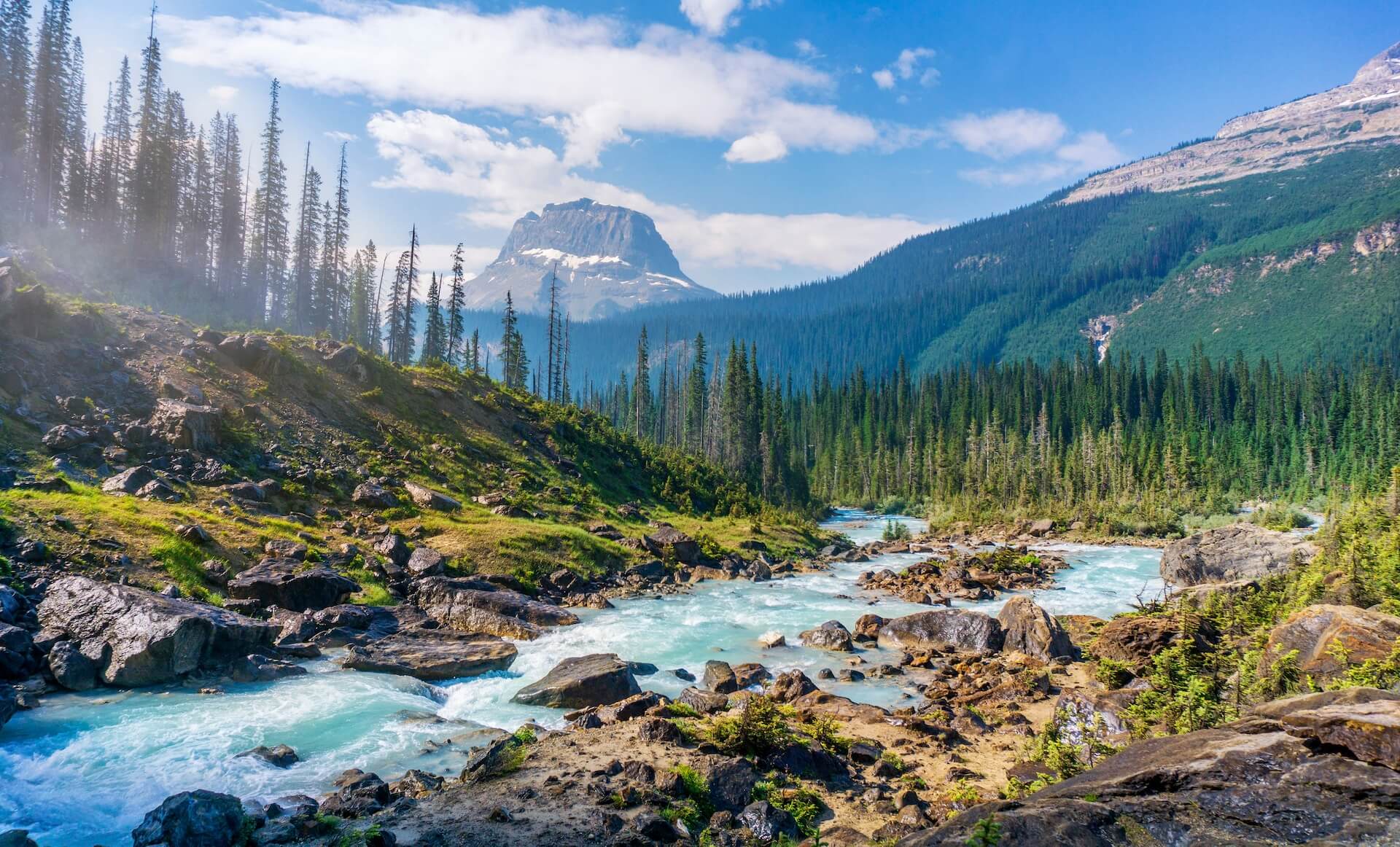
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function DirectionCarouselExample(props) {
const { slides } = props;
return (
<div class="flex flex-col gap-y-4">
<Carousel slides={slides} indicator="right" direction="vertical" />
</div>
);
}
Carousel Loop
Loop props allow to return to first element when item is last and vice-versa.
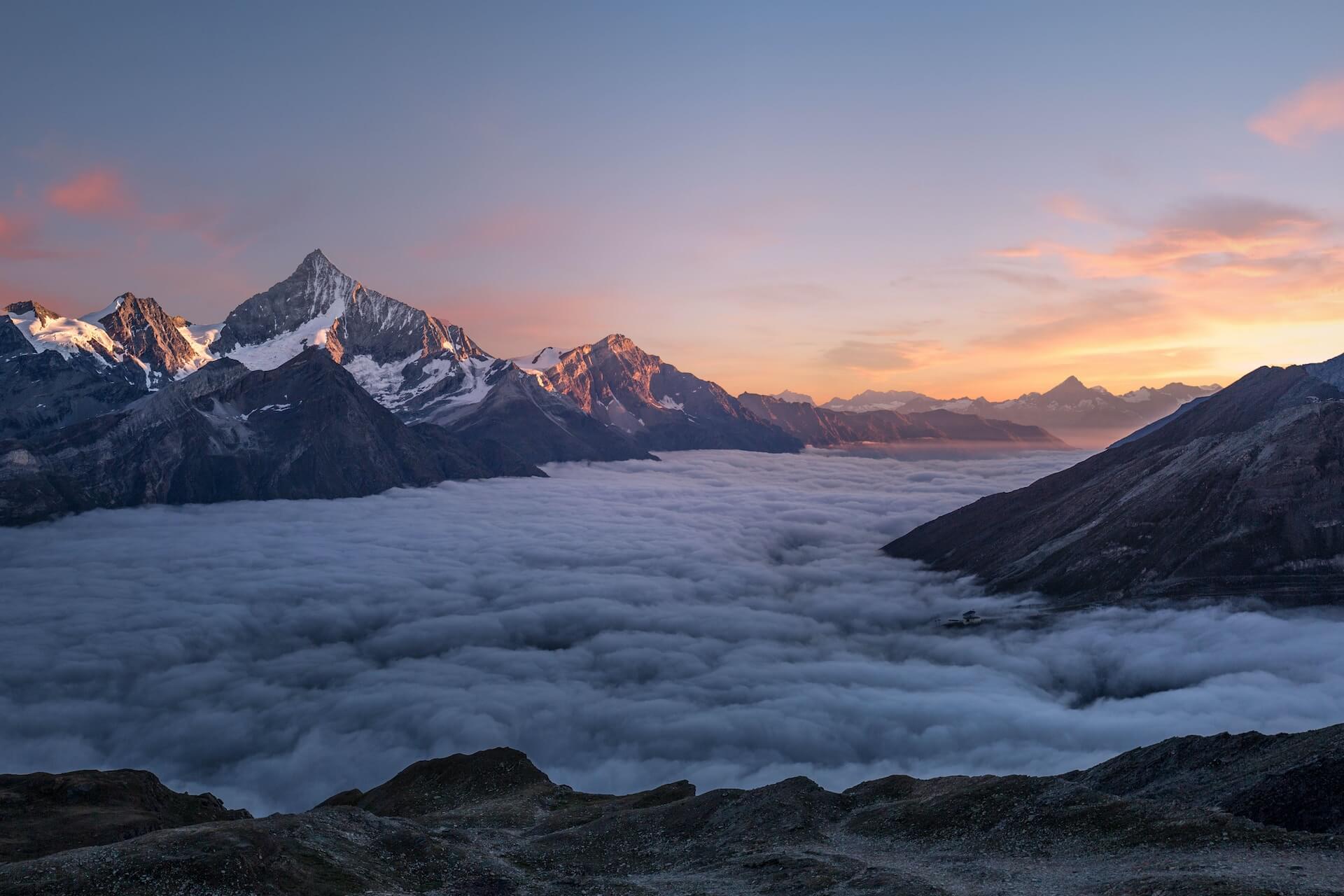
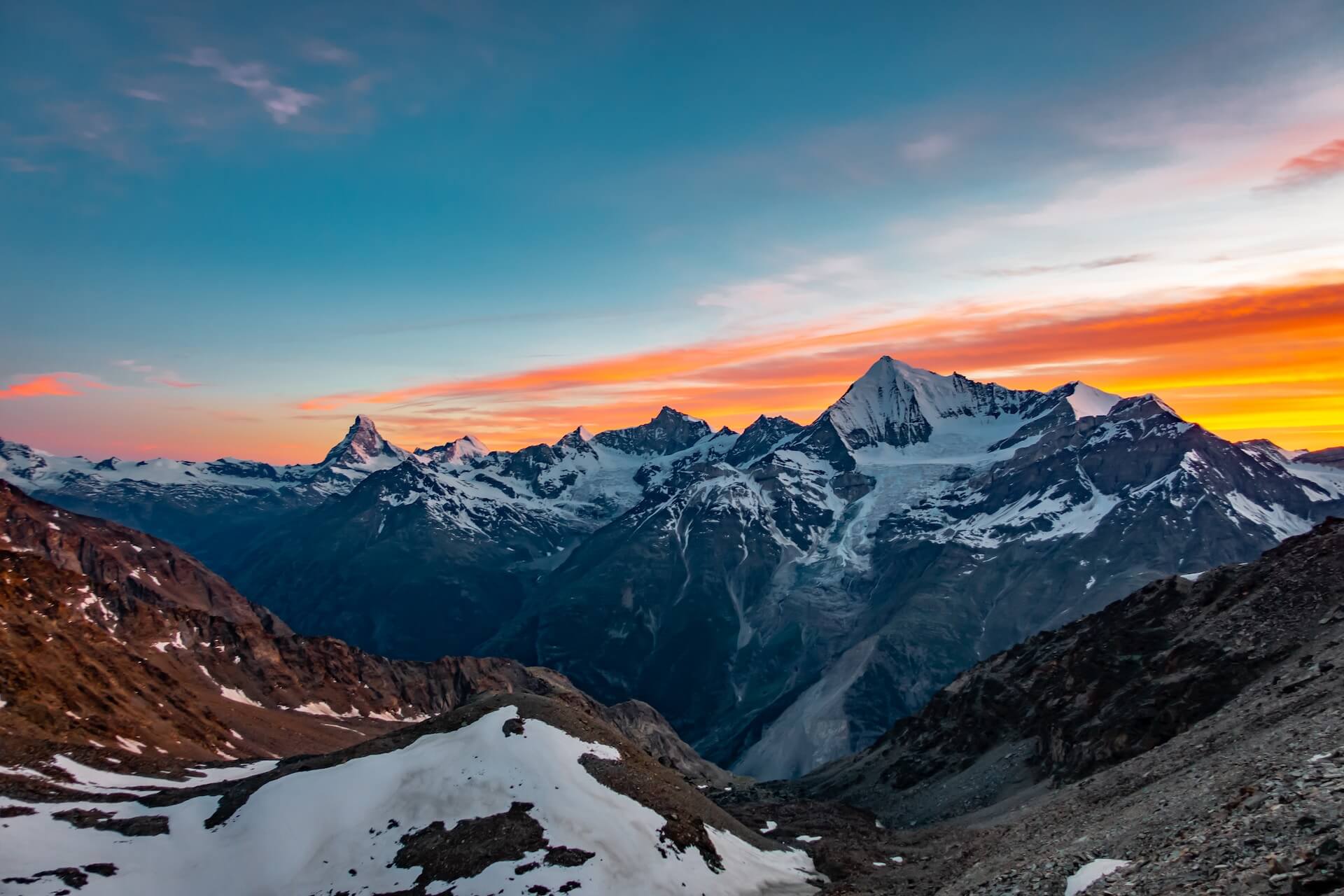
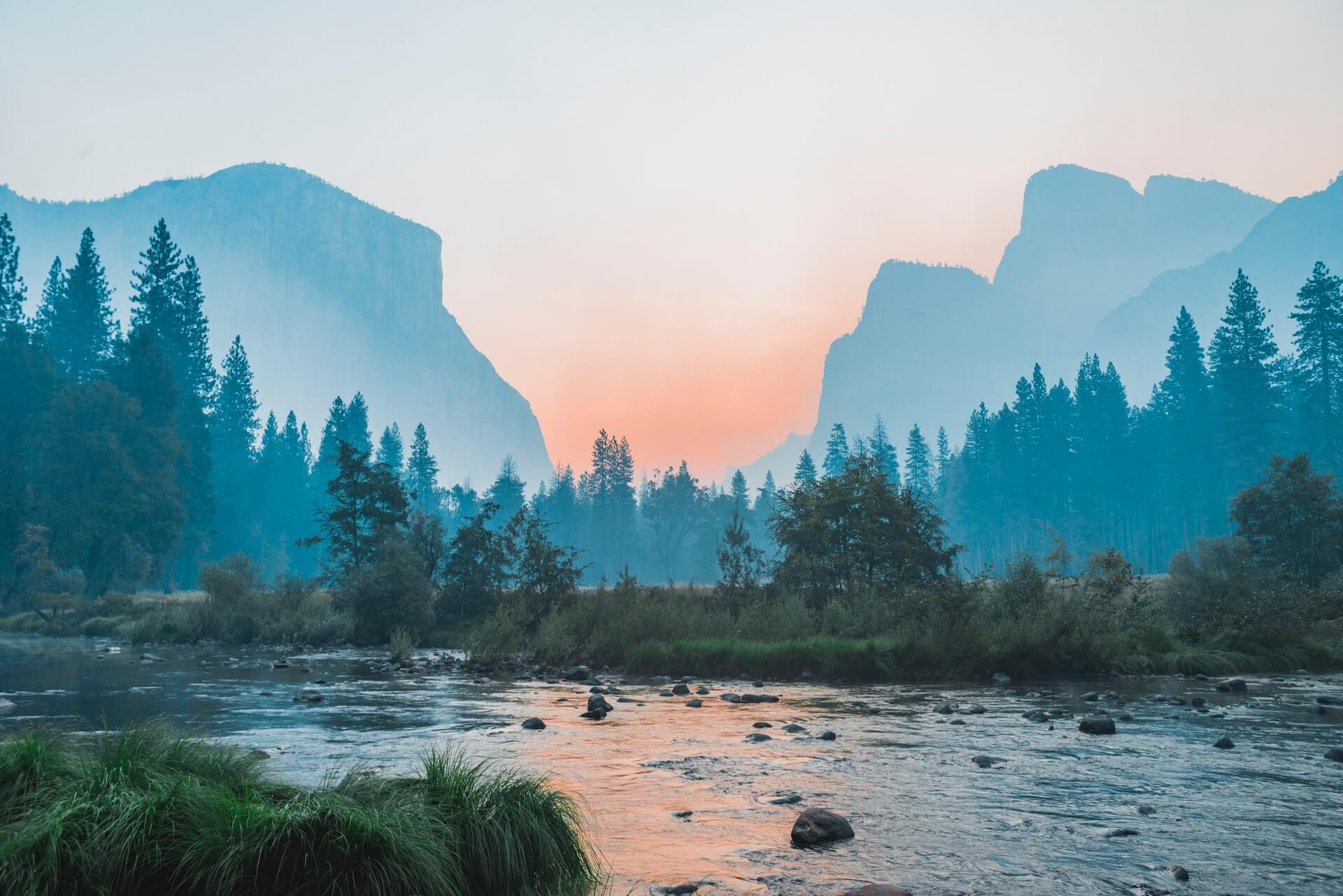
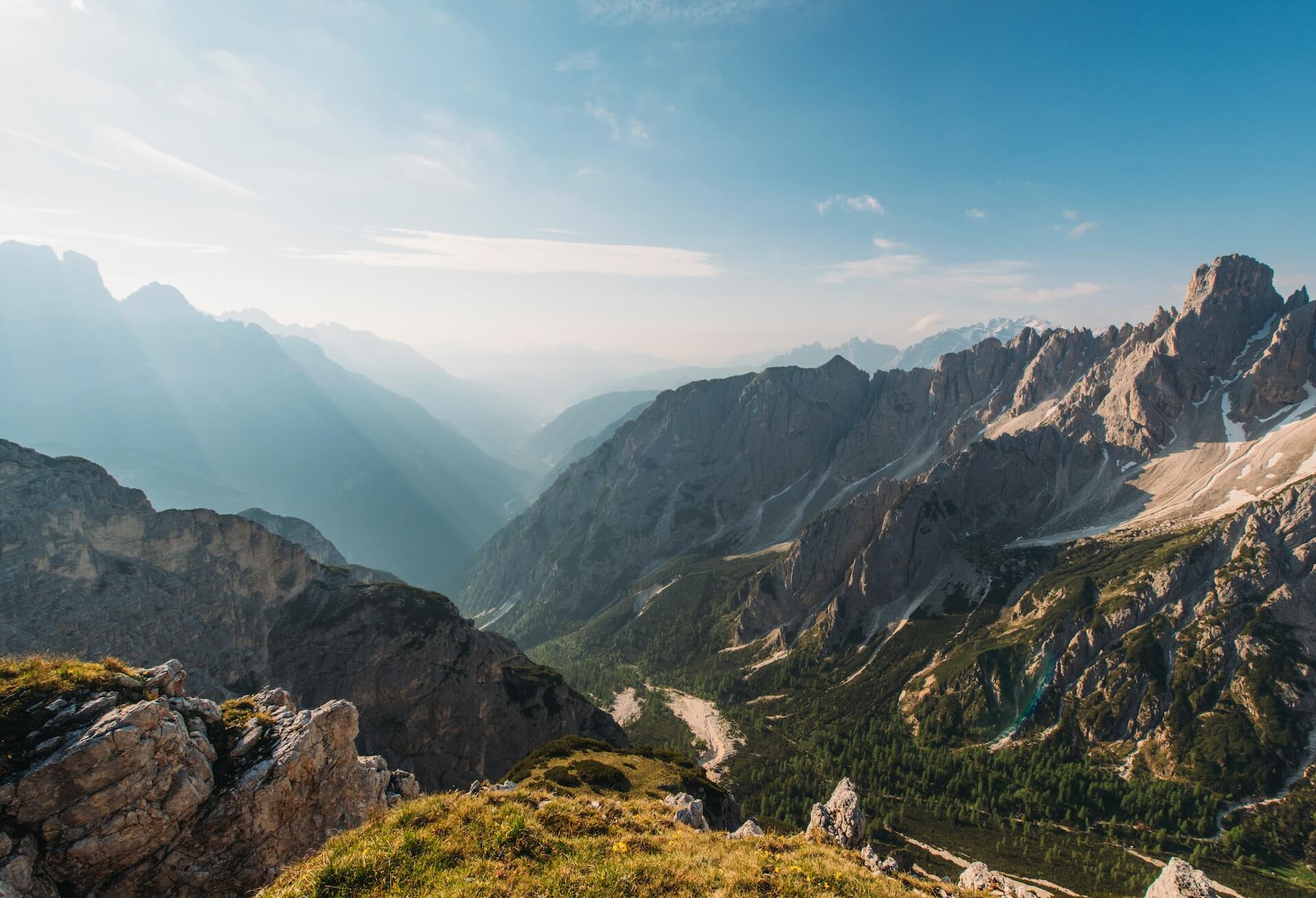
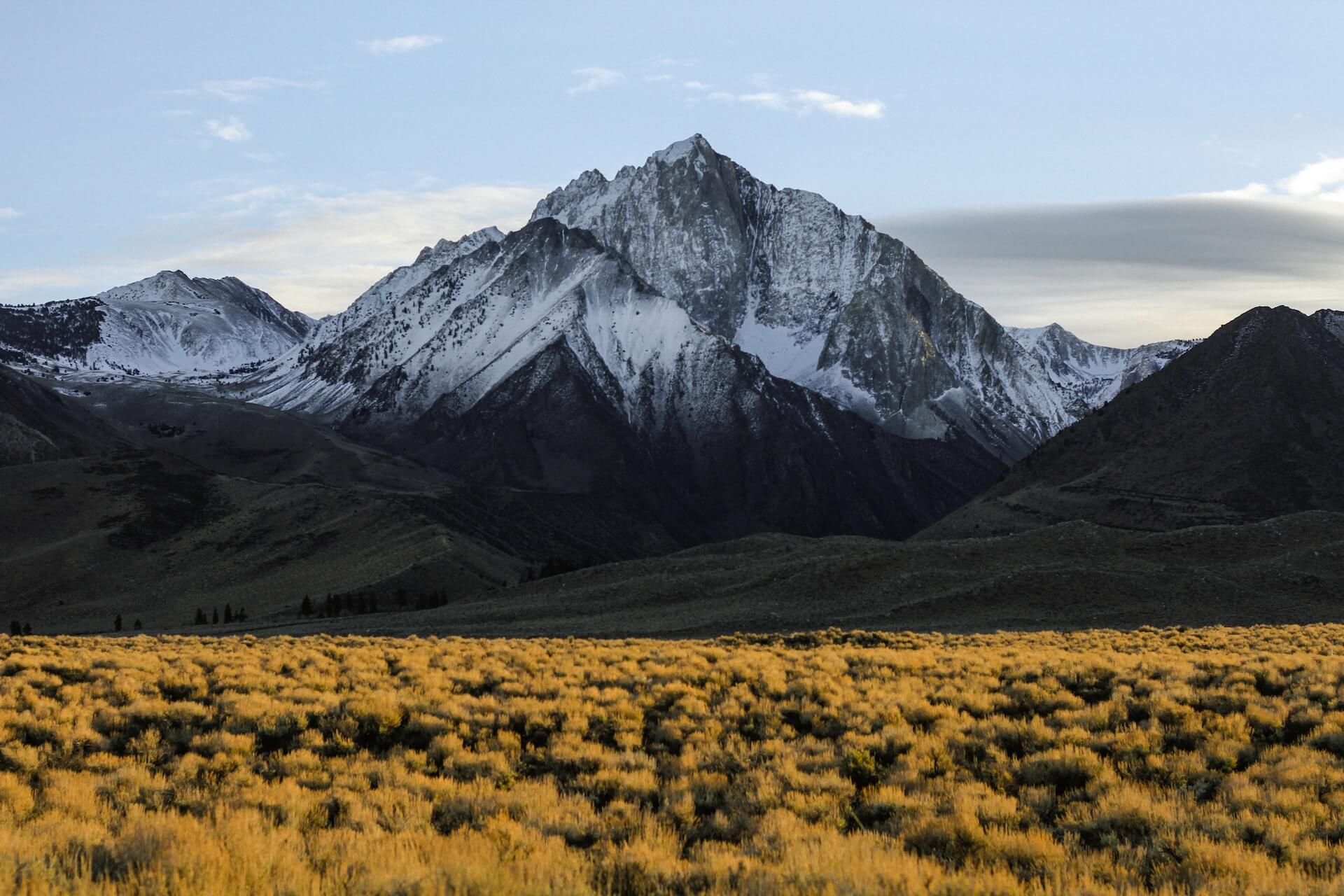
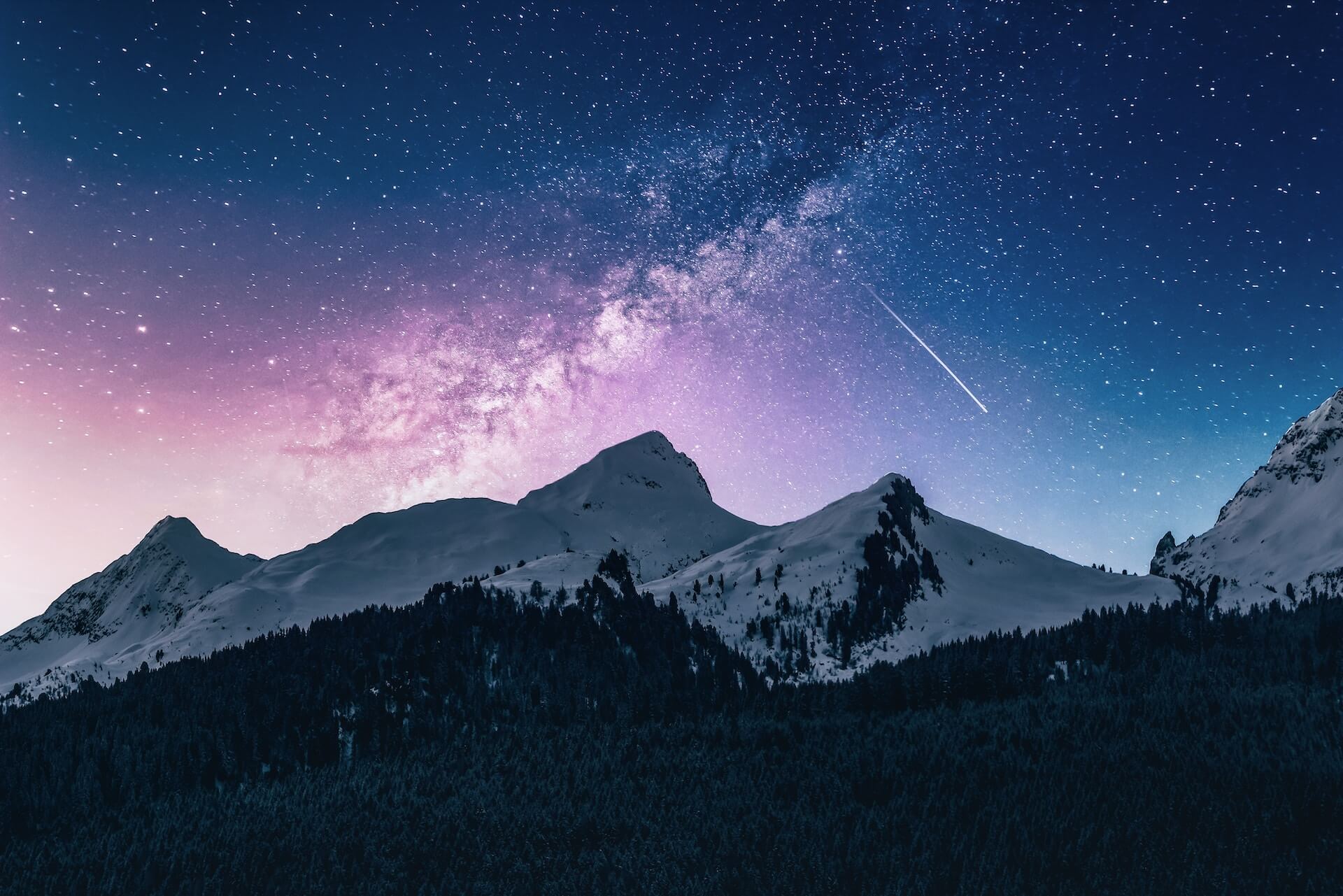
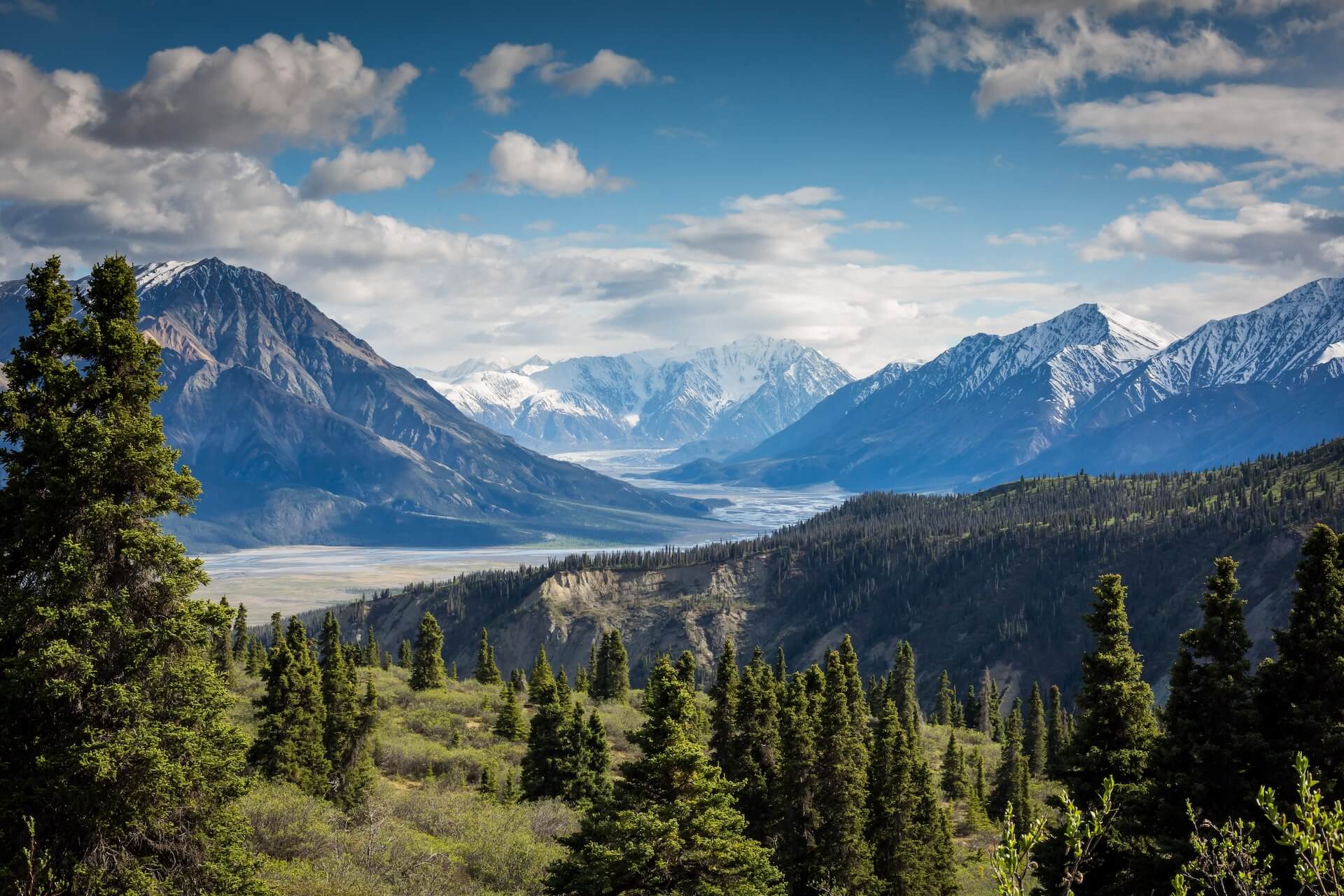
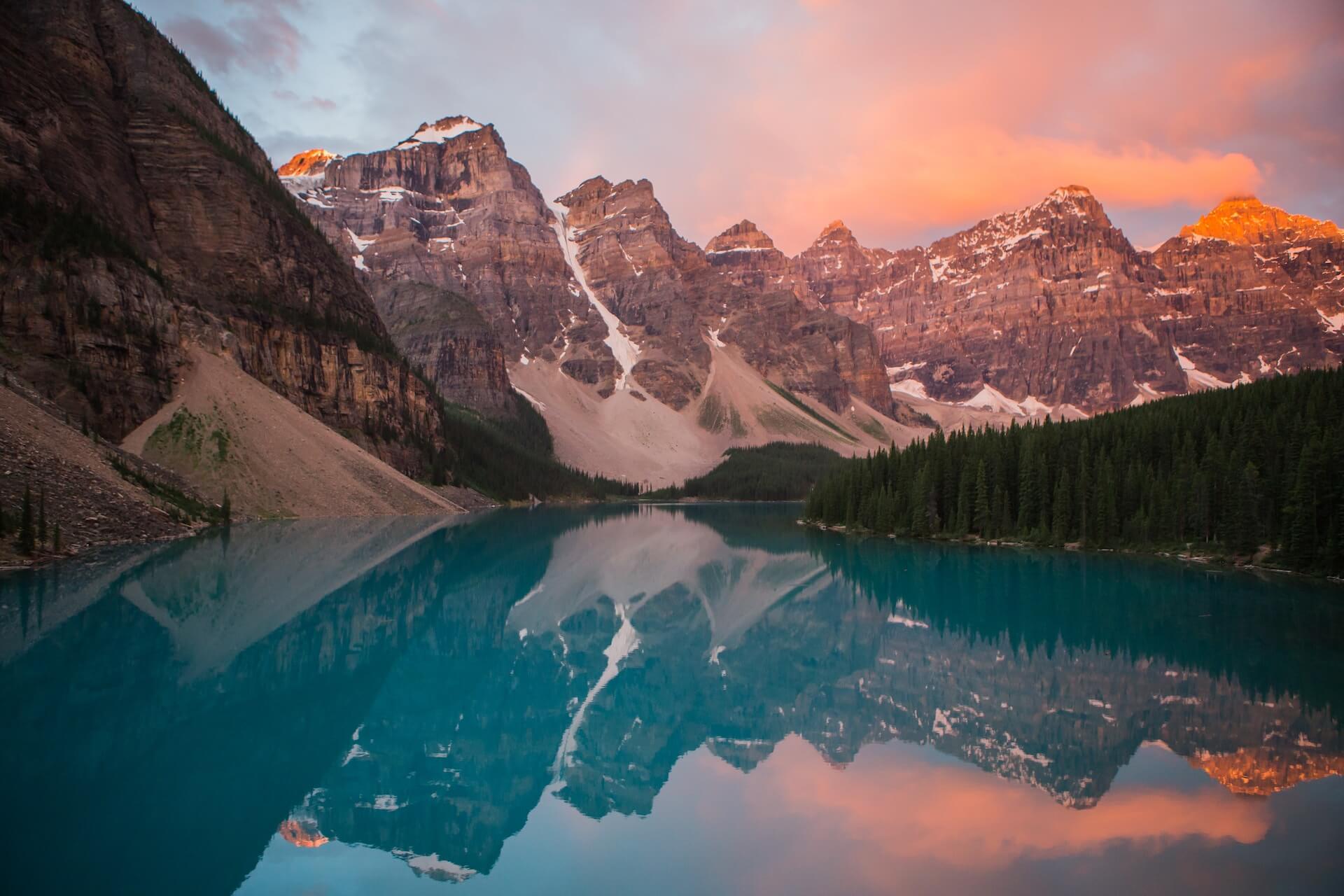
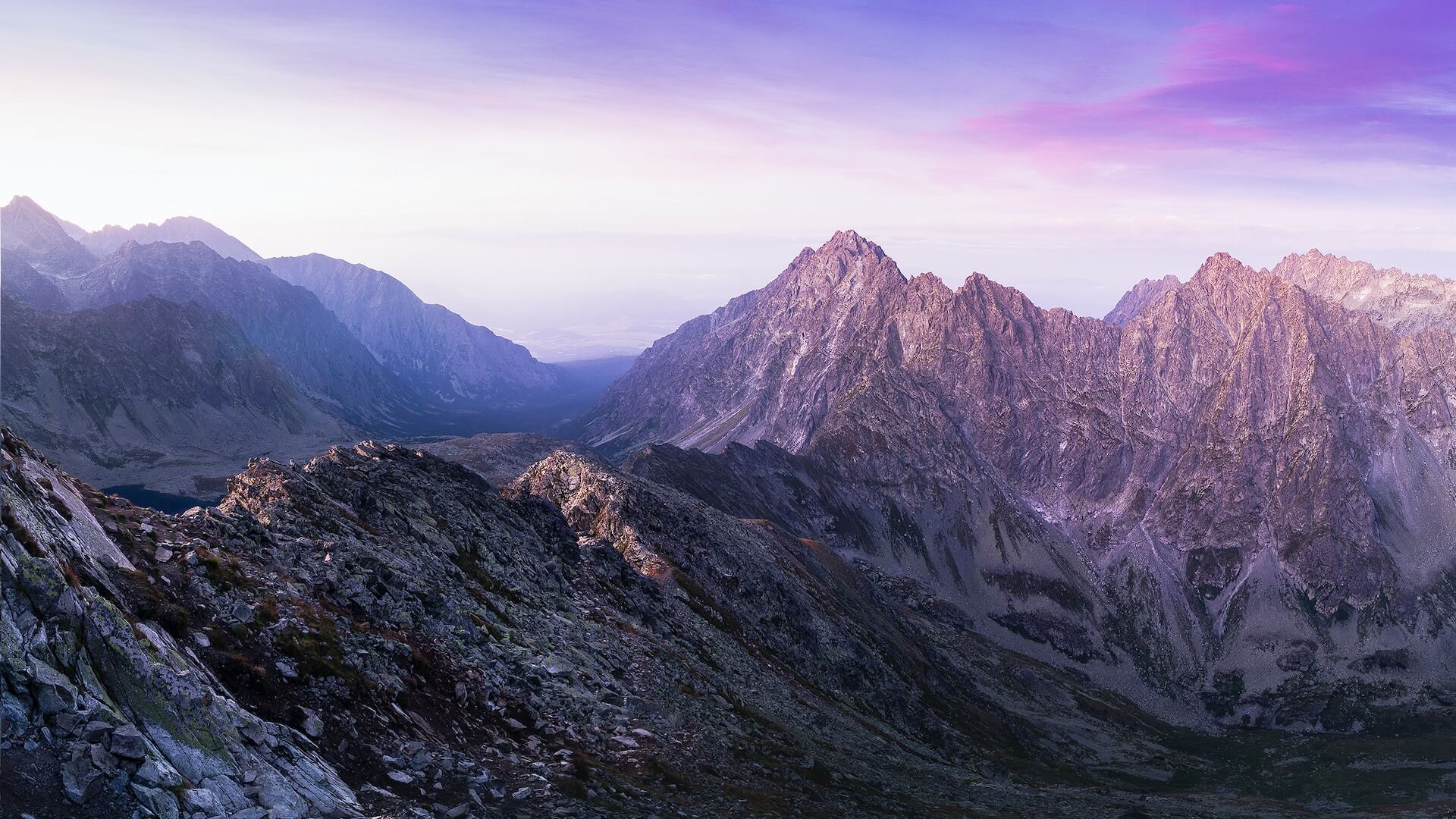
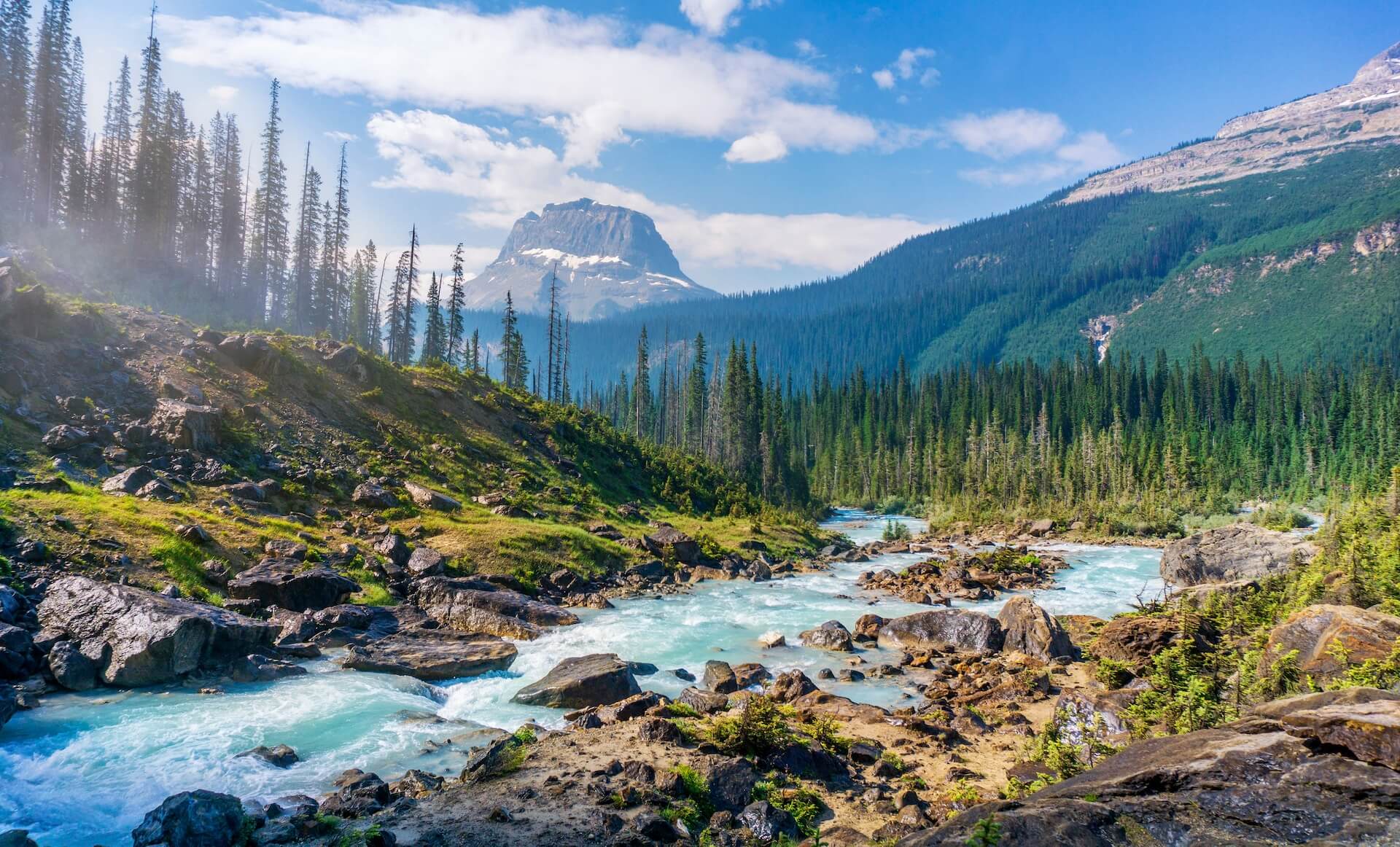
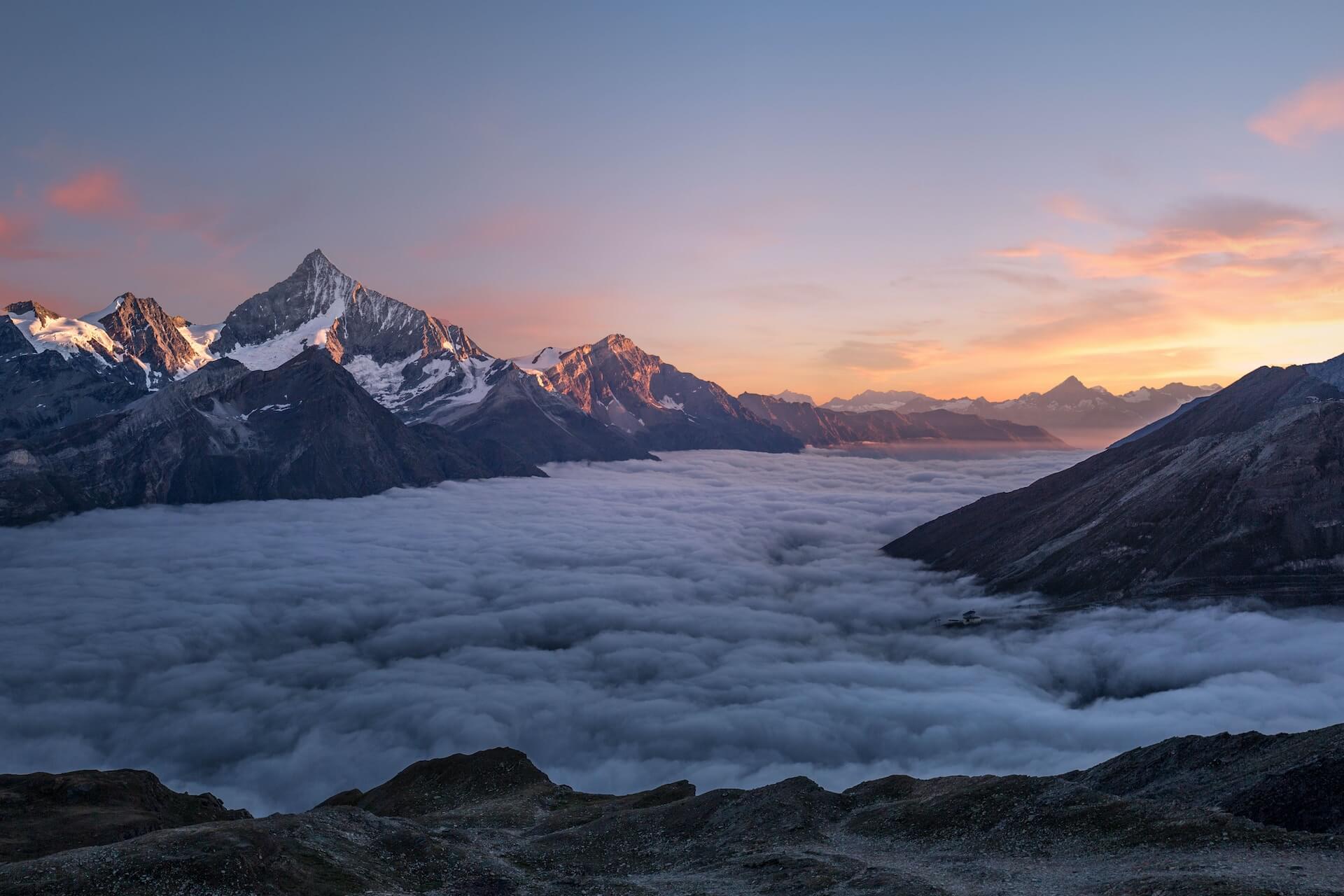
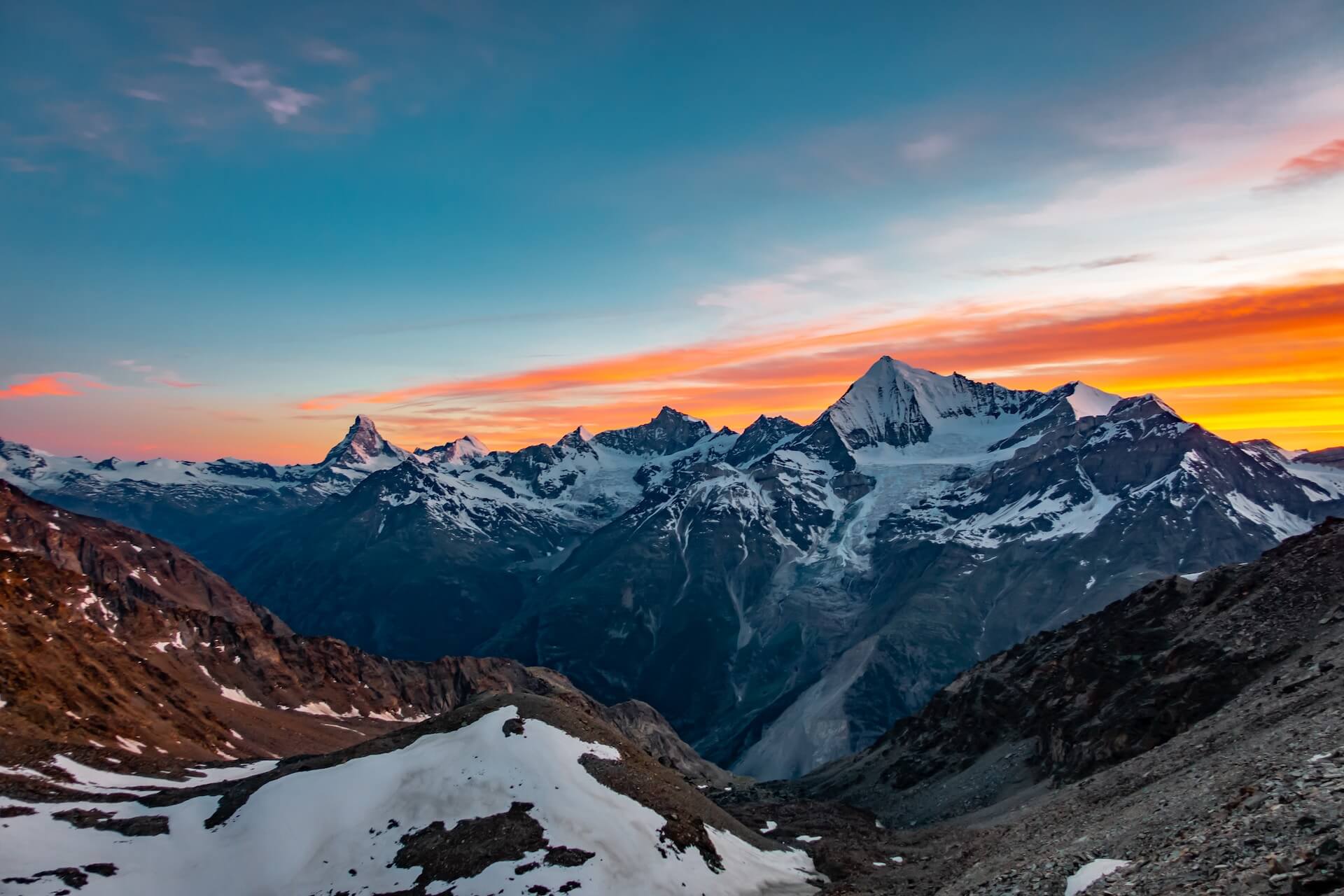
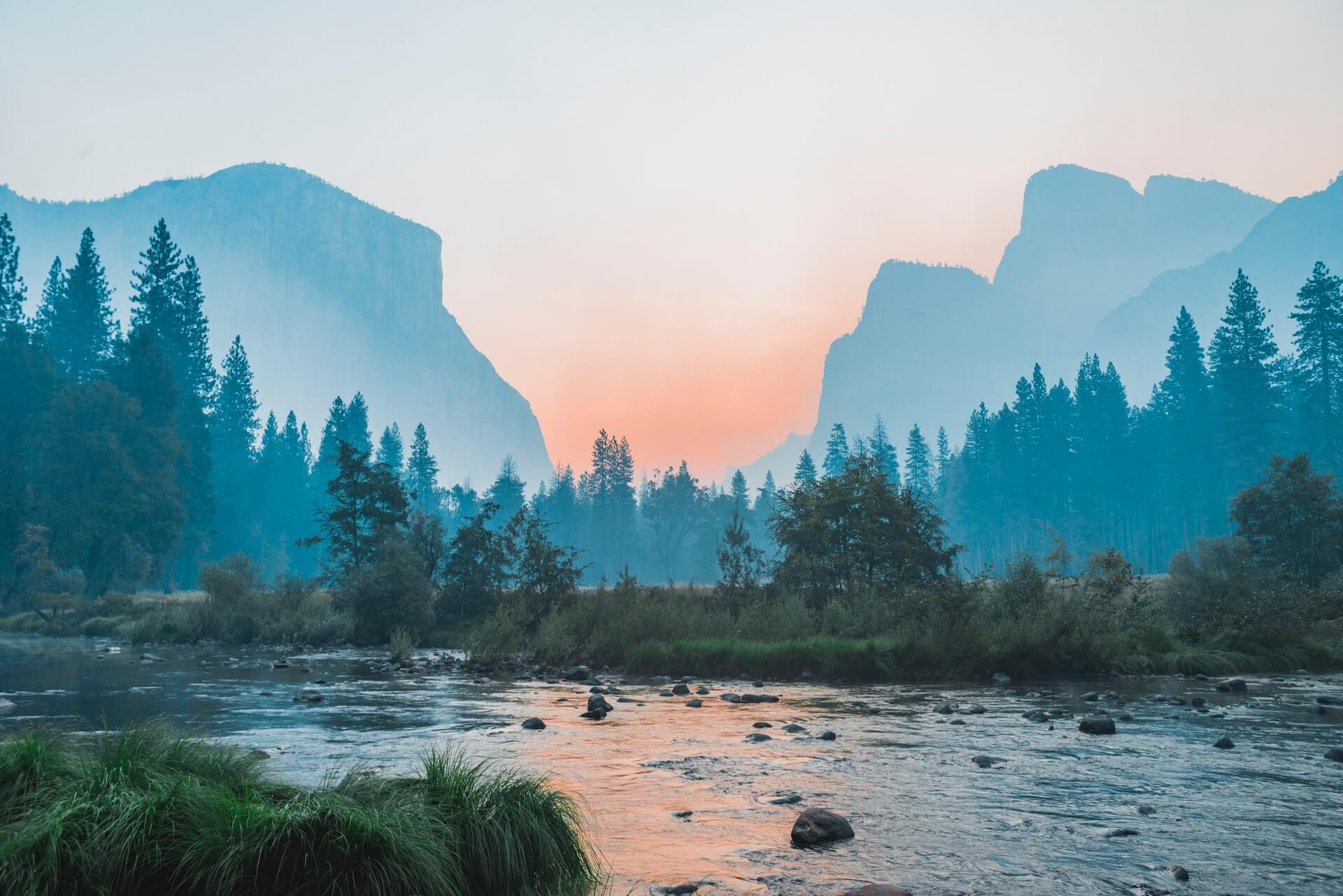
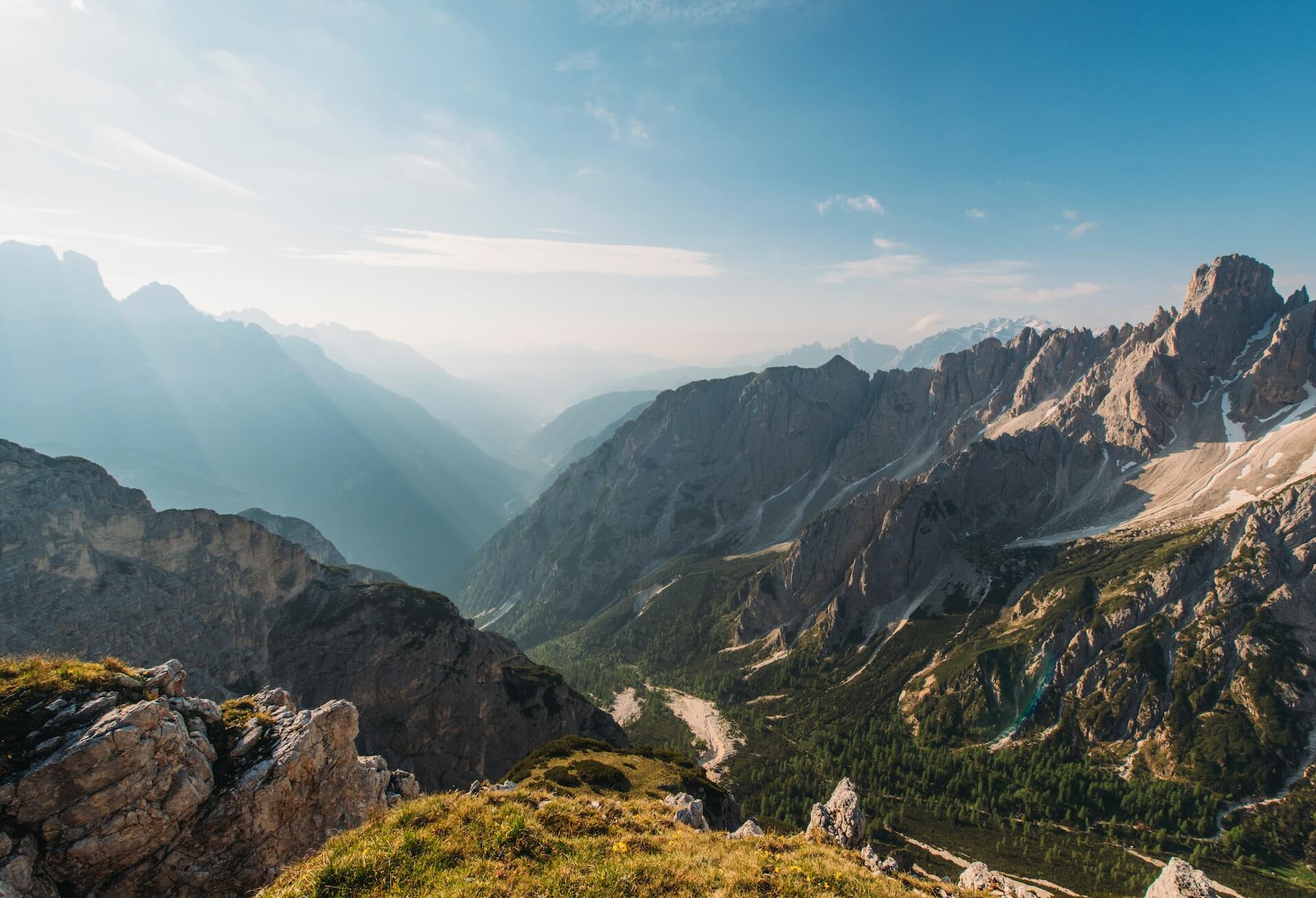
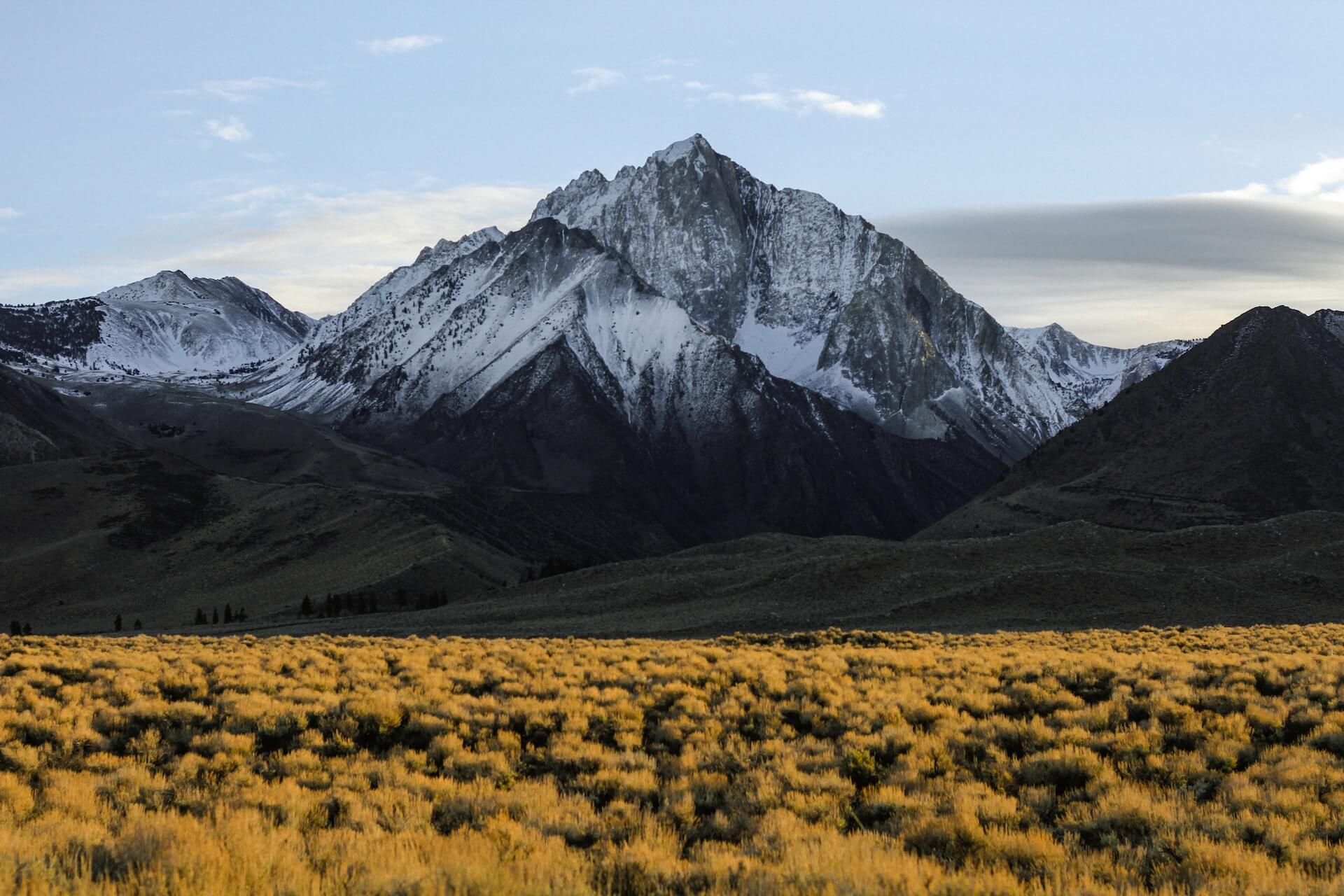
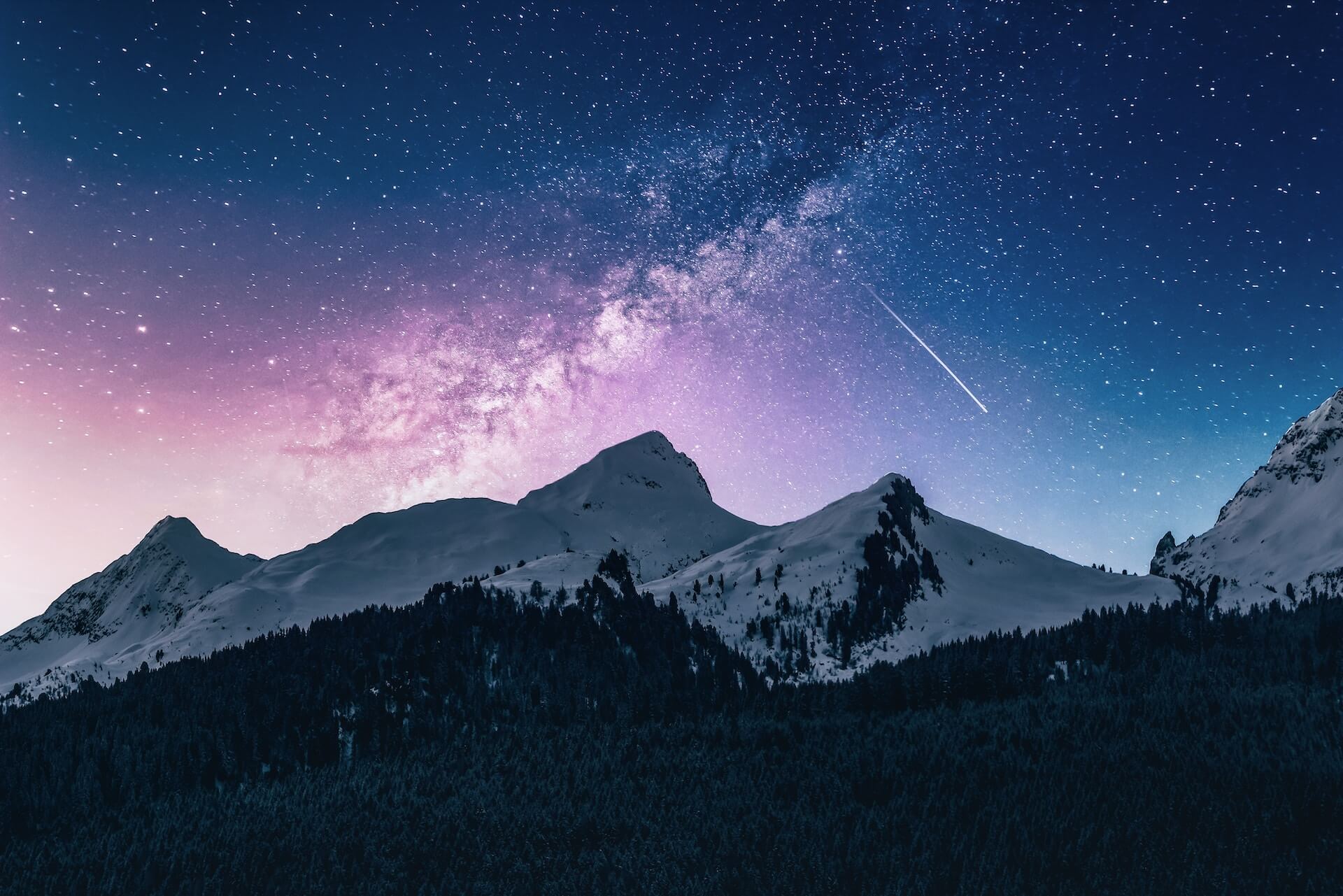
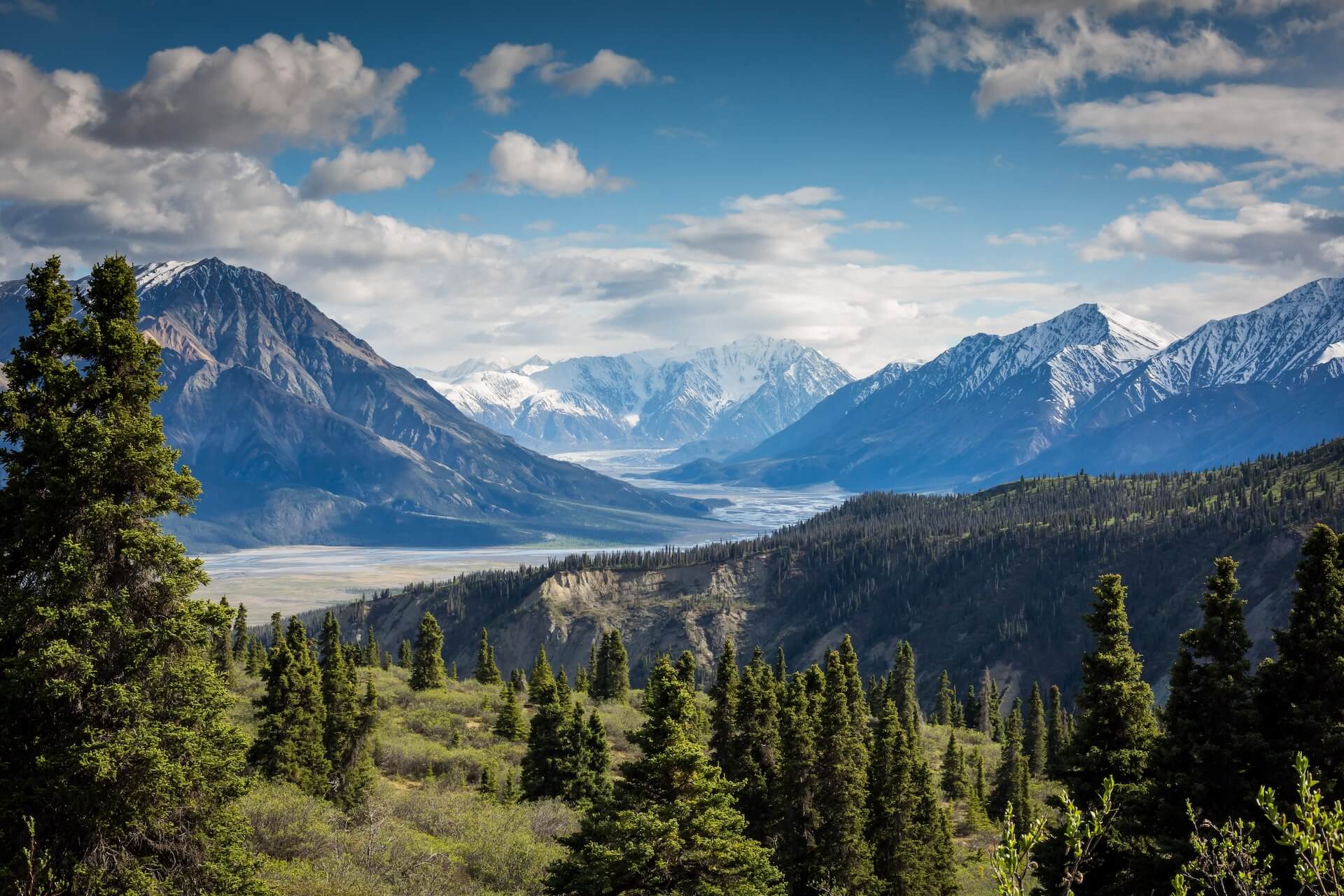
