Galleries
Overview
Gallery is a set on photos in a grid-like design. Based on your needs, JSXPine provides you 3 types of gallery.
Discover each one and their variants with examples below.
Rest Gallery
Take RestGallery as a way to reduce display all photos. Because too many images can slower your page loading, it's pragmatic to display a few.
With RestGallery, you set a number of images to display, and then, choose to display the others. By default, 3 images are displayed.
Here below some examples with different displayed images.
P.S: Notice that it's up to you to design gallery layout with class props. Thanks to tailwind, you can fully customize it.
- +
- +
- +
Copied !
import { RestGallery } from "$components/gallery.component";
/**
* @type {import("$common/props").JSXComponent<{ images: import("$components/image.component").ImageType[]}>}
*/
export function DefaultRestGalleryExample(props) {
const { images } = props;
return (
<div class="flex flex-col gap-y-12">
<RestGallery class="flex flex-wrap [&_li]:w-1/2" images={images} />
<hr />
<RestGallery class="flex flex-wrap [&_li]:w-1/4" images={images} />
<hr />
<RestGallery
nbDisplayedImages={4}
class="grid grid-cols-2 w-3/4 mx-auto gap-2"
images={images}
/>
</div>
);
}
Carousel Gallery
A trendy gallery is obviously carousel. It's a combination of Carousel component with a set of thumbnails as indicators.
You can change direction with _direction_ props and define the thumbnail width with thumbnailWidth props.
See example below:
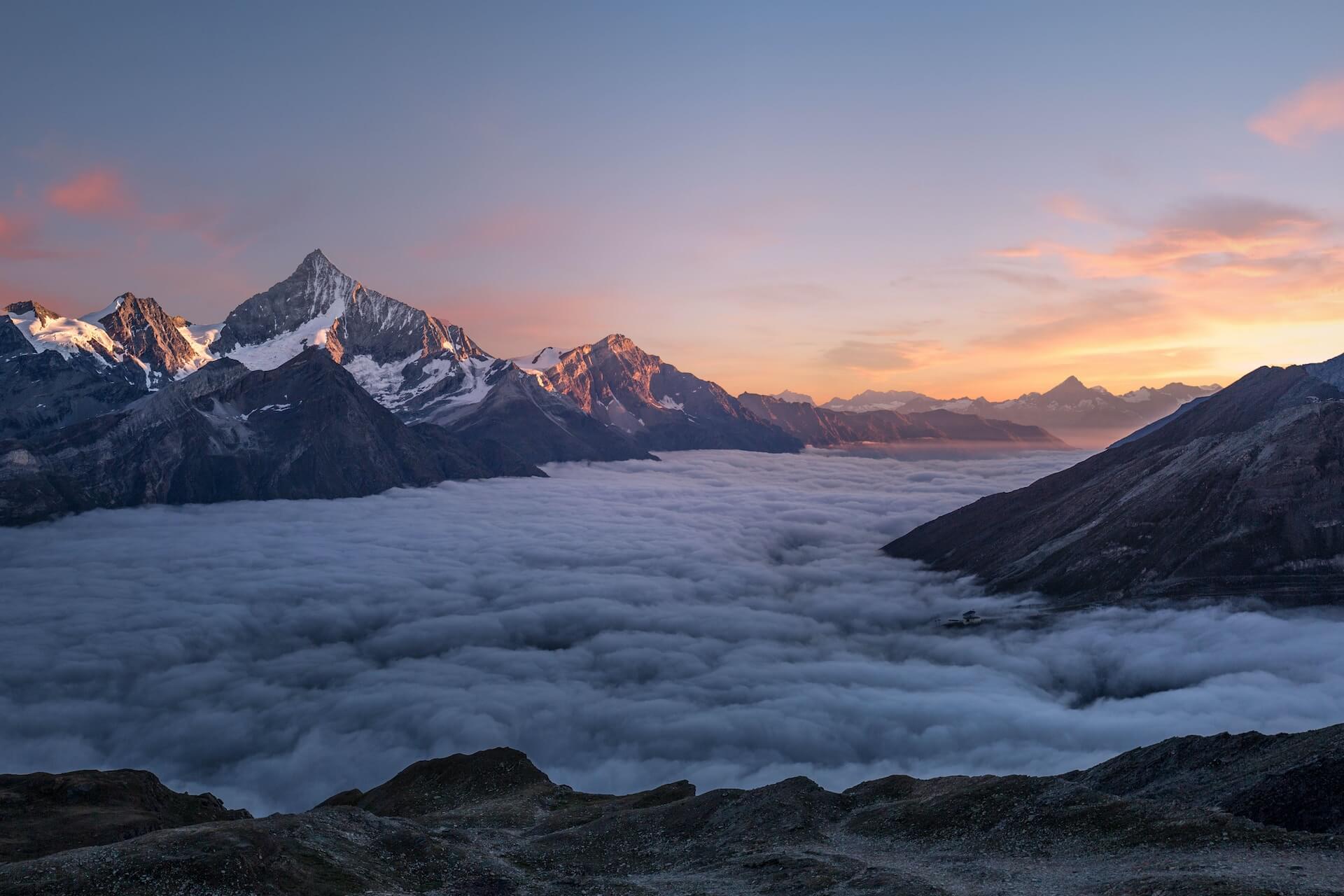
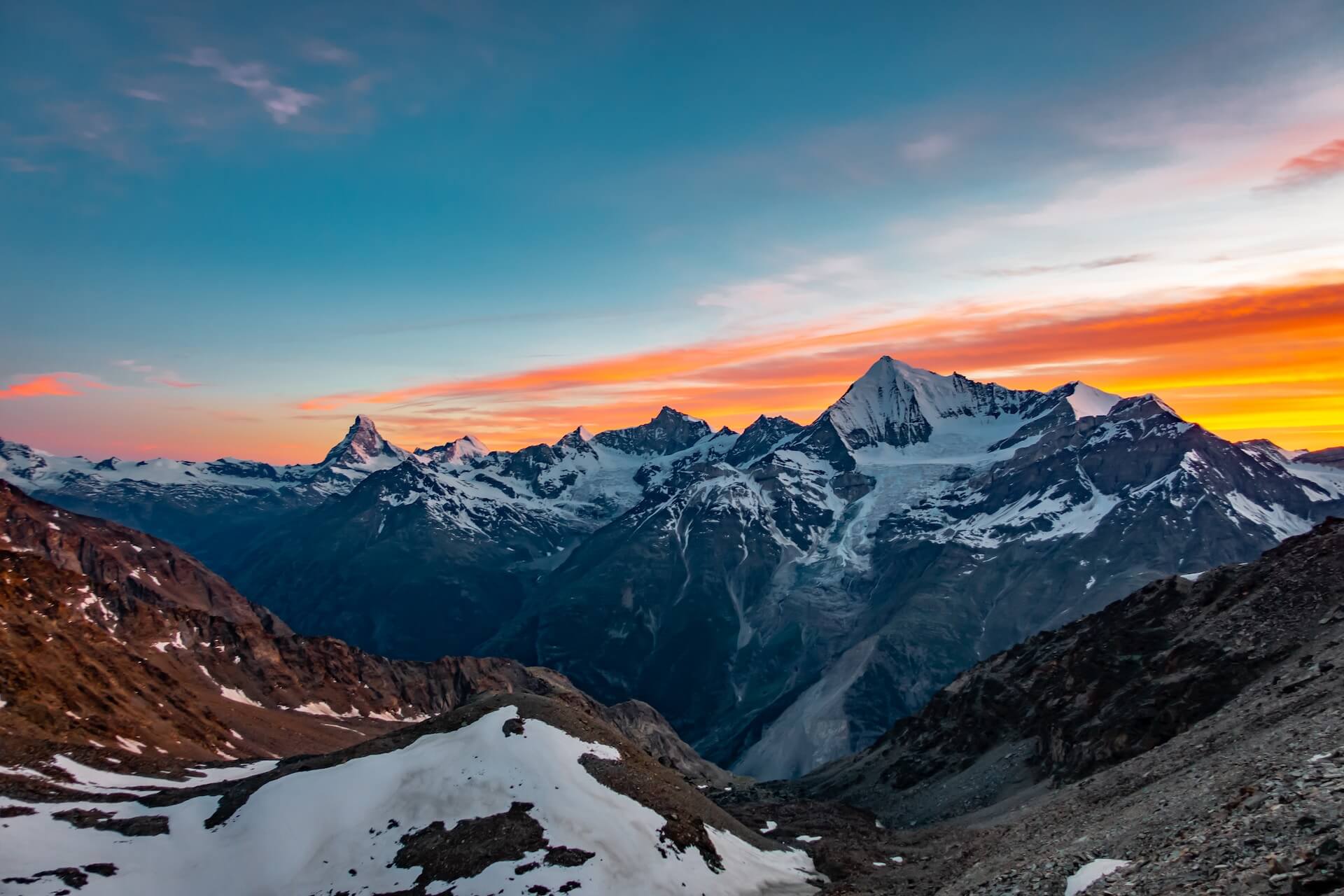
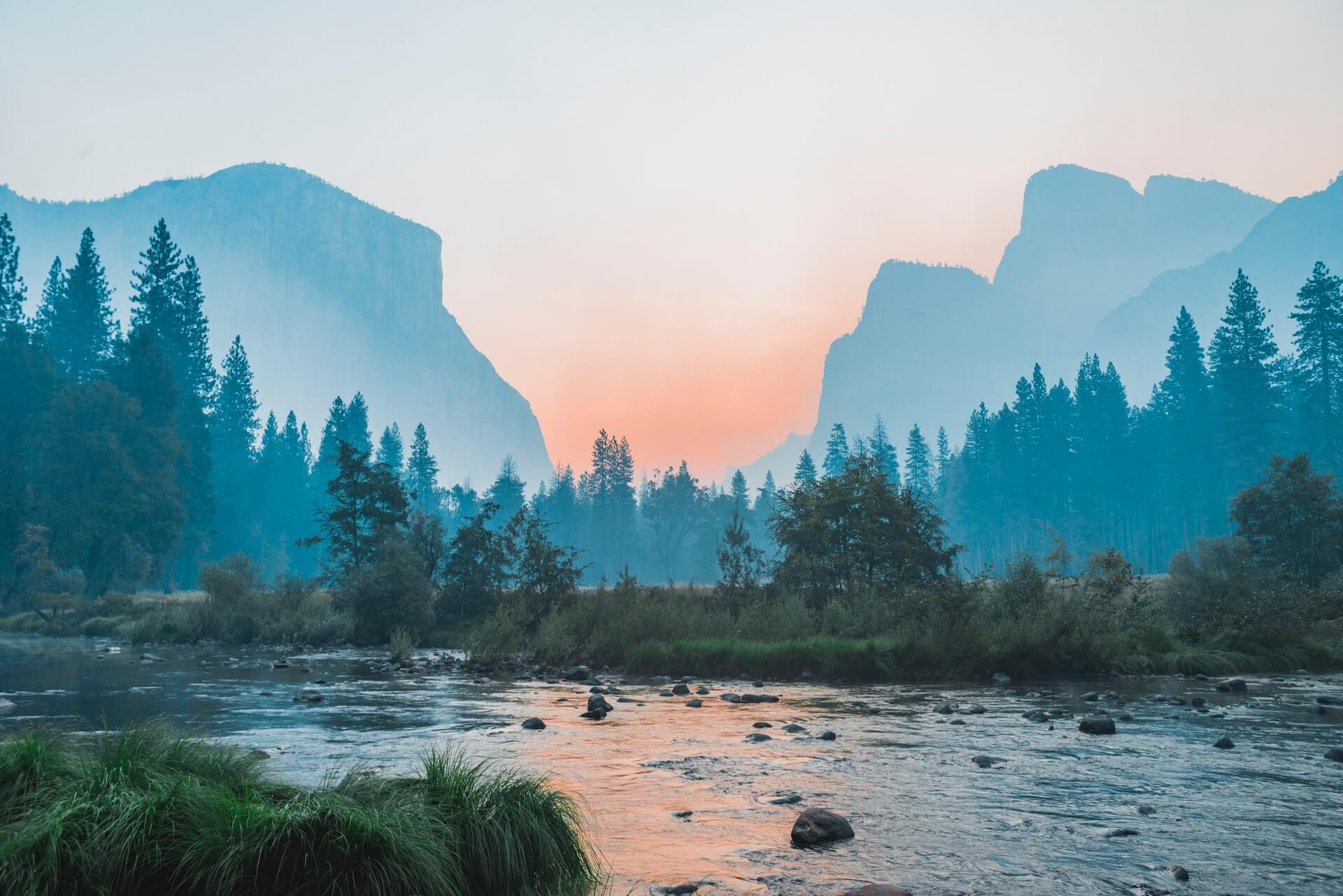
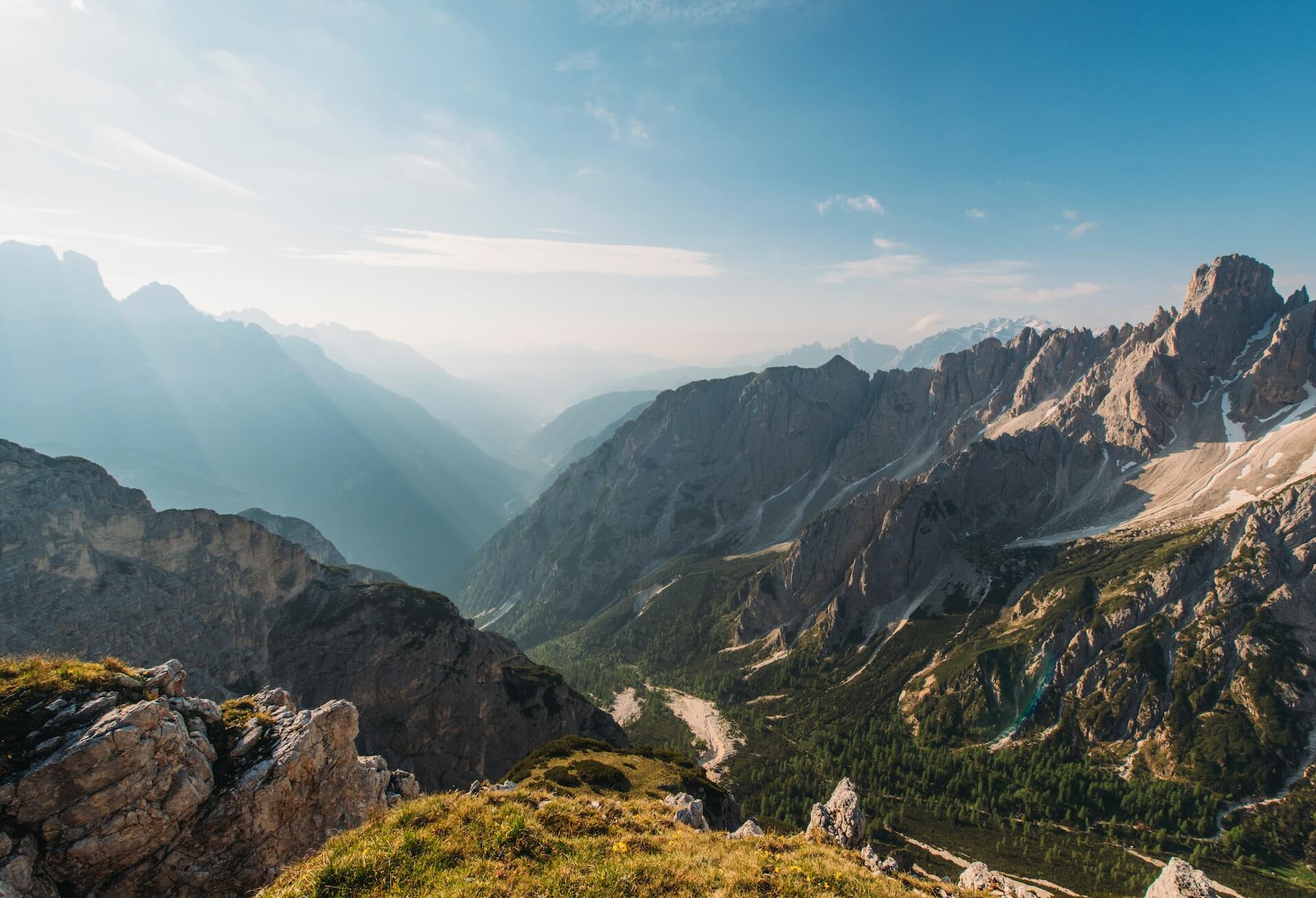
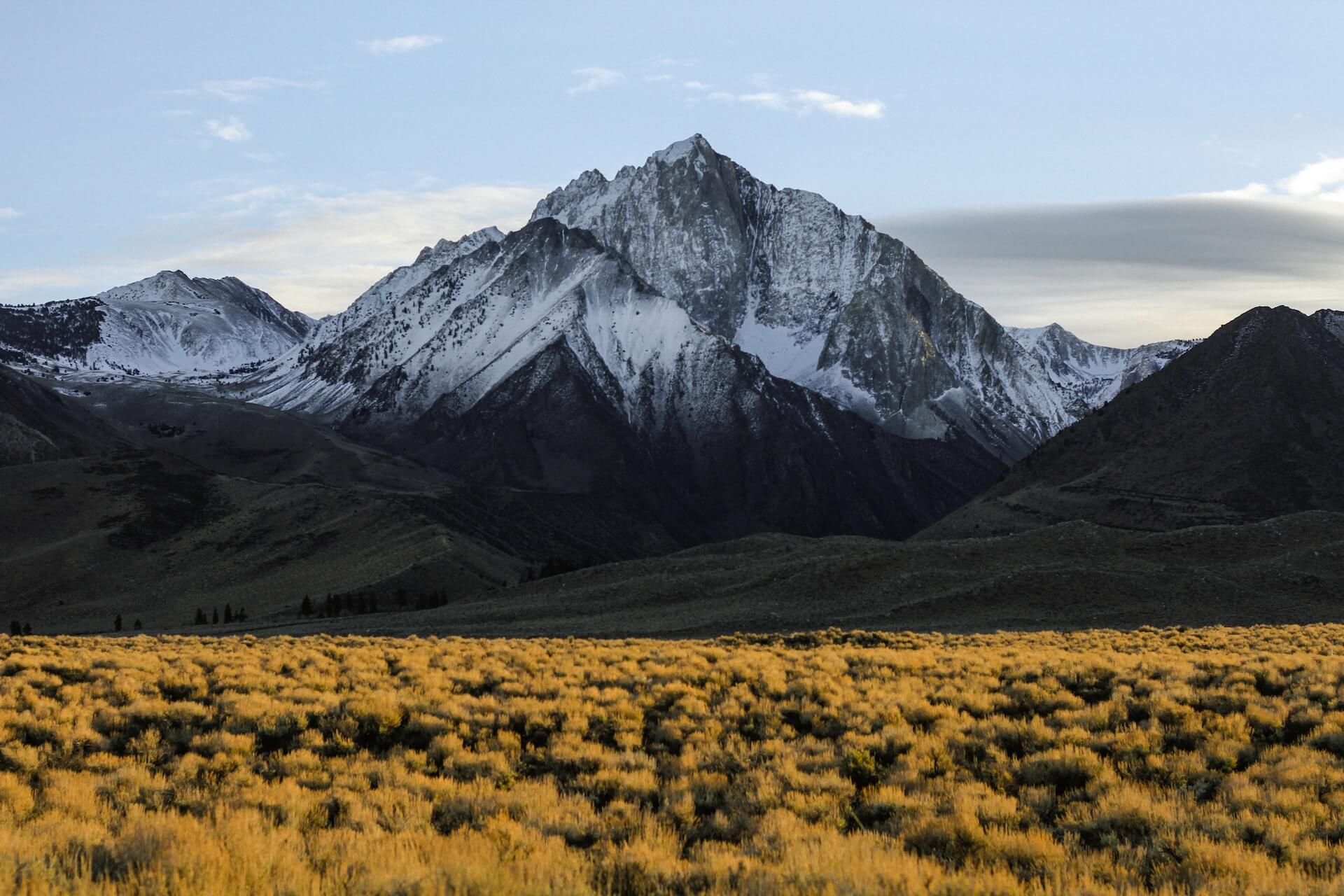