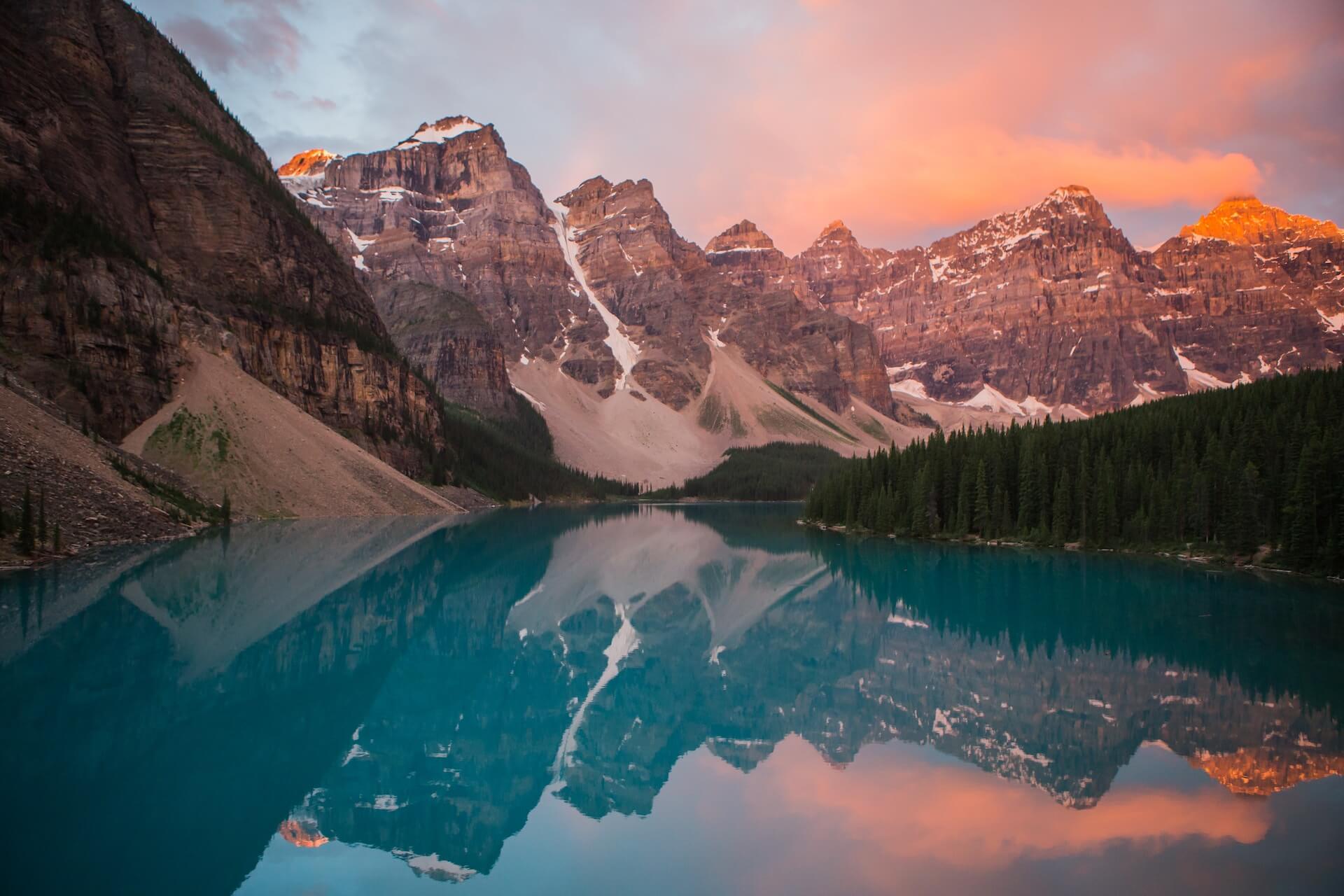
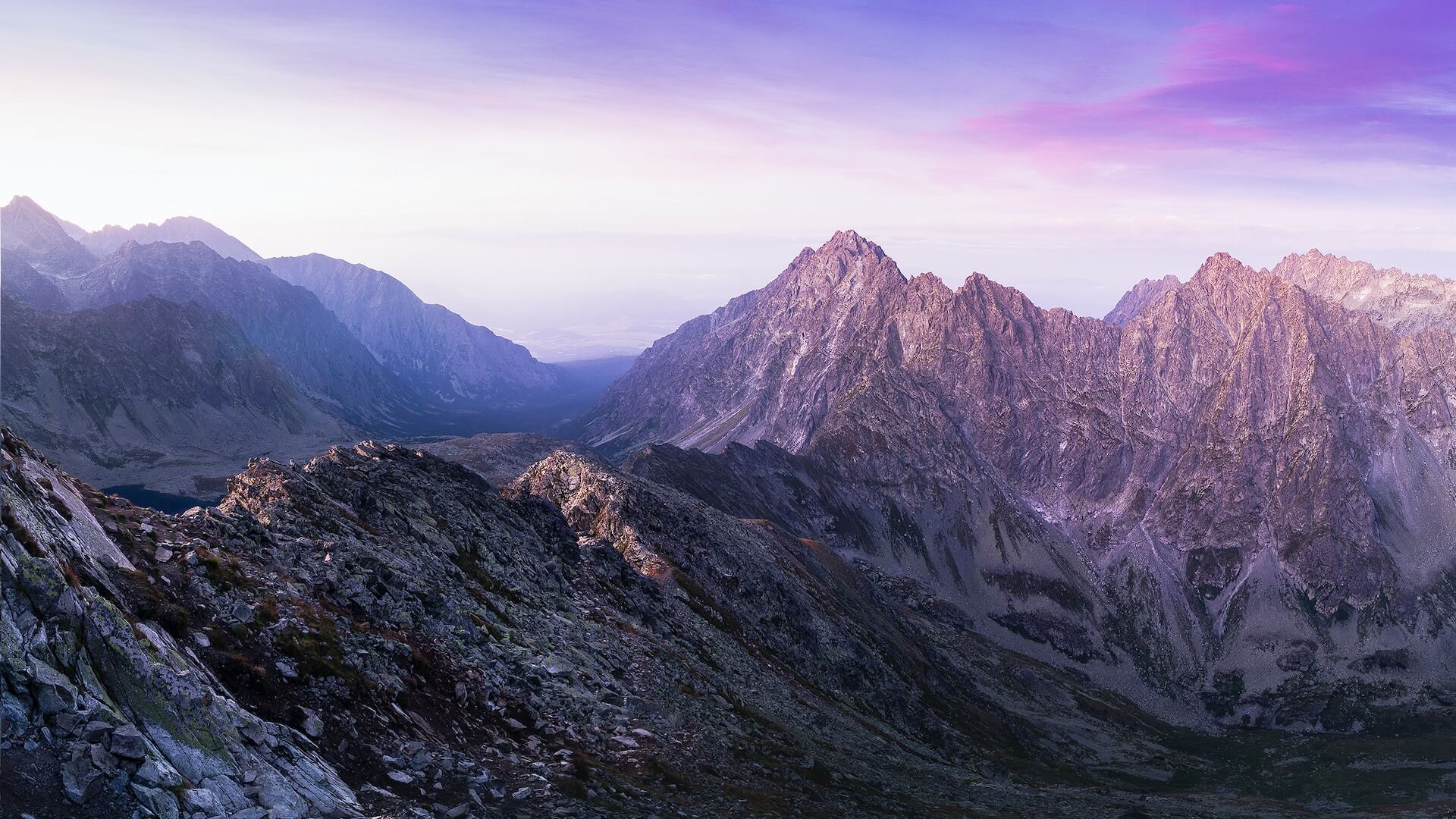
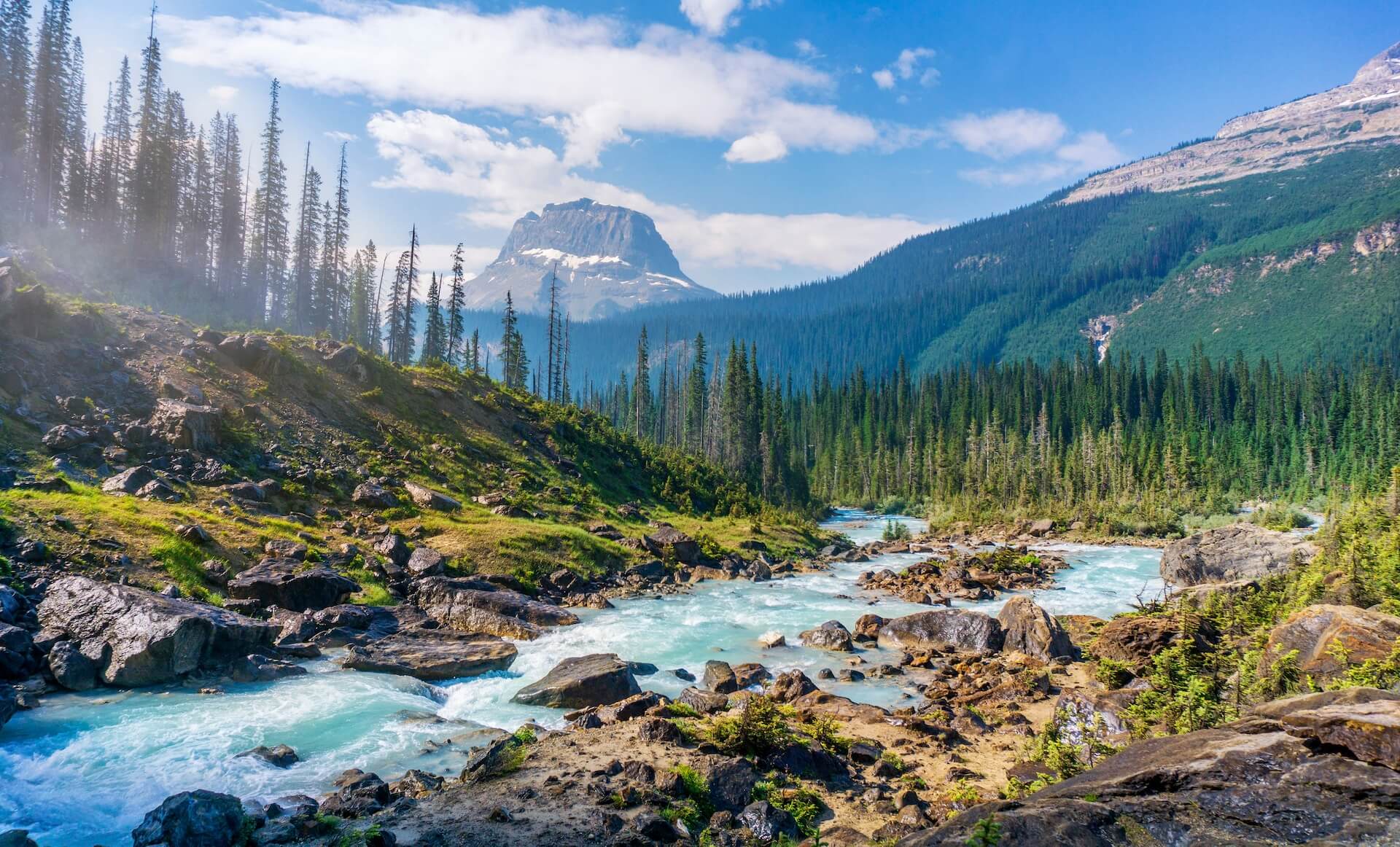
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function LoopCarouselExample(props) {
const { slides } = props;
return (
<div class="flex flex-col gap-y-6">
<Carousel slides={slides} loop />
<Carousel slides={slides} indicator="left" direction="vertical" loop />
</div>
);
}
Custom Slides
Default slot is for slides. Here is an example with our own image design.
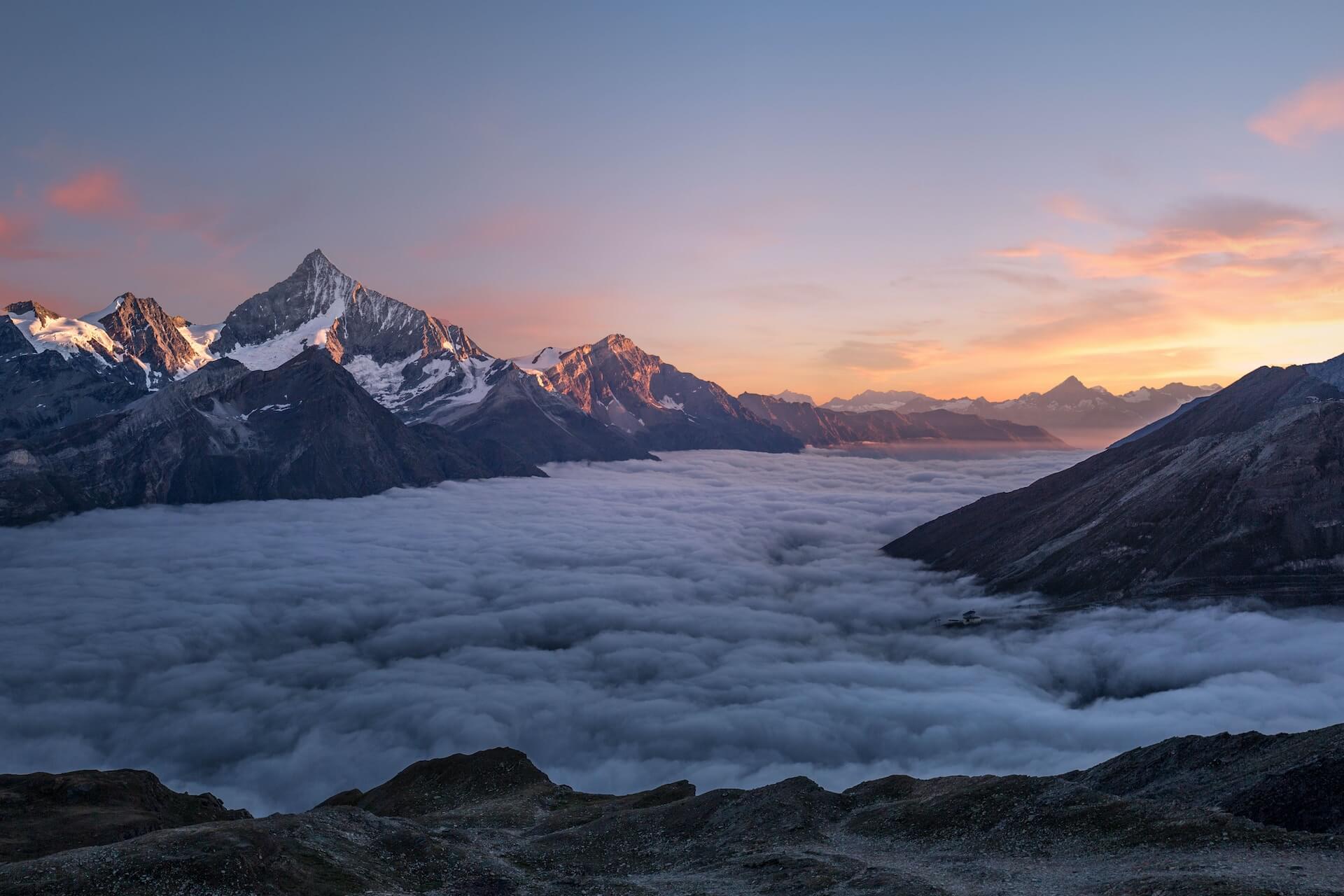
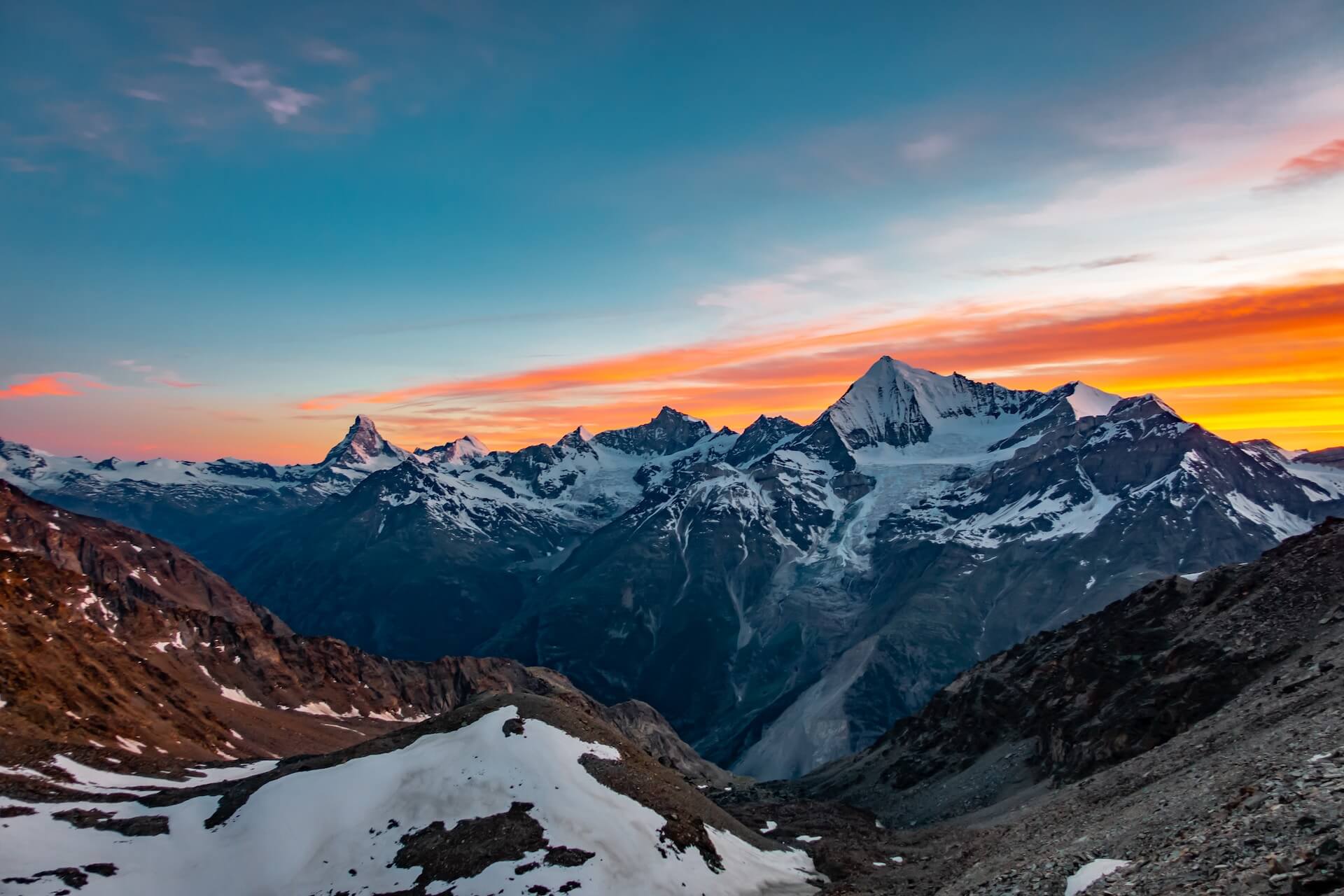
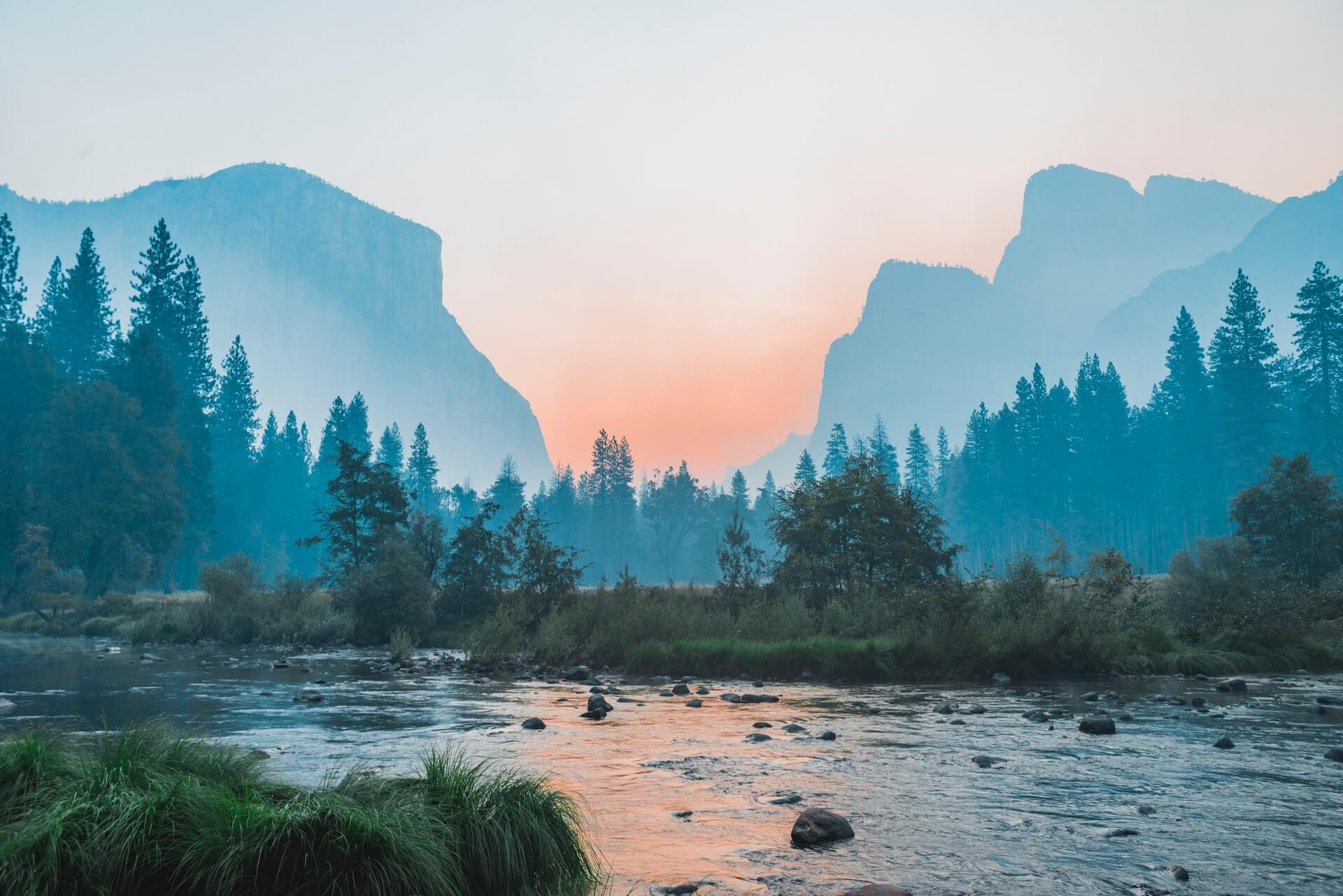
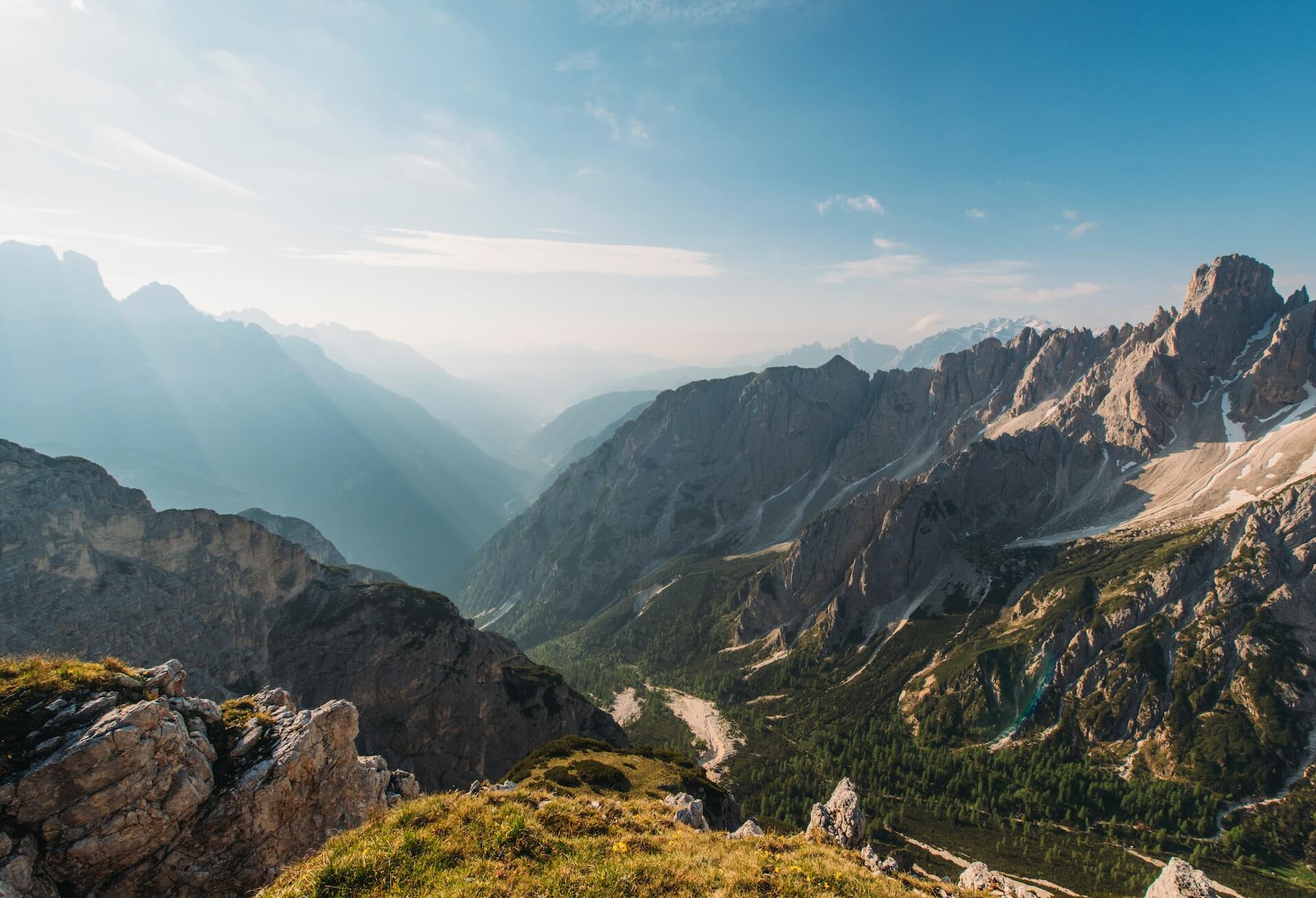
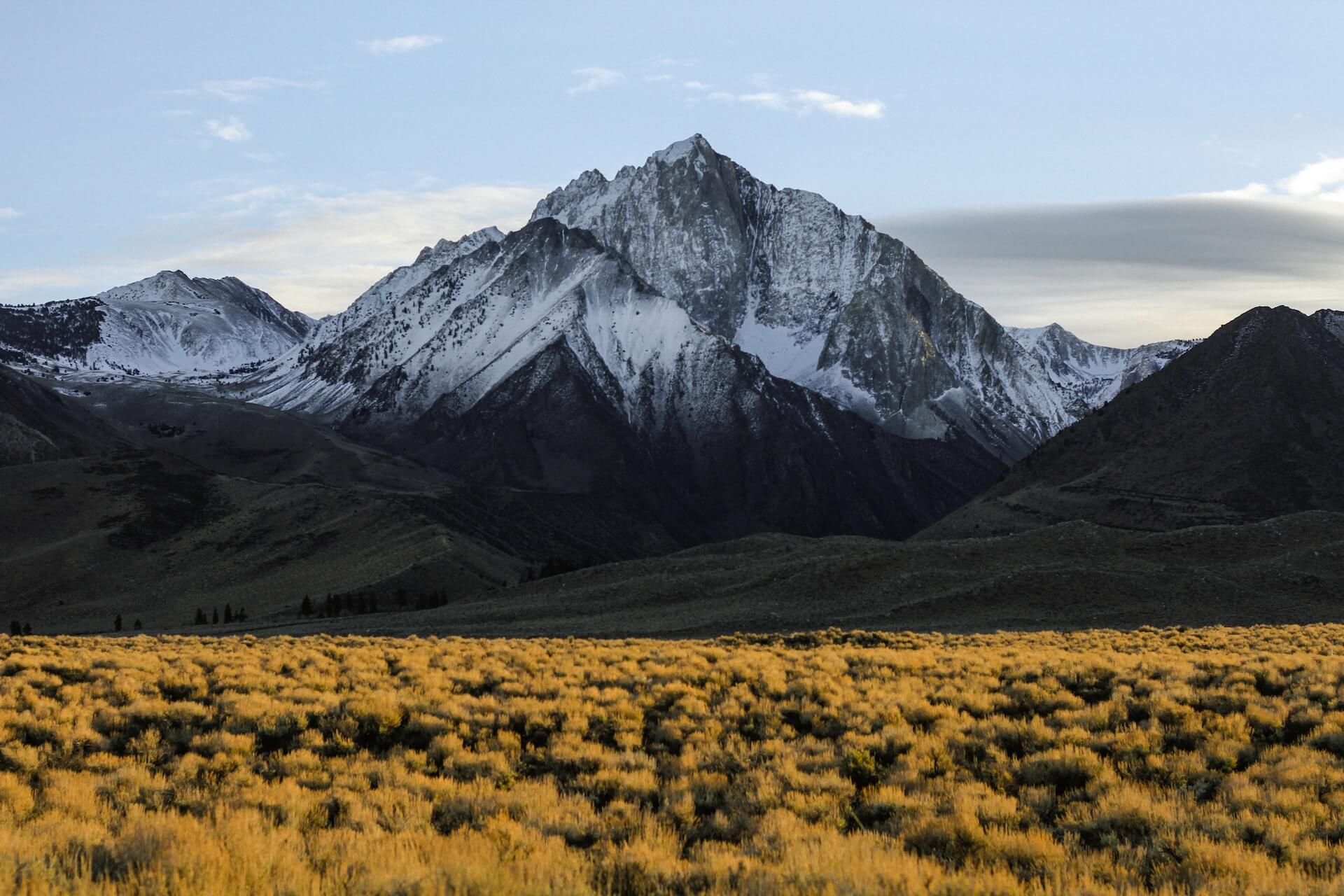
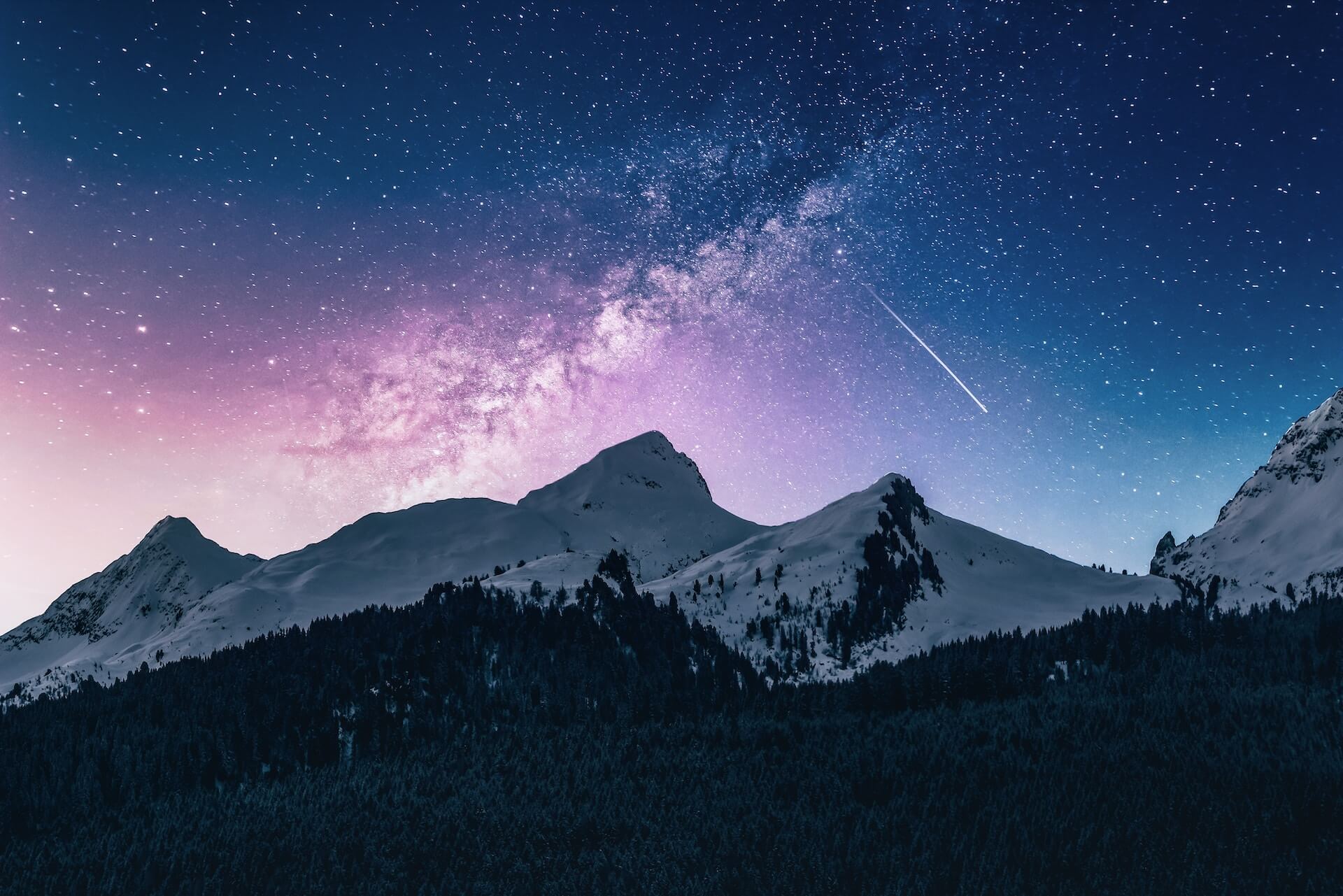
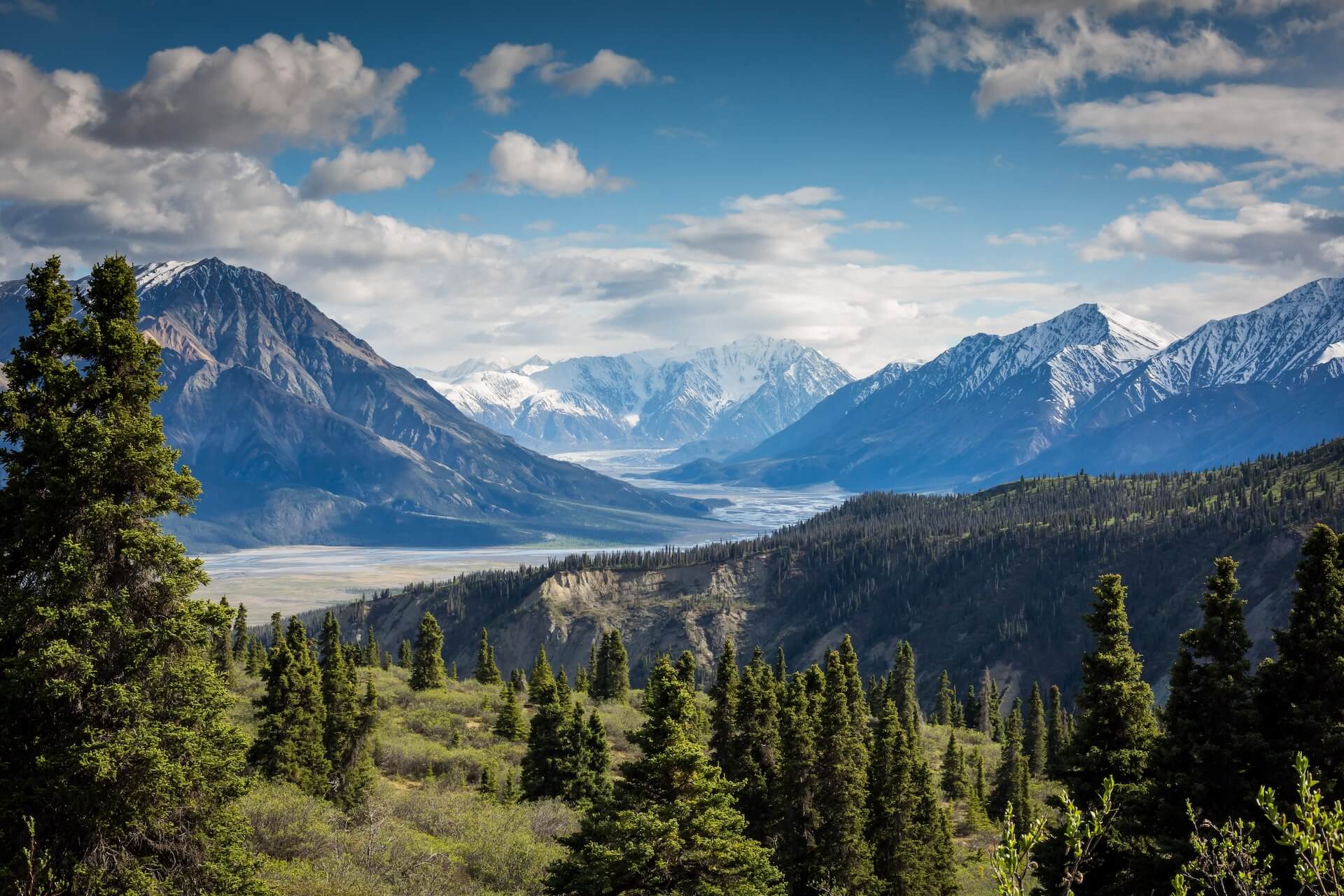
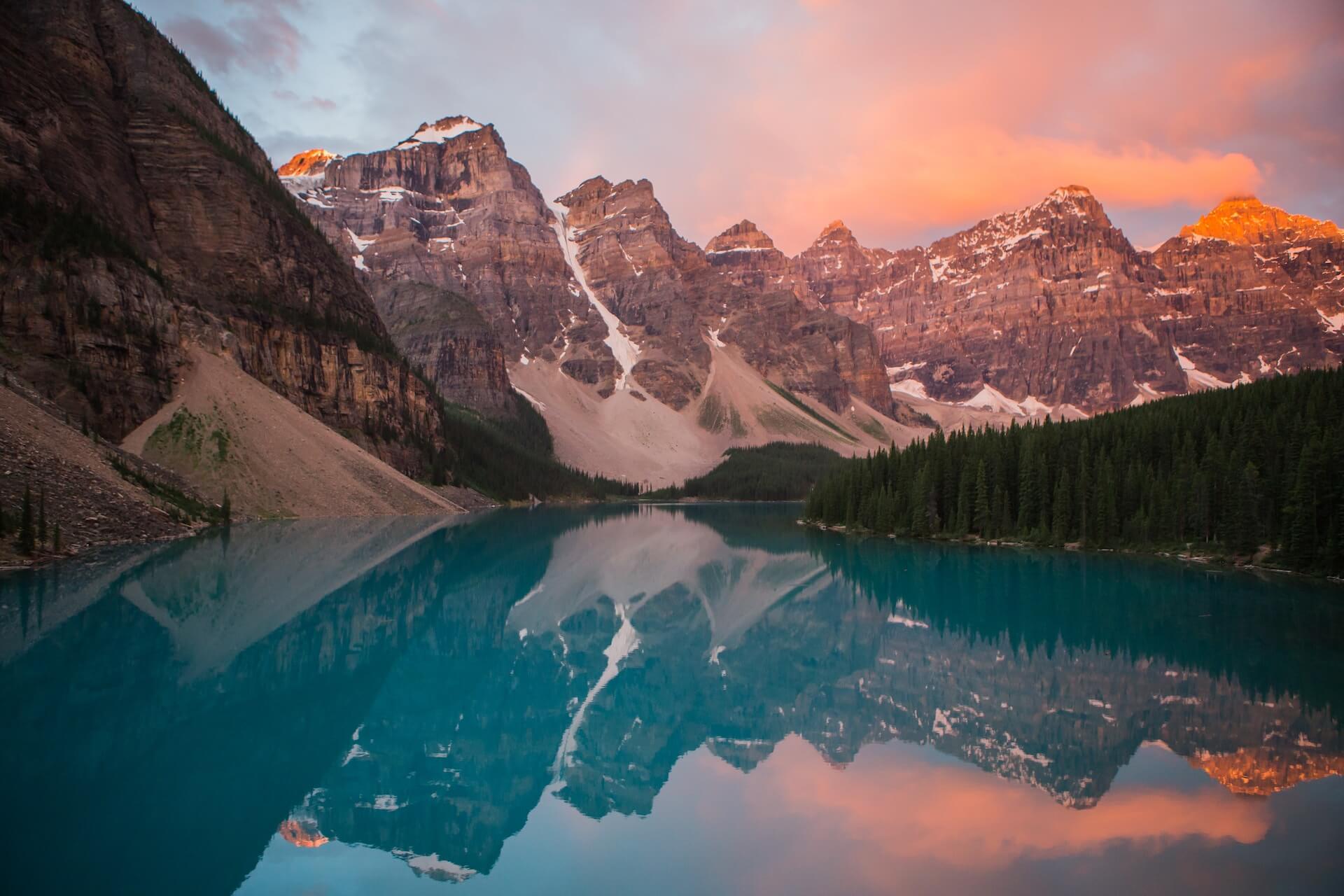
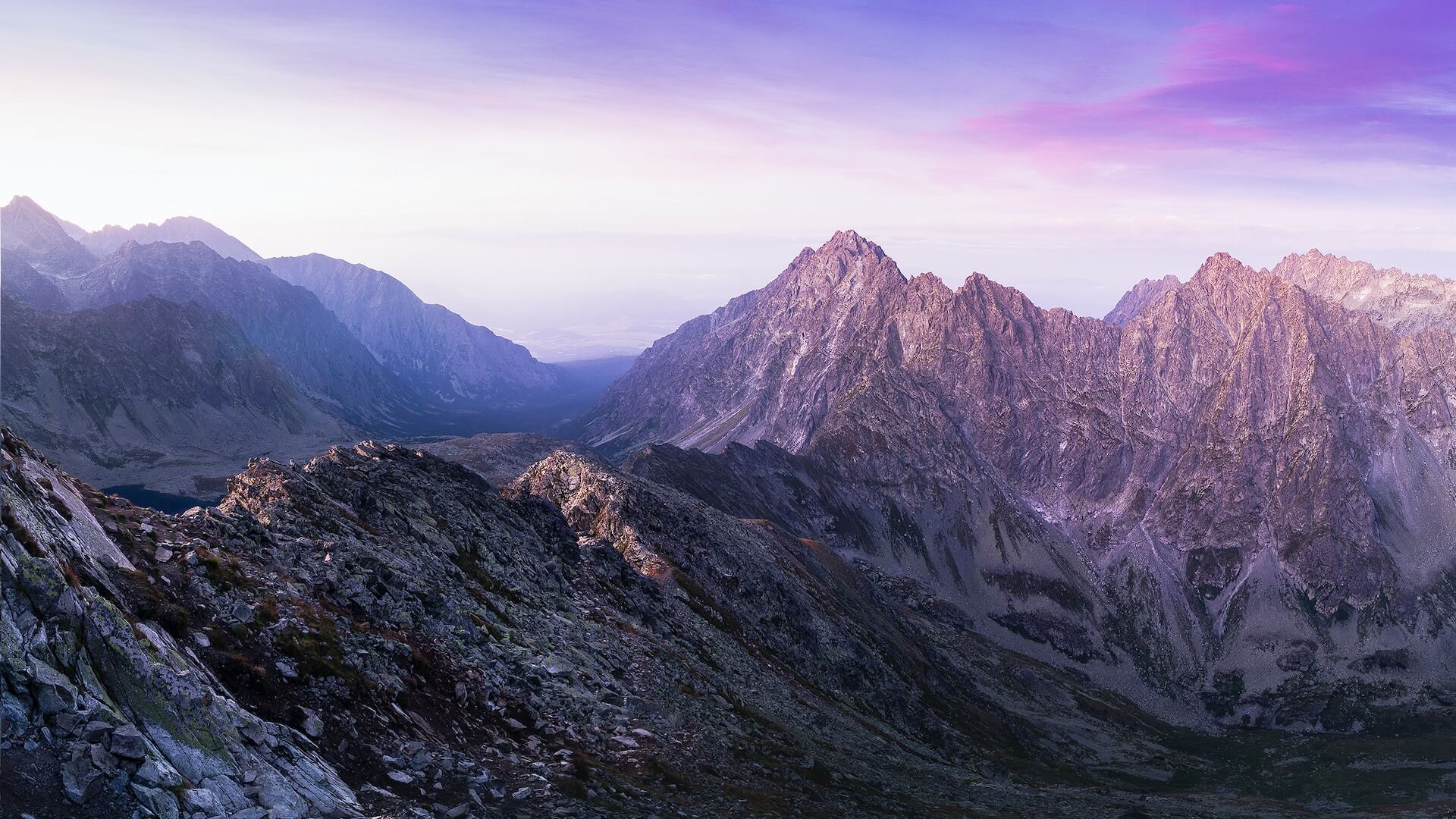
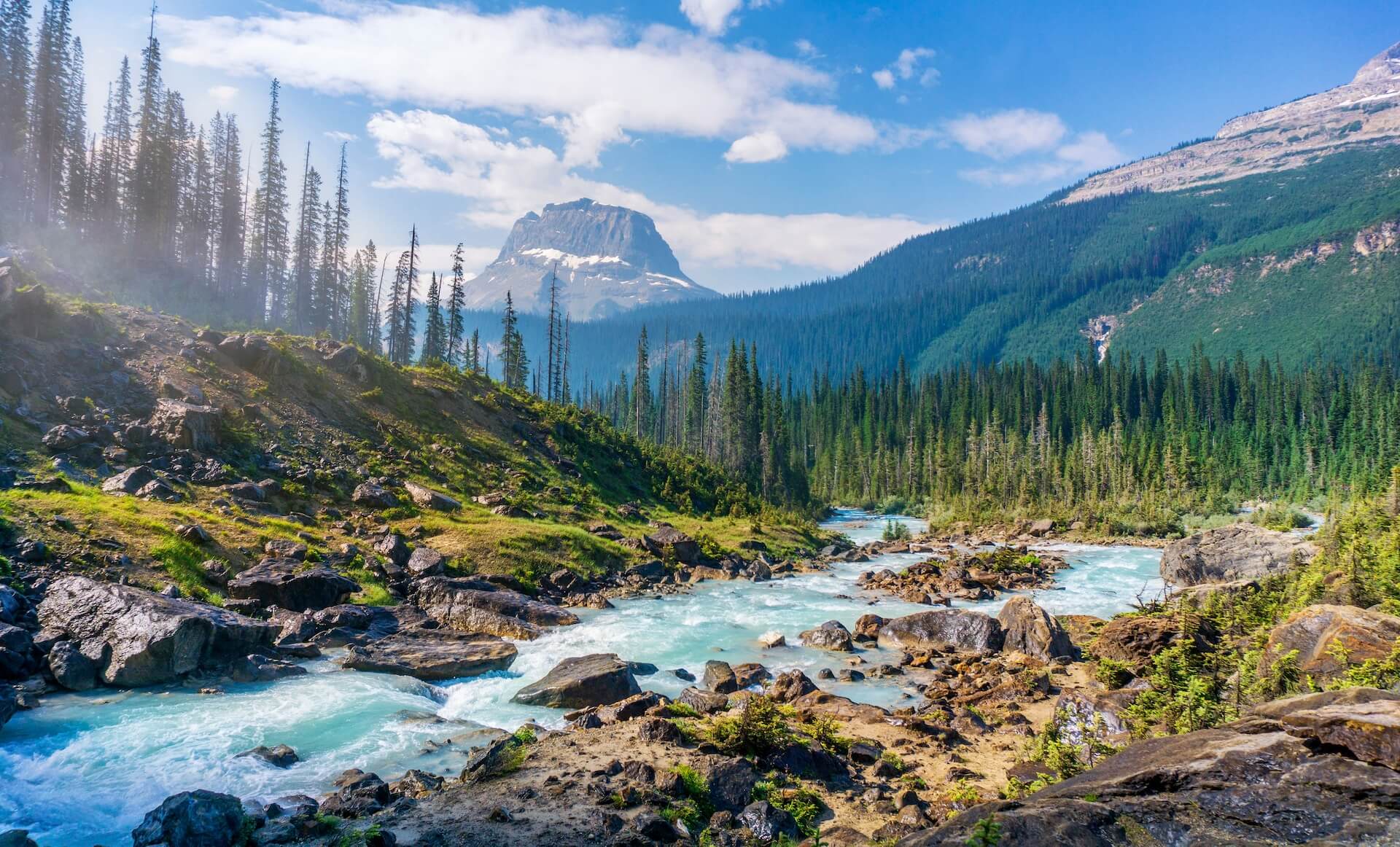
Copied !
import { Carousel } from "$components/carousel.component";
import { Image } from "$components/image.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function CustomSlidesImageCarouselExample(props) {
const { slides } = props;
return (
<Carousel loop>
{slides.map((image) => (
<Image src={image.src} alt={image.alt} />
))}
</Carousel>
);
}
However, images are not the only slides you can put in carousel. Be aware that it's up to you to properly define your css class to have expected layout.
This is an example with just some section tag and dummy content inside as slides.
This is Slide 1
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Doloremque voluptatem aliquid nam, quaerat eligendi quasi iste sed, placeat consequuntur enim ad quis tenetur praesentium impedit! Suscipit cumque natus ipsam earum.
This is Slide 2
Lorem ipsum dolor sit amet consectetur adipisicing elit. Debitis, perspiciatis.
This is Slide 3
Lorem ipsum dolor sit amet consectetur adipisicing elit. Nostrum aliquid vel eligendi minus nesciunt rerum illo beatae eos dolor. Esse voluptatem excepturi eligendi ab rem, nam possimus sed sequi quaerat corrupti consequuntur molestias sint rerum repudiandae explicabo est necessitatibus non autem vitae hic illo officiis obcaecati asperiores maxime. Facere, eum?
This is Slide 4
Lorem ipsum dolor sit amet consectetur adipisicing elit. Natus dolor dicta cumque nemo. Architecto quam odit obcaecati laboriosam sapiente inventore, dolorem iusto? Numquam velit sed minima culpa magni sapiente. Distinctio quas voluptatem sed dignissimos cumque heading sequi ratione consequatur at, dicta iste repellat non! Quam quod totam aperiam magni repellat dolorum dolore mollitia illo, fugit qui possimus voluptatibus! Maiores eveniet consectetur voluptates nostrum magni doloribus in, ea autem itaque nam maxime minus necessitatibus illum ad?
This is Slide 5
Lorem ipsum dolor sit, amet consectetur adipisicing elit. Veritatis magni, aperiam repellendus nisi itaque facere! Consequatur beatae voluptas reiciendis minus quidem inventore ea heading harum dicta obcaecati aut nam sunt ipsum odit dolore doloribus iure nemo quasi, officia asperiores. Vero?
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: {color: string, text: string }[] }>}
*/
export function CustomSlidesCarouselExample(props) {
const { slides } = props;