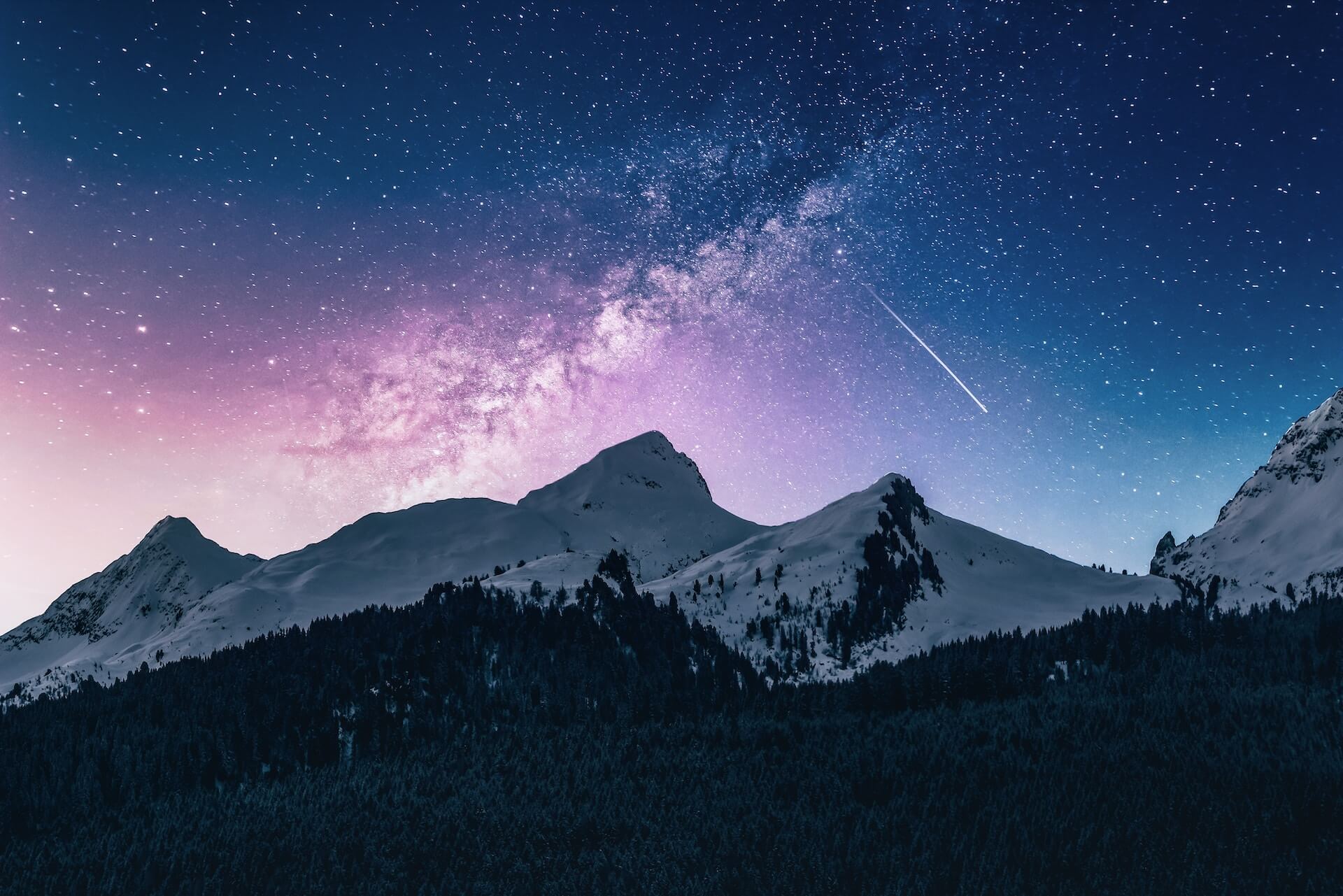
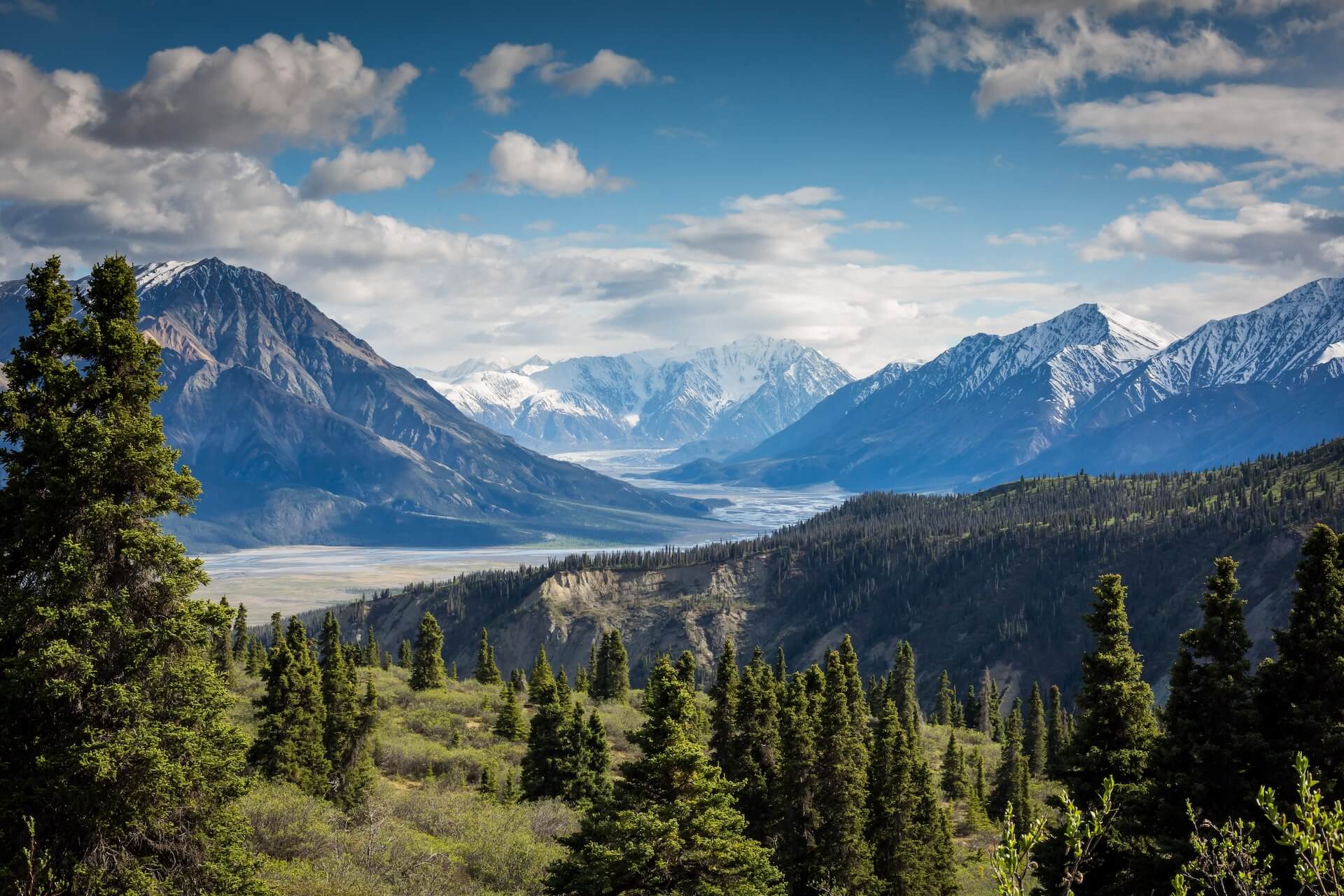
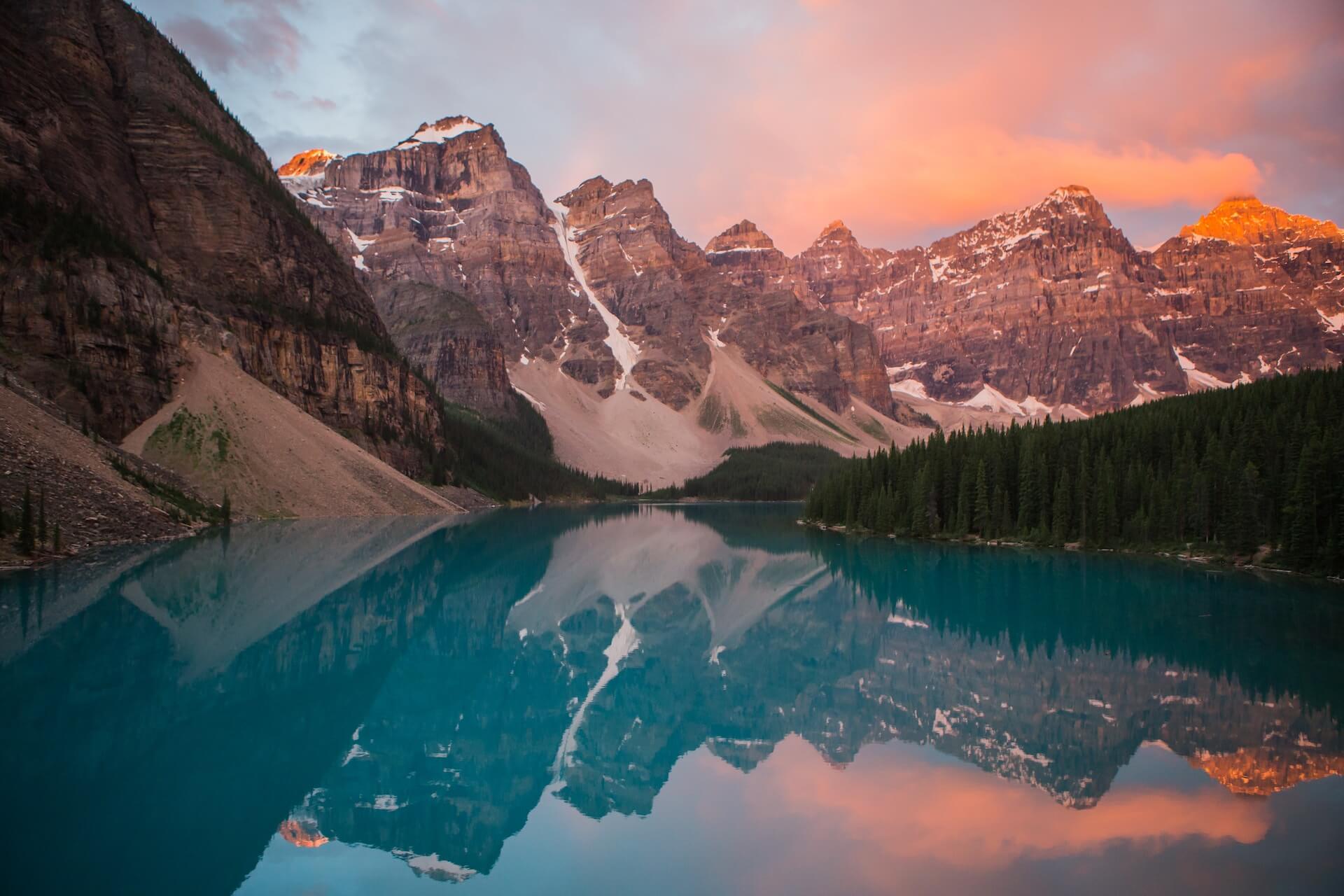
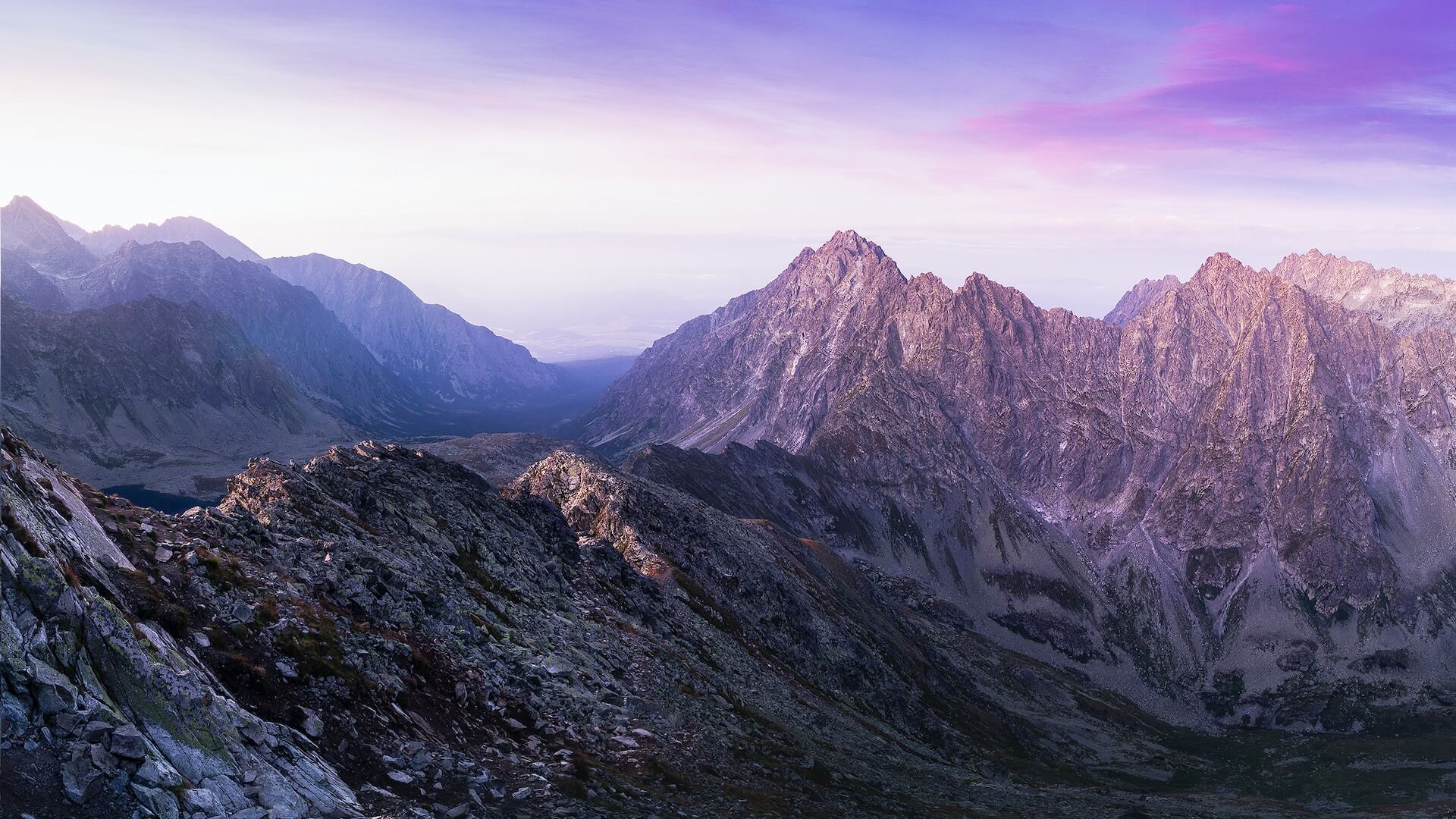
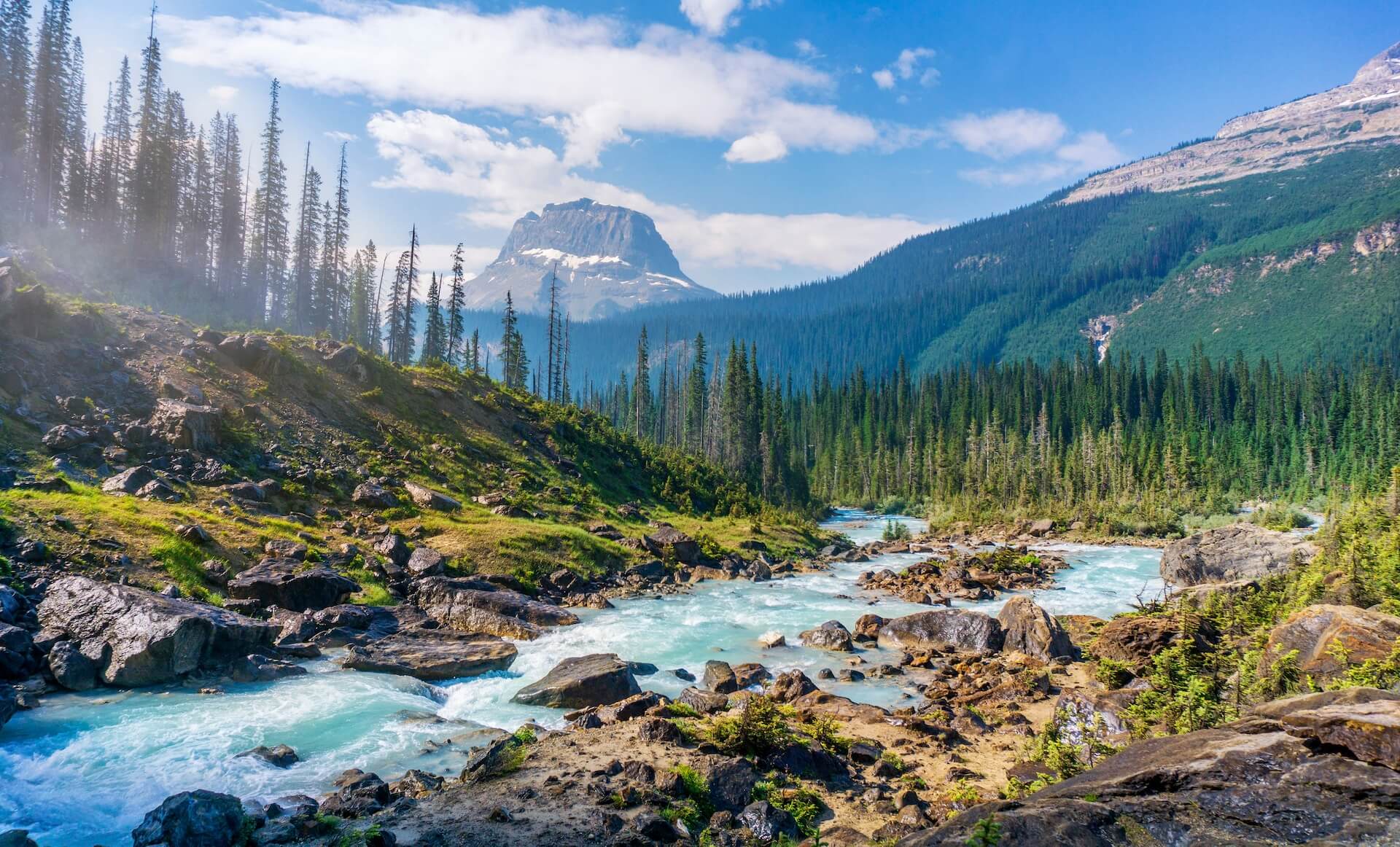
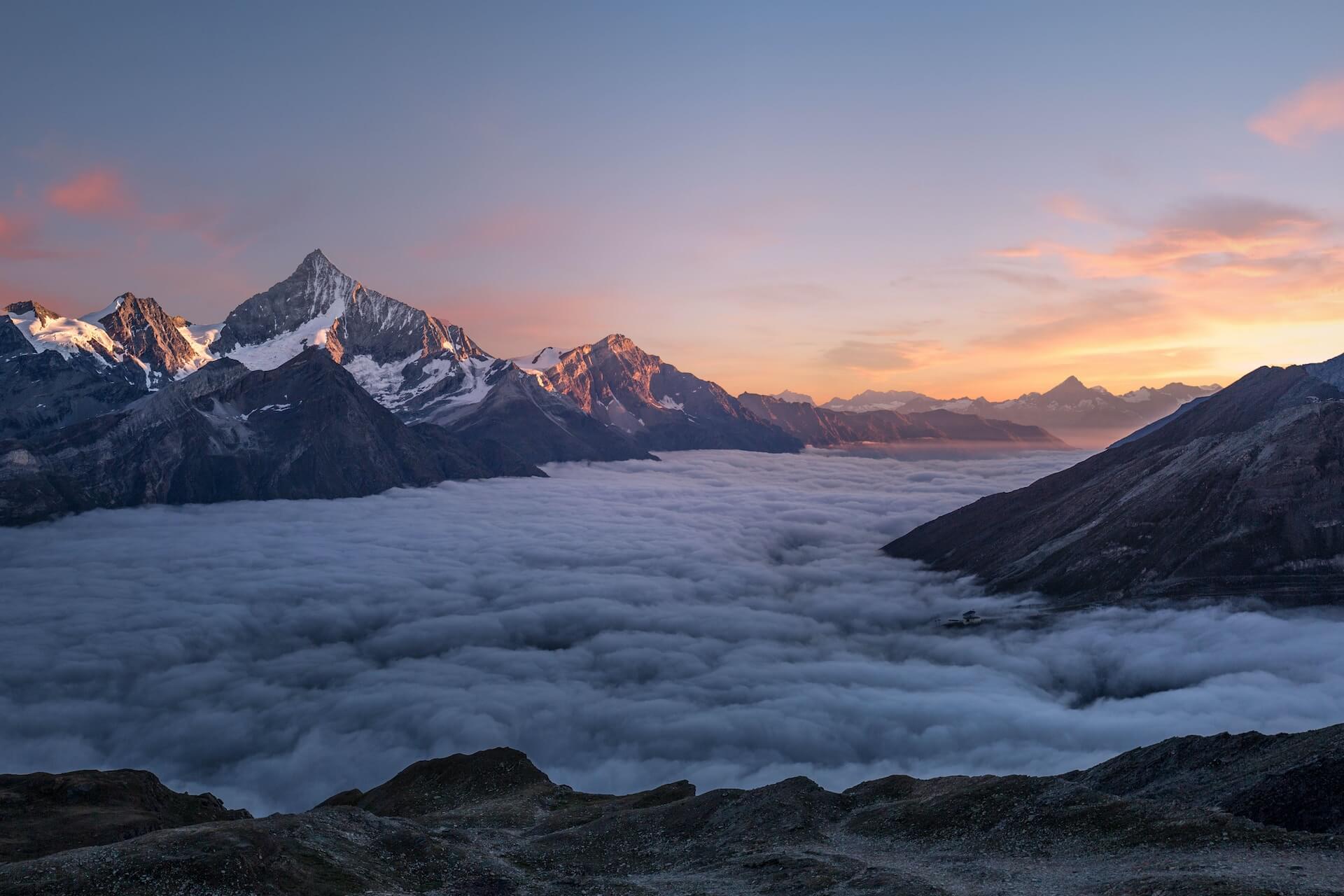
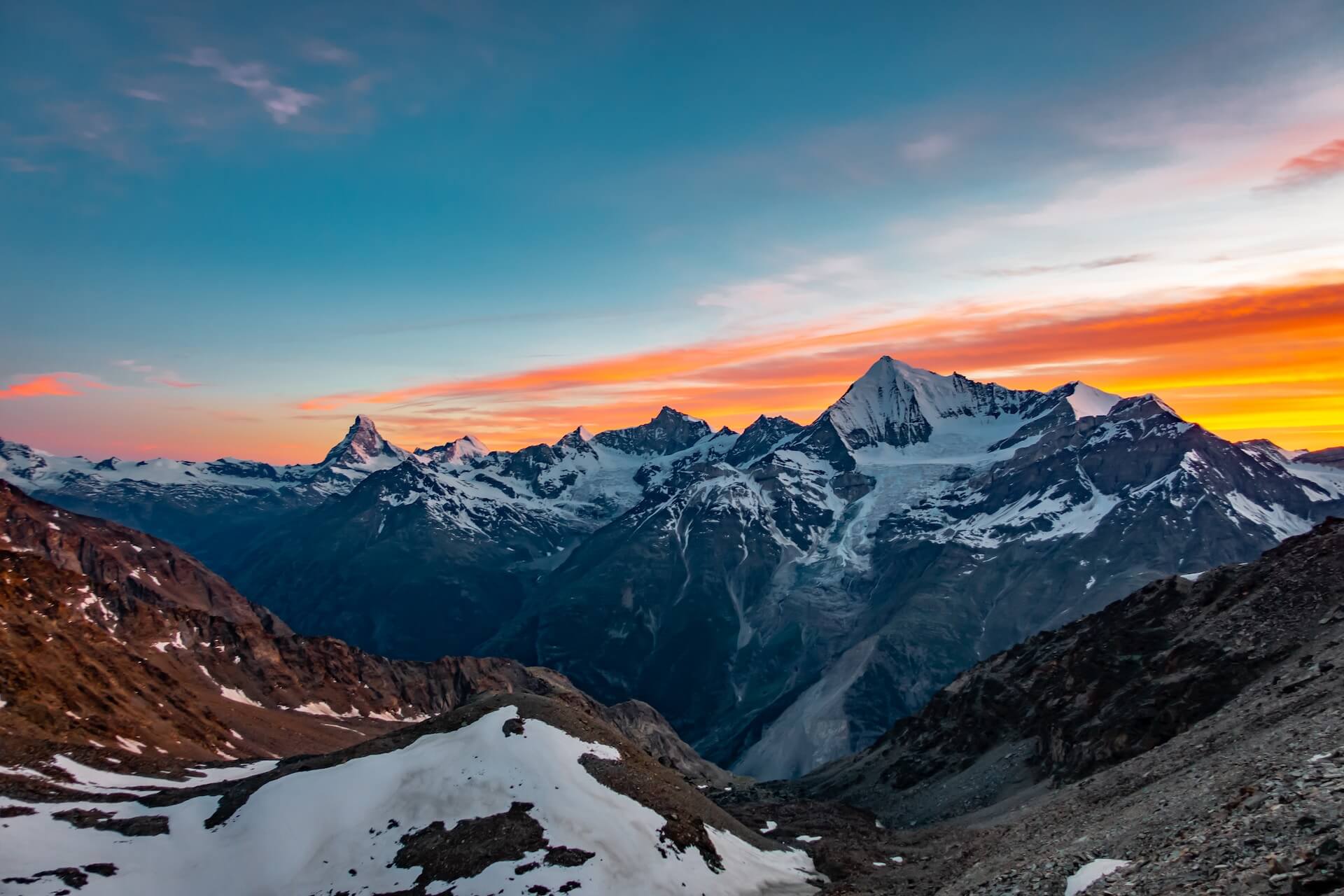
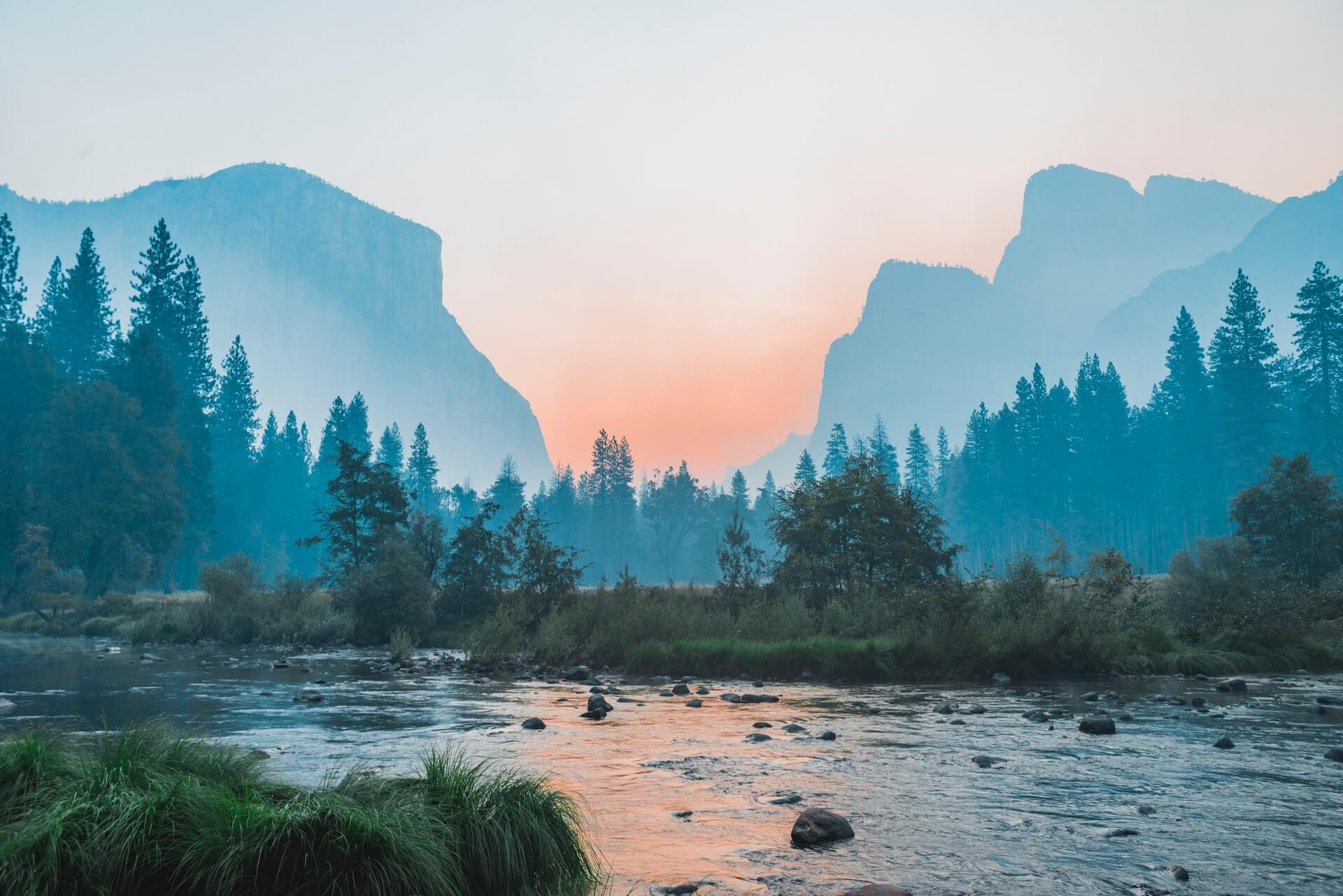
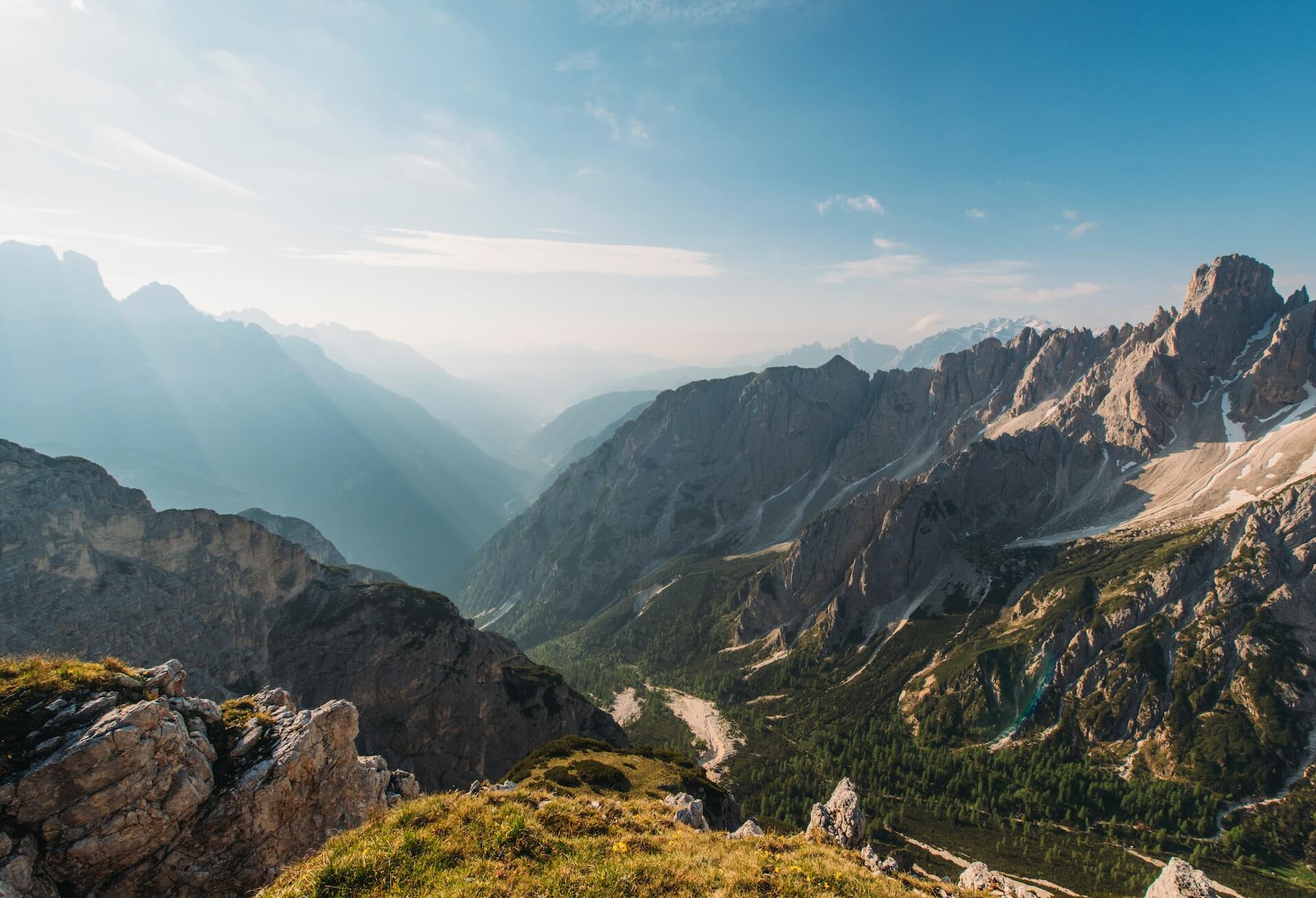
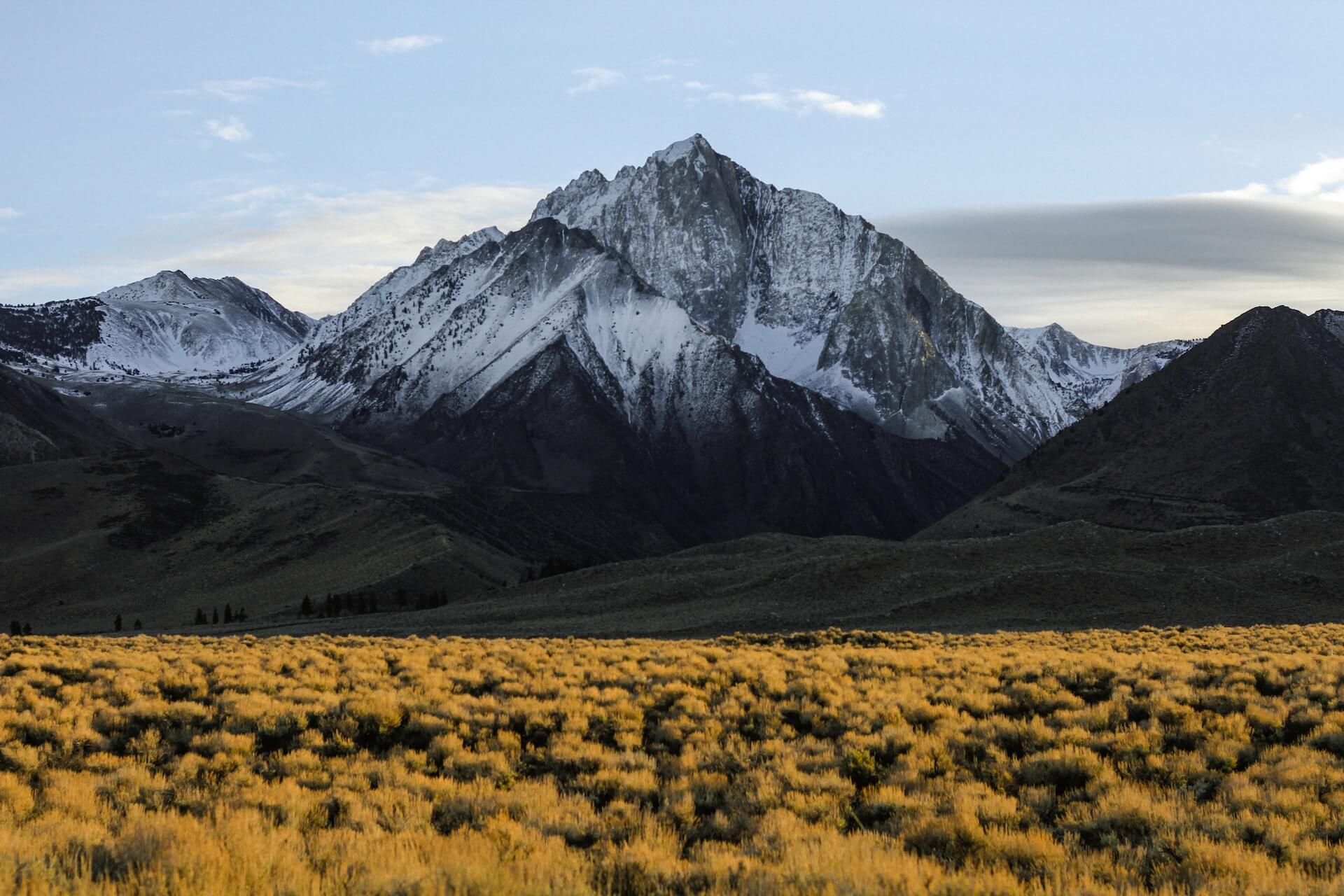
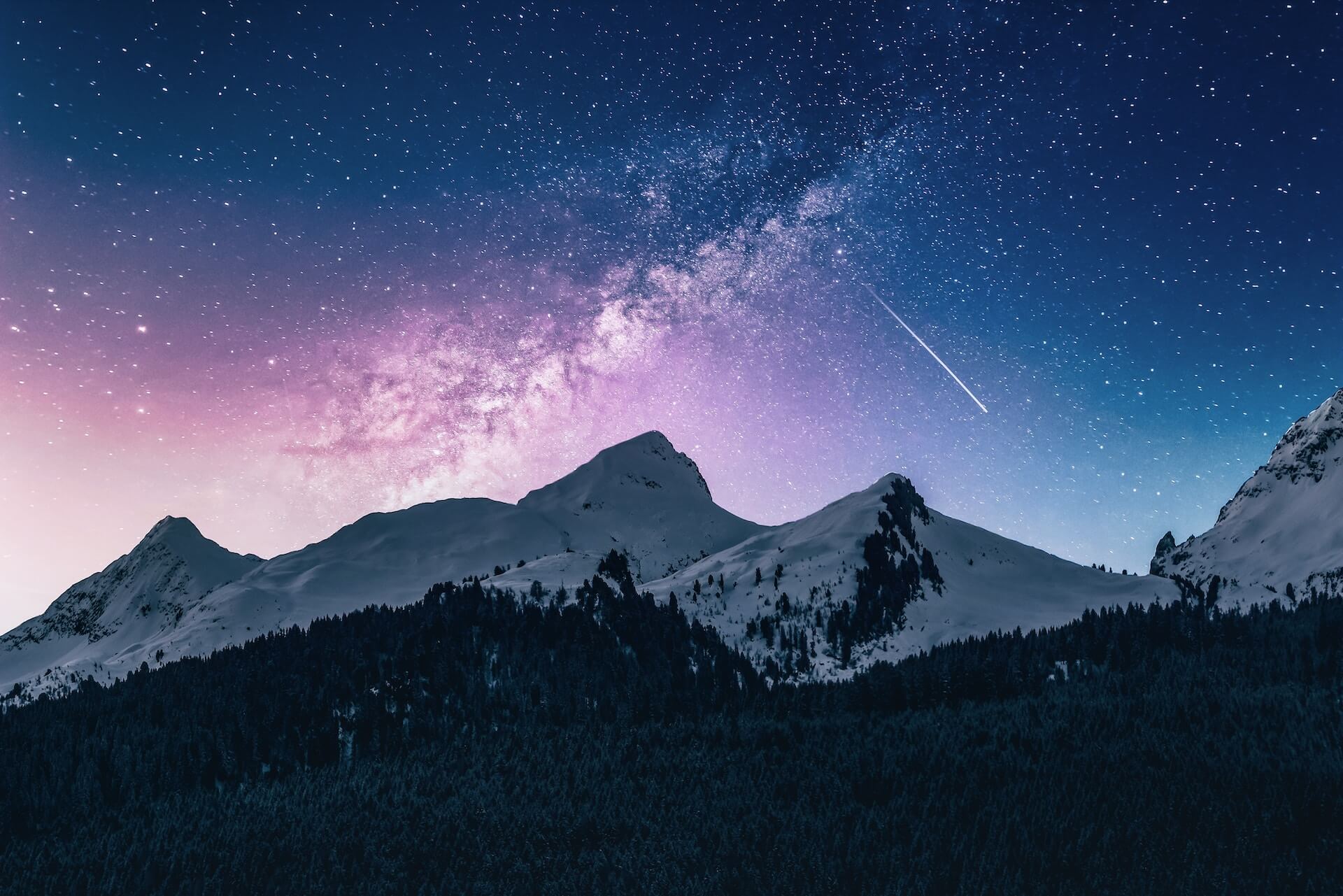
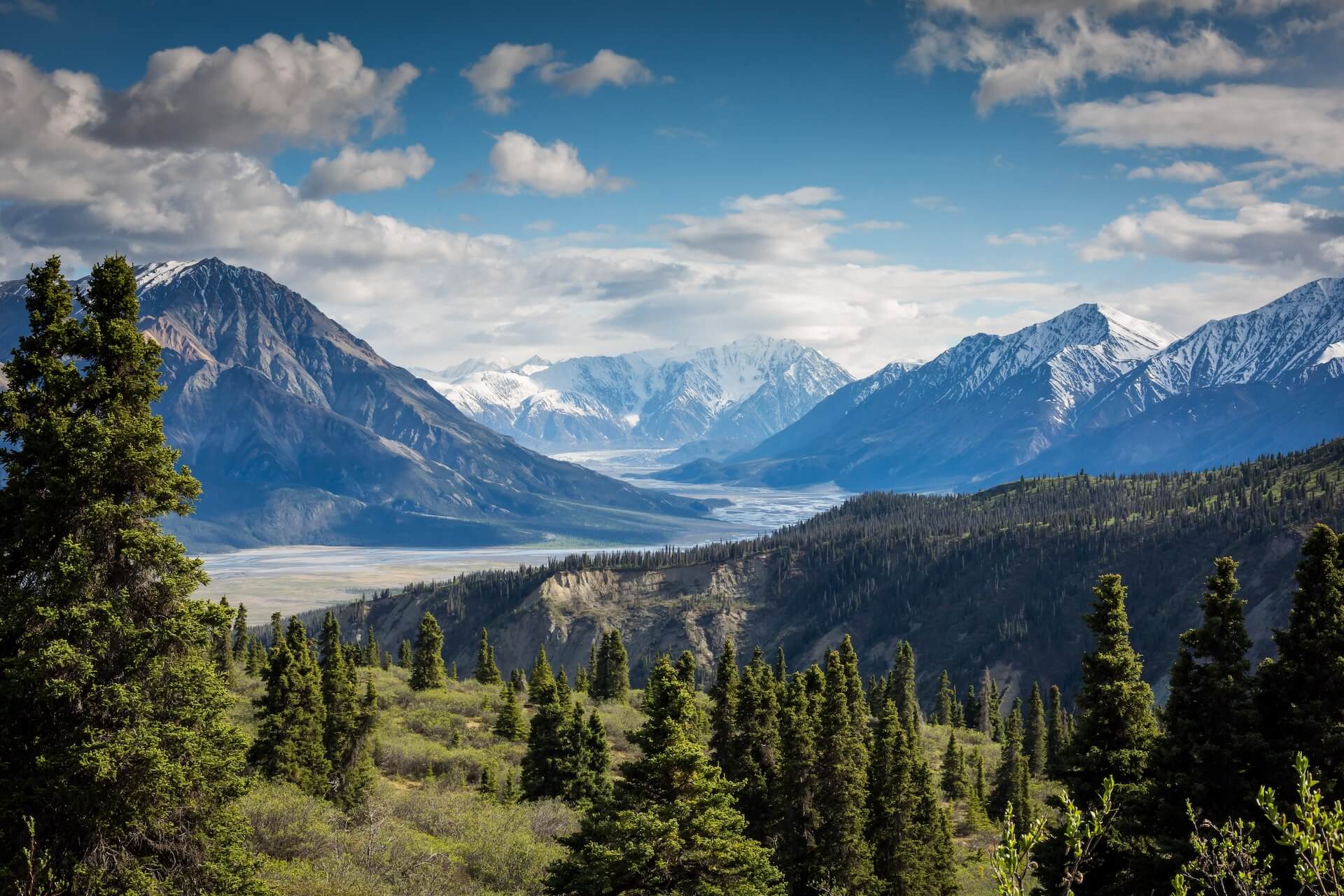
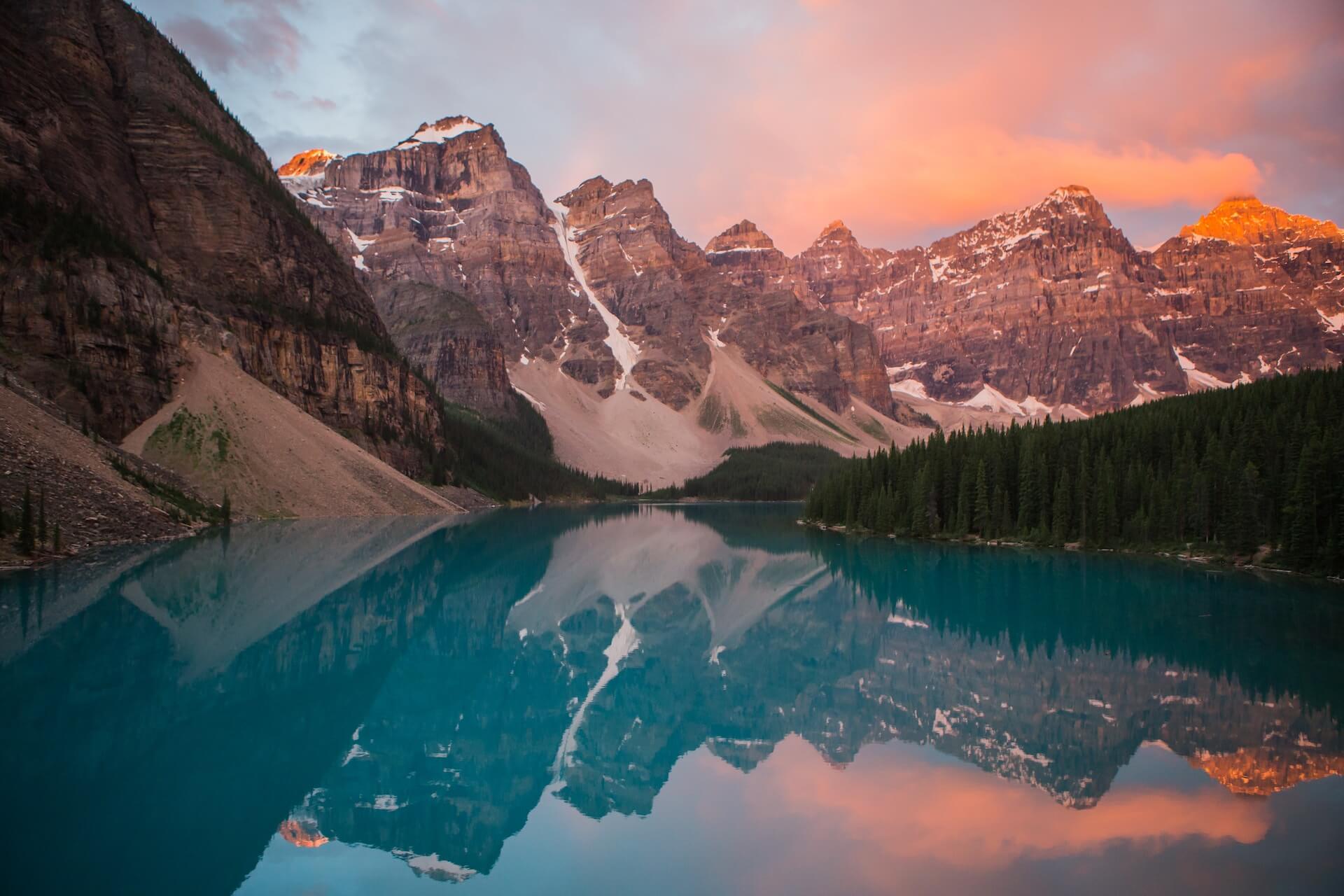