return (
<Carousel hideNavigations indicator>
{slides.map((item, index) => (
<div
class={`min-w-full h-full flex flex-col gap-y-2 items-center p-2 md:p-6 ${item.color}`}
>
<h3>This is Slide {index + 1}</h3>
<p class="text-sm flex items-start" safe>{item.text}</p>
</div>
))}
</Carousel>
);
}
Custom Indicators
Indicators can also be customize. Just set an html tag with slot name as indicators.
As reminder, you'll have to deal with indicators position based on direction yourself. Below an example with indicators as numbers.
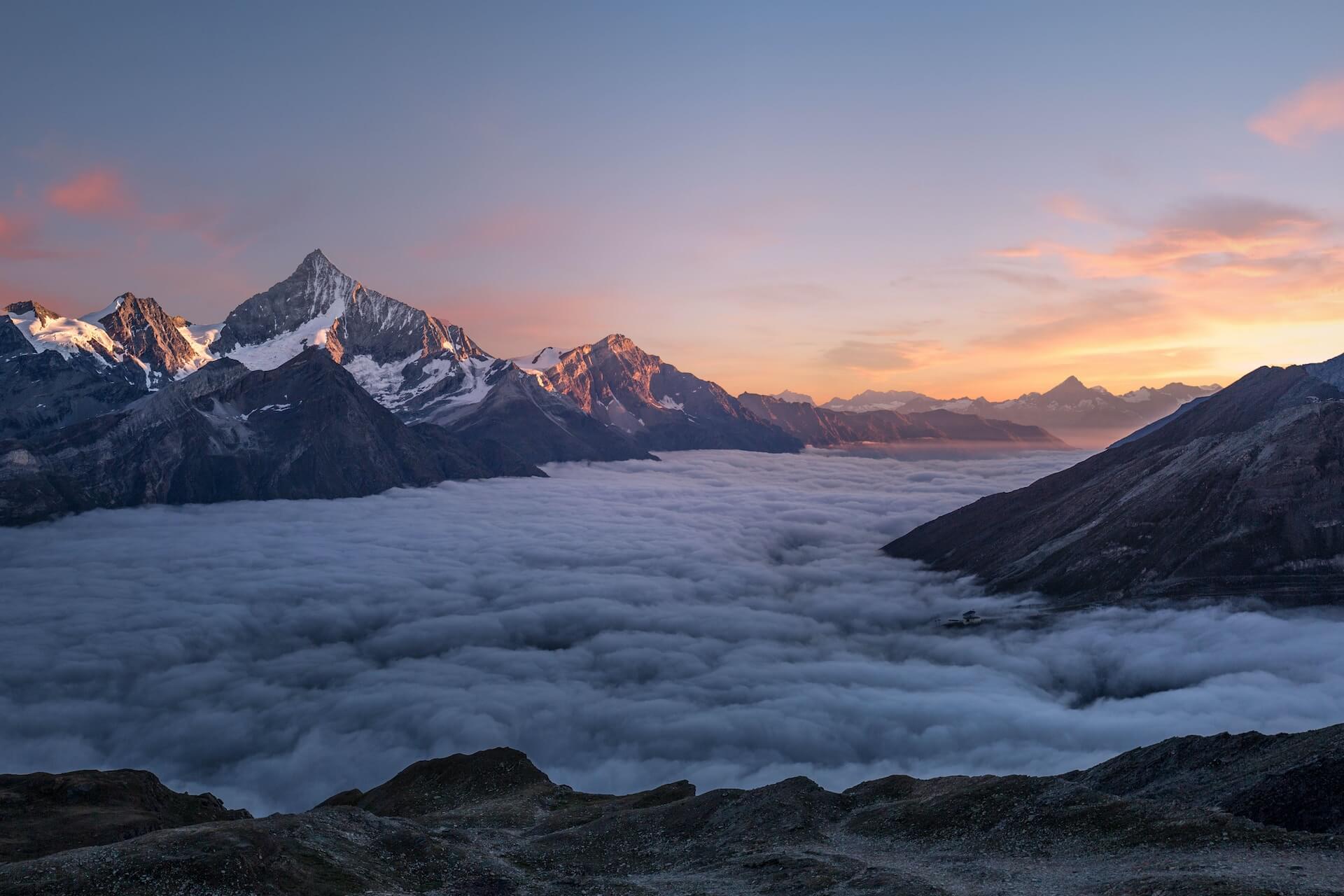
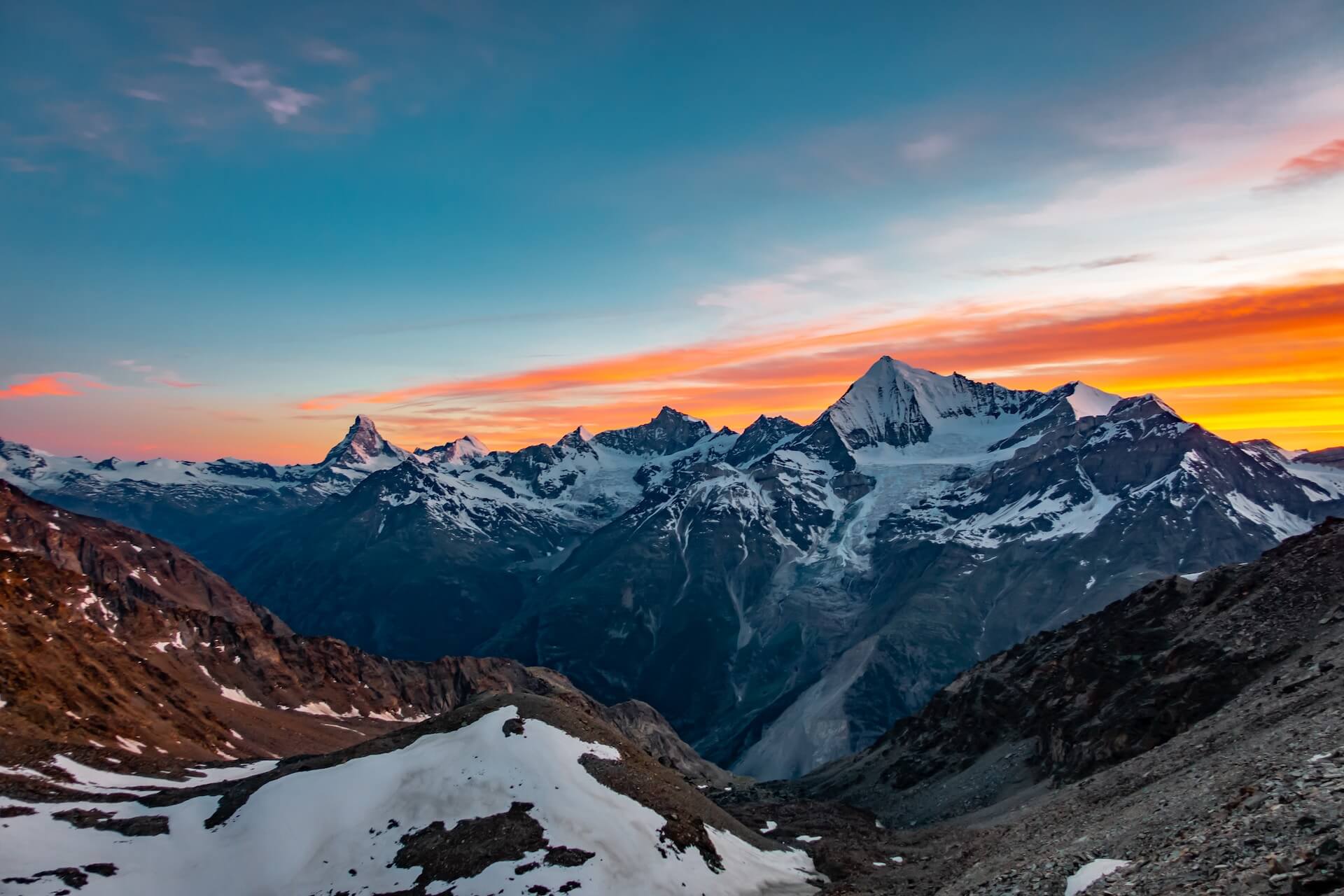
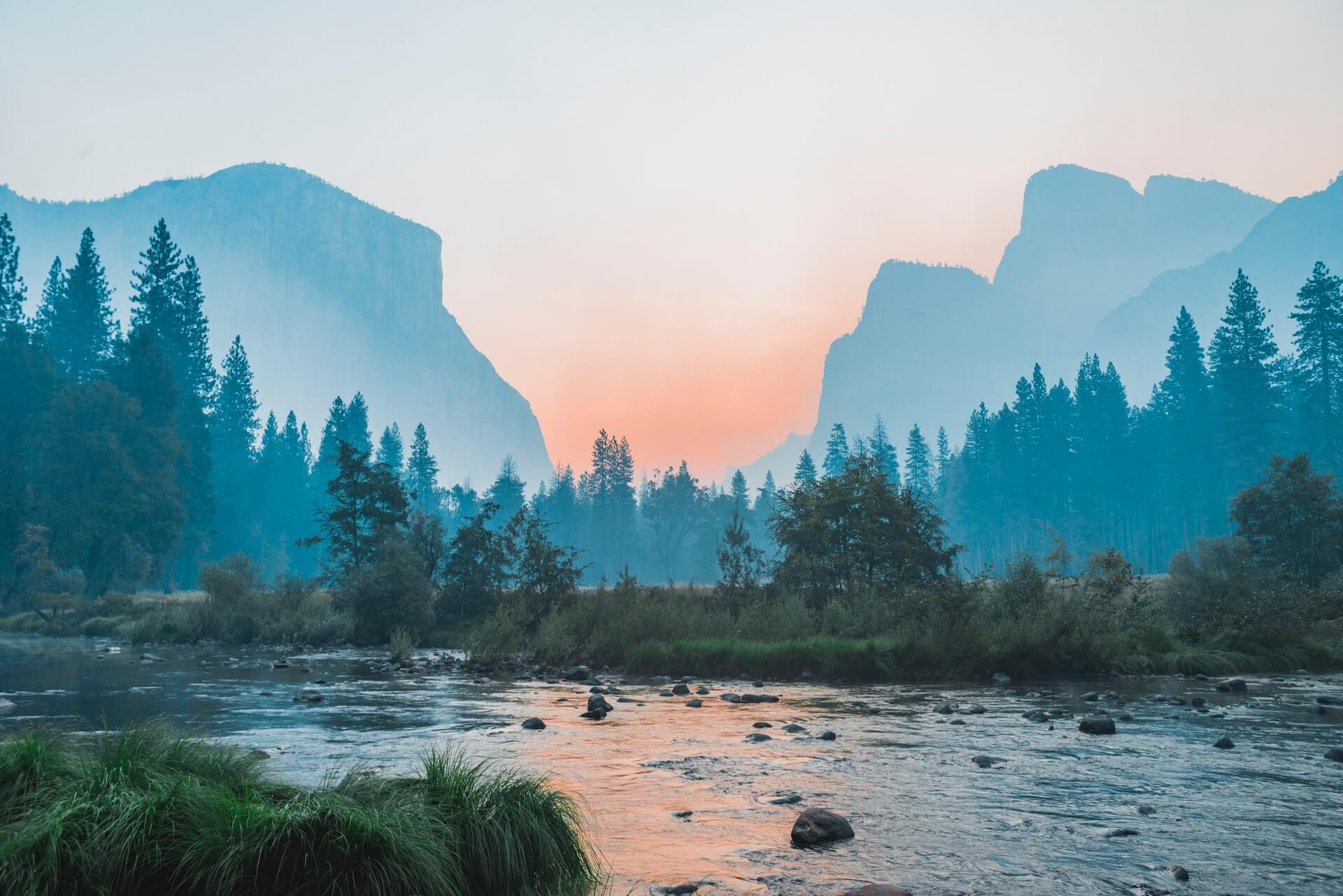
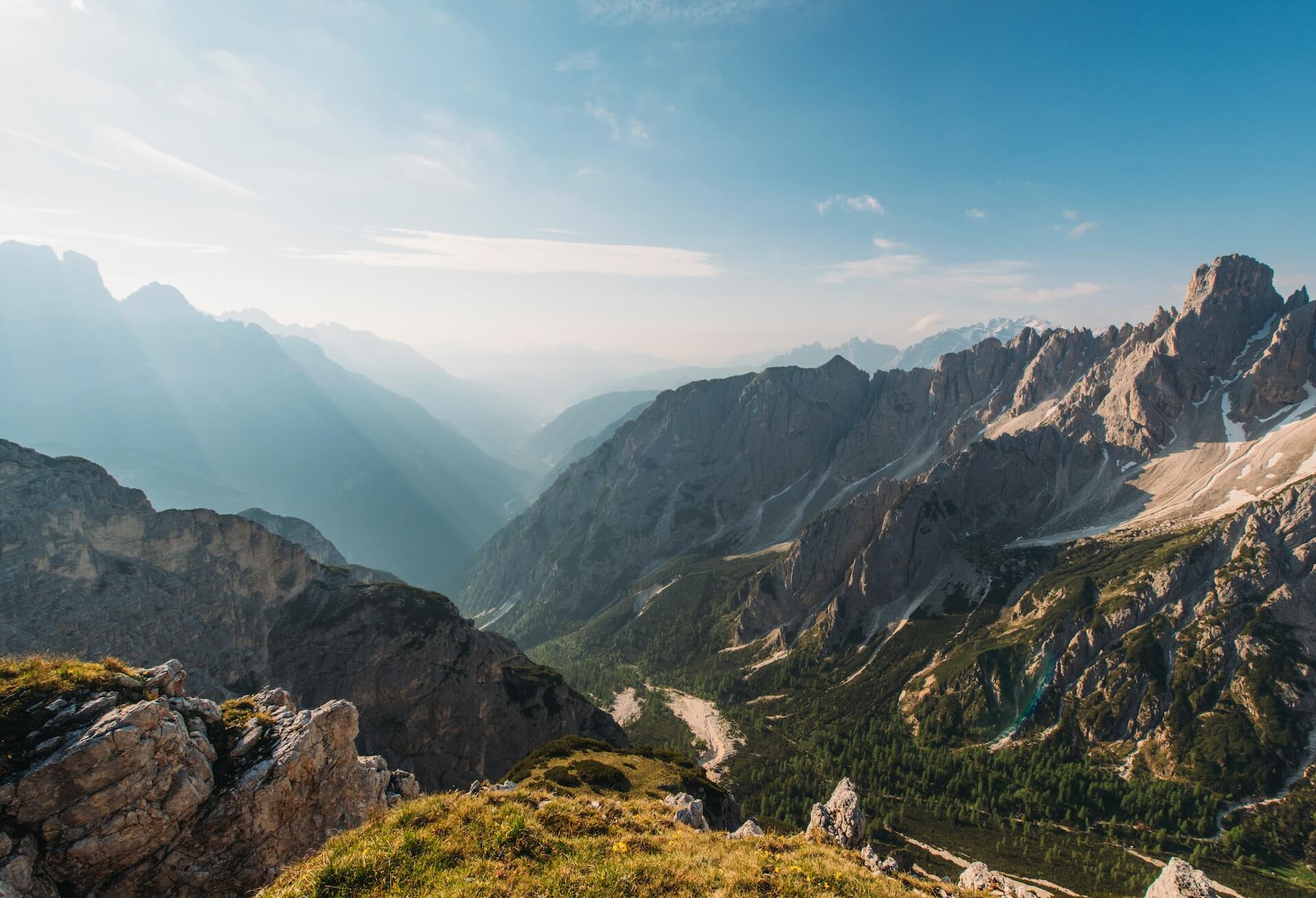
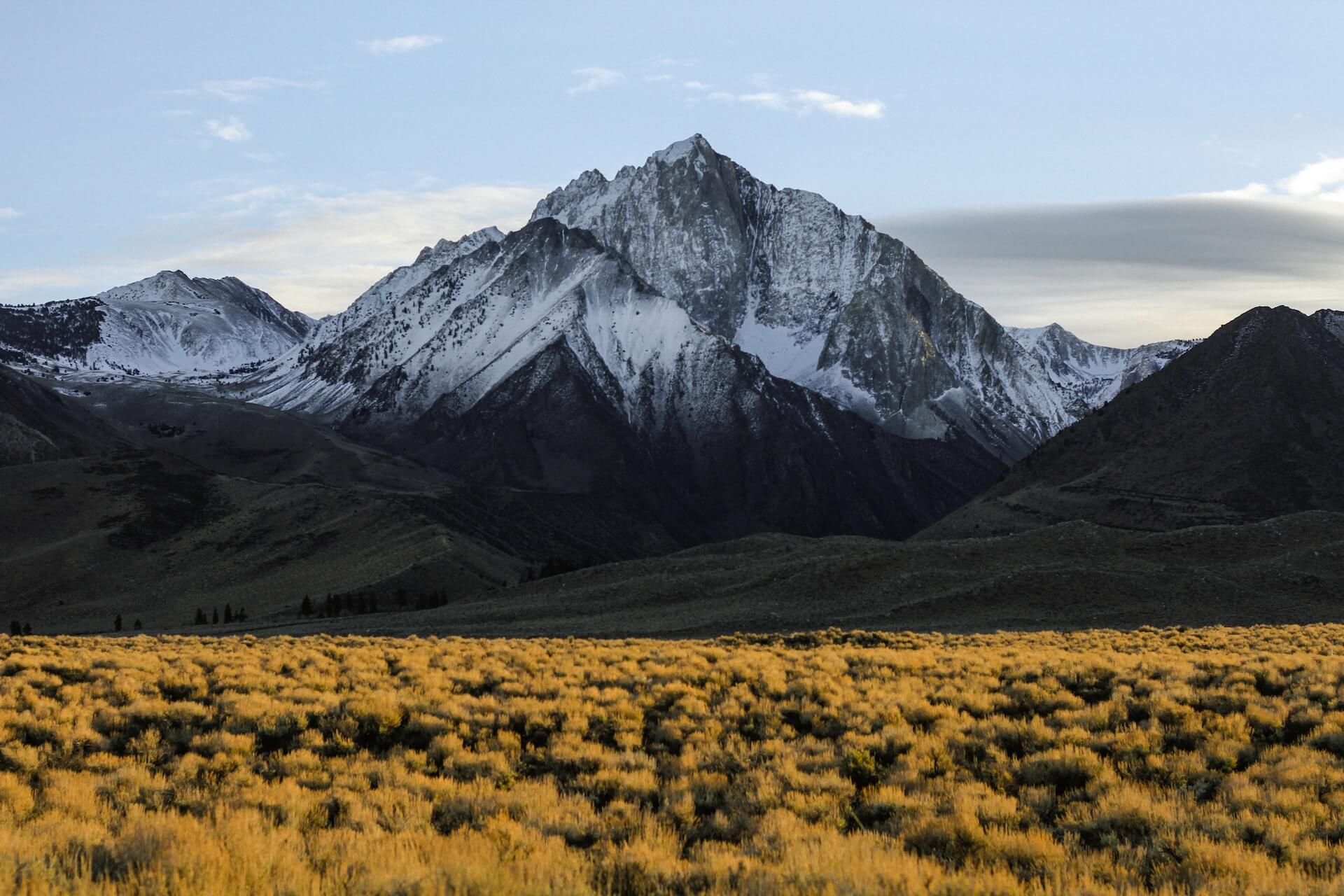
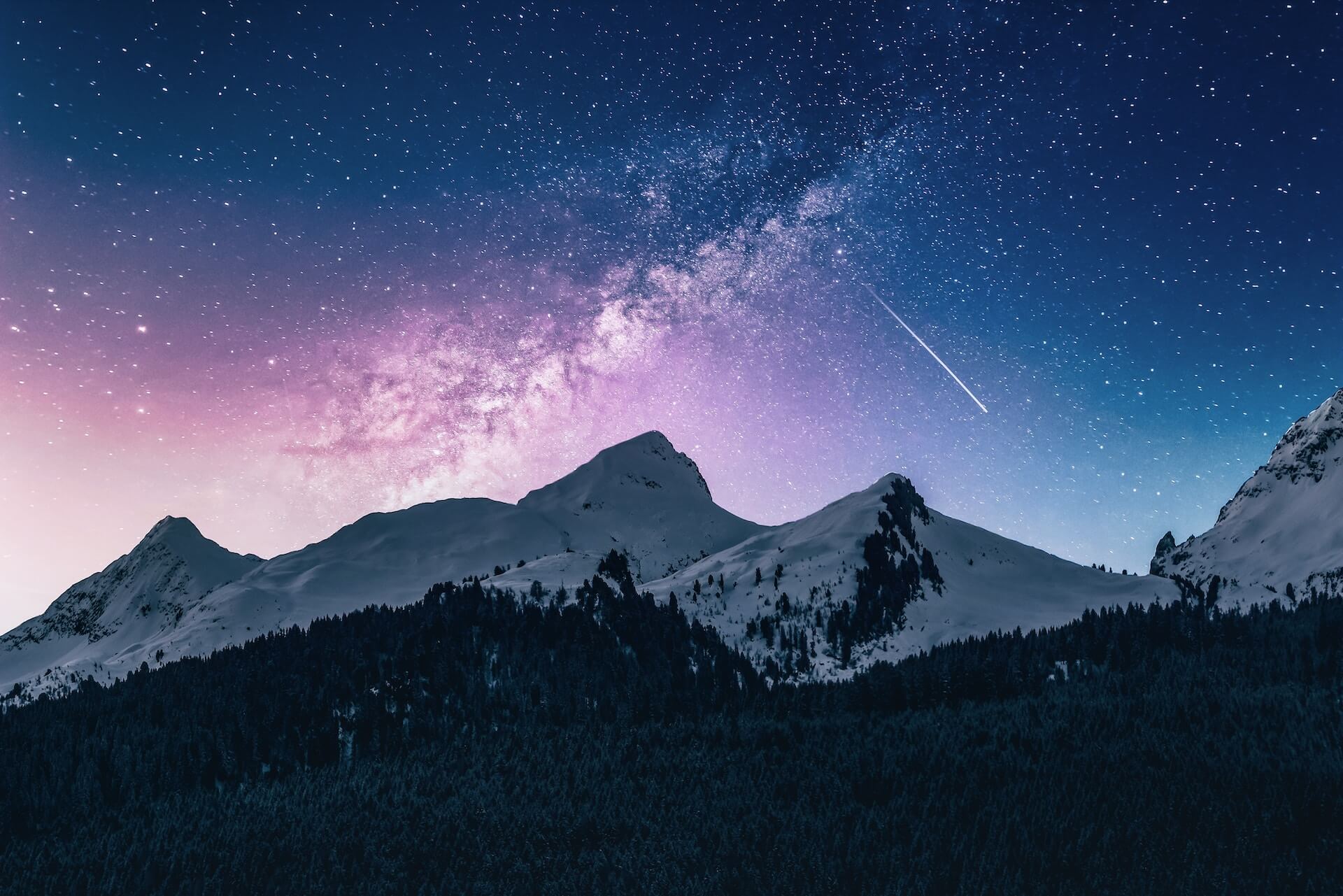
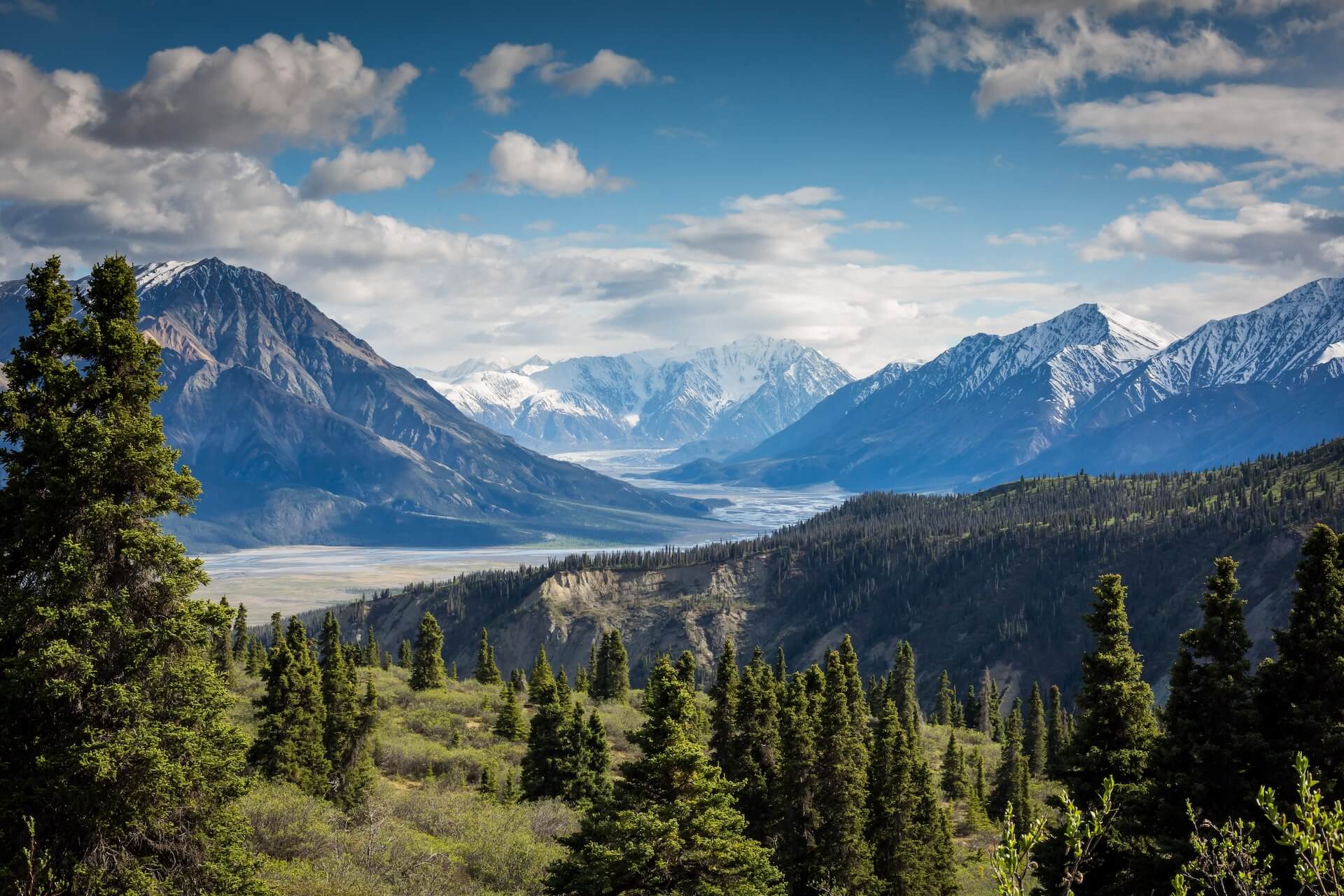
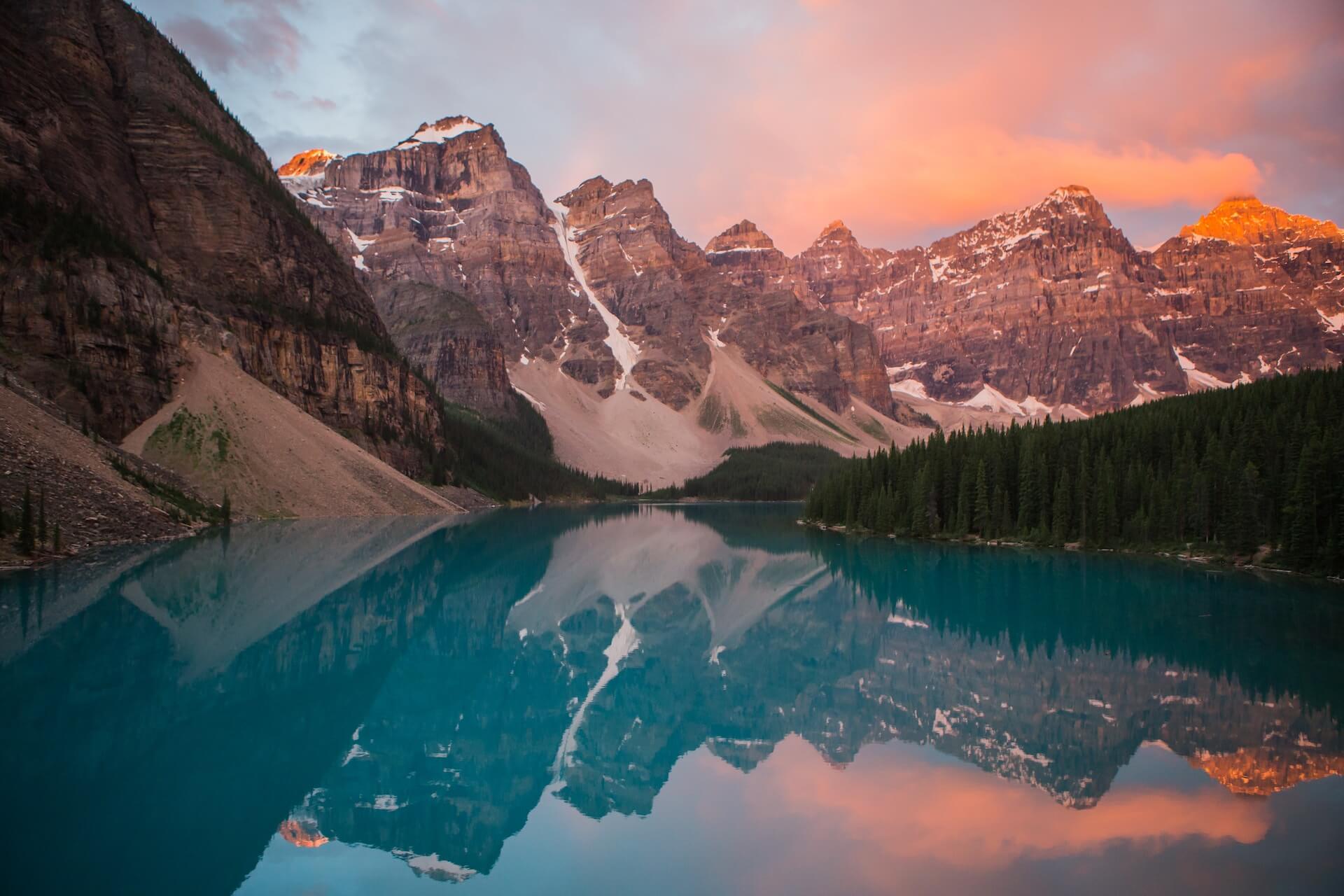
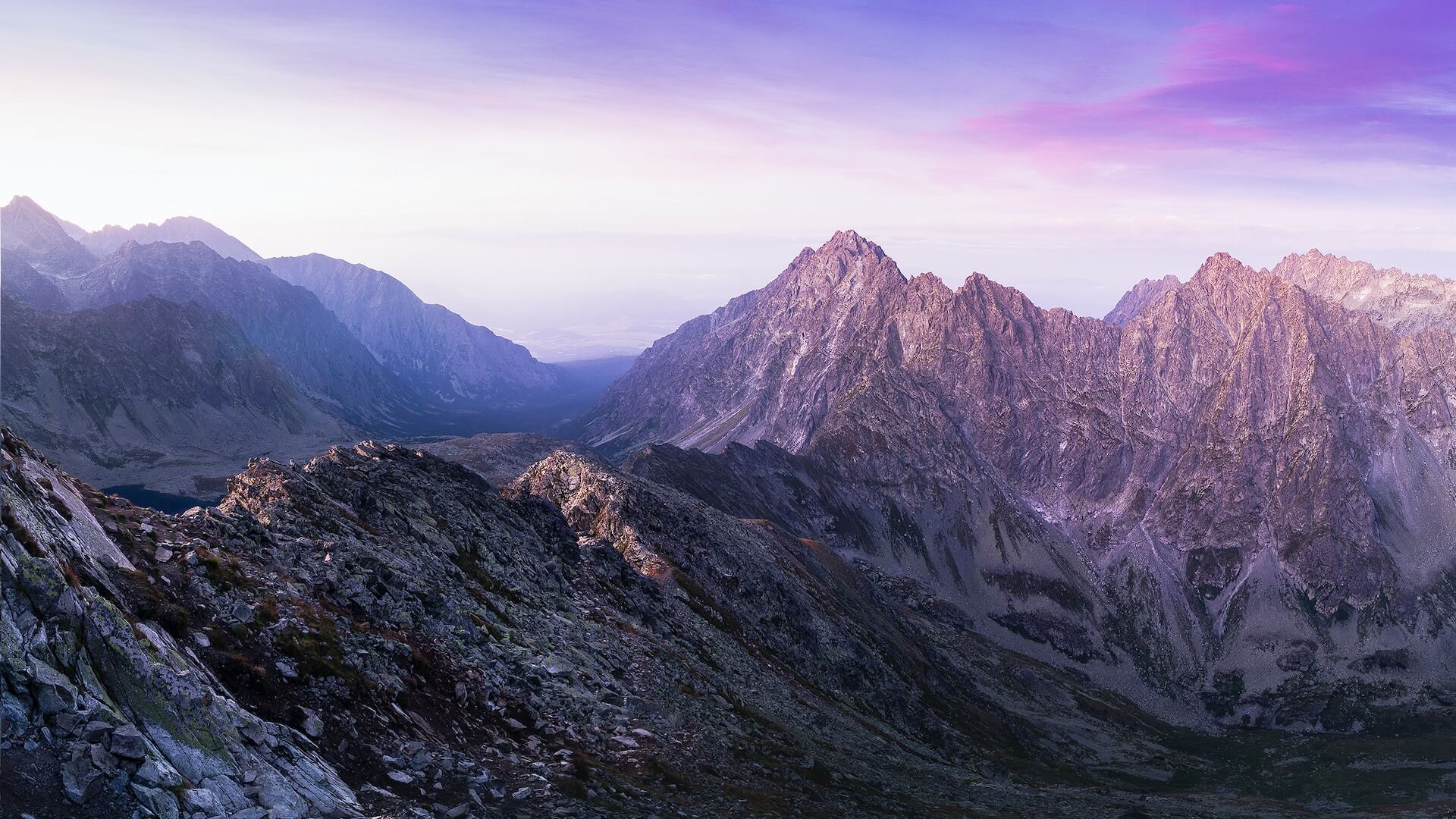
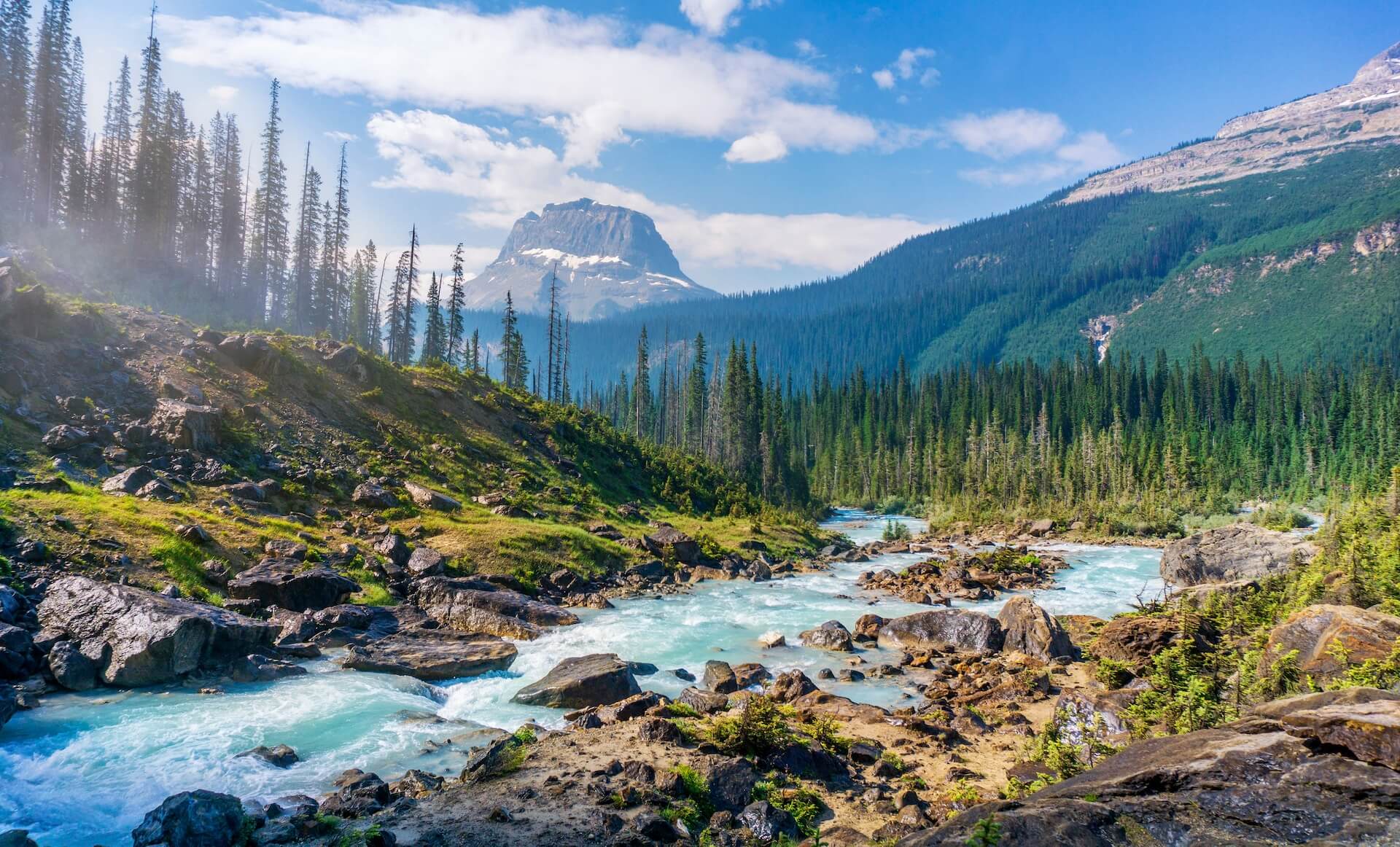
Copied !
import { Carousel, CarouselIndicators } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function DefaultCarouselExample(props) {