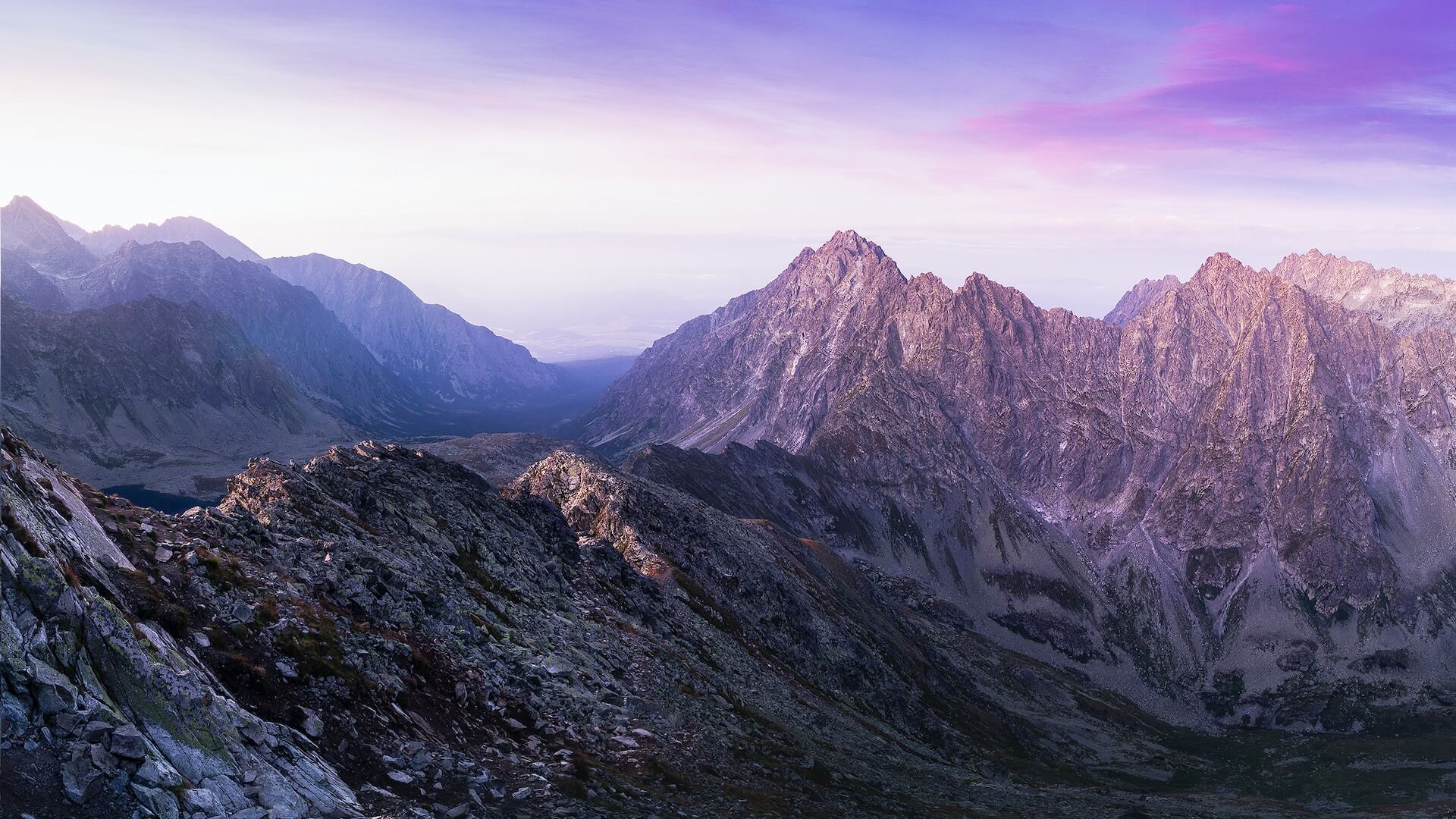
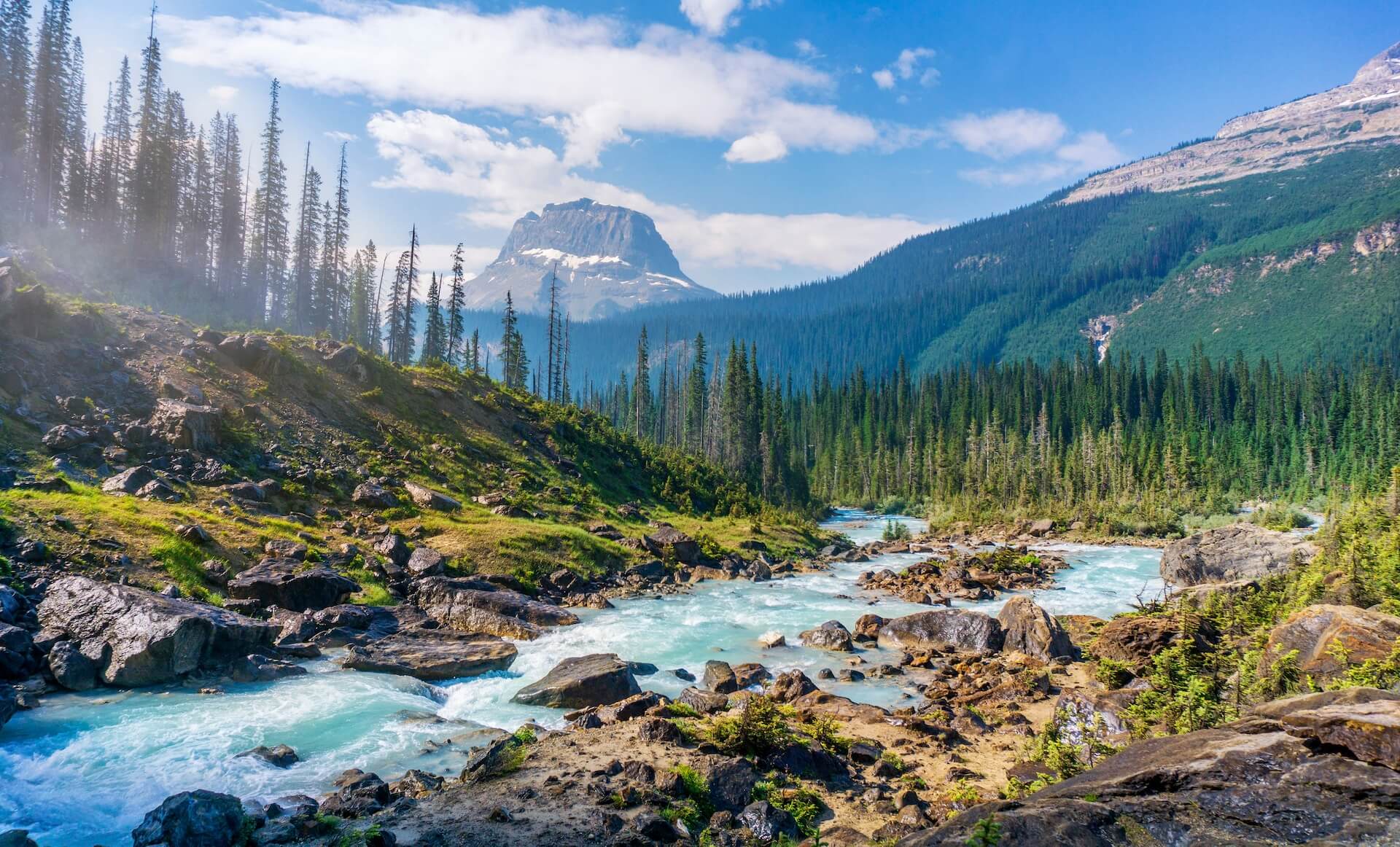
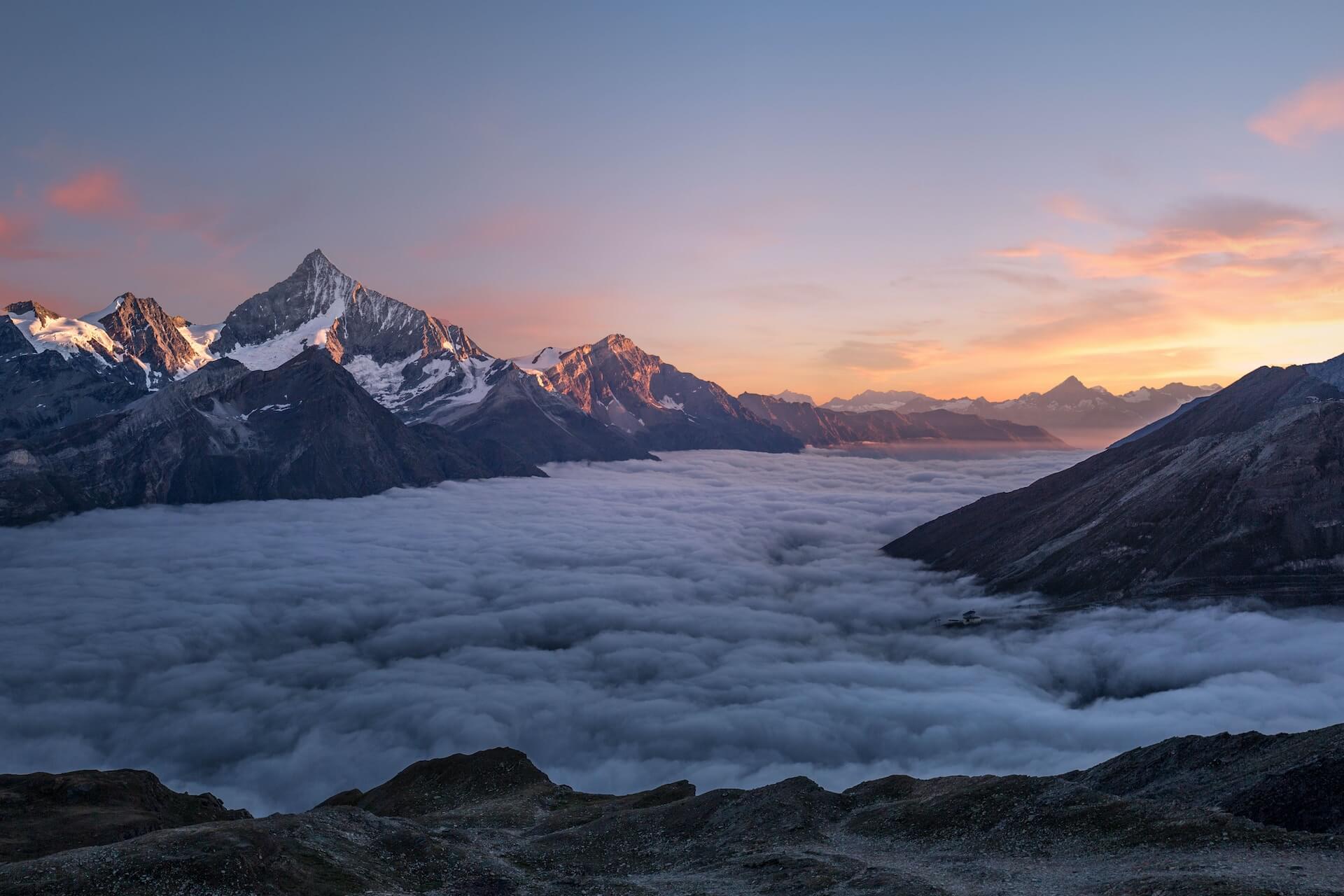
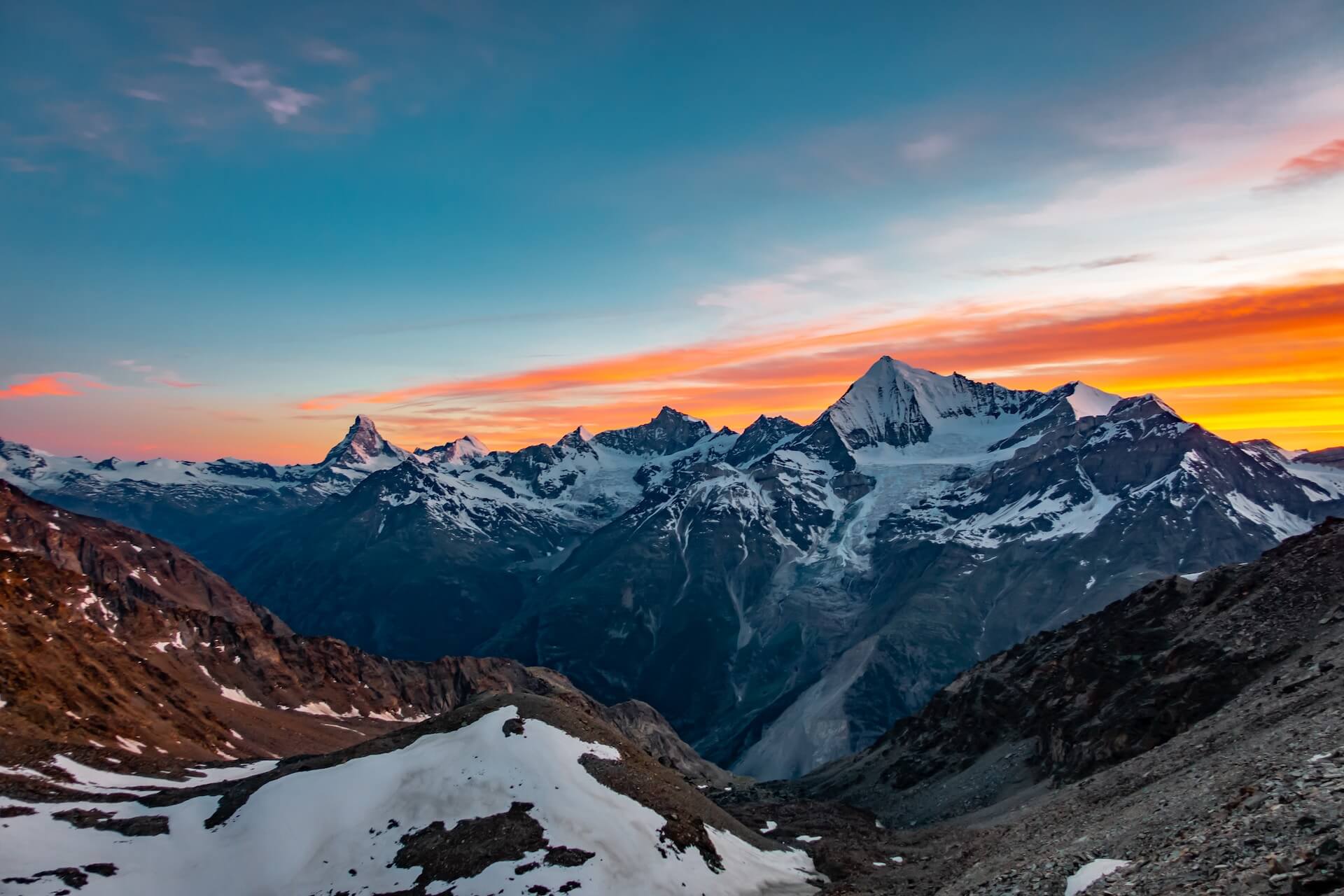
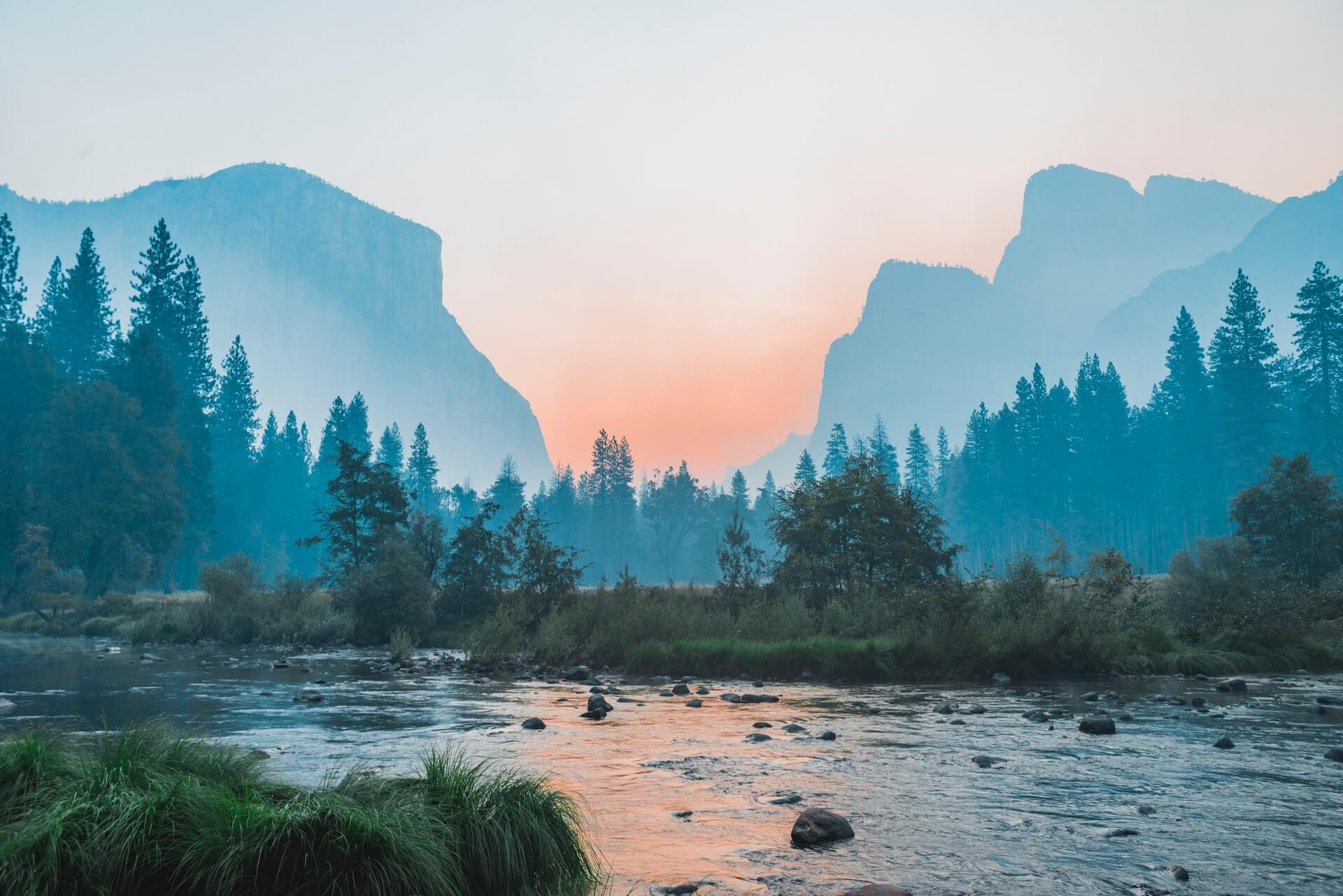
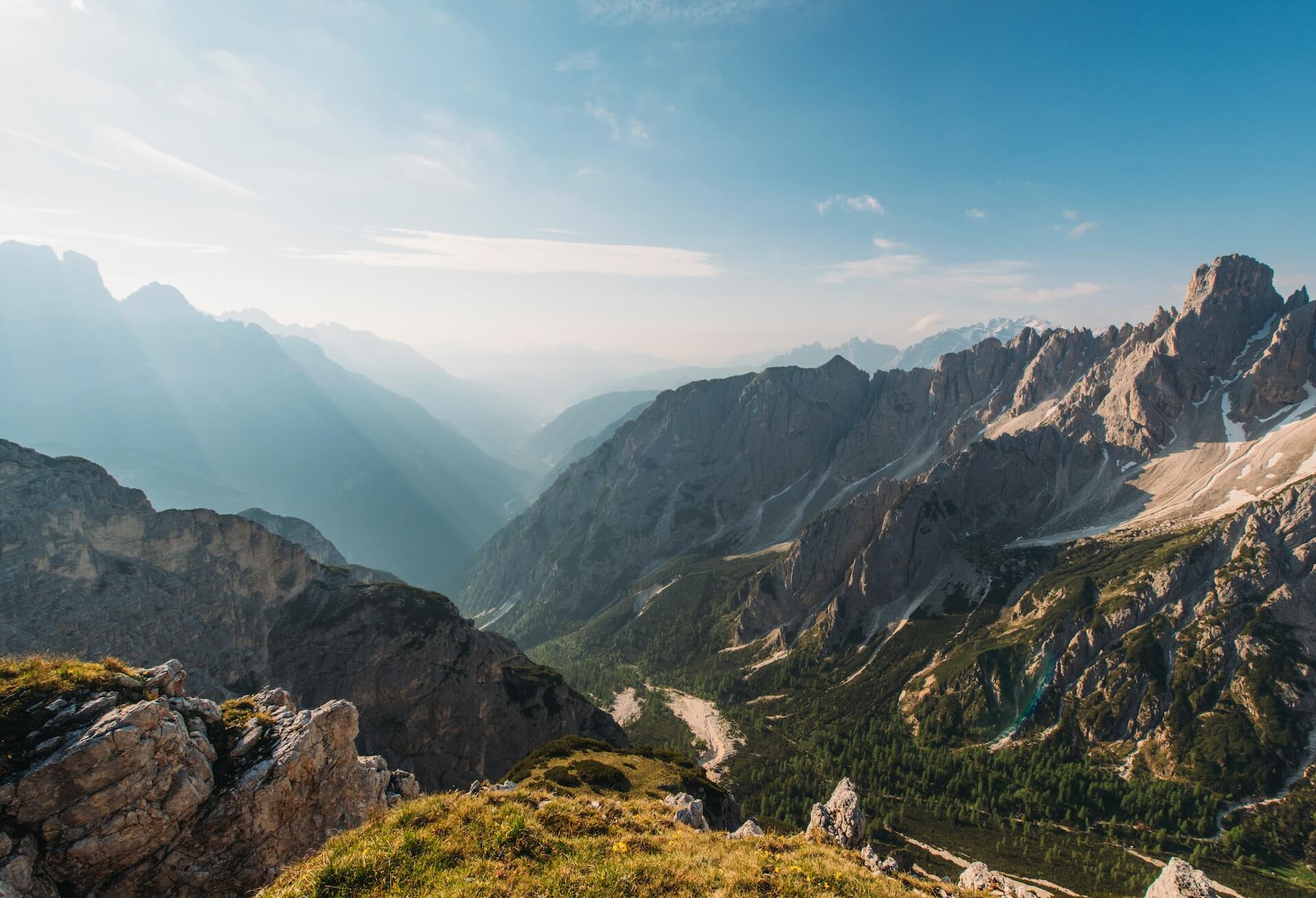
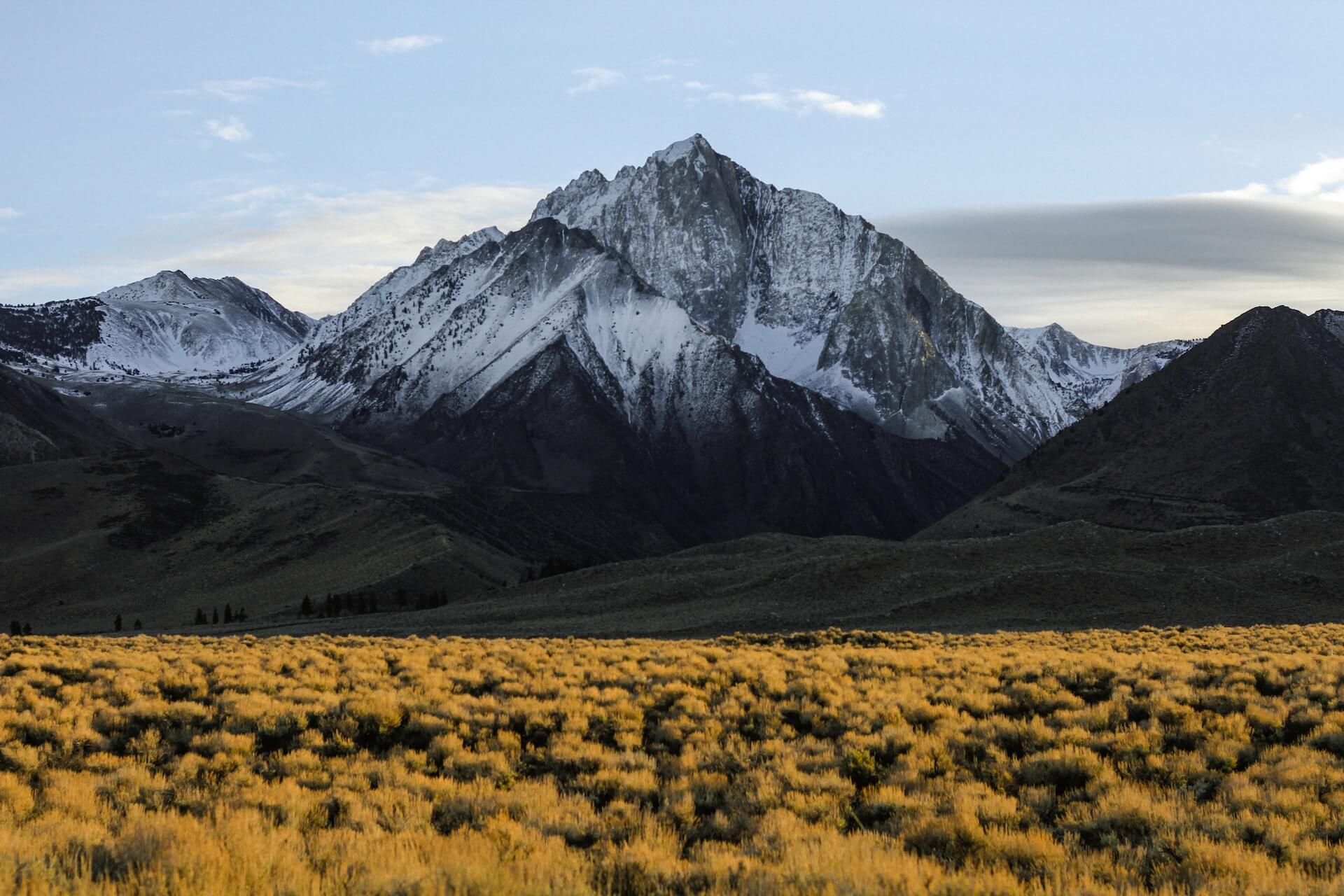
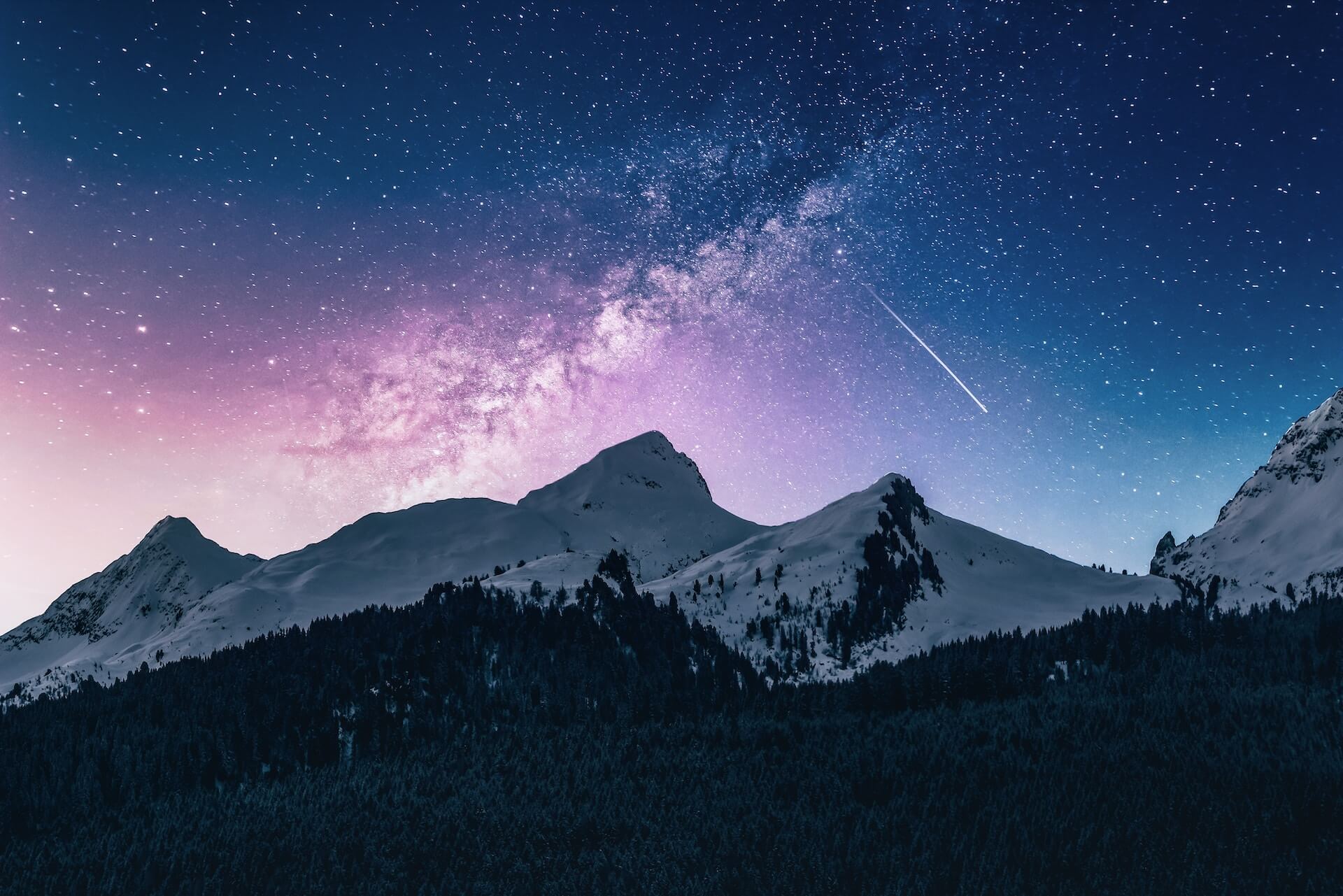
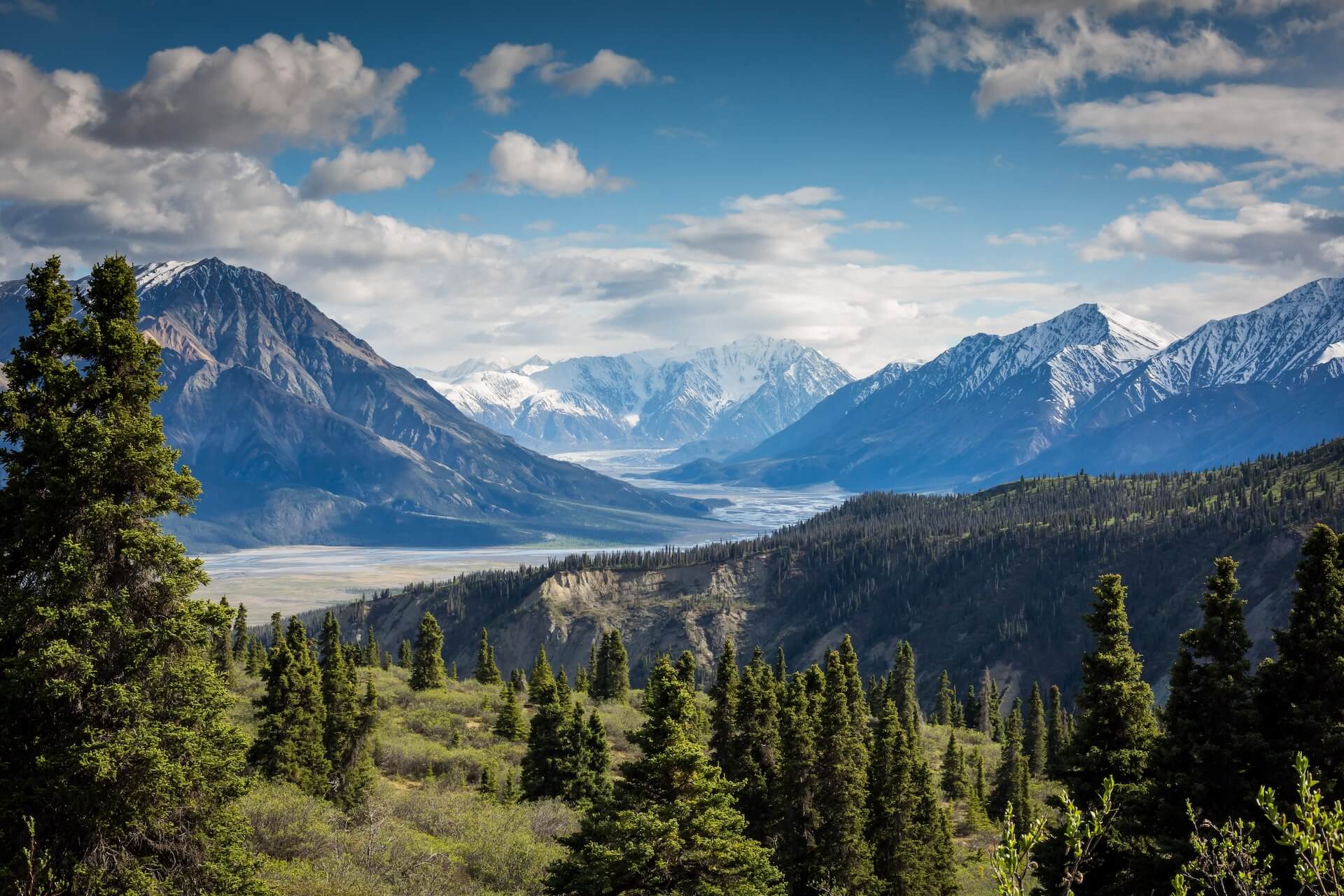
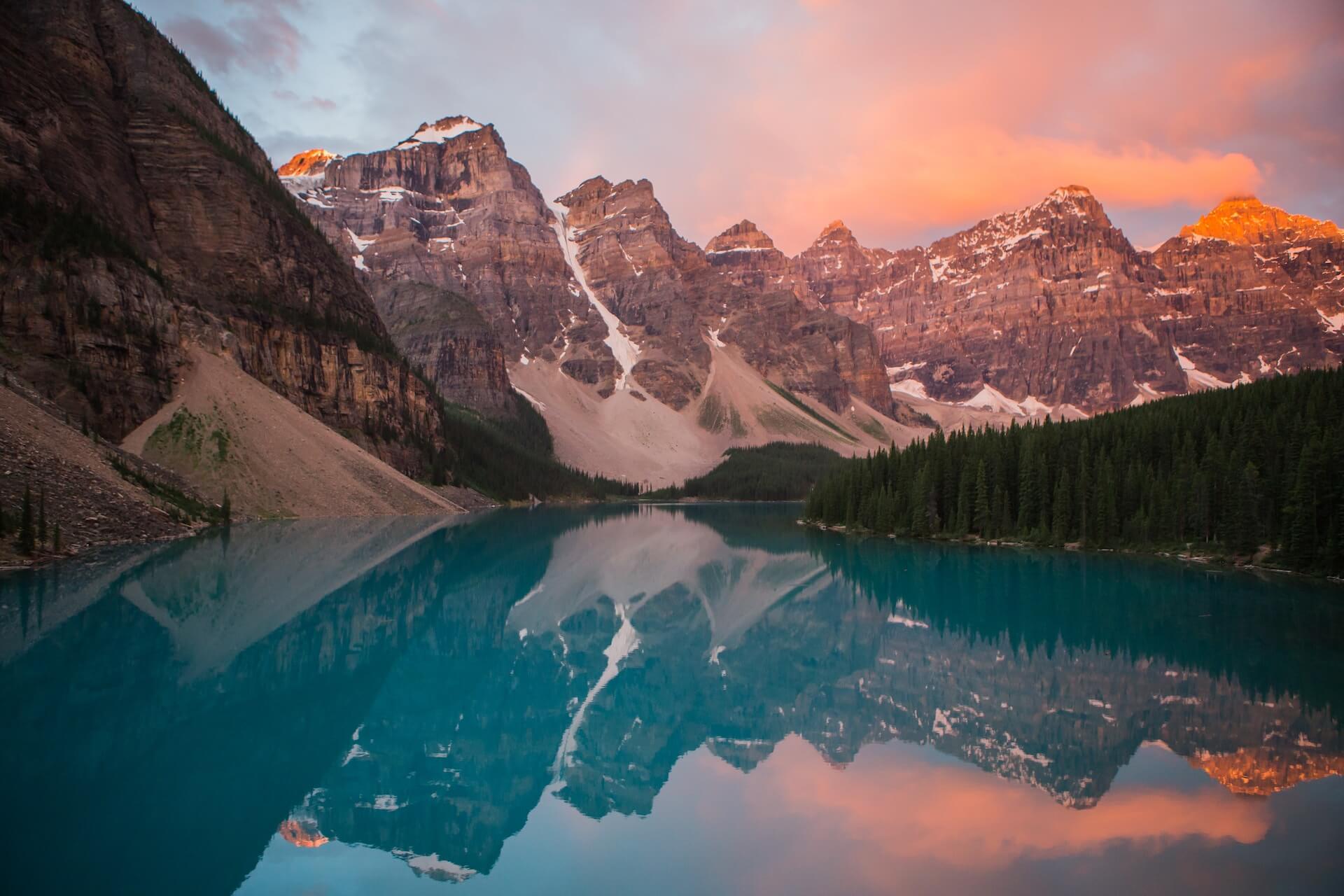
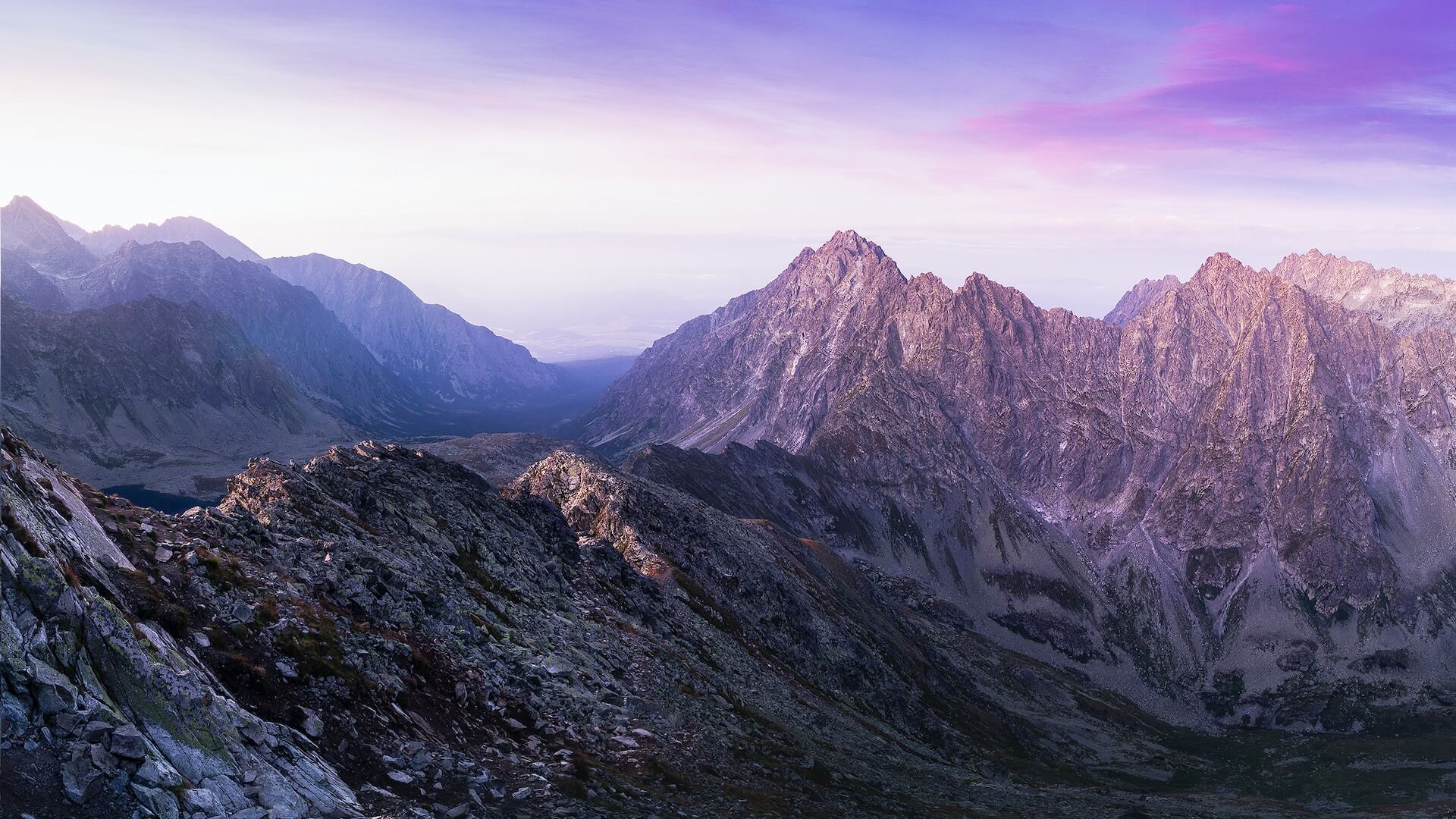
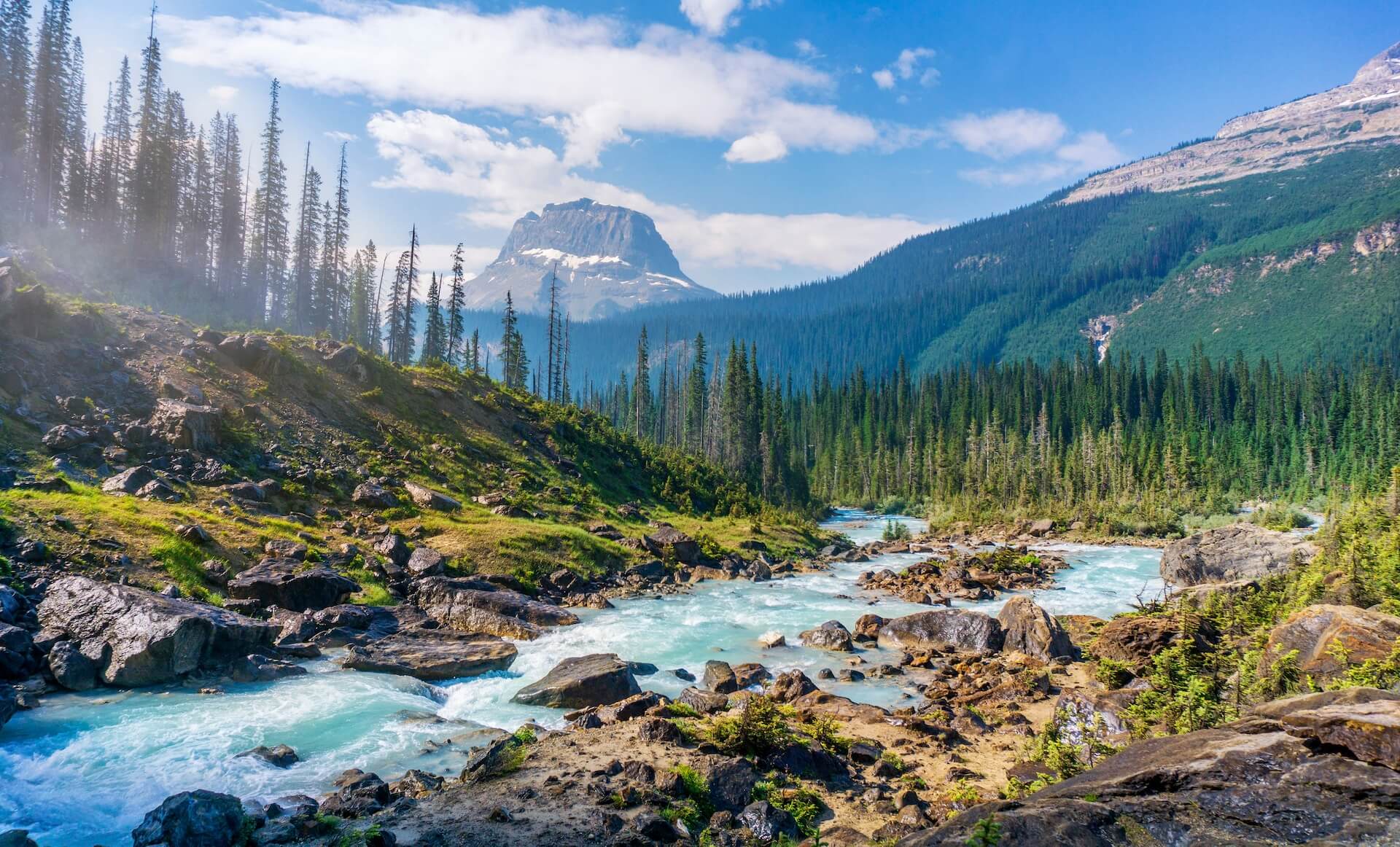
Copied !
import { CarouselGallery } from "$components/gallery.component";
/**
* @type {import("$common/props").JSXComponent<{ images: import("$components/image.component").ImageType[]}>}
*/
export function DefaultCarouselGalleryExample(props) {
const { images } = props;
return (
<div class="bg-slate-200 p-4 flex flex-col gap-y-8 relative w-full">
<CarouselGallery images={images} />
<CarouselGallery direction="vertical" images={images} />
<CarouselGallery thumbnailWidth="200px" images={images} />
</div>
);
}
Zoom Gallery
Because it's a well-known feature, gallery has zoom props which, you already guess, provides a zoom of the clicked image.
See these two examples below respectively about rest and carousel galleries.
- +
Copied !
import { RestGallery } from "$components/gallery.component";
/**
* @type {import("$common/props").JSXComponent<{ images: import("$components/image.component").ImageType[]}>}
*/
export function ZoomRestGalleryExample(props) {
const { images } = props;
return <RestGallery images={images} zoom />;
}
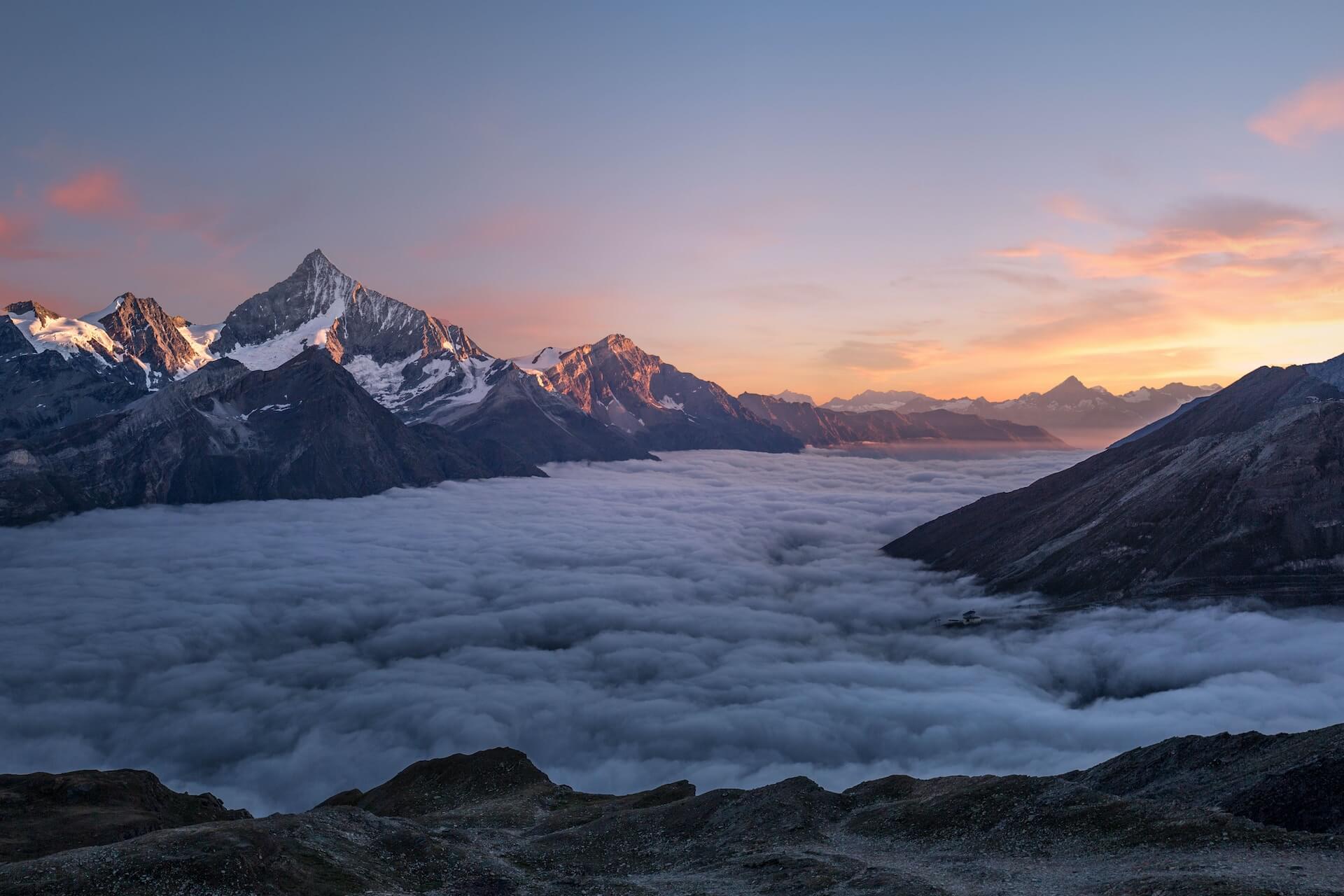
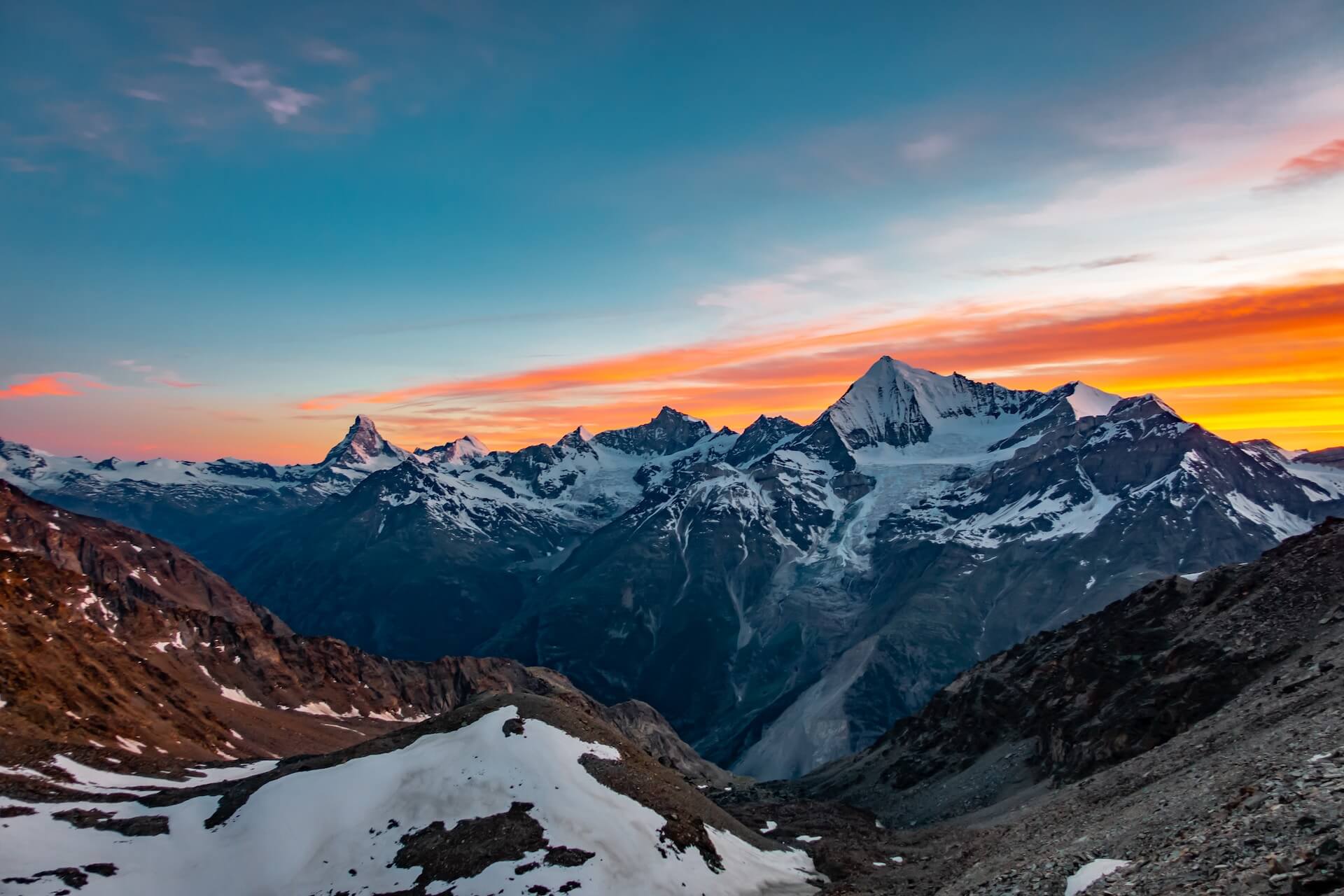
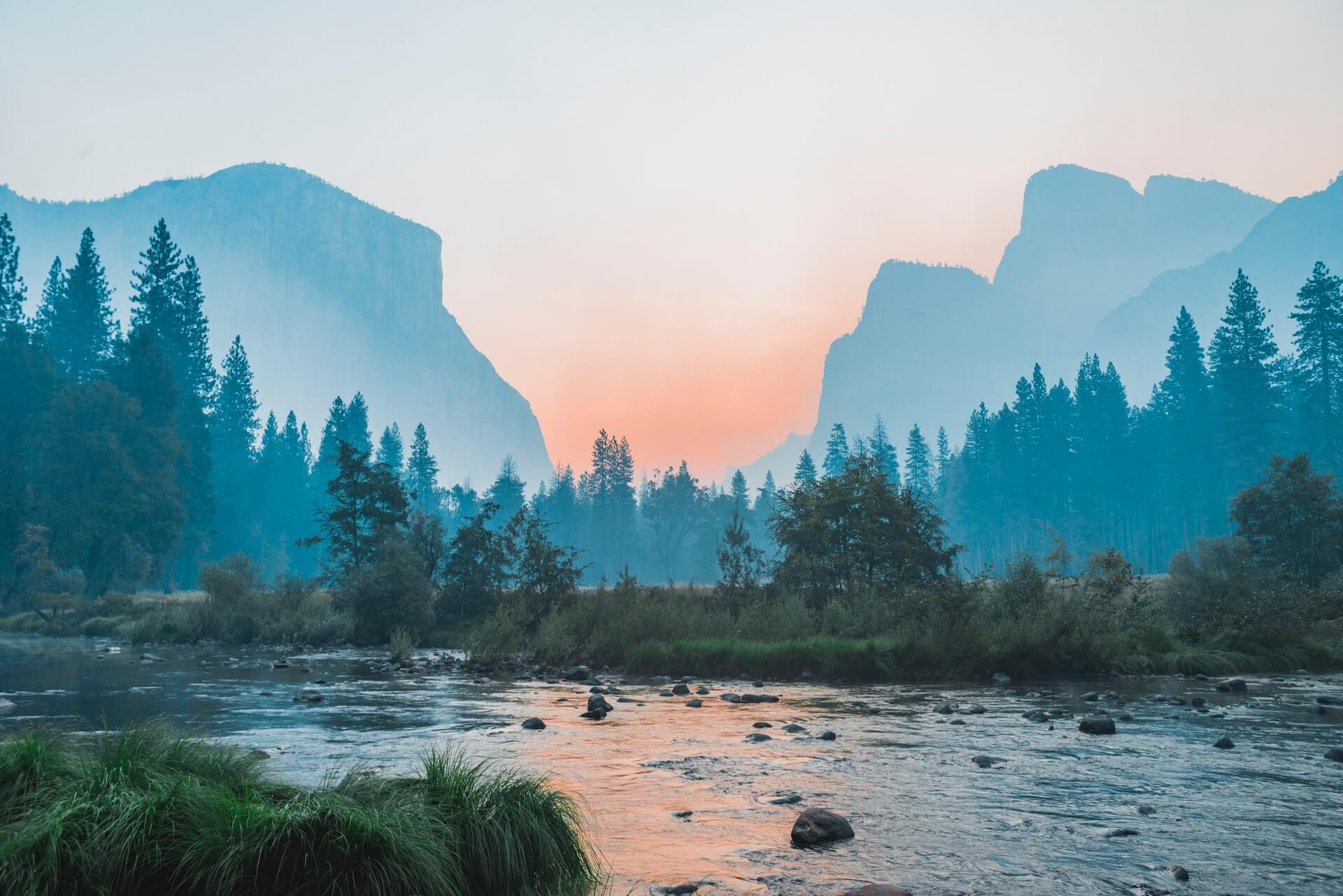
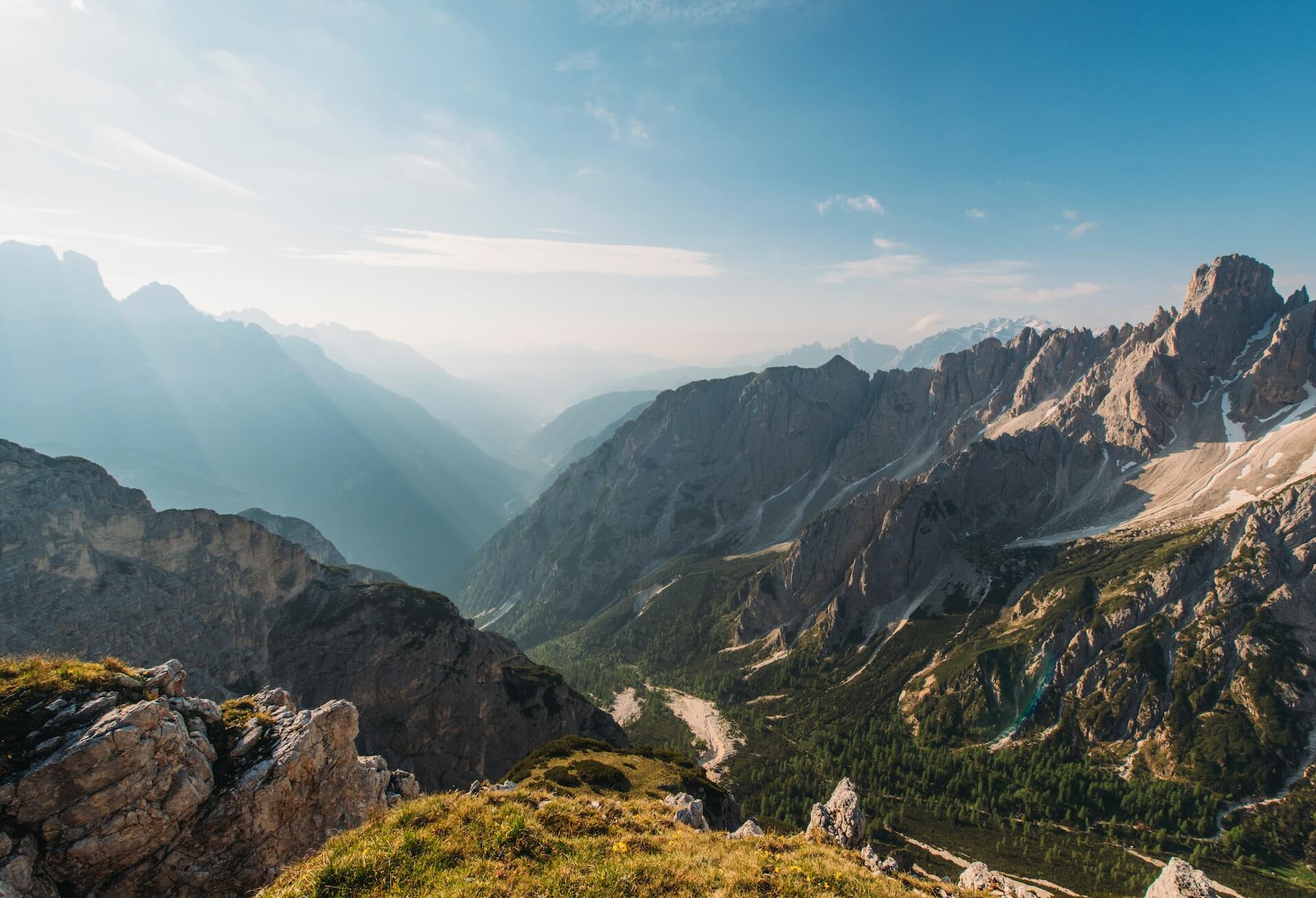