const { slides } = props;
return (
<Carousel
slides={slides}
indicators={
<CarouselIndicators>
{slides.map((image, index) => (
<li>
<button
x-on:click={`setActiveIndex(Number(${index}))`}
x-bind:disabled={`isActive(Number(${index}))`}
class="cursor-pointer p-2 bg-white text-slate-700 disabled:bg-slate-900 disabled:text-white disabled:cursor-not-allowed"
>
<span>{index + 1}</span>
</button>
</li>
))}
</CarouselIndicators>
}
loop
indicator
/>
);
}
Multiple Slides
A great feature of carousel is to show multiple slides.
Just set a number to slidesToShow props as shown in examples below.
PS: For custom slides, it's up to you to manage slide sizes.
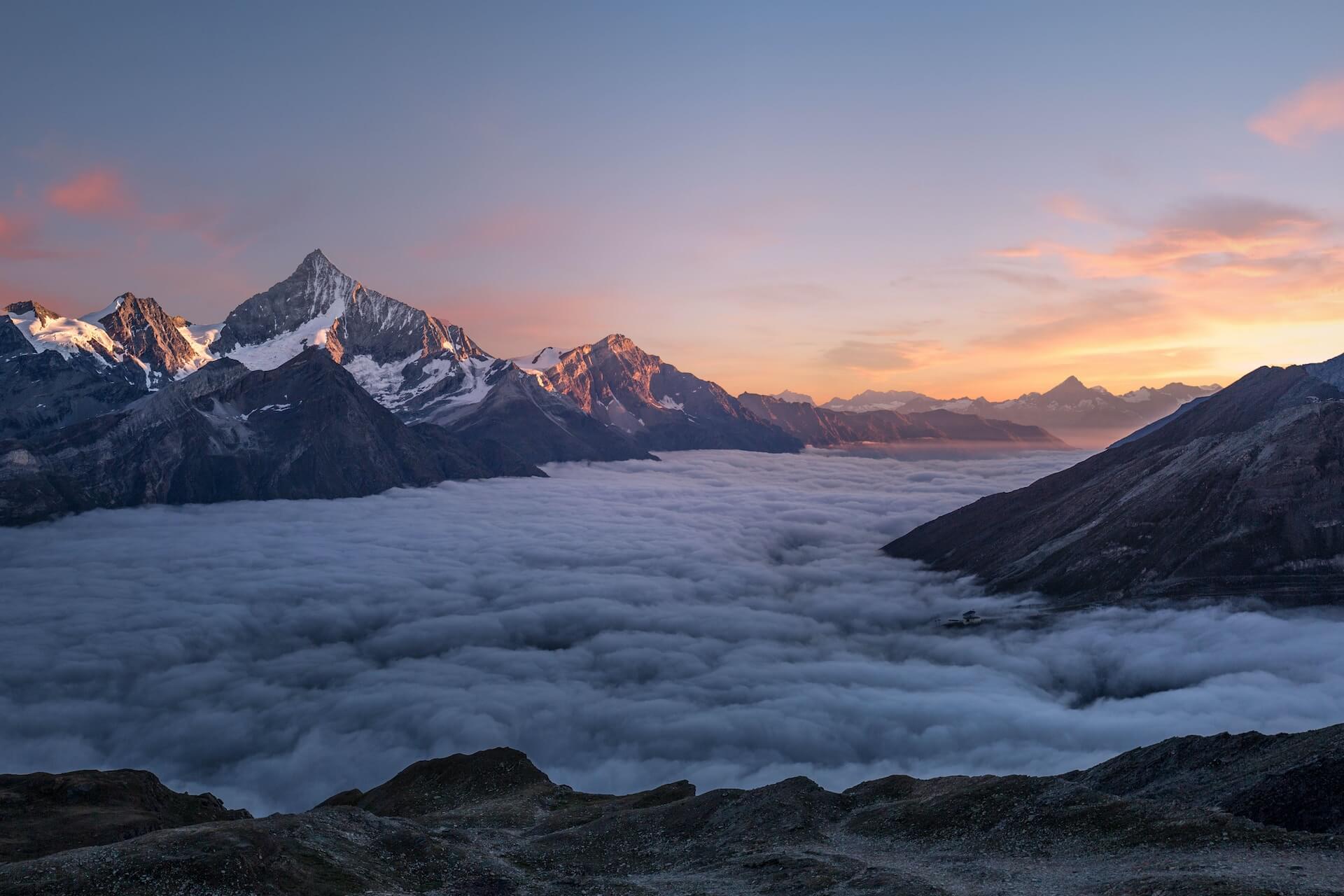
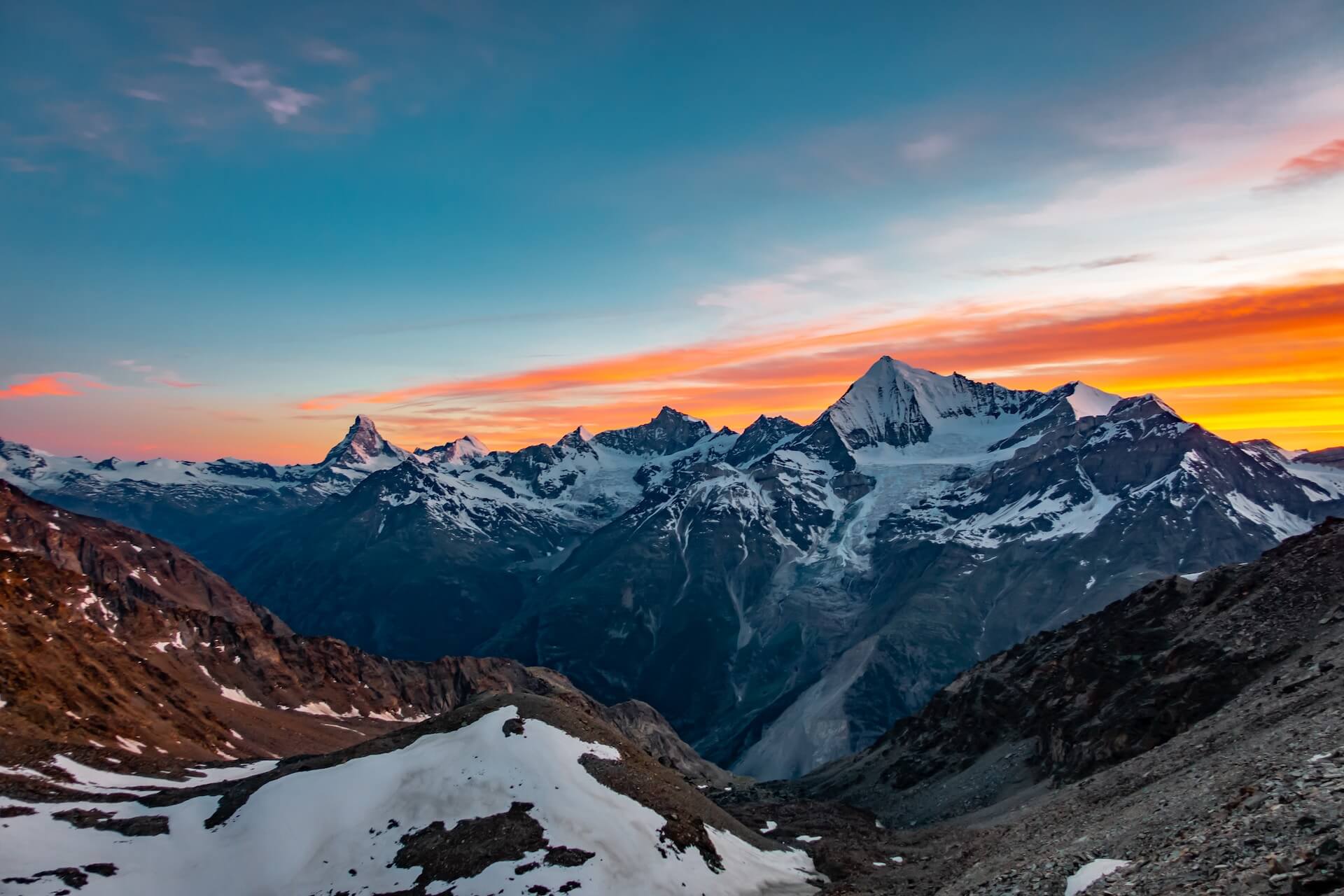
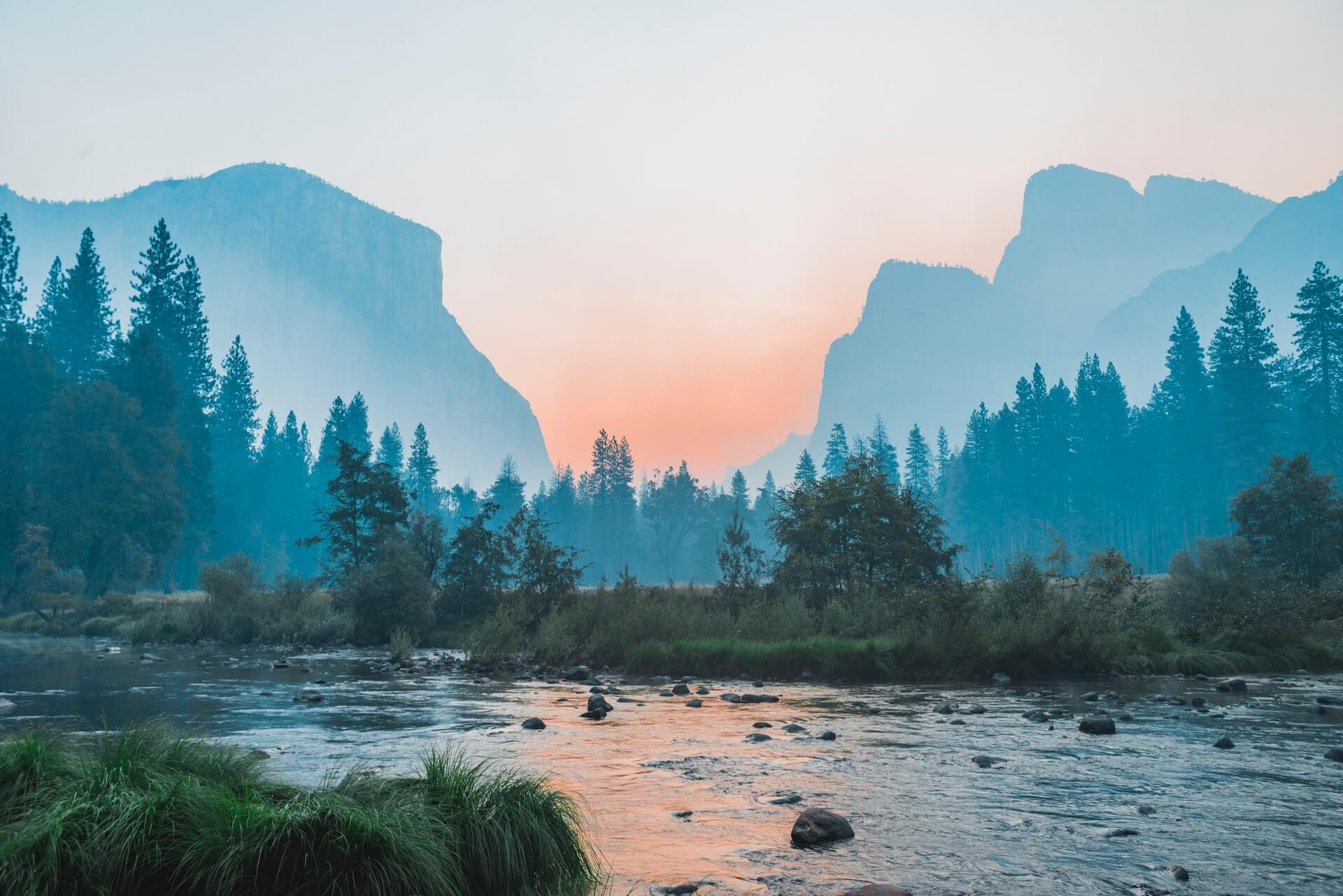
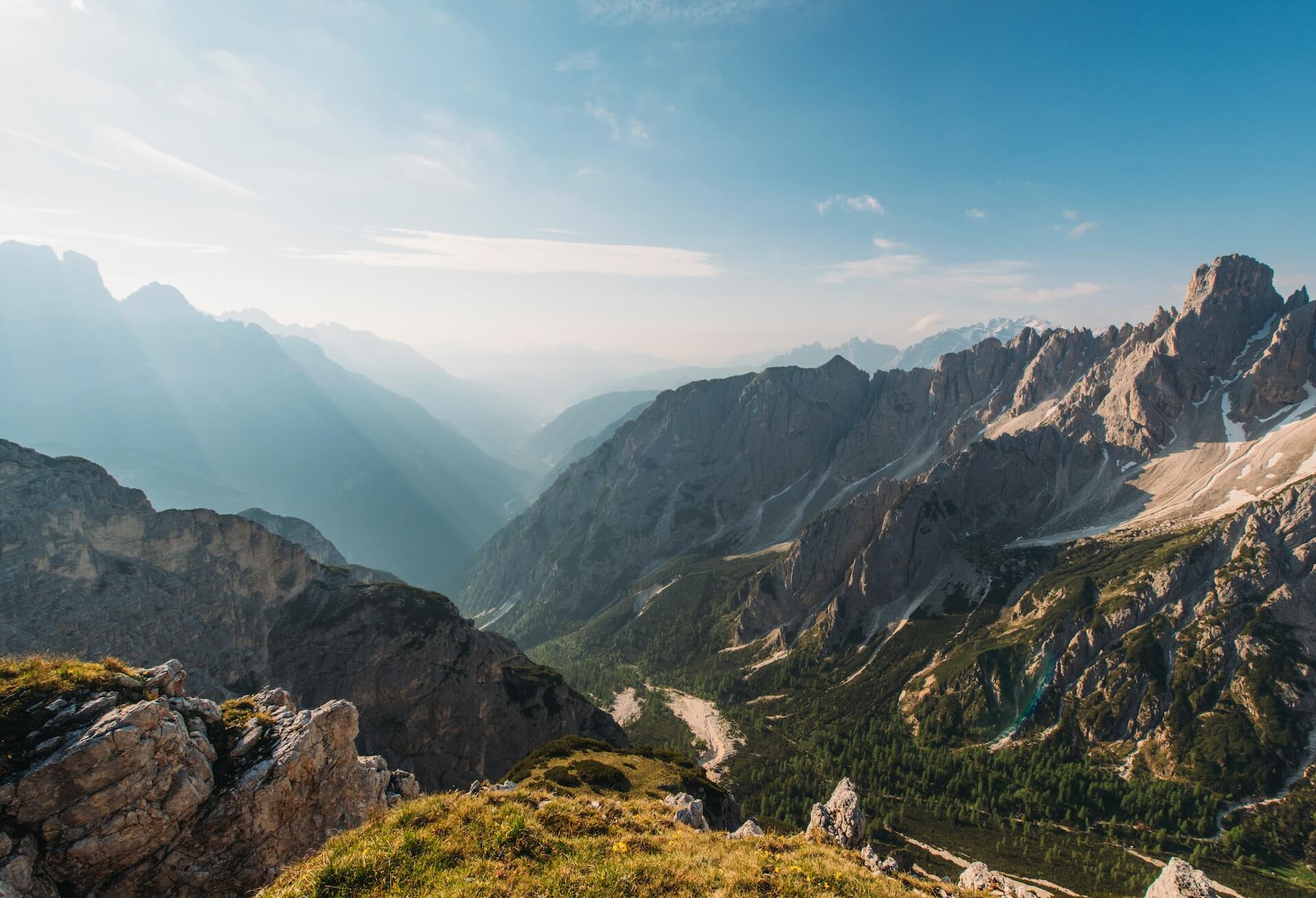
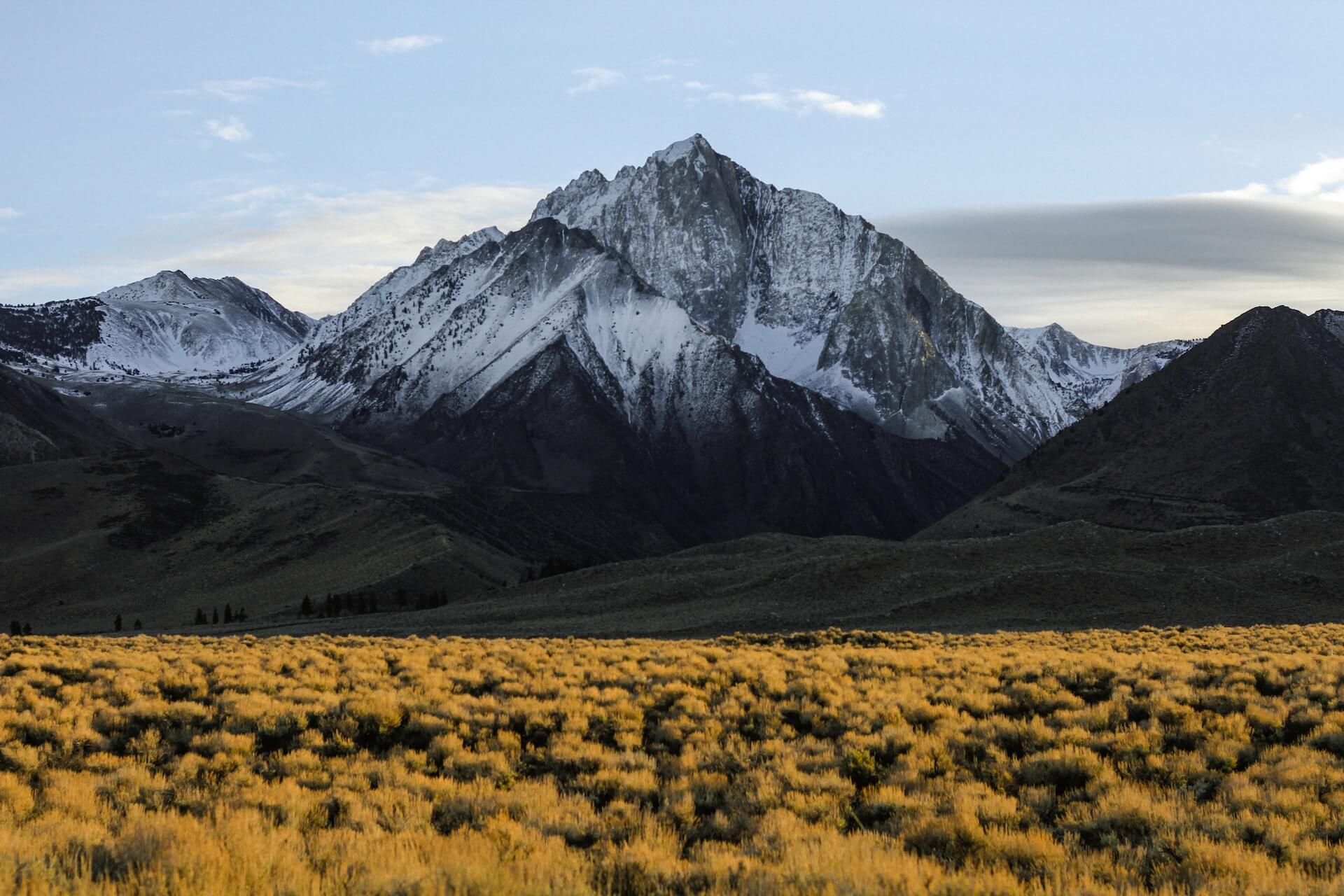
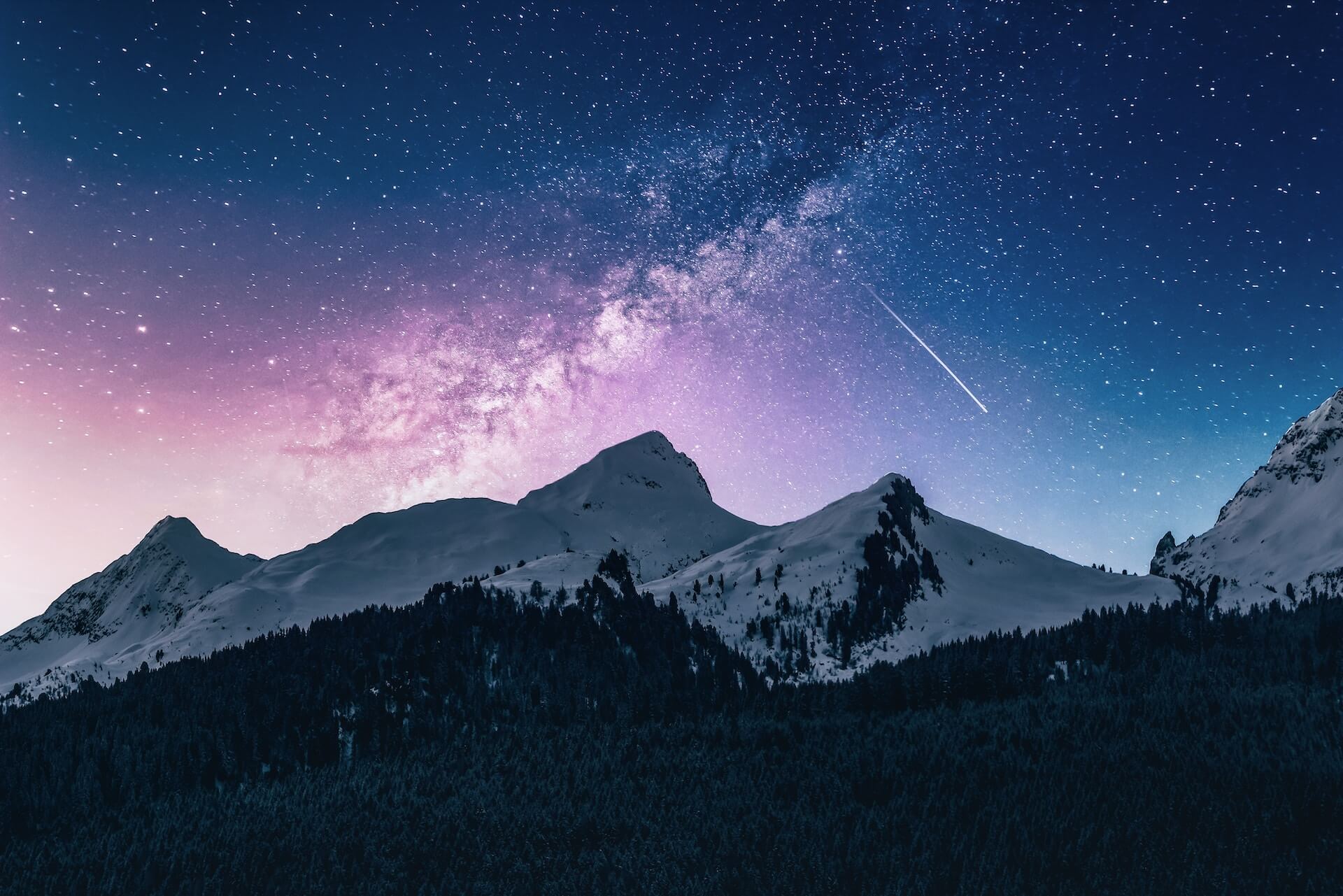
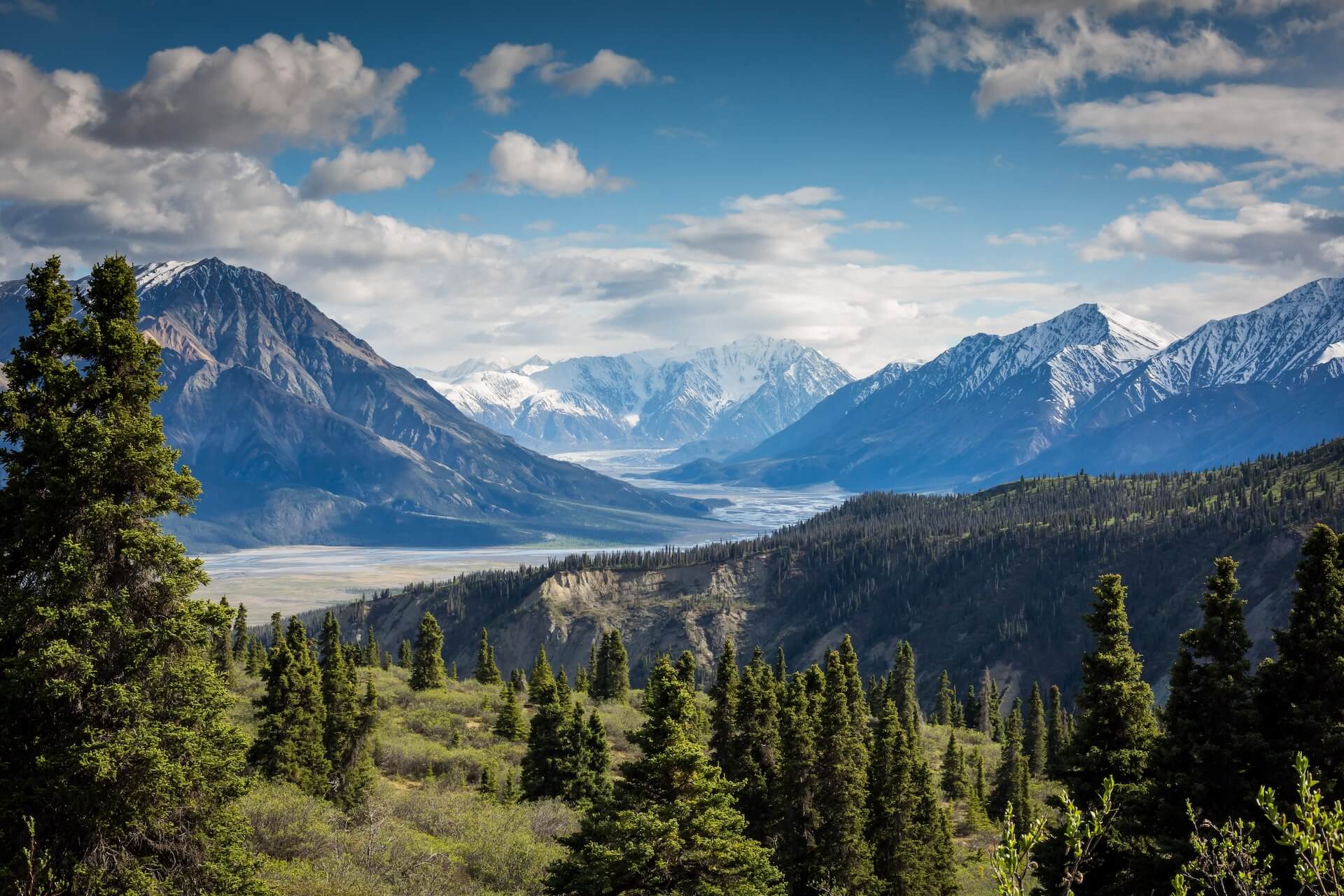
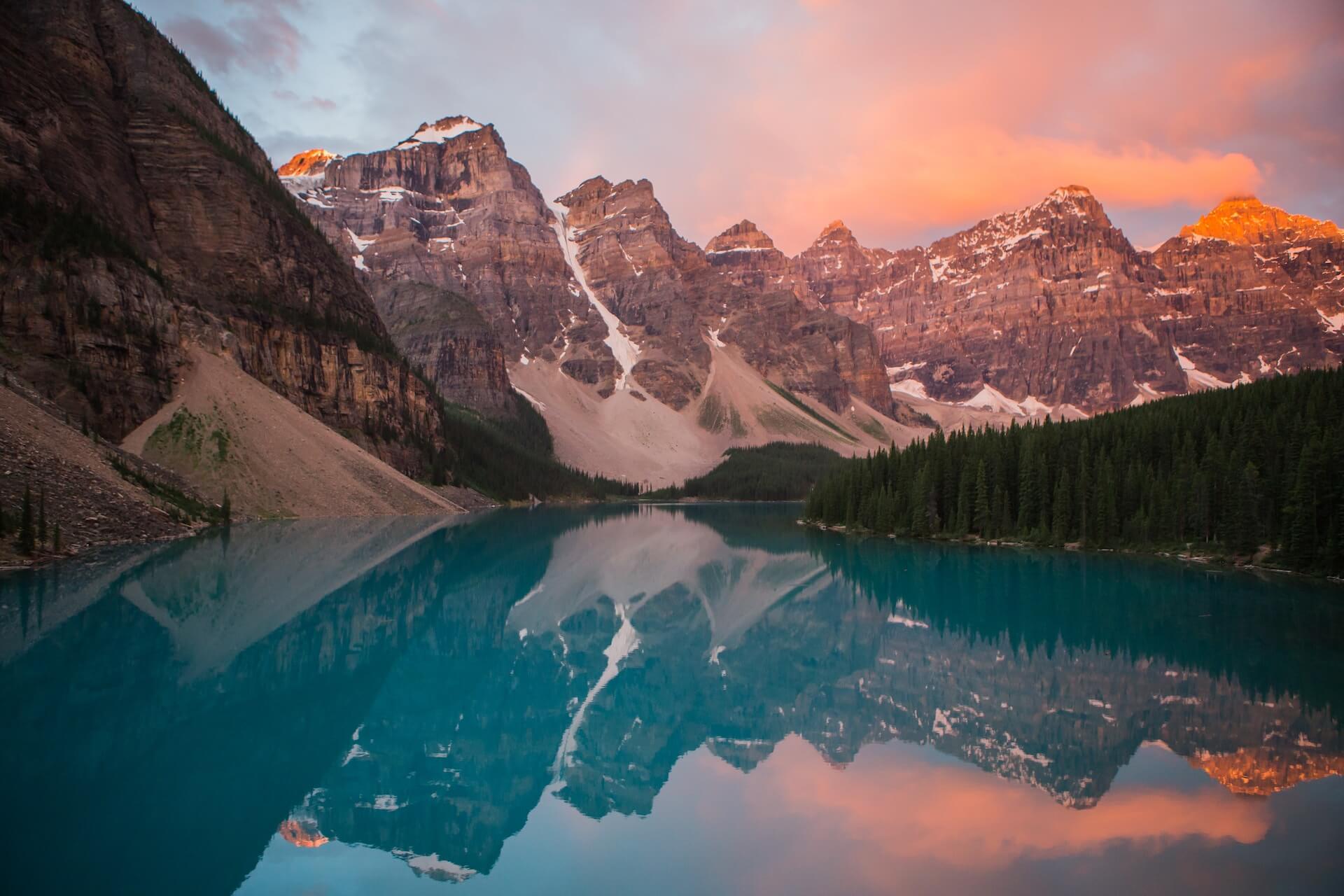
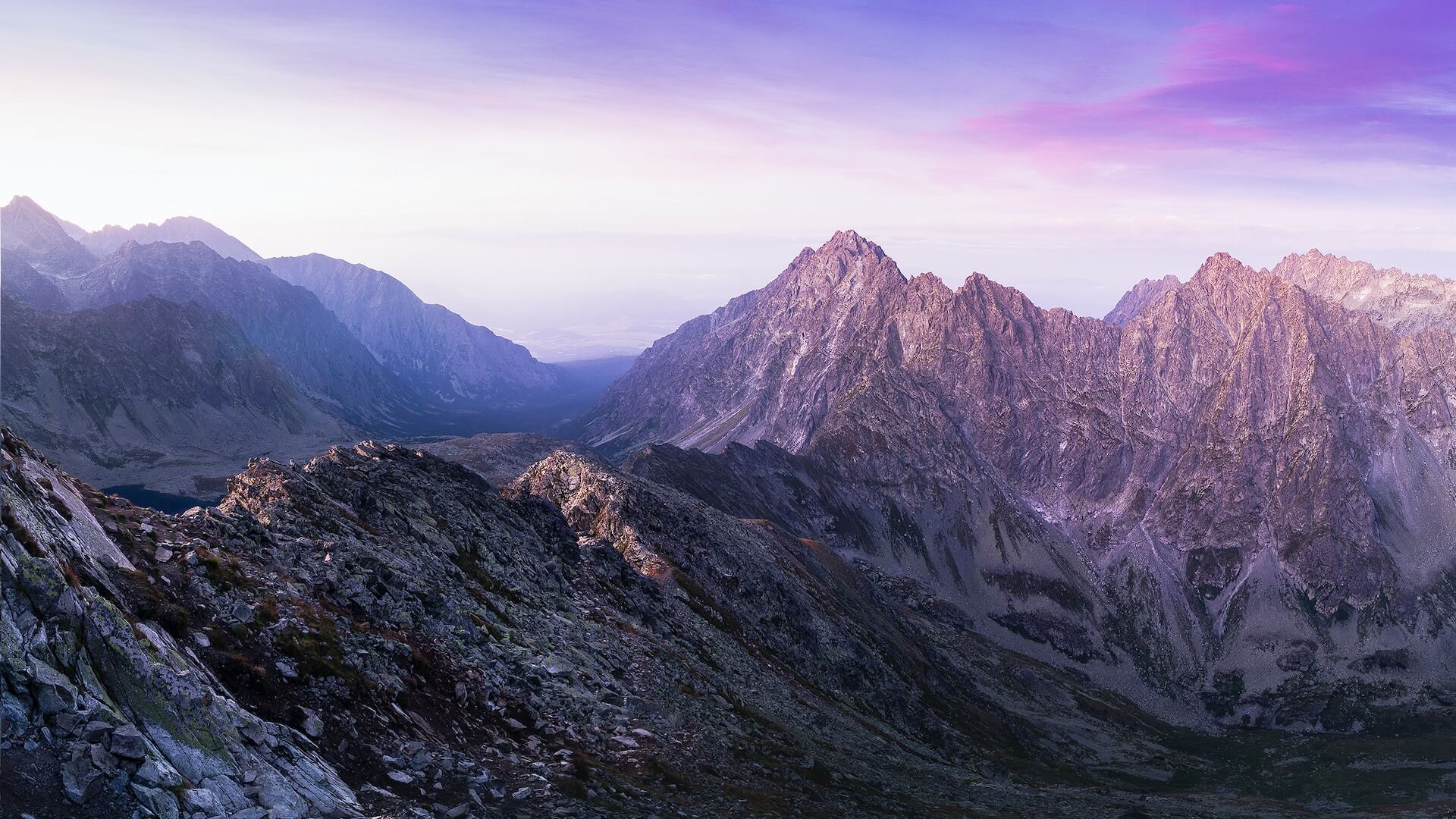
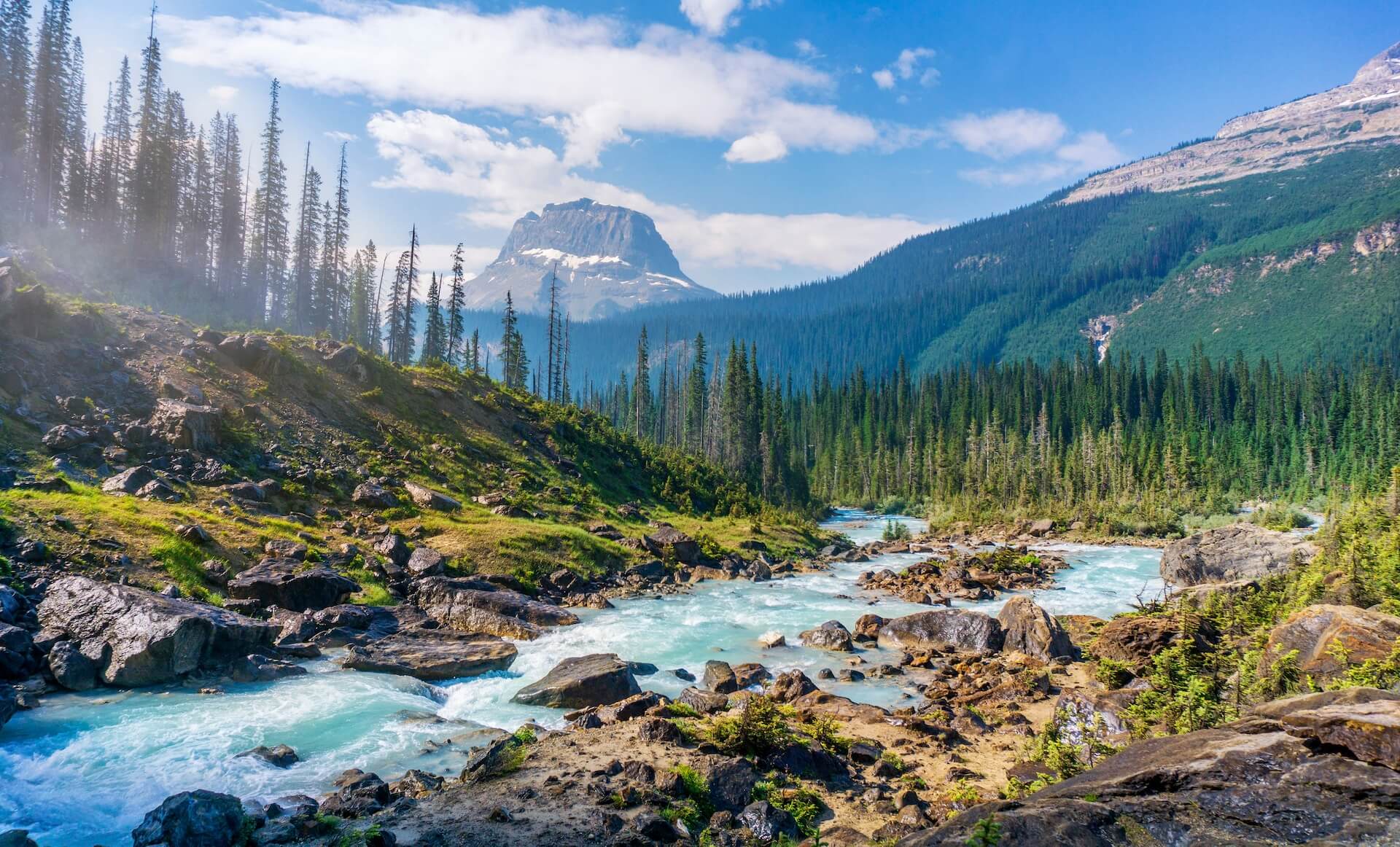
Copied !
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function MultipleSlidesWith2CarouselExample(props) {
const { slides } = props;
return <Carousel slides={slides} slidesToShow={2} />;
}
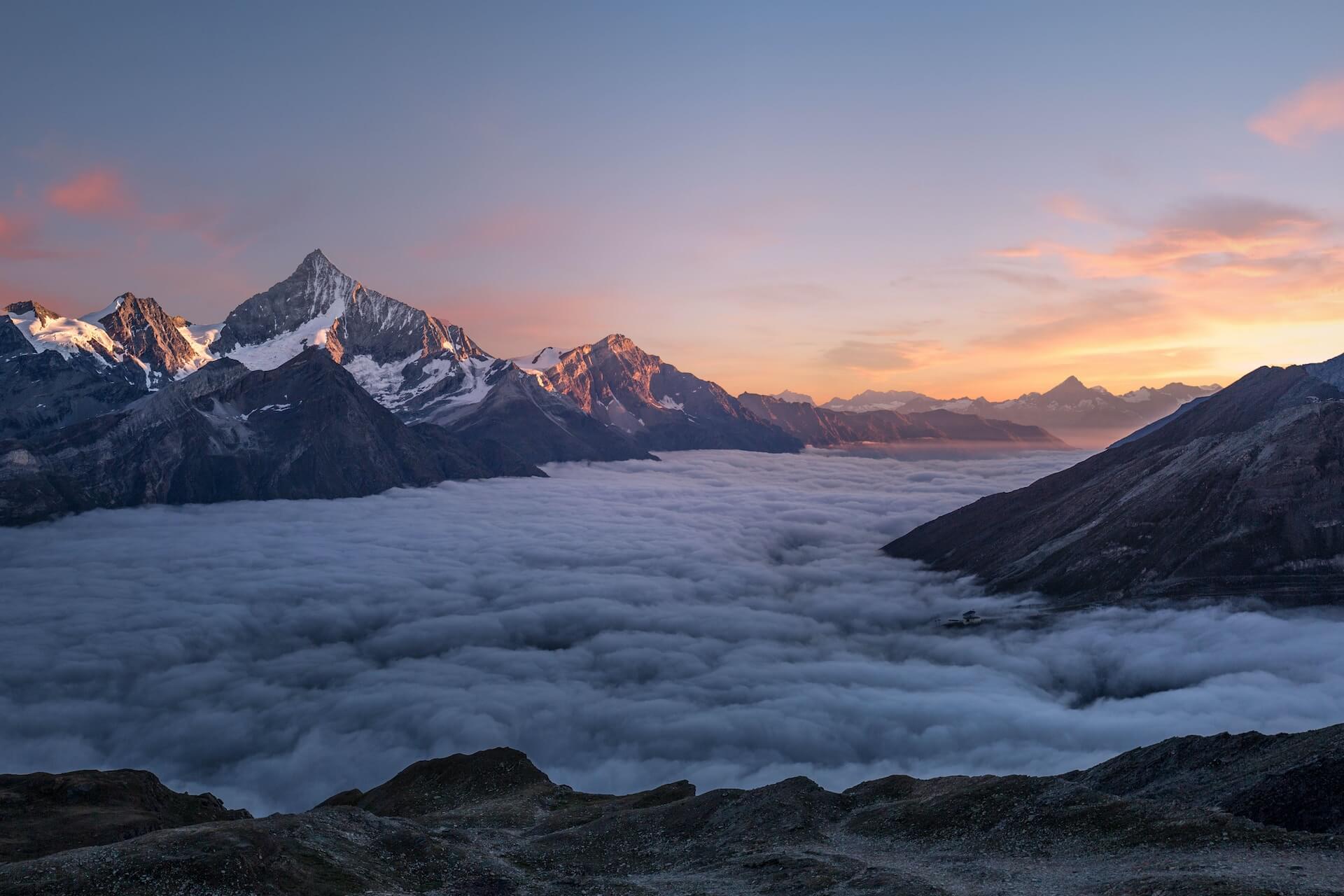
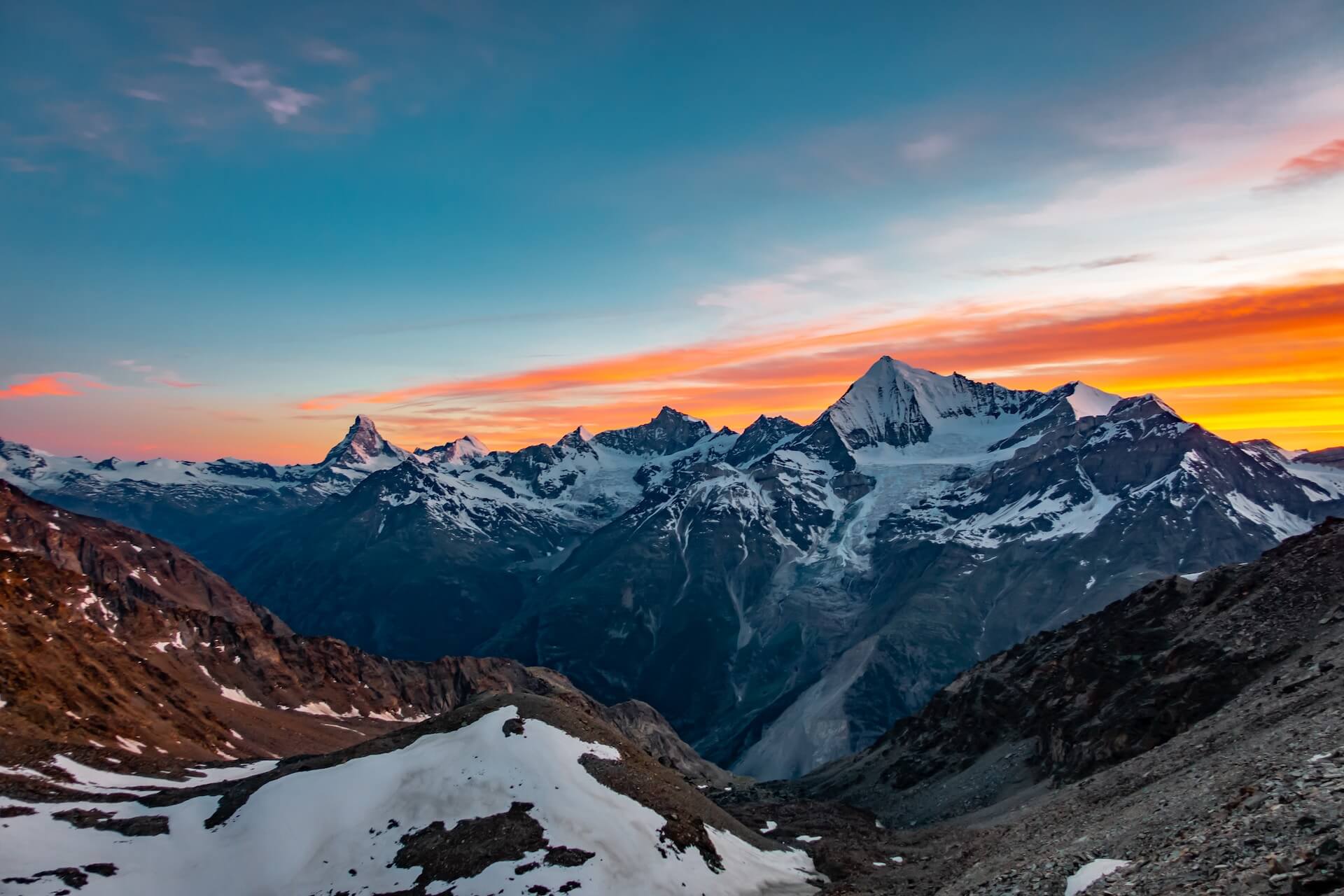
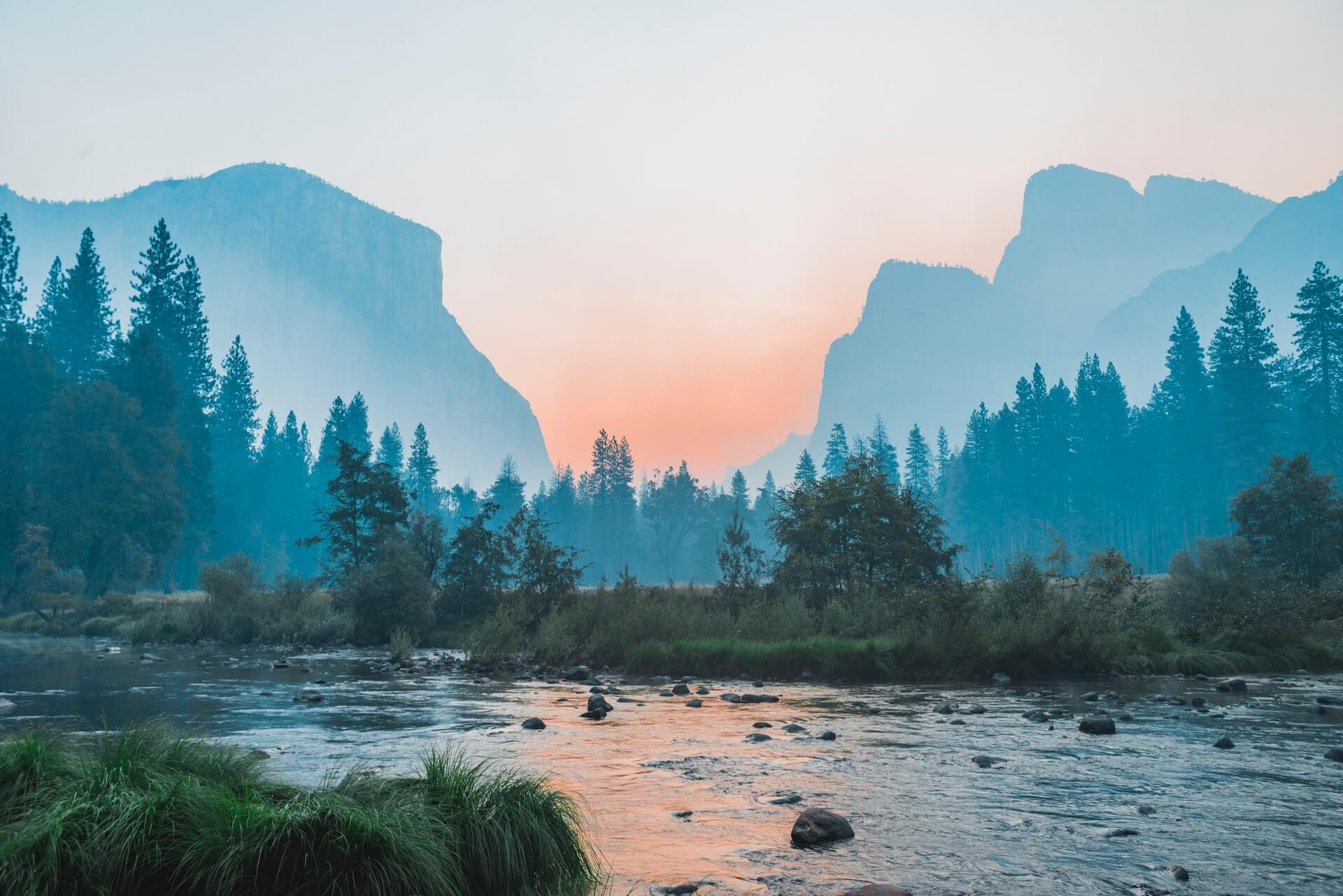
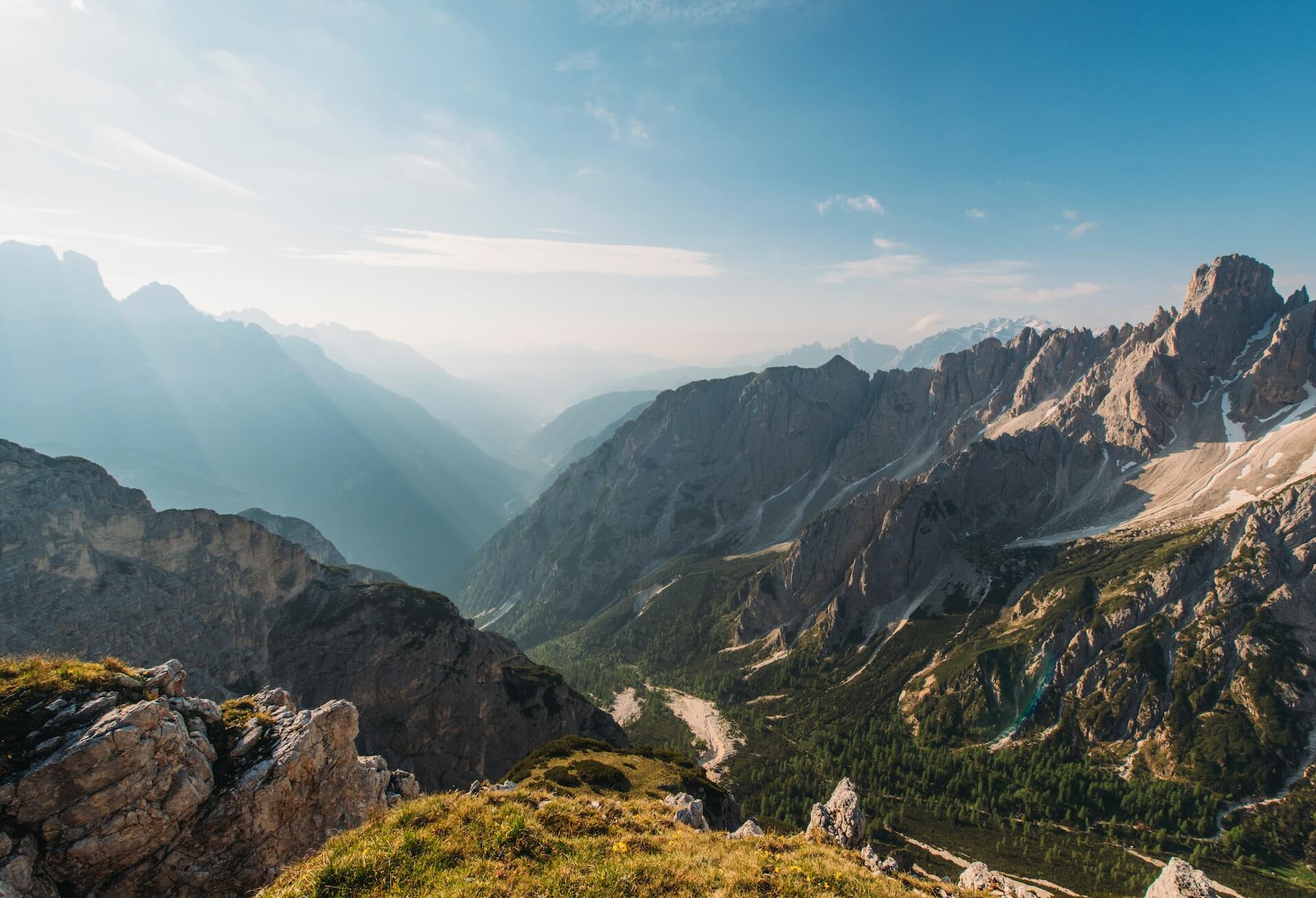
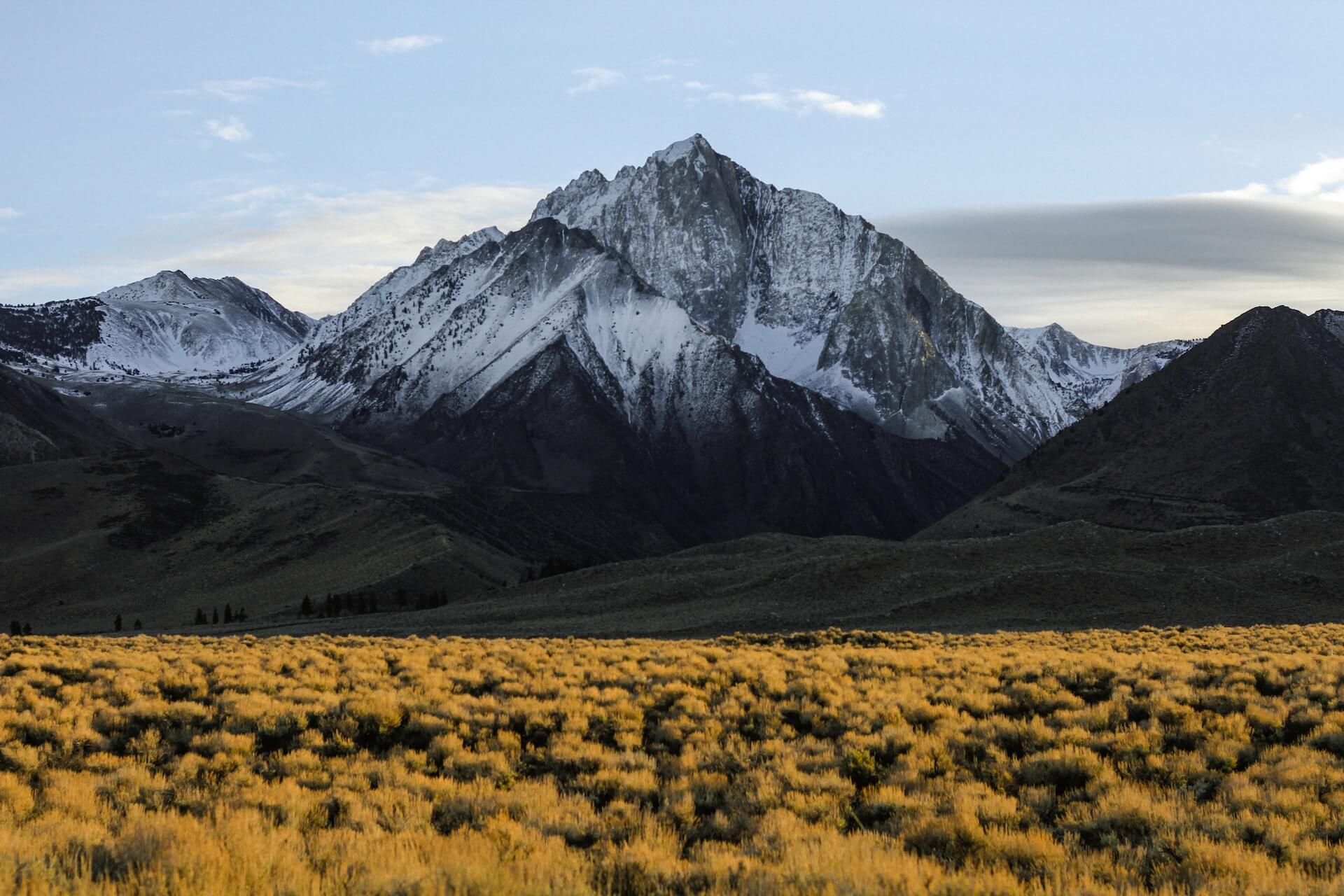
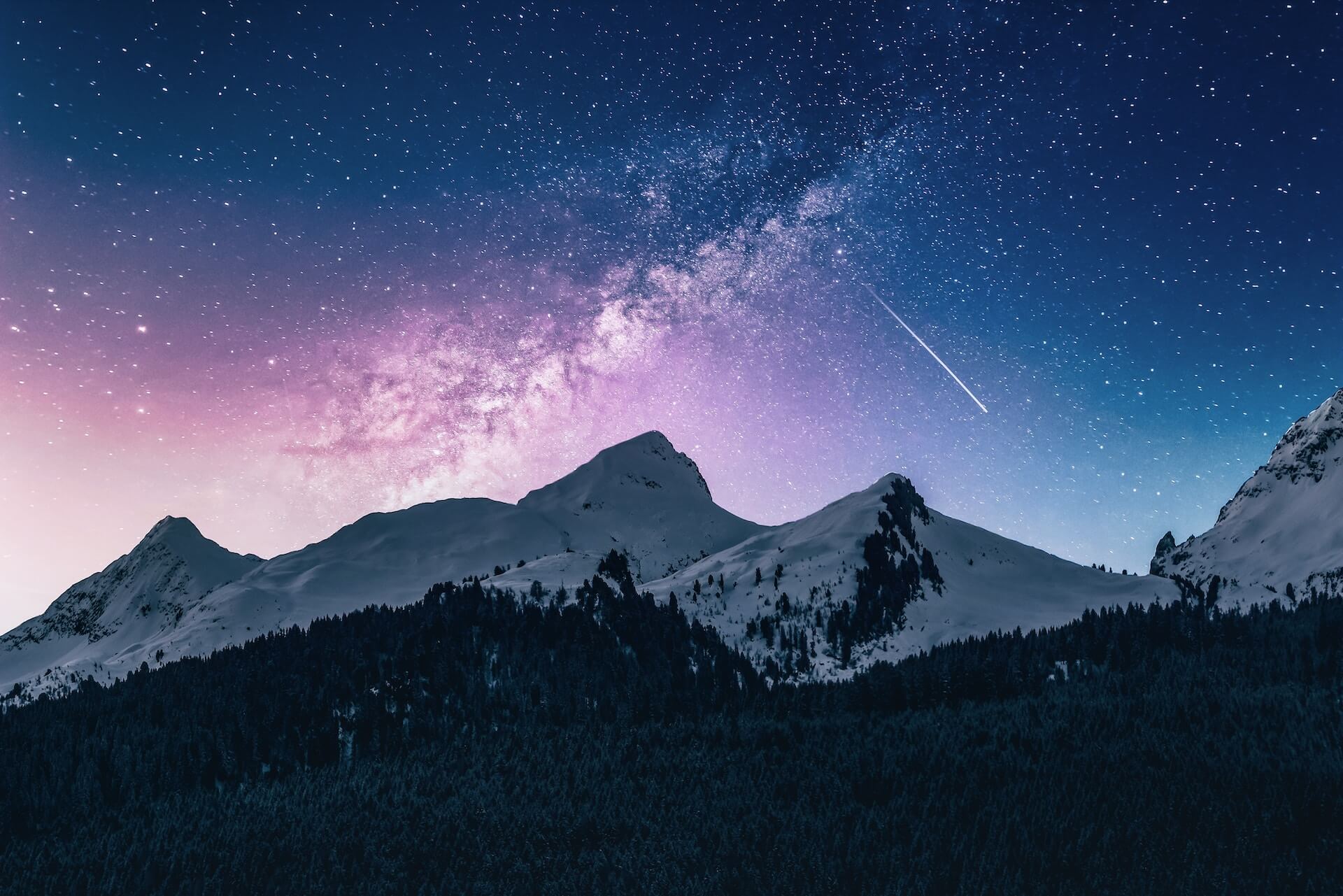
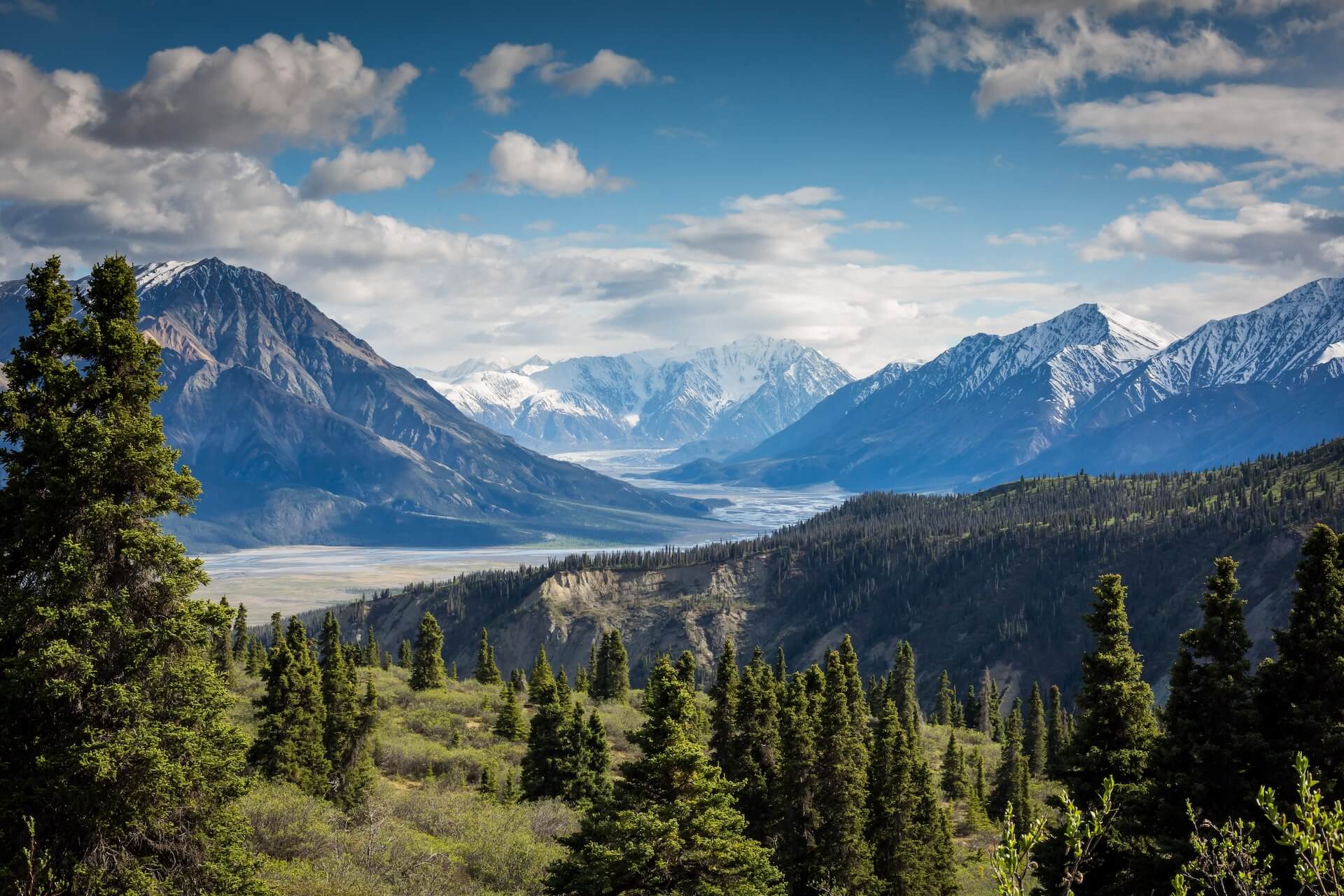
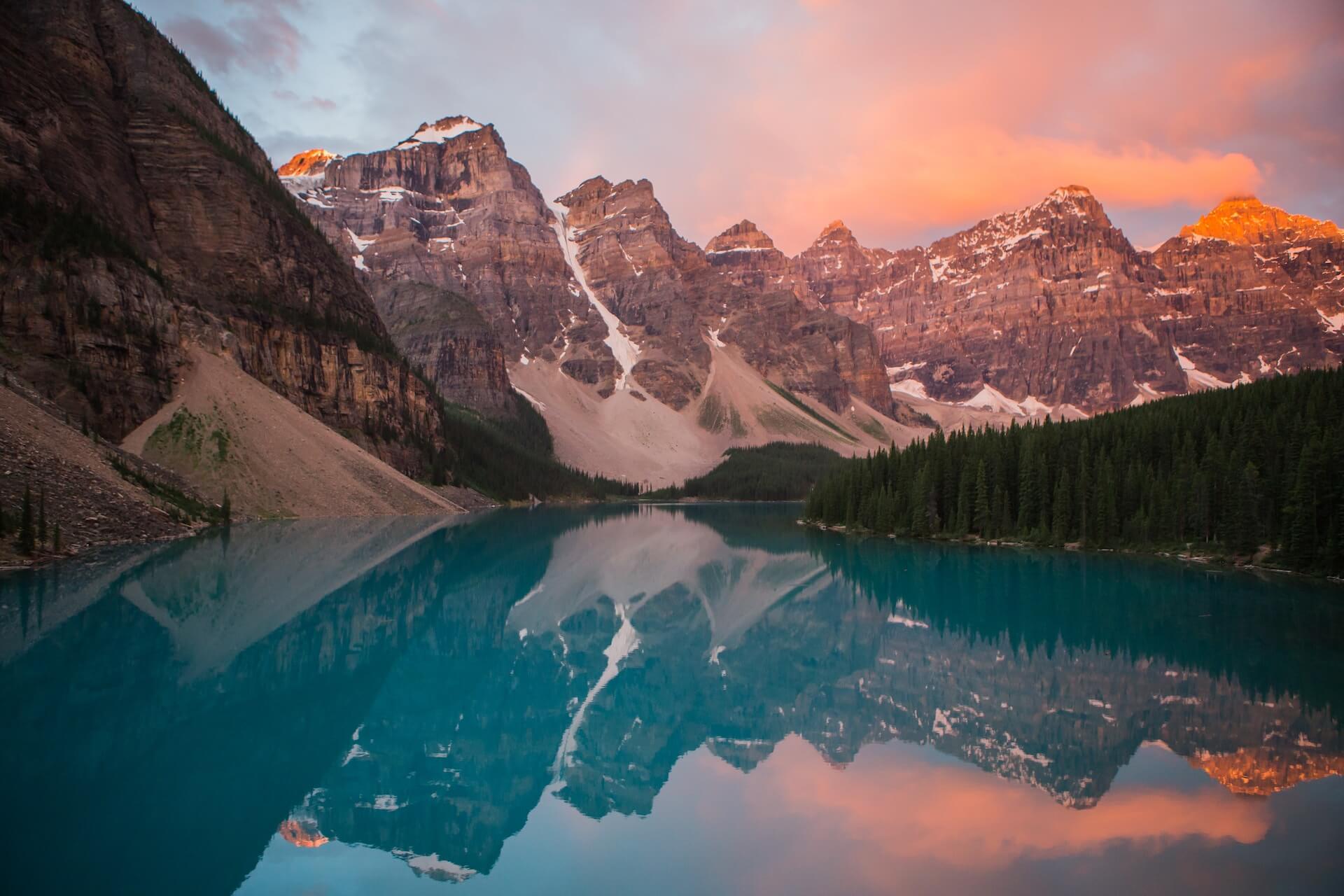
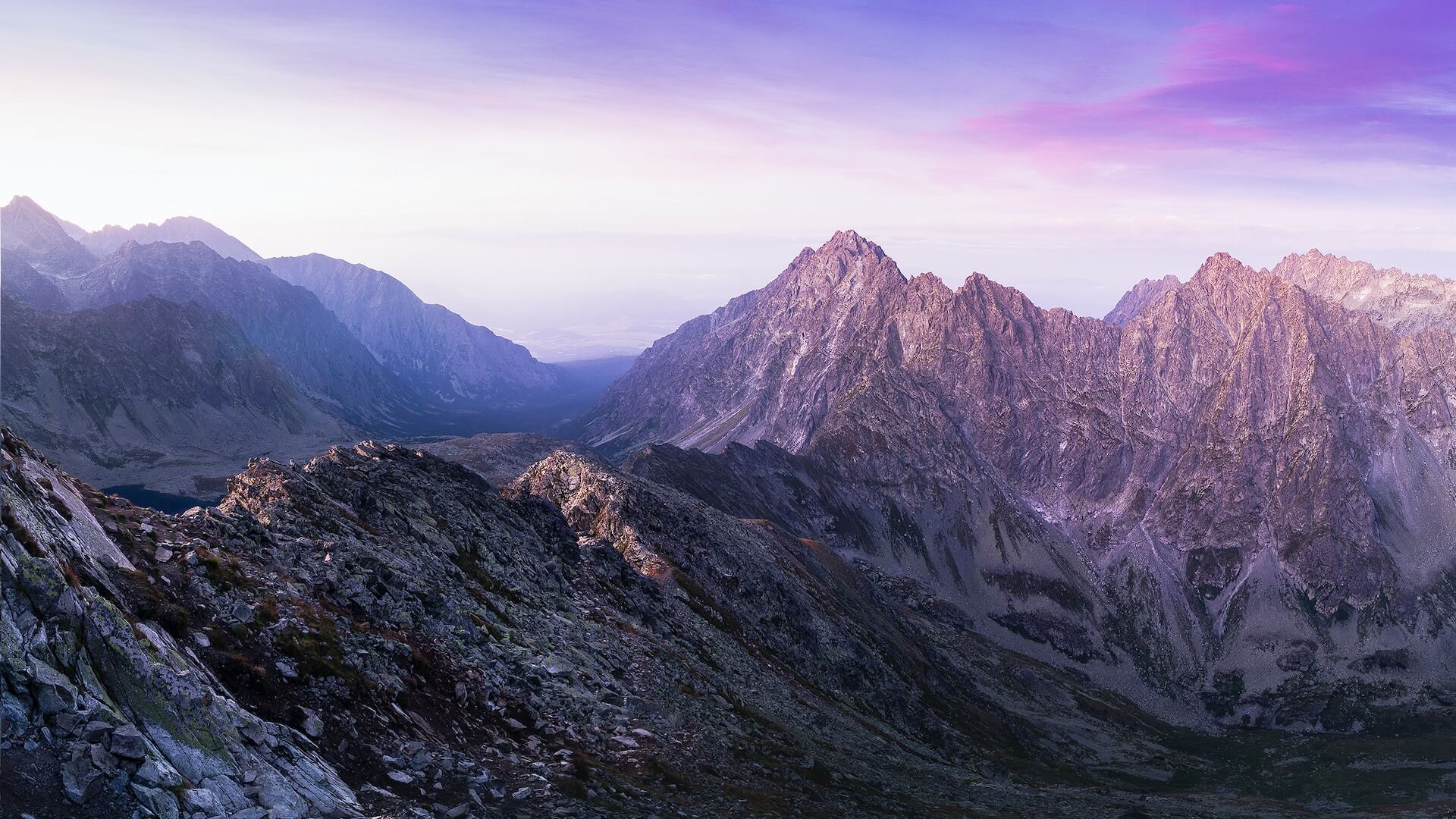
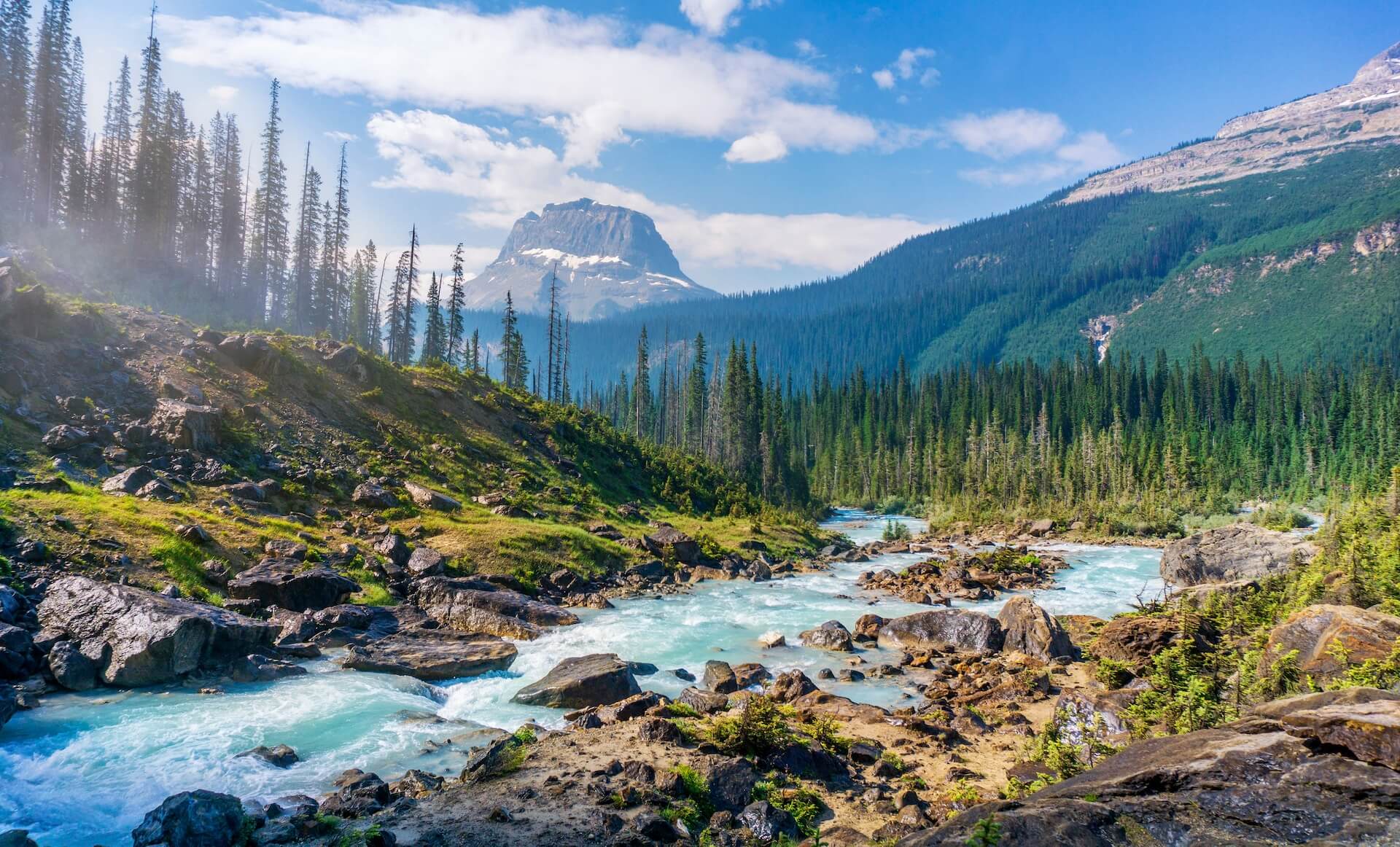
Copied !