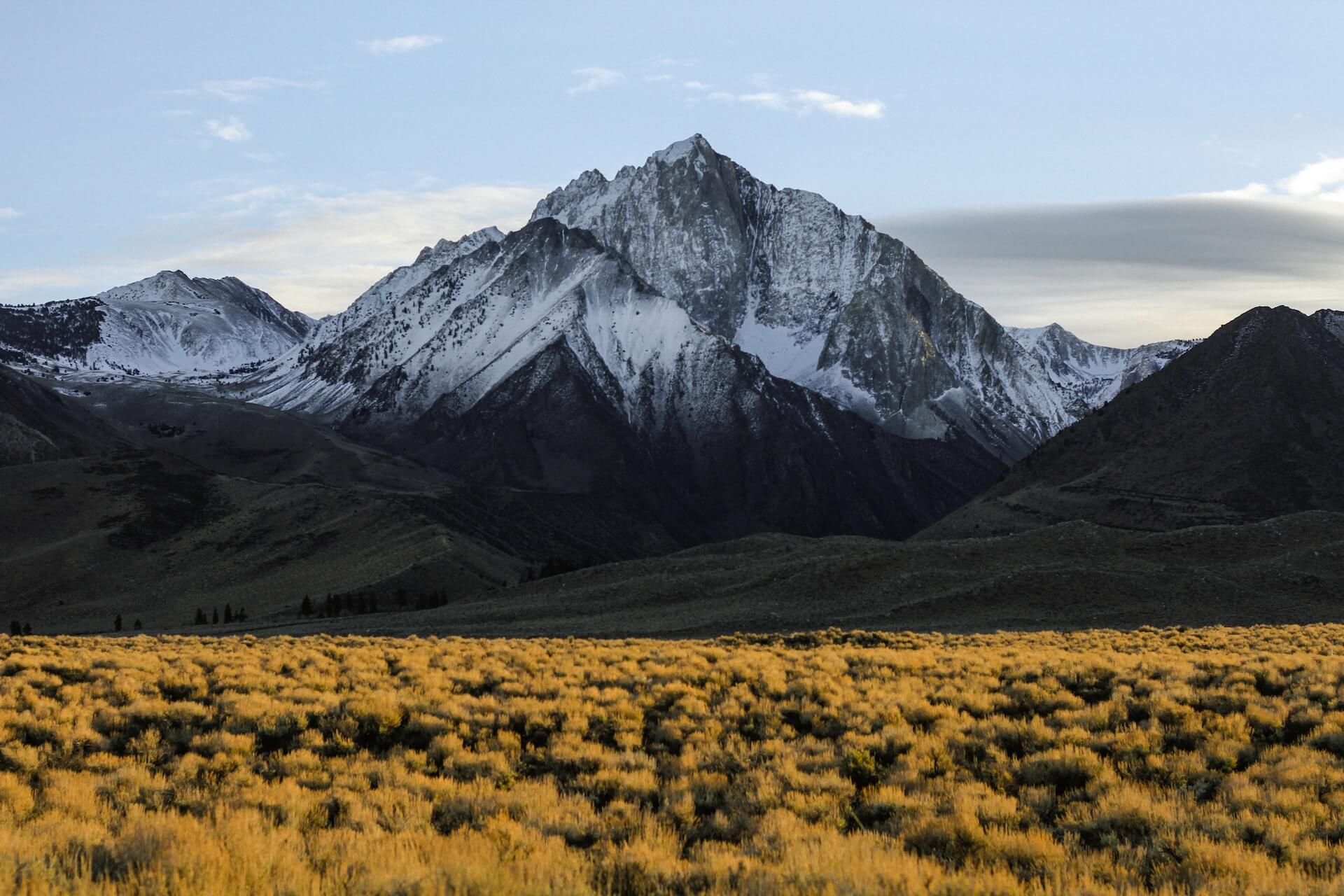
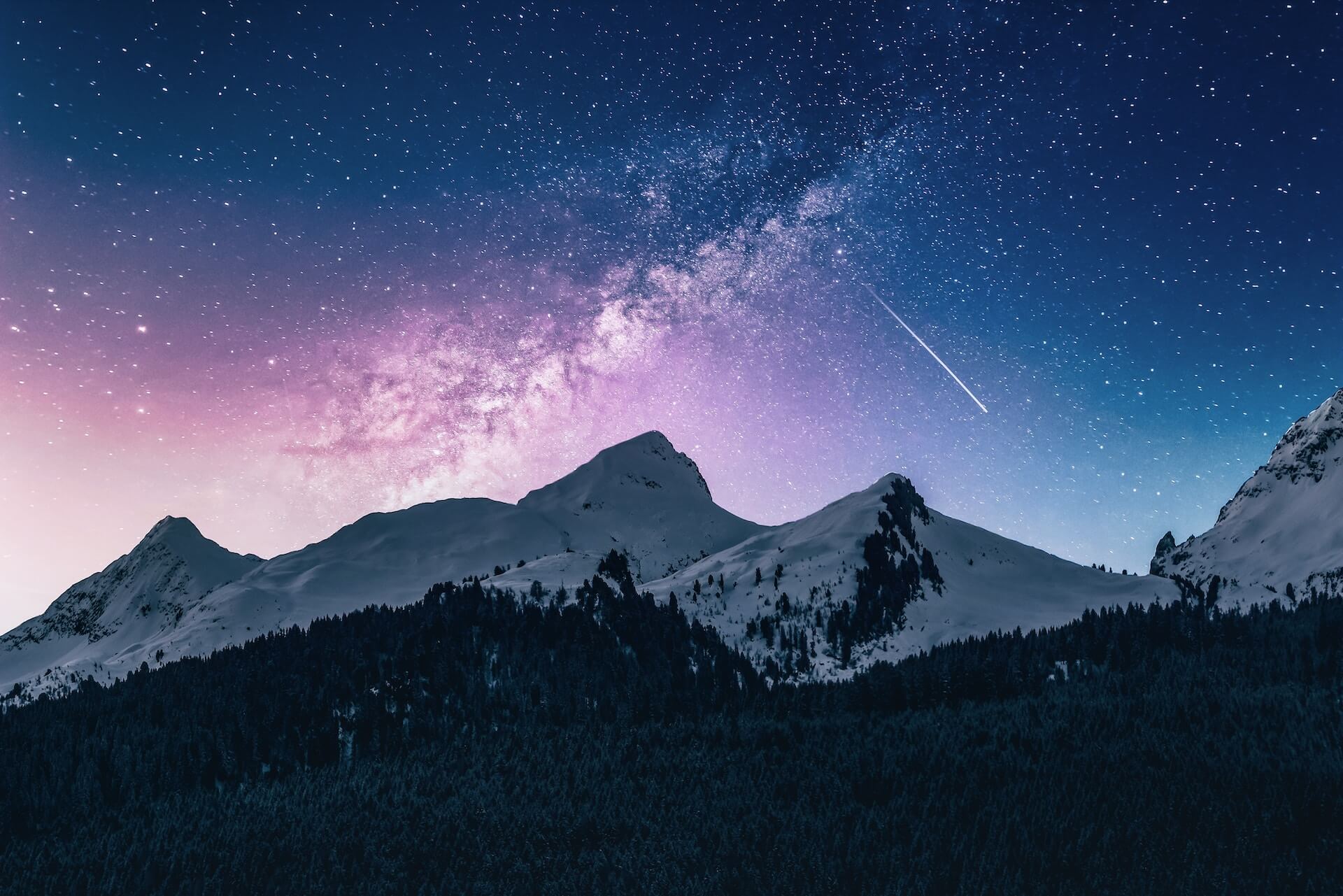
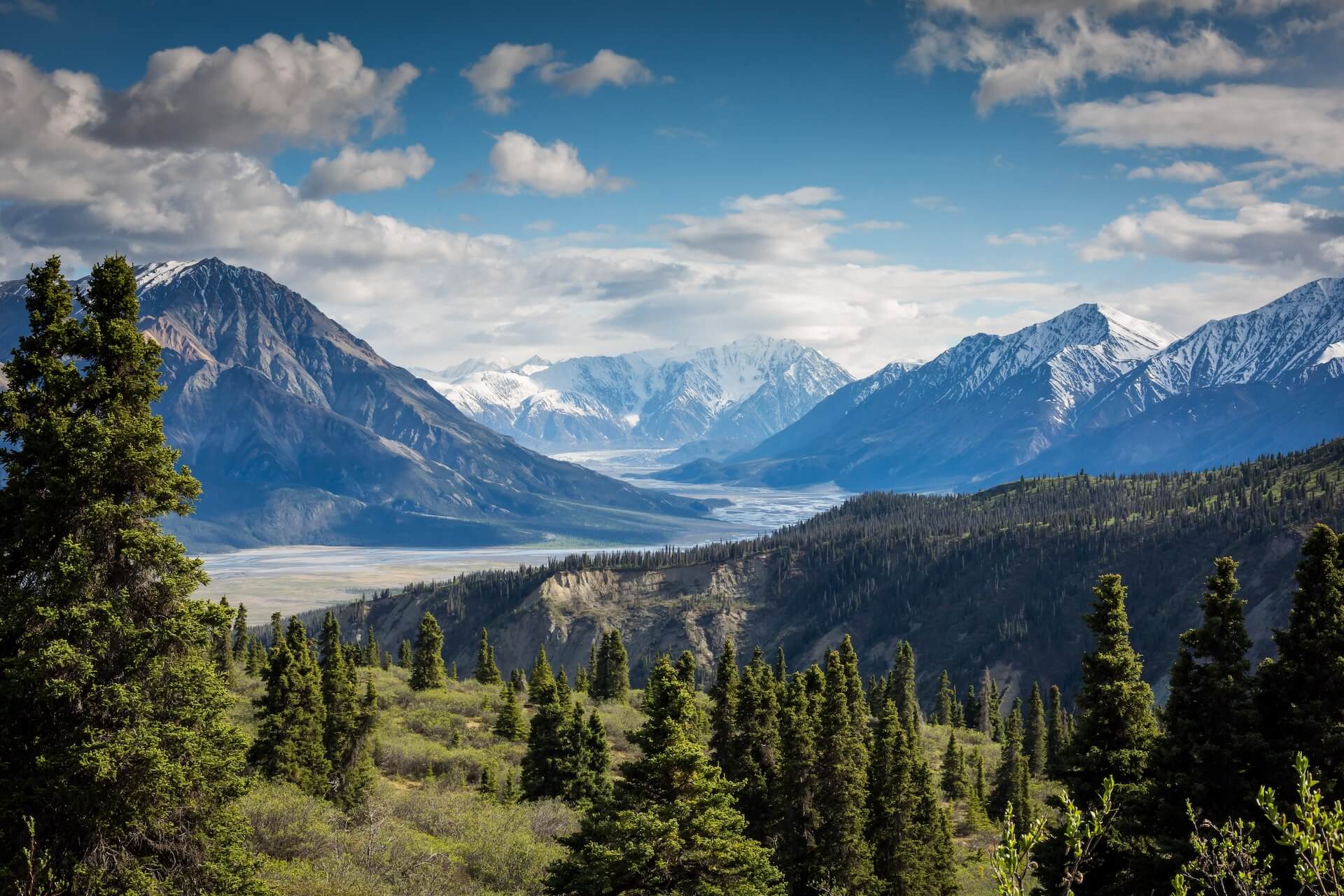
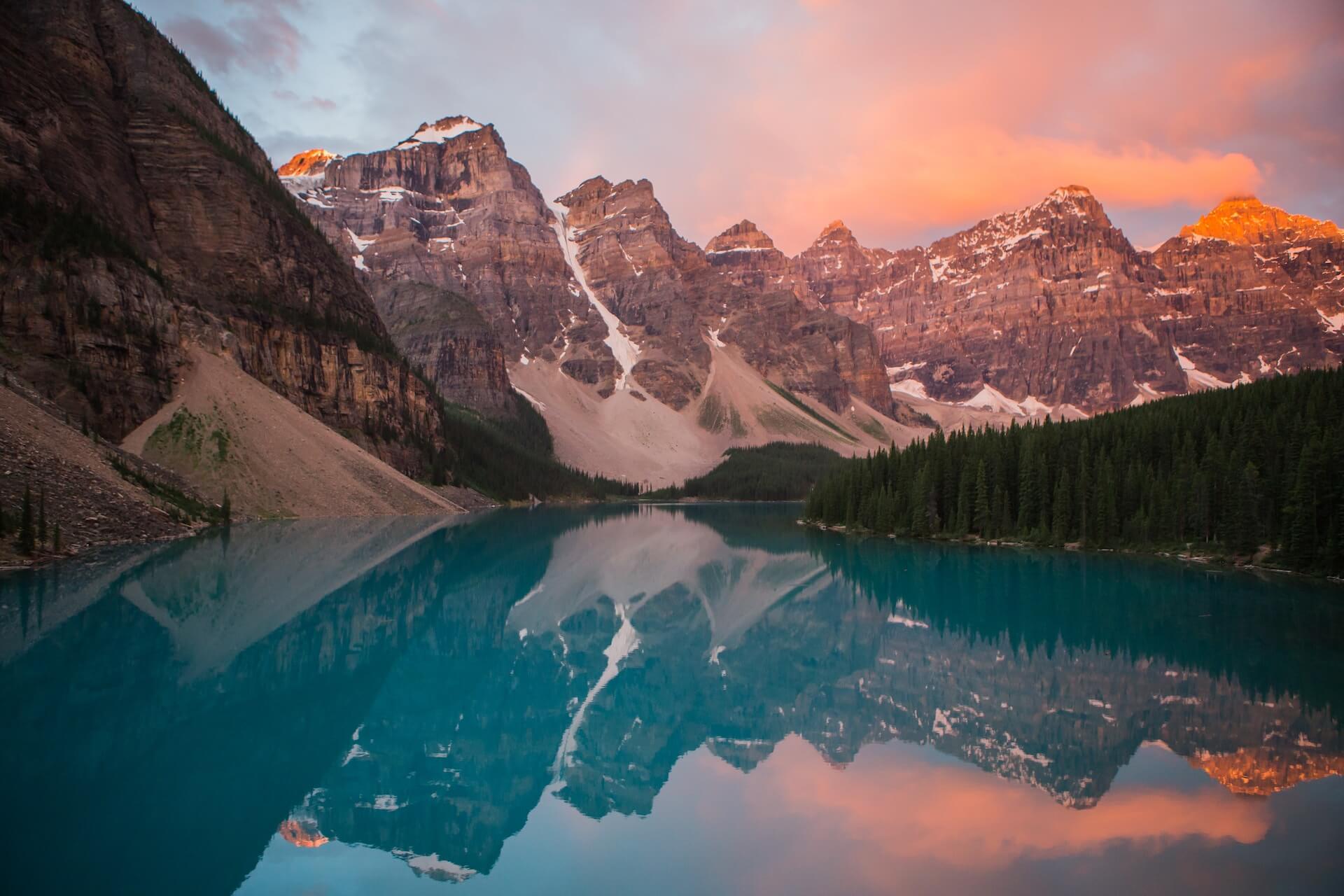
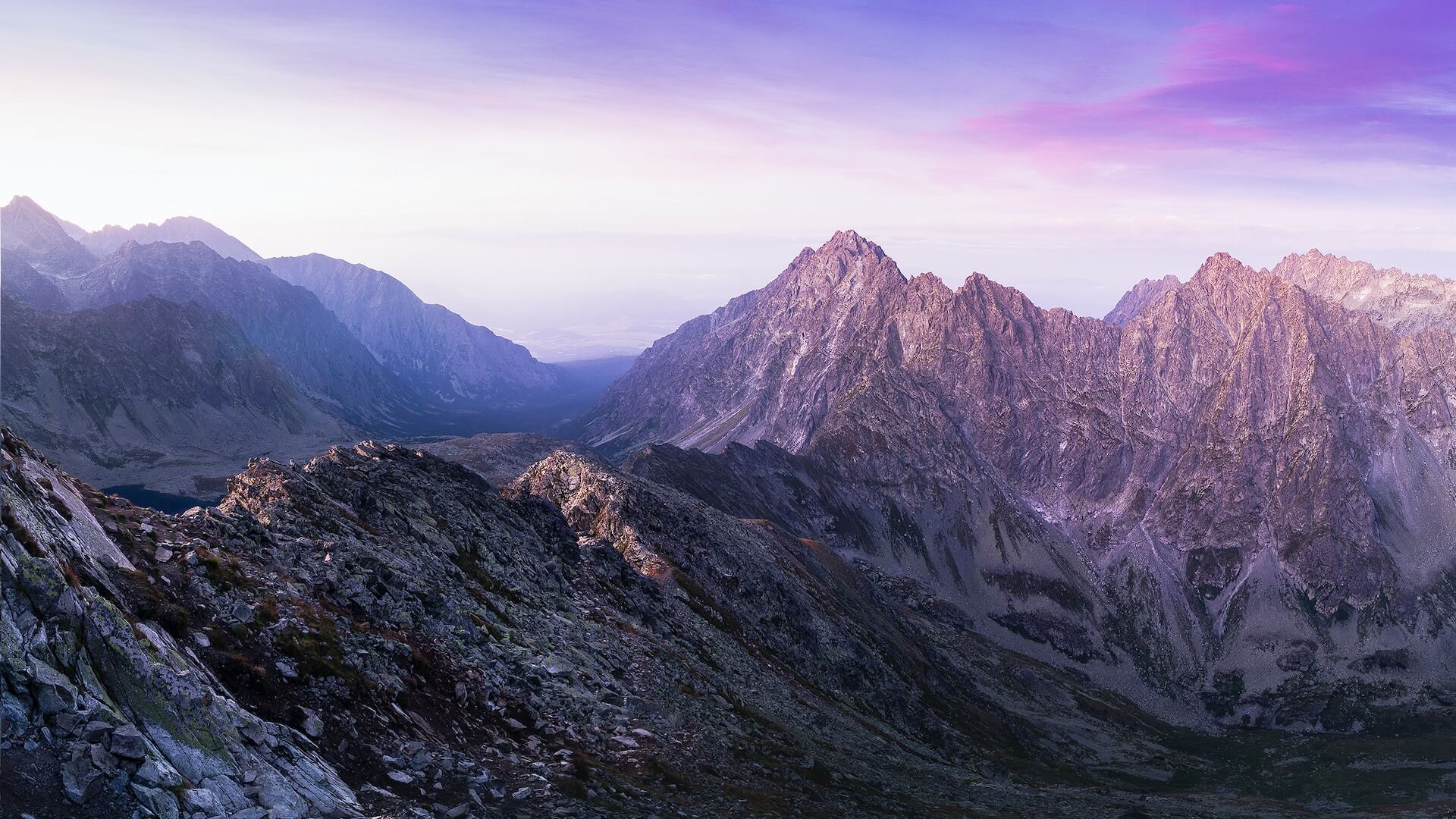
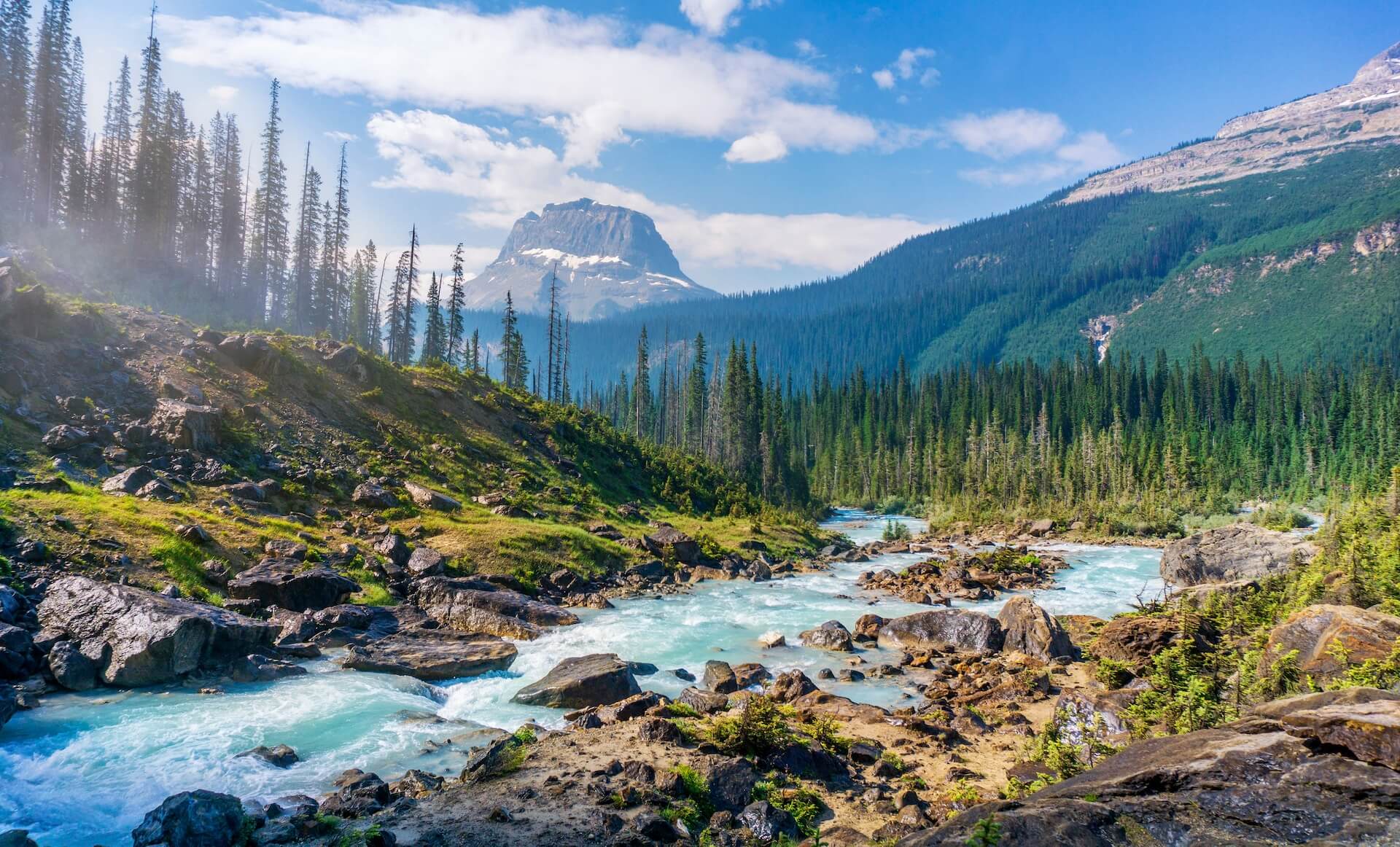
Copied !
import { CarouselGallery } from "$components/gallery.component";
/**
* @type {import("$common/props").JSXComponent<{ images: import("$components/image.component").ImageType[]}>}
*/
export function ZoomCarouselGalleryExample(props) {
const { images } = props;
return <CarouselGallery images={images} zoom />;
}