import { InfoButton } from "$components/button.component";
import { Carousel } from "$components/carousel.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function MultipleSlidesWith3CarouselExample(props) {
const { slides } = props;
return (
<Carousel
slides={slides}
slidesToShow={3}
direction="vertical"
indicator="left"
navigations={
<div>
<InfoButton
x-bind:disabled="!loop && areFirstSlidesToShow"
x-on:click="previous()"
borderRadius="pill"
class="absolute left-1/2 -translate-x-1/2 top-4"
>
<span>Previous</span>
</InfoButton>
<InfoButton
x-bind:disabled="!loop && areLastSlidesToShow"
x-on:click="next()"
borderRadius="pill"
class="absolute left-1/2 -translate-x-1/2 bottom-4"
>
<span>Next</span>
</InfoButton>
</div>
}
loop
/>
);
}
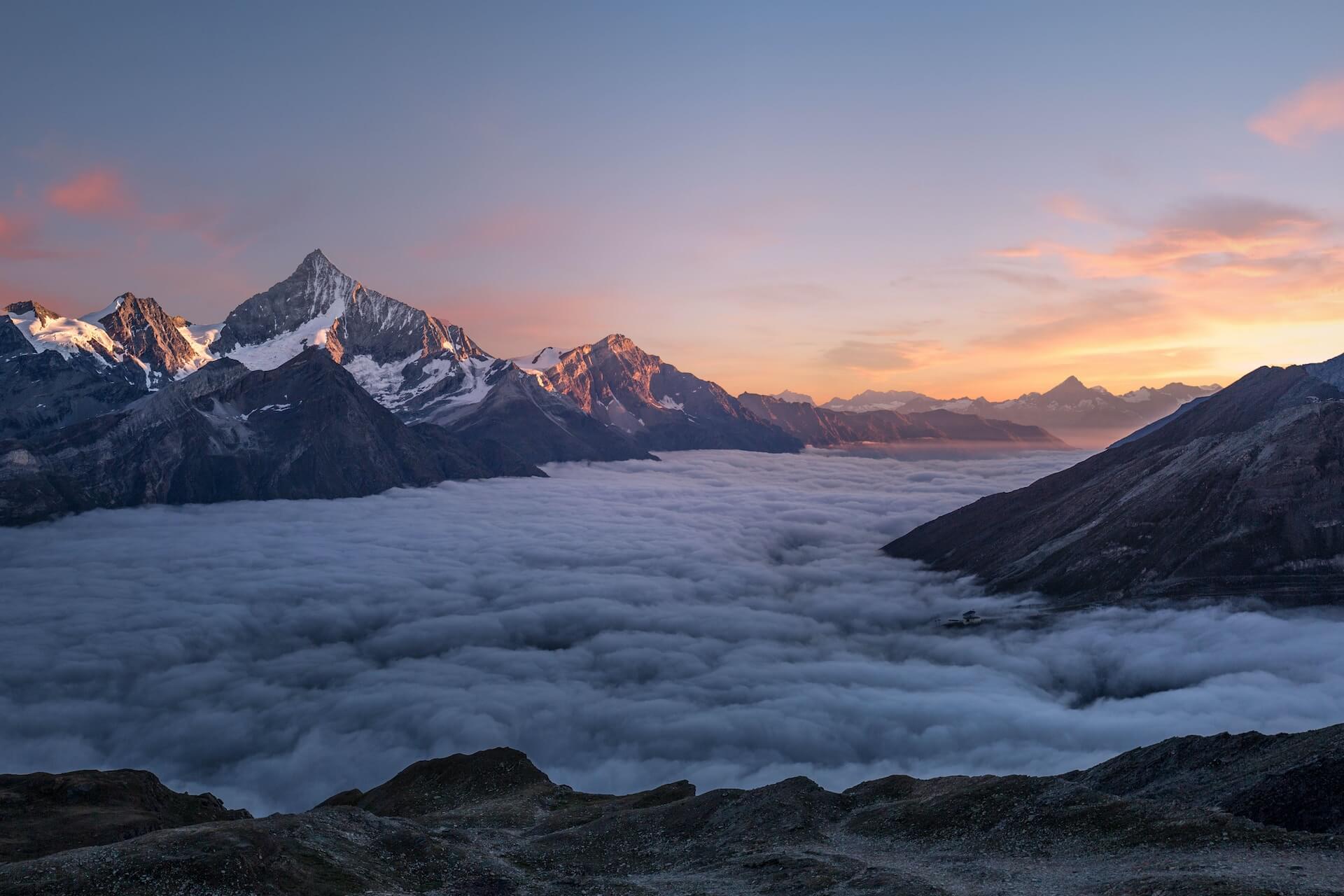
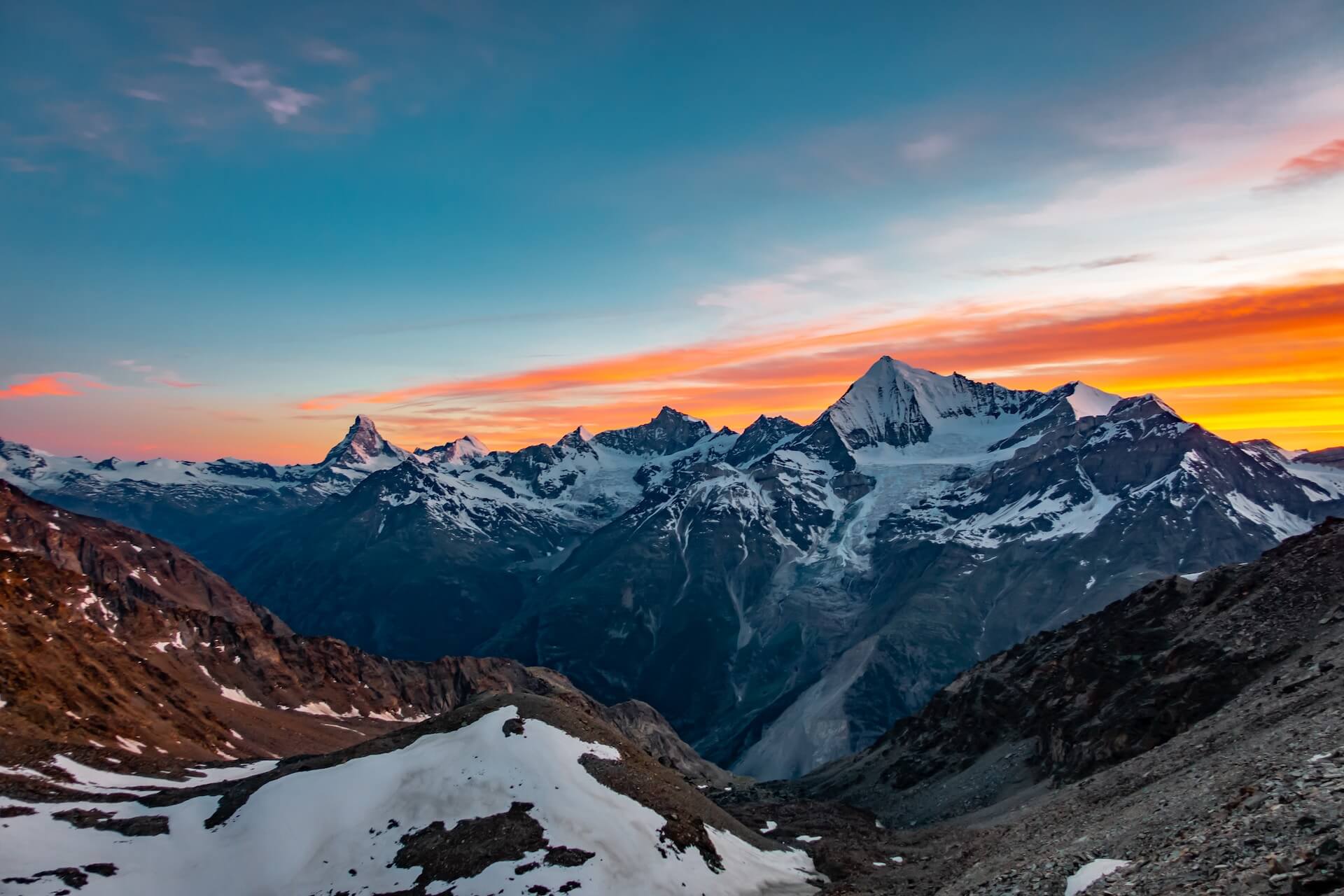
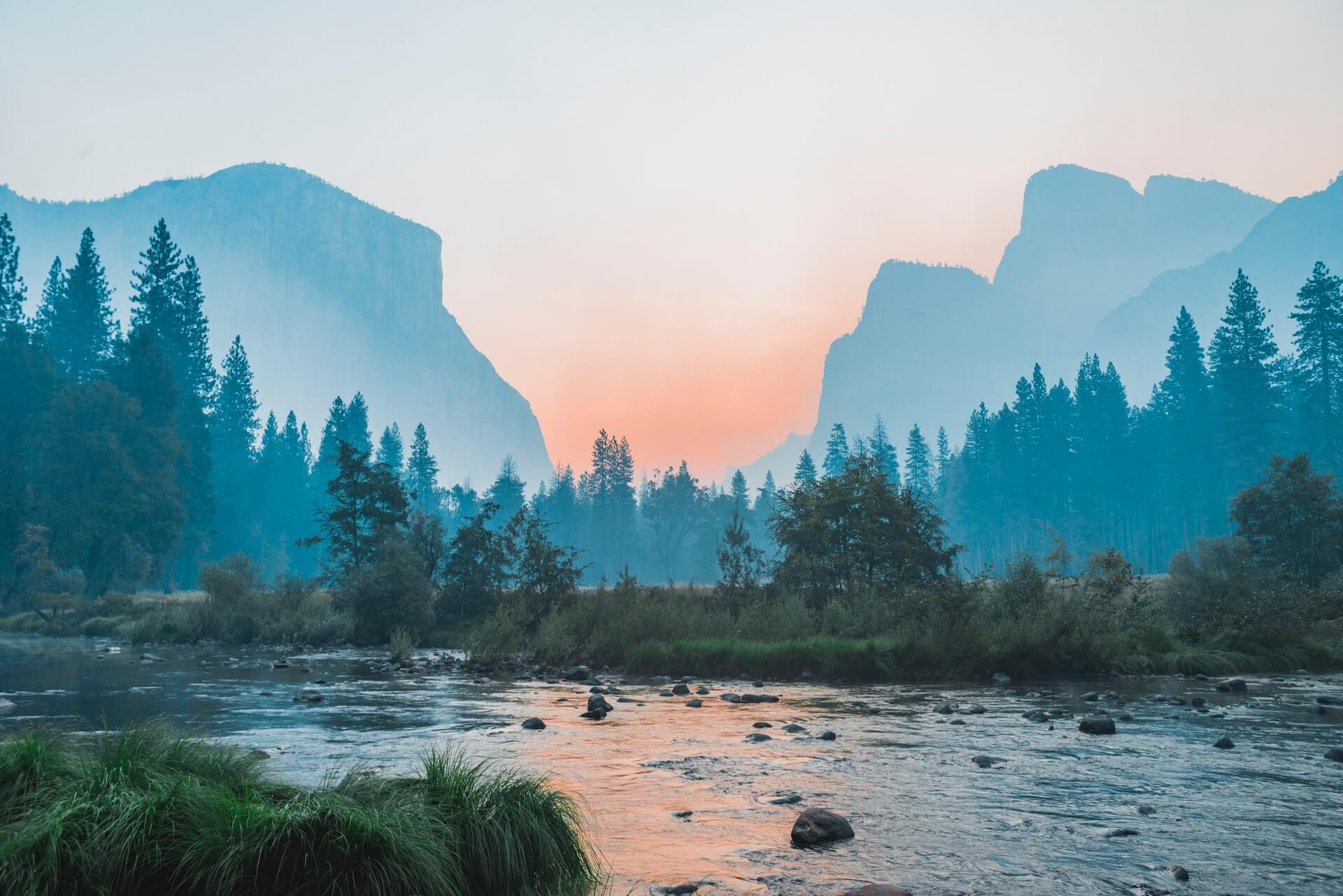
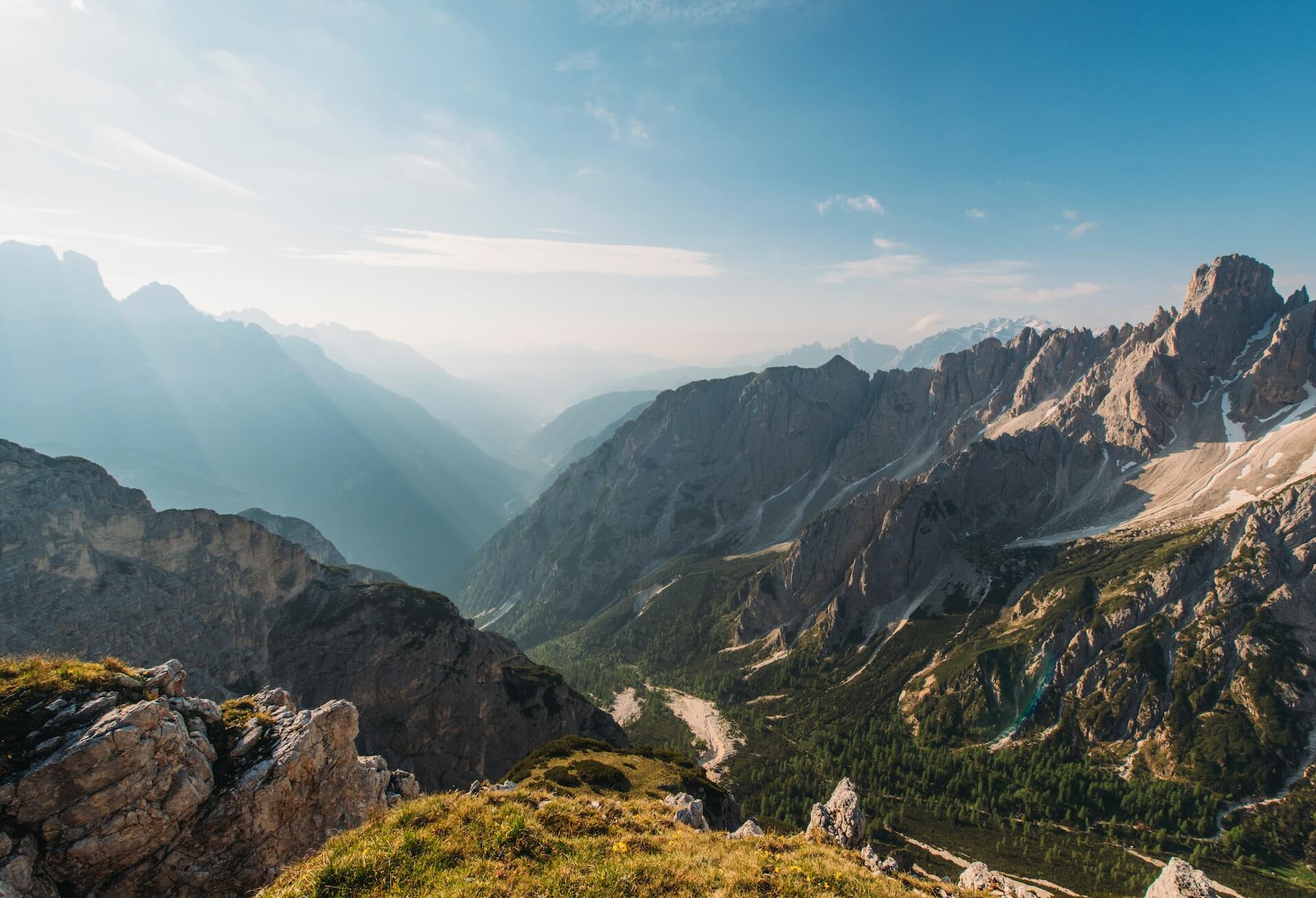
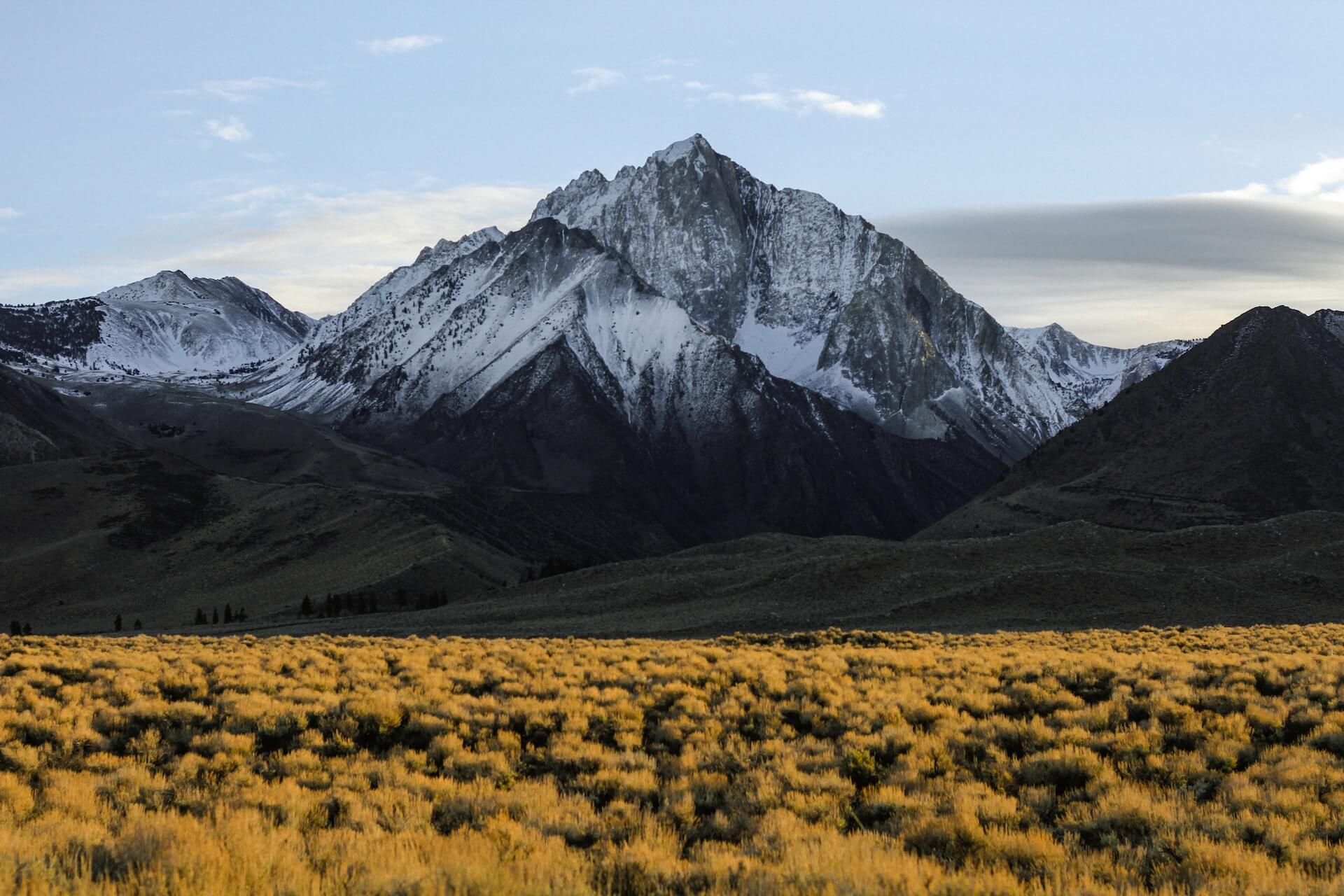
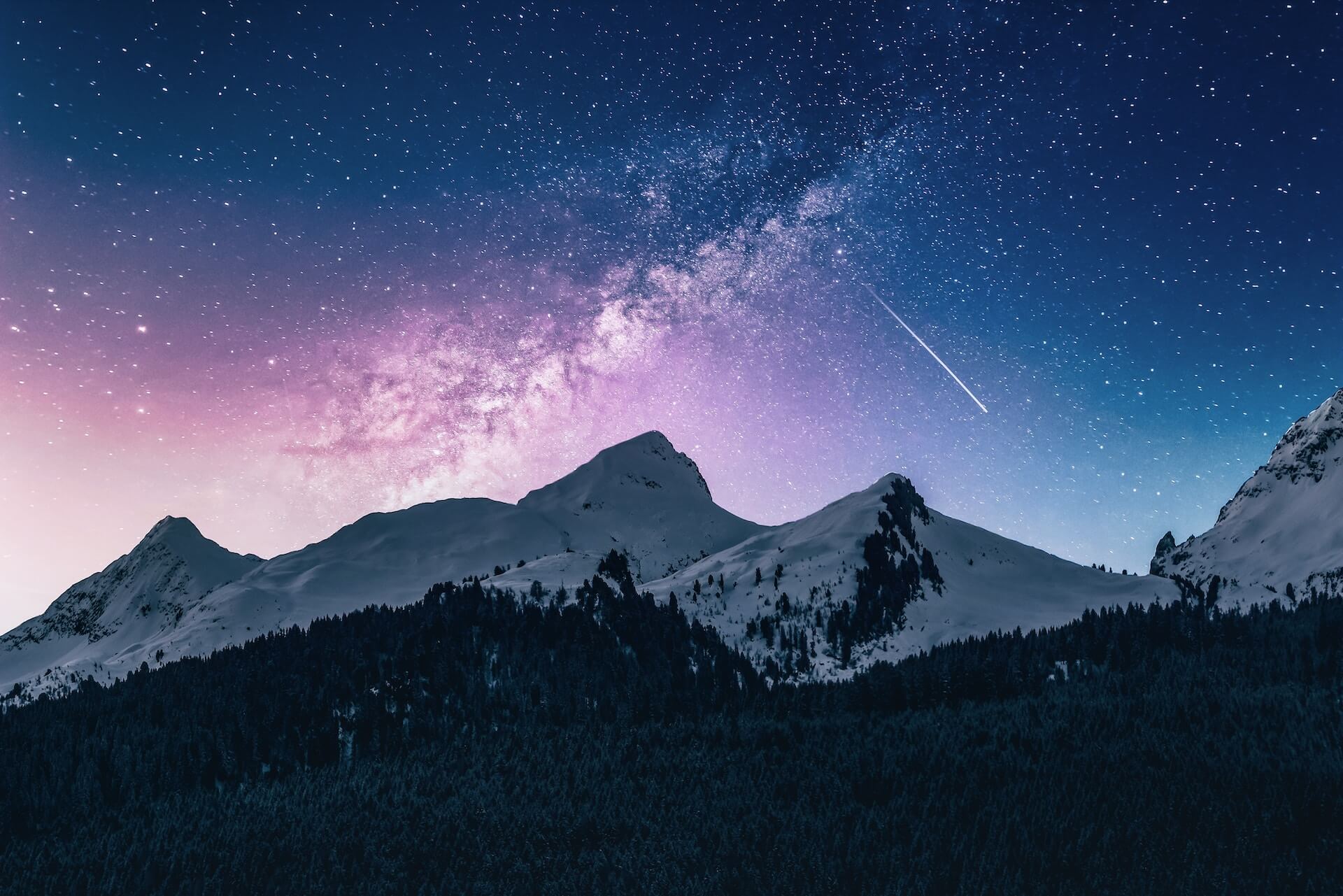
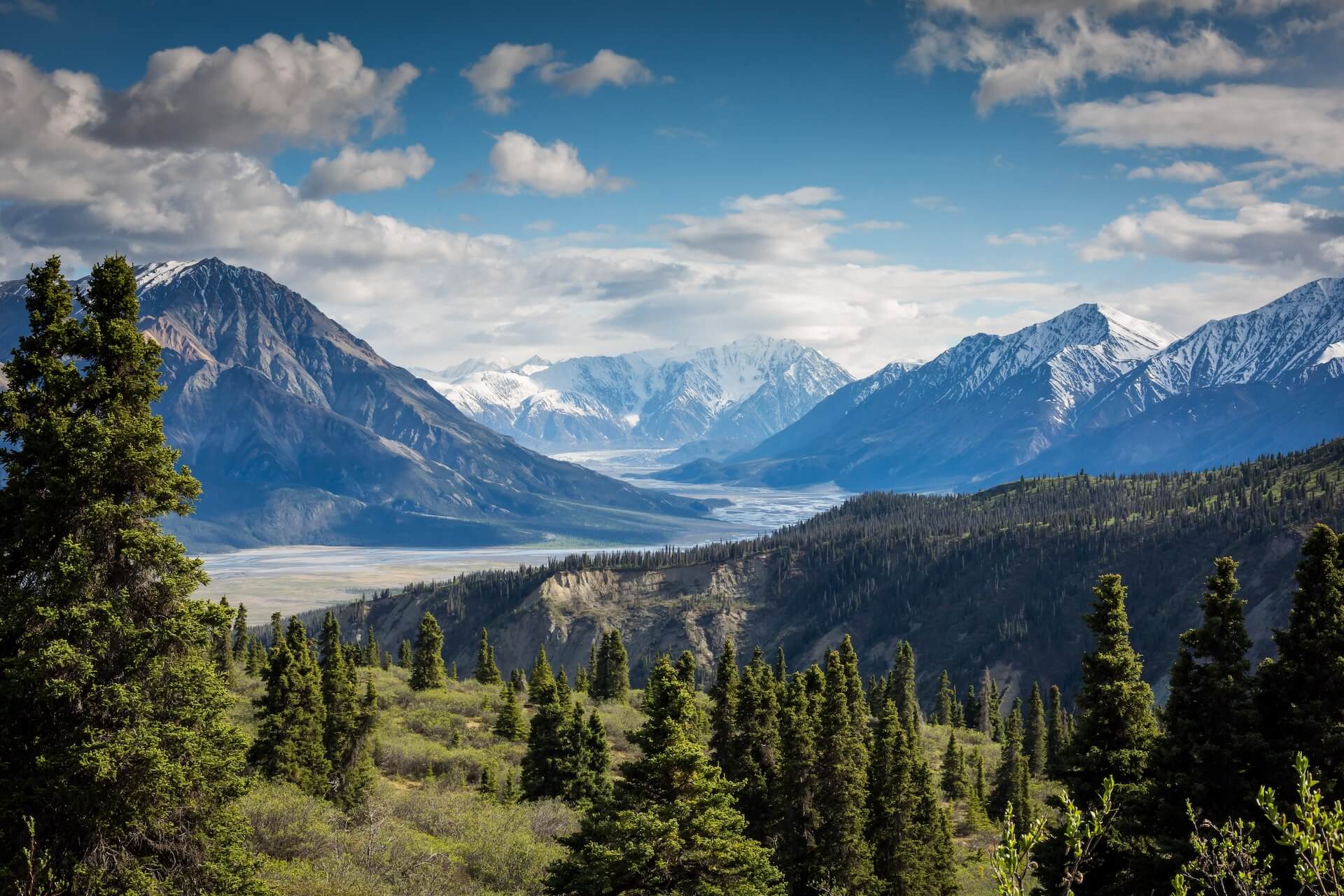
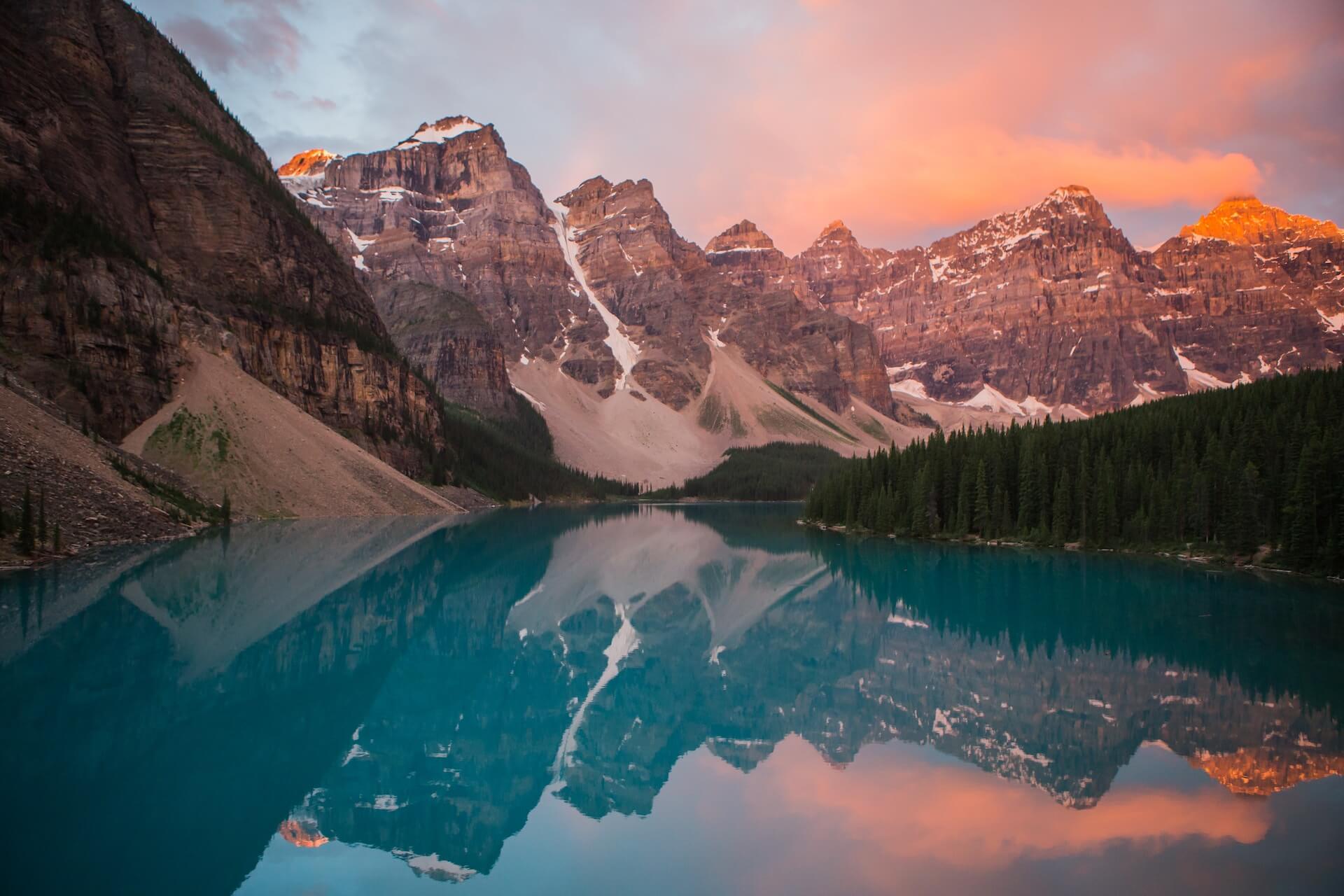
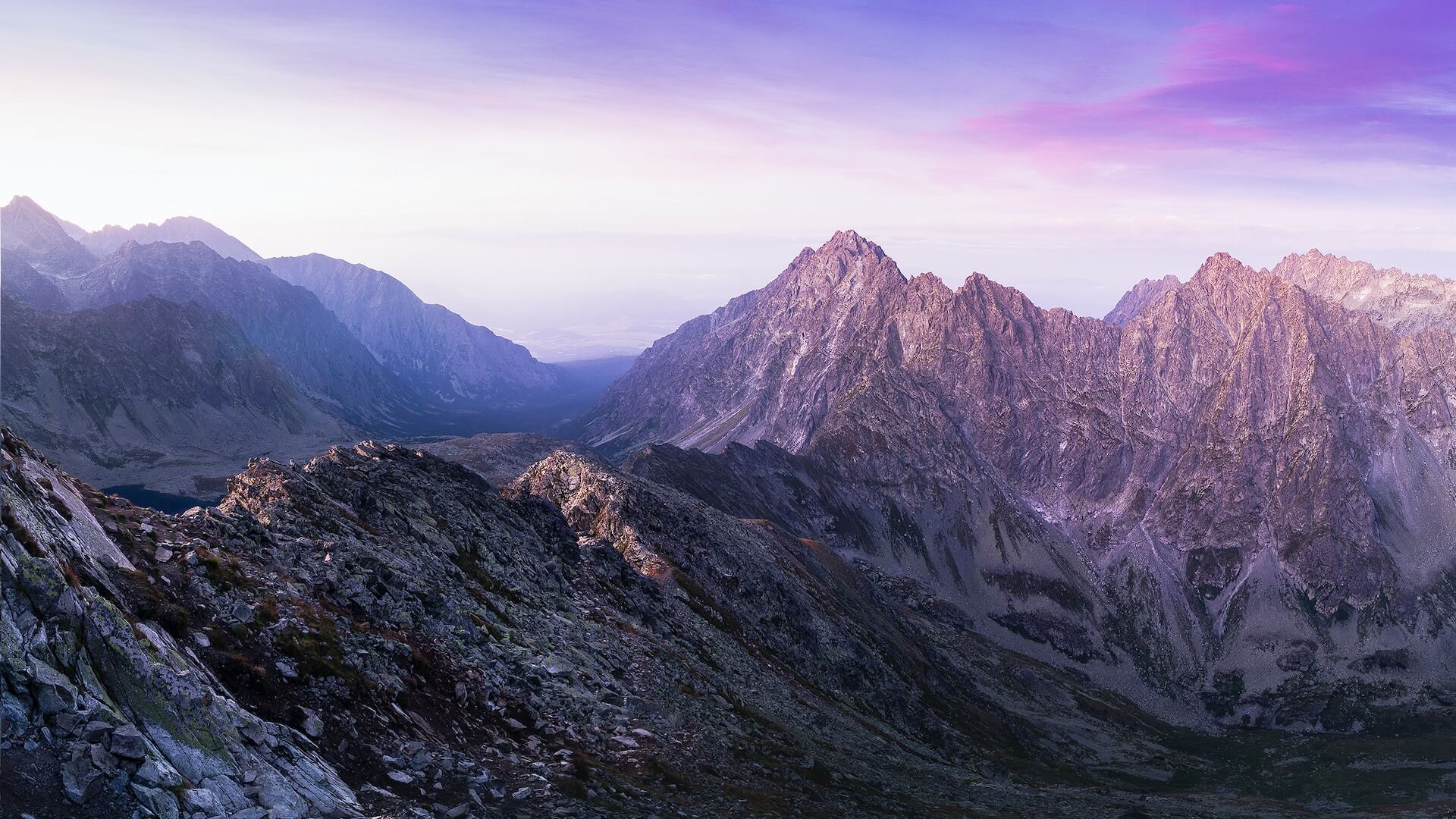
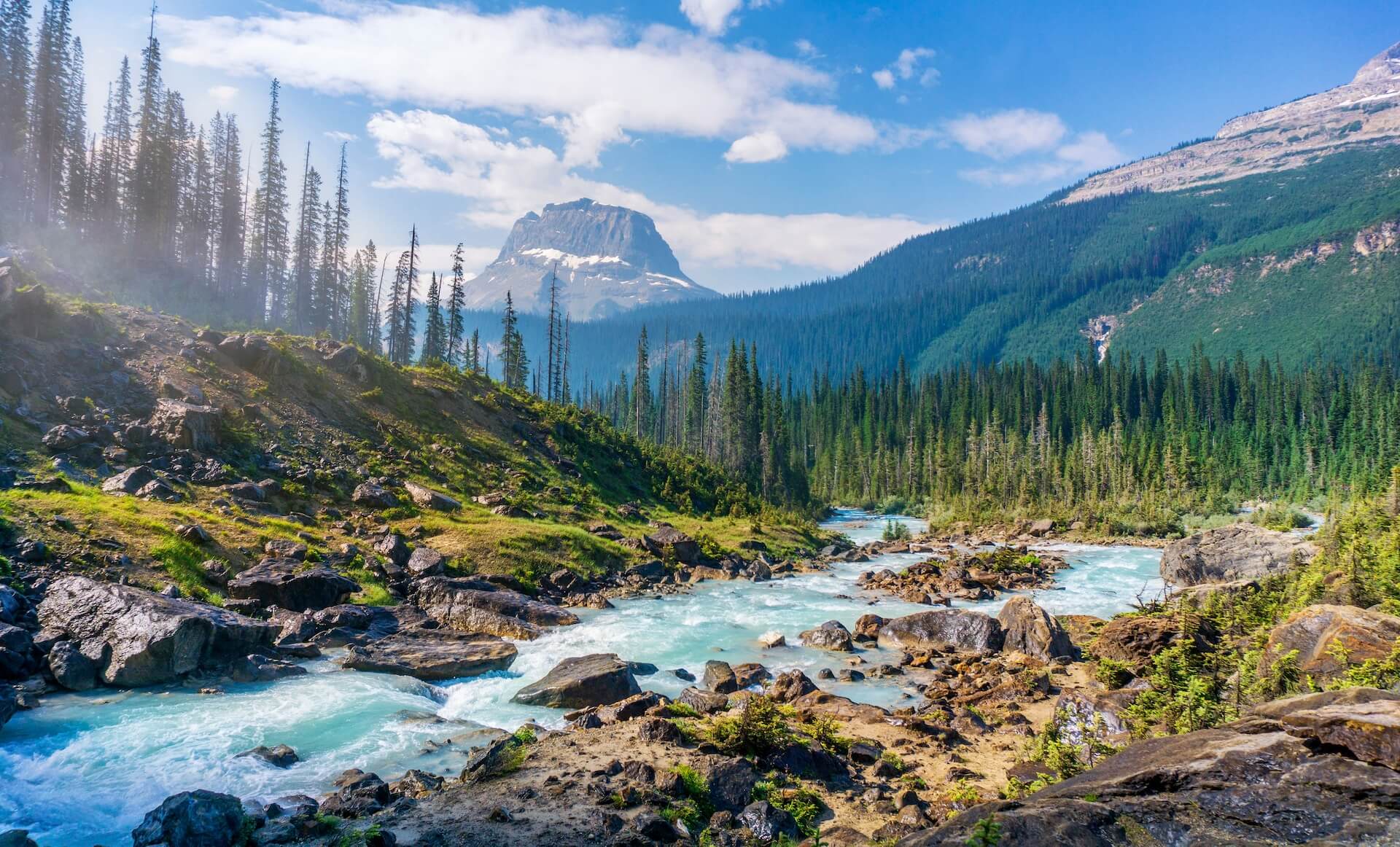
Copied !
import { Carousel } from "$components/carousel.component";
import { Image } from "$components/image.component";
/**
* @type {import("$common/props").JSXComponent<{ slides: import("$components/image.component").ImageType[] }>}
*/
export function MultipleCustomSlidesWith2CarouselExample(props) {
const { slides } = props;
return (
<Carousel slidesToShow={3} loop>
{slides.map((image) => (
<Image class="w-1/3 h-full" src={image.src} alt={image.alt} />
))}
</Carousel>
);
}